Returning vs Printing
00:00 Now let’s take a look at the difference between returning a value and printing a value. It does seem like returning and printing are very similar operations.
00:12
It’s because they both do produce some type of result. These similarities are even more noticeable when working interactively. Let’s compare two functions that both provide the greeting "Hello, world"
.
00:27 One does this by printing the message, the other by returning it. Let’s go ahead and define this first function,
00:48 Since the function is designed to produce output, we see the greeting displayed. Now let’s do the returning version.
01:03
This one returns the greeting "Hello, world"
.
01:10
When I call this, the interpreter—once the function has finished executing—will display the return value, and we get the string 'Hello, world'
.
01:24
Notice, in this case, the quotes telling us that the return value is a string object. They didn’t appear in the print version, since we were telling Python to print a string and the print()
function doesn’t display the quotes. Well, that would suggest to us that the differences are even harder to detect when performing the same experiment with a number instead of a string.
01:49
Here we have functions that are printing and returning the number 42
. This first function prints the number 42
, and so when I call it, it carries out its one statement, which displays the output. Remember, it does return None
, but None
isn’t shown in the interpreter when that’s the return value.
02:16
And here’s our old friend return_42()
.
02:23
And again, when I call return_42()
, it only has the return
statement, which the interpreter then takes the return value and displays it.
02:35
So, the output’s identical, so does it really matter? Well, it matters when you’re writing scripts. Let’s take a look at this program. It defines a function to add two numbers, and then it calls that function to add 2
plus 2
.
02:52
The function returns, but does not display the result, which makes sense. And so later, we would like to perform an operation using that function, so we call it to add the numbers, 2 + 2
.
03:08
Now, if I just import the add()
function
03:15 and call it interactively, because we’re in the interpreter, the interpreter is going to run the function, get the return value, and display it for us. However, if I run the entire program by importing the entire module,
03:39
This entire program was executed. The add()
function was defined and I performed the add operation on 2
and 2
, but I didn’t have an output statement, so there is no output. I could even, if I wanted to, execute this from the command line.
04:00 And again, the entire program is run and with no output statement, there’s nothing to display. In a script, if I want something displayed, it must use a print statement.
04:16 I don’t want to put the print statement in the function. We’ll be using this function in many different contexts, and we won’t always want the sum displayed when it’s called.
04:27 So in this particular execution of the program, the intent is to display the result of this function call. It’s down here, where we want to include the print statement.
04:43 So we add the print statement and the function call where we actually want the result displayed. And now if I run this program, I do indeed see the result.
04:59
And if I did this interactively by importing the entire module, the program is run and I do get the output of 4
. Since in practice we do much more with scripts than we do interactively, we can’t count on the interpreter to display results for us, so be sure to use a print statement anytime you want something output.
05:27
Now that we understand the role of the return
statement, we can start exploring the different ways it can be used. First, we’ll look at how you can return multiple values in a single return
statement.
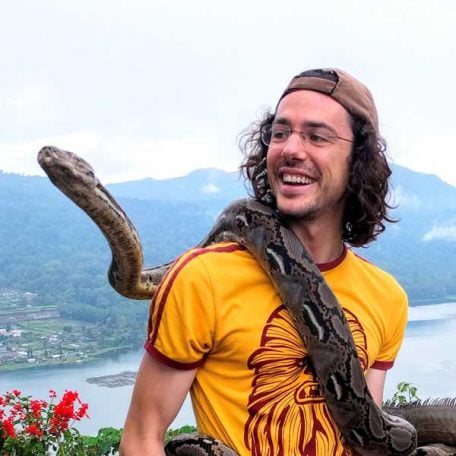
Martin Breuss RP Team on Aug. 25, 2021
Hi @Ricardo.
Yes, if you want to import adding
to use add()
in a different script, then you’d want to remove the print()
call from where Howard put it in this lesson.
There’s a way to keep print()
calls like this one around for demonstration purposes, but avoid having them called when you import the script as a module. Check out how to Use if __name__ == "__main__"
to Control the Execution of Your Code for more info on that.
Ricardo Joseh Lima on Aug. 25, 2021
Hi thanks for the comment and for the suggestion of the course, will surely put it on top of the list.
Become a Member to join the conversation.
Ricardo Joseh Lima on Aug. 24, 2021
In the same way that it is not a good idea to place print inside a function, isn’t it also not a good idea to place print in the file where the function is? Because doing so, when importing the function to use in another case (3,3), one will get 4 and 6 (4 from (2,2) of the function where the function is) and 6 from calling the function in another file, is that correct?