Functional Programming With Python
Learning Path ⋅ Skills: Python, Functional Programming, Lambda Functions, Built-in Functions, Map, Filter, Reduce, Scope, Decorators, Recursion, Code Efficiency, Readability
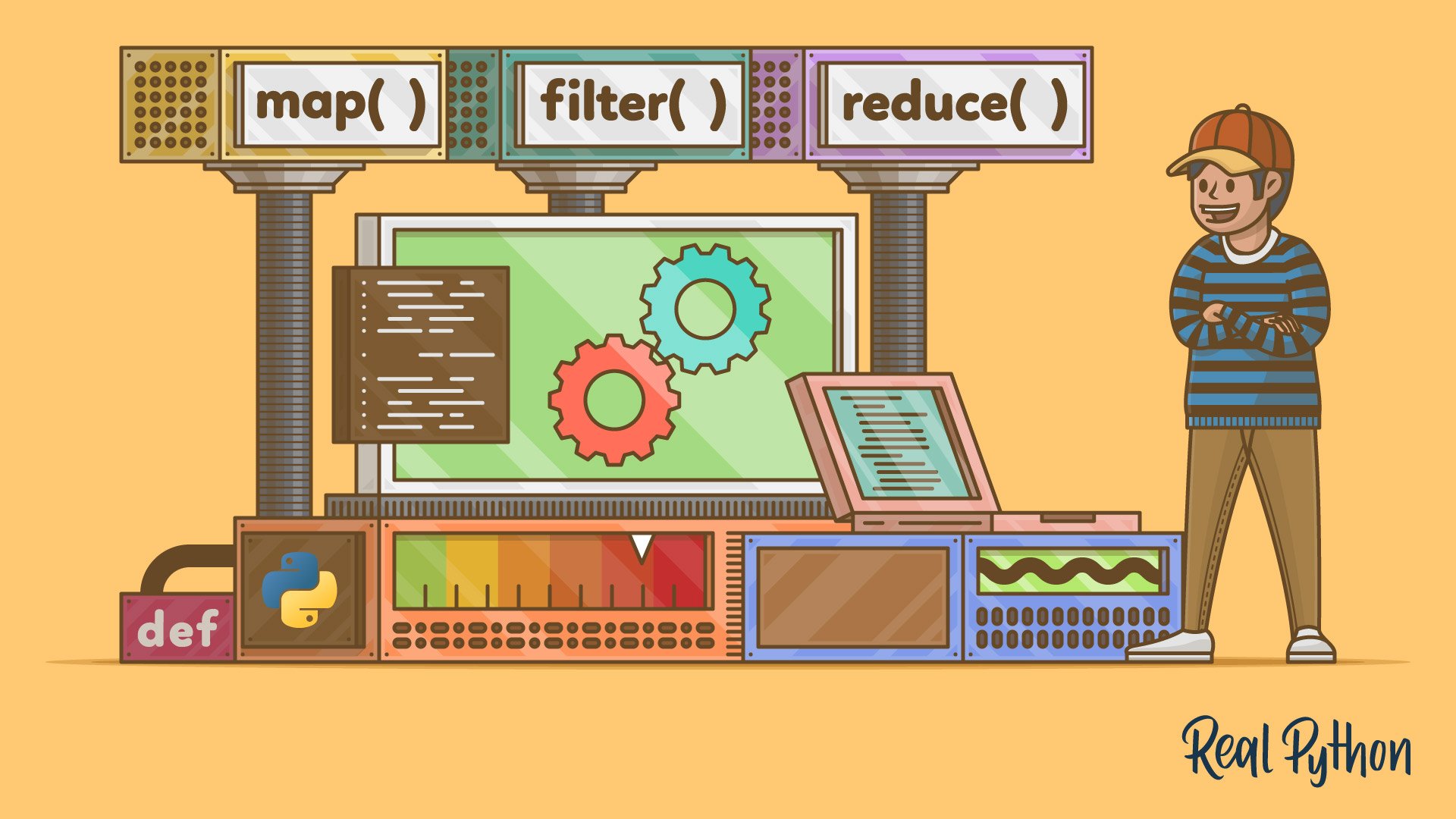
Unlock the power of functional programming in Python! From lambda functions to built-in functions like map()
and filter()
, and advanced topics like closures, decorators, and recursion. This path will elevate your coding skills and make your code more efficient and readable.
