Animating a Scrolling Display
00:00
Animating a scrolling display. A static table is fine for displaying static data, but what if you want to display dynamic data in real time? Rich has a class named .Live
that helps you to do this.
00:14
.Live
is a context manager that takes control of the console formatting, allowing you to update whatever field you like without disturbing the rest of the layout.
00:25 For this live demo, you’ll make use of some real cryptocurrency data captured from a free crypto API to keep the demo focused on the display aspects. You’ll use canned data to simulate real-time updates.
00:38 There are a hundred entries that you’ll present in a table, only 16 rows deep. To do this, you’ll scroll the data through the table in an endless loop, stimulating the continuous arrival of new data.
00:51 In a real application, you might be getting real-time updates from an API, which you’d then add to the bottom of the table while allowing the older data to scroll off the top.
01:01 The overall visual effect would be very similar to the demo that you’ll be coding here. Since the crypto data is a little bulky, you’ll put it in a separate JSON file.
01:12 Typing this out would be rather long-winded, so make sure you’ve downloaded the course materials and make use of the included file, which is seen on screen.
01:22 This JSON file contains a single large data structure, a list of dictionaries, each containing the named fields of interest. The real API reports more than 2000 symbols, but these 100 will be enough for the demo.
01:39
Next, you’ll write the module live_table.py
containing the code to display the dynamic table. This code will read the data from the crypto_data.json
file you’ve just seen.
01:54
First, the relevant imports are performed, most of which you’ll have seen before, but with the addition of Live
from rich.live
,
02:17
the new module contains a single main function make_table()
. This function uses Rich’s Table
class to generate a formatted table containing a section of the data.
02:45 Much of this code will look familiar from the static table code you saw in the last video and for good reason only. The details are different for the most part,
03:39
but there is one embellishment compared to the code you saw before. These lines provide conditional formatting for the PCT change
field, depending on whether it’s greater than 5% or less than 5%.
04:16 The data is read from the JSON file creating the list of dictionaries that will be passed into the table.
04:30
Here you repeat the data in order to avoid complicated logic. At the end of the dataset, the num_lines
variable determines the number of lines in the displayed table.
04:41
The animation magic happens when you invoke the .Live
context manager. The optional screen=True
parameter enables a nifty feature of .Live
.
04:51
The original text display is saved and the live text appears on an alternate screen. The effect of this is that your program will seamlessly restore the original display when the function returns and exits the .Live
context.
05:04
The first parameter passed to .Live
is the table created by make_table()
. The program will call this function each time it updates the display. On its first call, make_table()
receives the first num_lines
row of coin data.
05:20
In the subsequent calls wrapped in .live.update()
, the data progressively scrolls using the index value as the starting point.
05:36 To simulate streaming data, you slice the static data.
05:46
You use the modulo operator to restart at index zero when you’ve shown all the available data in the table. Notice that the .live.update()
code occurs within an infinite loop.
05:57
When you’ve seen enough of the scrolling table, you can interrupt the code by pressing Ctrl+C
. The interruption is caught by the suppress
context manager, which exits the loop, and the .Live
context manager stops the animation and returns cleanly to the previous display.
06:12 You can invoke the table demo from the console
06:22
on screen. You can see the scrolling table. If you’d like to see a different table height, then you can modify num_lines
in the code or even make it a parameter for the script.
06:33 There are many ways you can customize this code for your particular use case, but even with no significant changes, you have a table which will present relevant information to the user in an attractive manner.
06:47 In the next section of the course, you’ll take a look back at what you’ve learned.
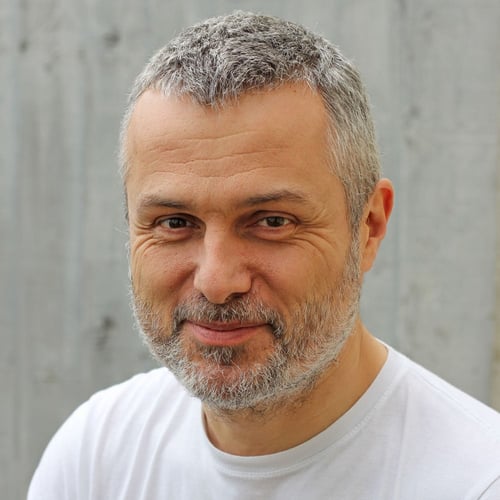
Darren Jones RP Team on Feb. 29, 2024
The first one that springs to mind is that you can easily preserve the original console contents with screen=True
, and it avoids having to manually do the work that I’d imagine the alternate code you’ve created does. It’s certainly possible to re-create much of what Live
does without using it, but it provides convenience and simplicity, and it’s used by progress indicators and status indicators.
The documentation at rich.readthedocs.io/en/stable/live.html has more depth on this, as well as a couple of examples.
Become a Member to join the conversation.
maceww6438 on Feb. 24, 2024
I’m not seeing the importance of Live. I simplified the loops and replaced the calls to Live with console.print(make_table…) and got the same effect (except for a “clear screen” between prints).
Do you have another example?