Functional Programming With Python
Learning Path ⋅ 13 Resources
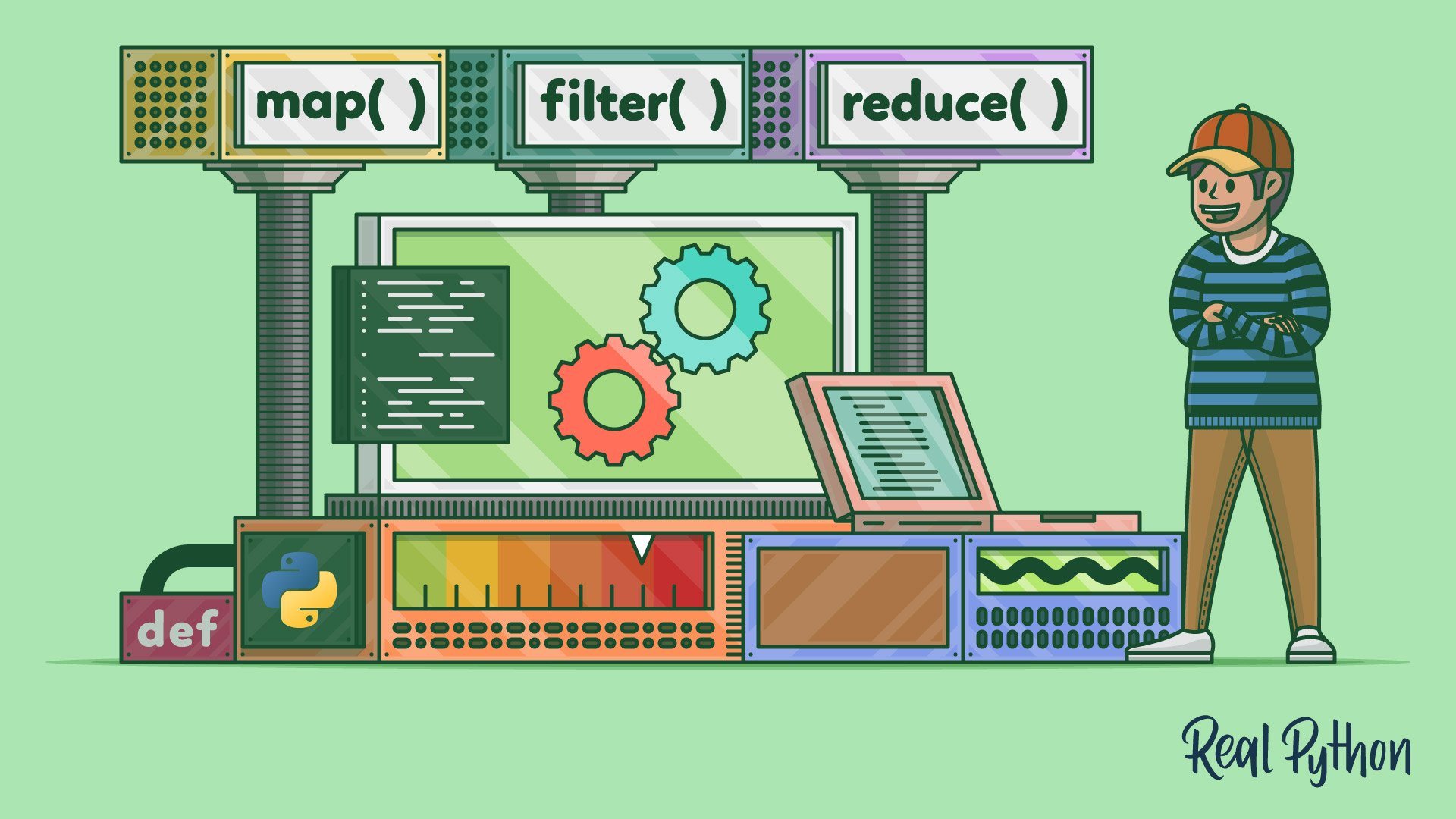
Tutorial
Functional Programming in Python: When and How to Use It
Learn about functional programming in Python. You'll see what functional programming is, how it's supported in Python, and how you can use it in your Python code.
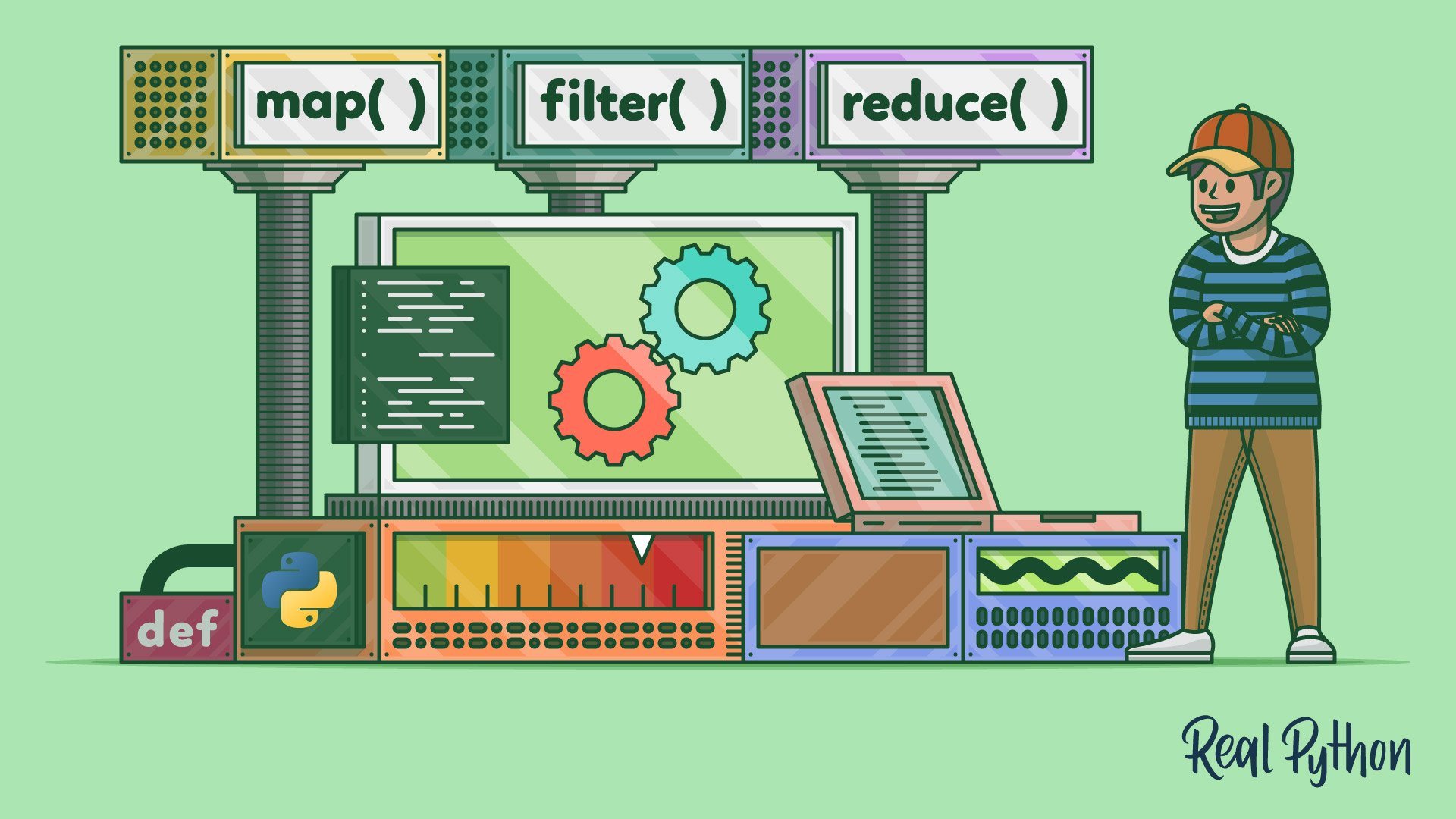
Interactive Quiz
Functional Programming in Python: When and How to Use It
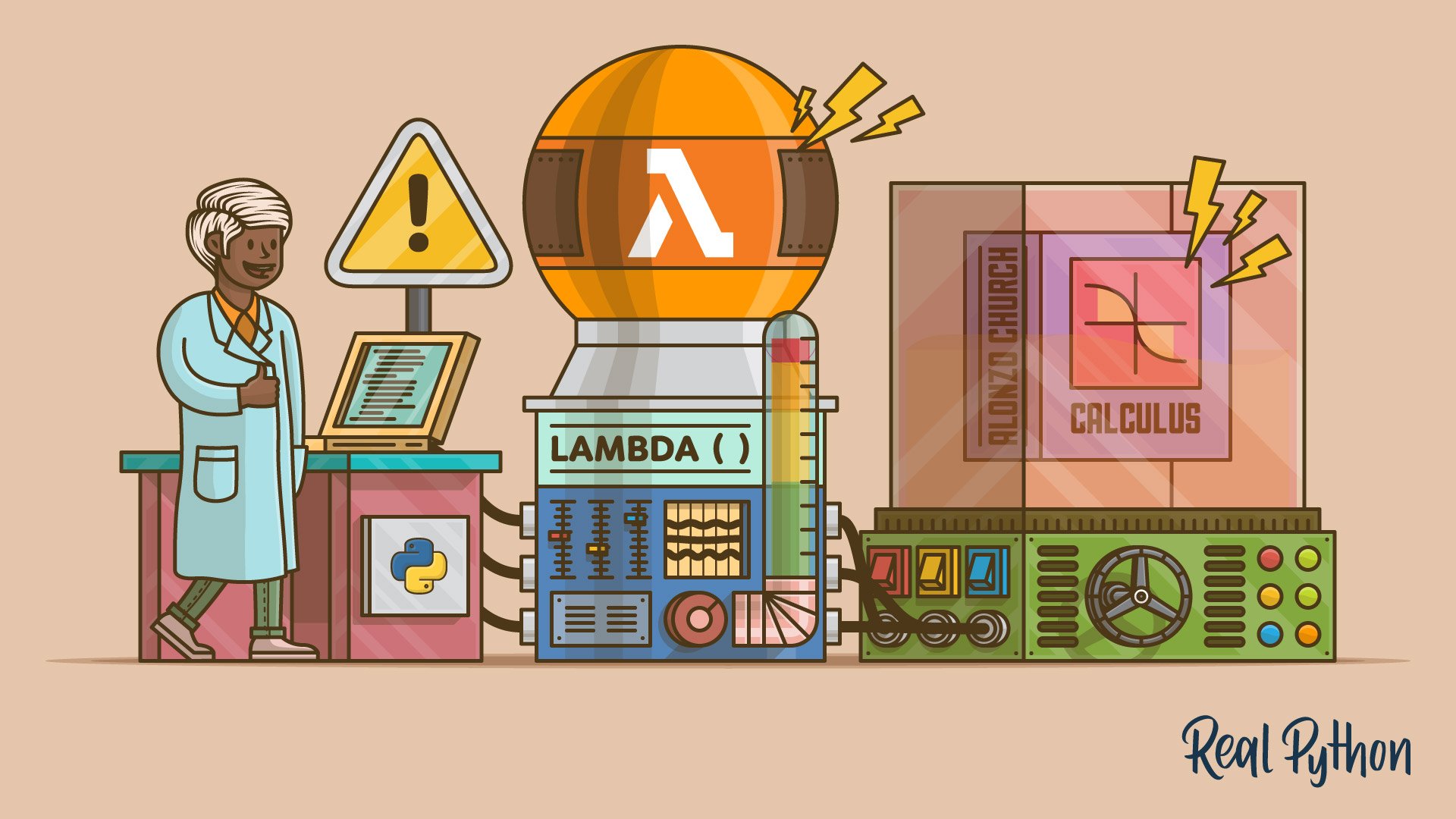
Course
Using Python Lambda Functions
Learn about Python lambda functions. You'll see how they compare with regular functions and how you can use them in accordance with best practices.
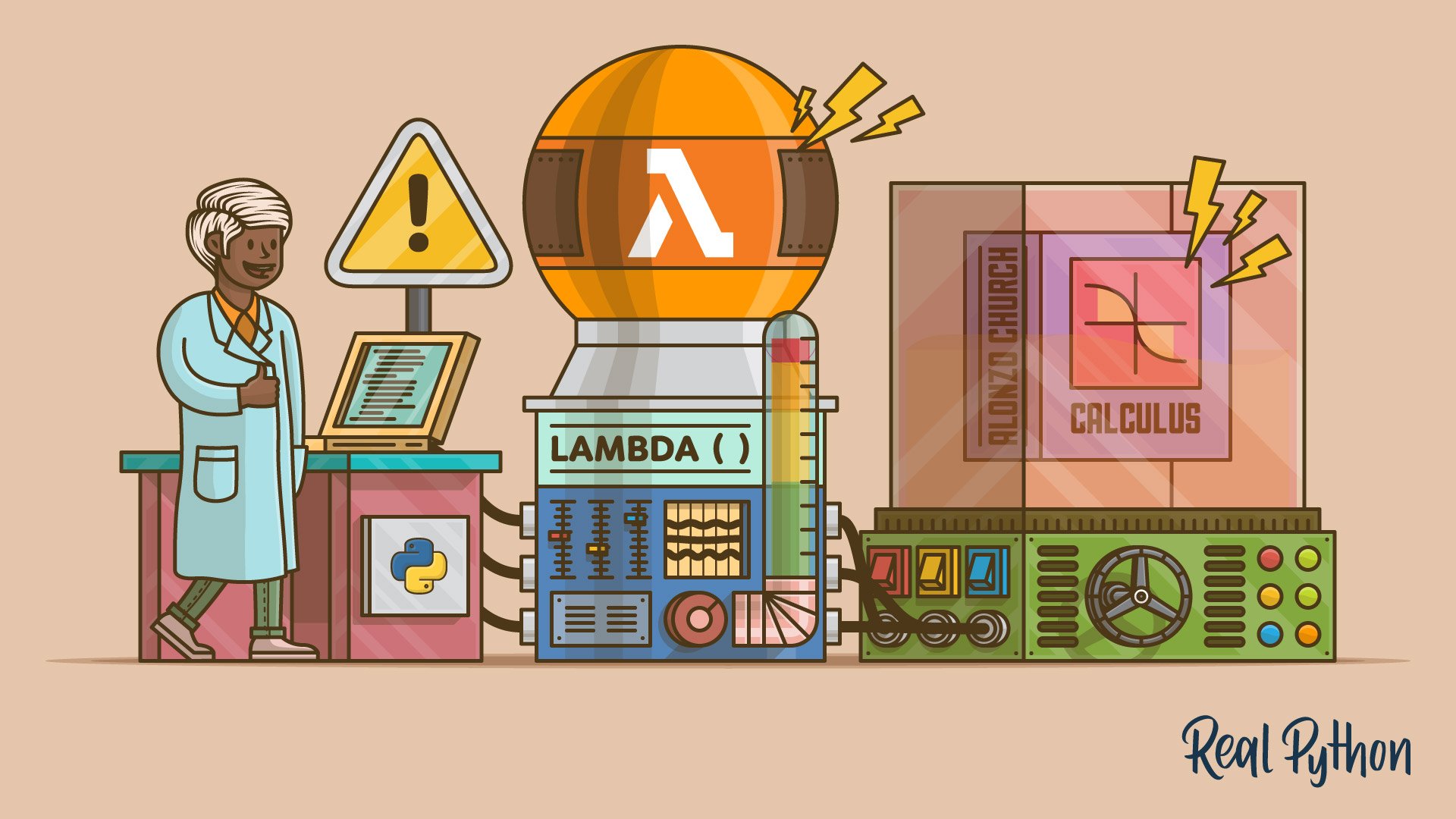
Interactive Quiz
Python Lambda Functions
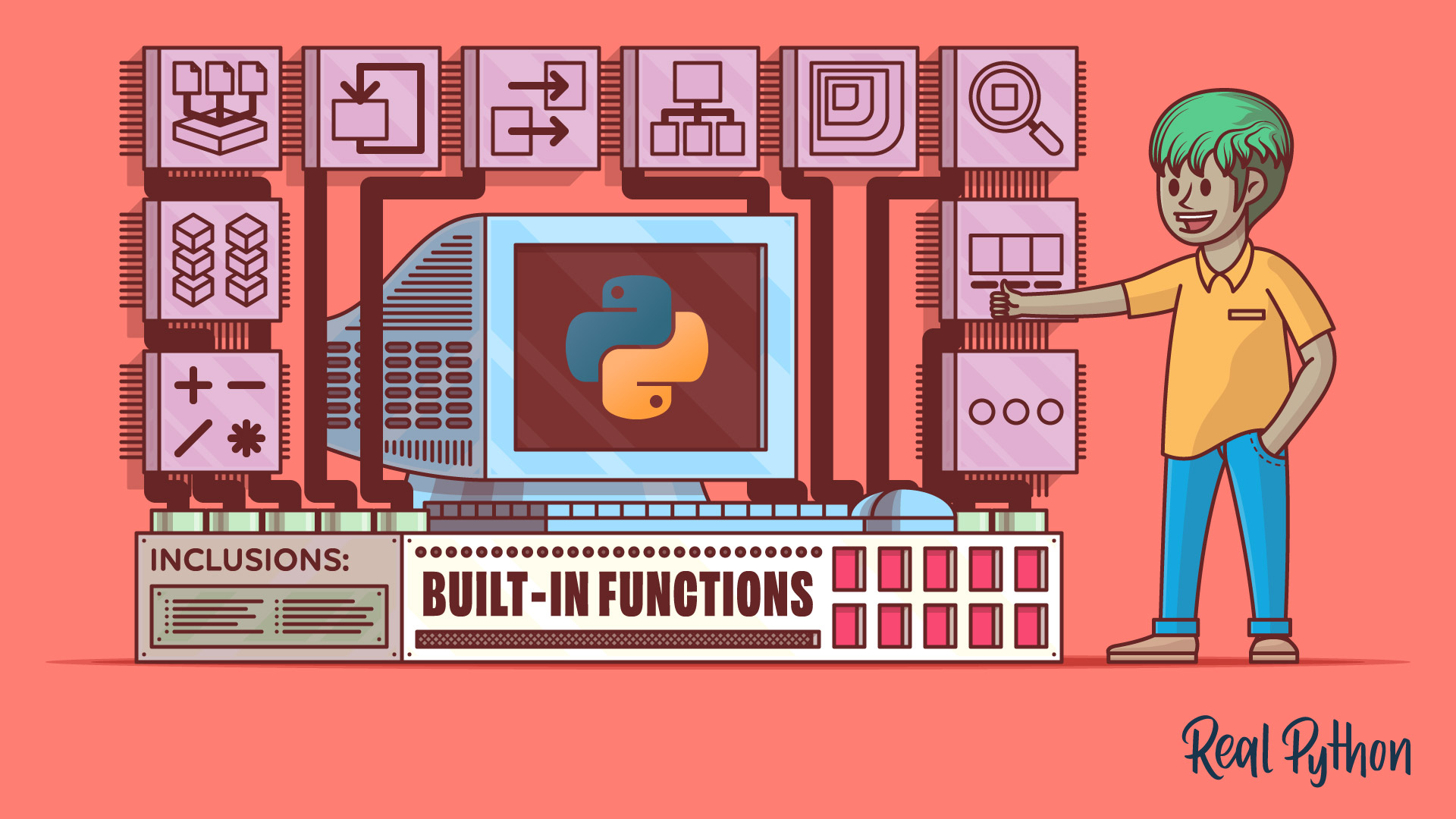
Tutorial
Python's Built-in Functions: A Complete Exploration
Learn the basics of working with Python's numerous built-in functions. You'll explore how to use these predefined functions to perform common tasks and operations, such as mathematical calculations, data type conversions, and string manipulations.
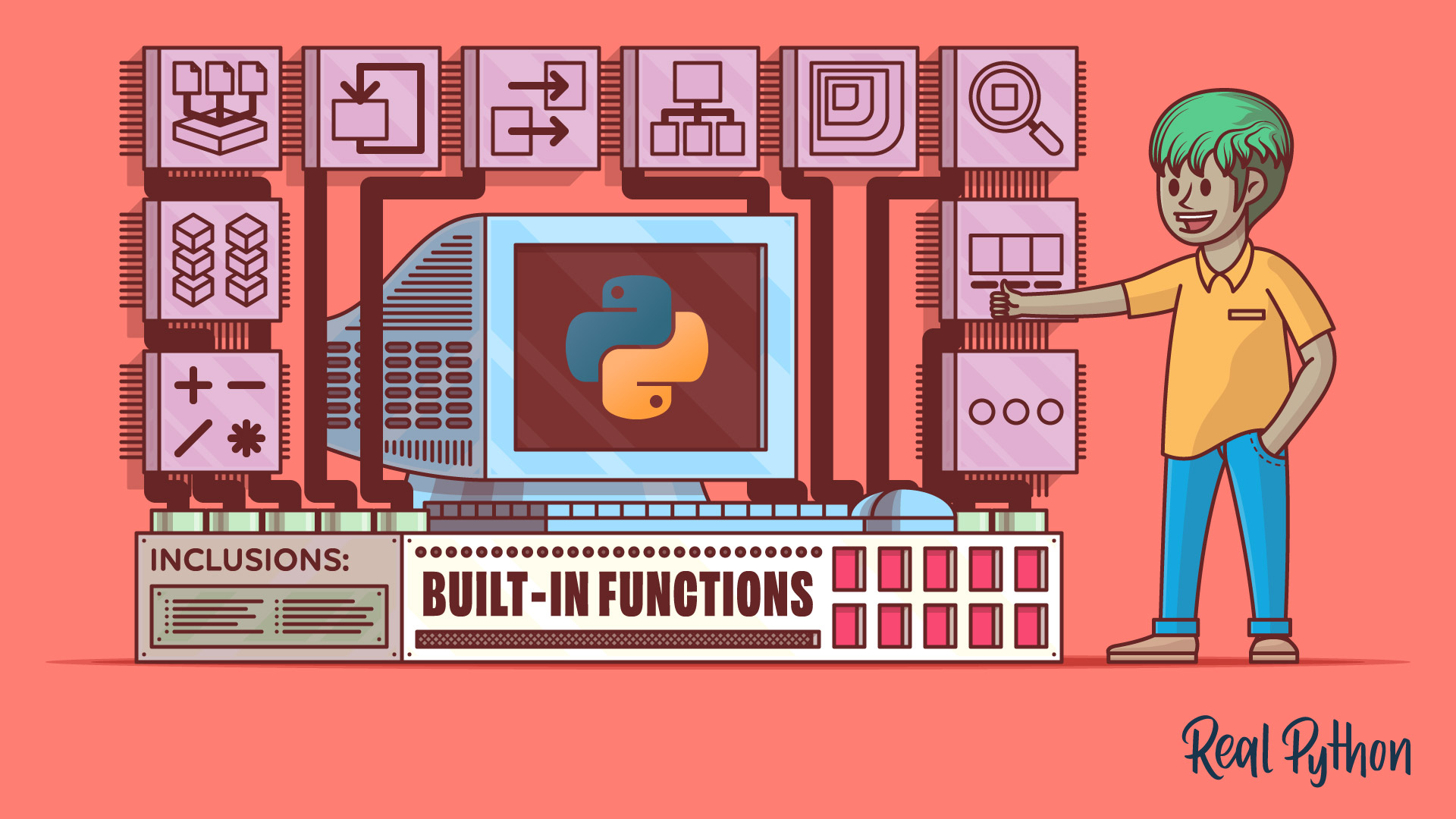
Interactive Quiz
Python's Built-in Functions: A Complete Exploration
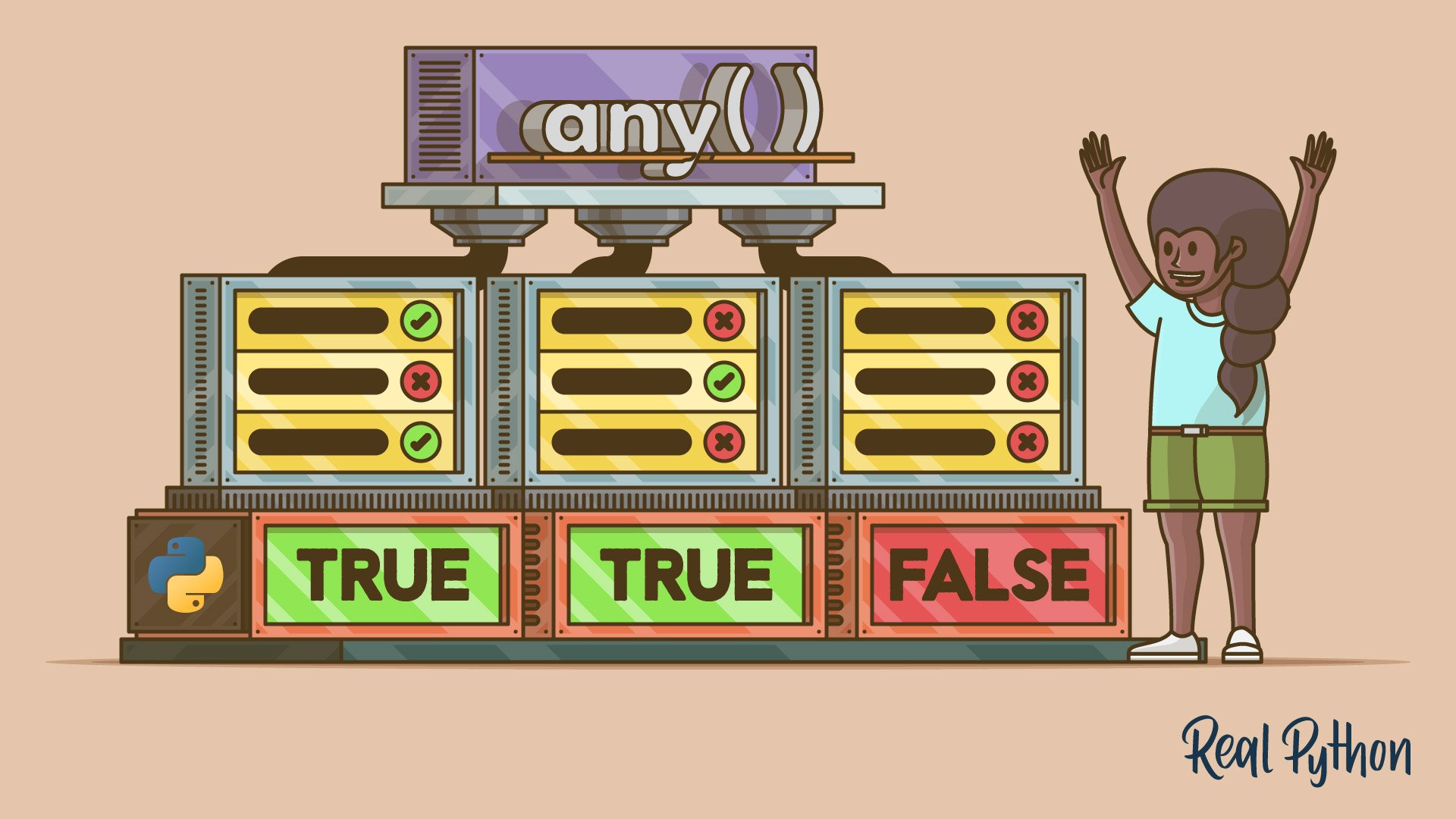
Course
Python any(): Powered Up Boolean Function
If you've ever wondered how to simplify complex conditionals by determining if at least one in a series of conditions is true, then look no further. This video course will teach you all about how to use any() in Python to do just that.
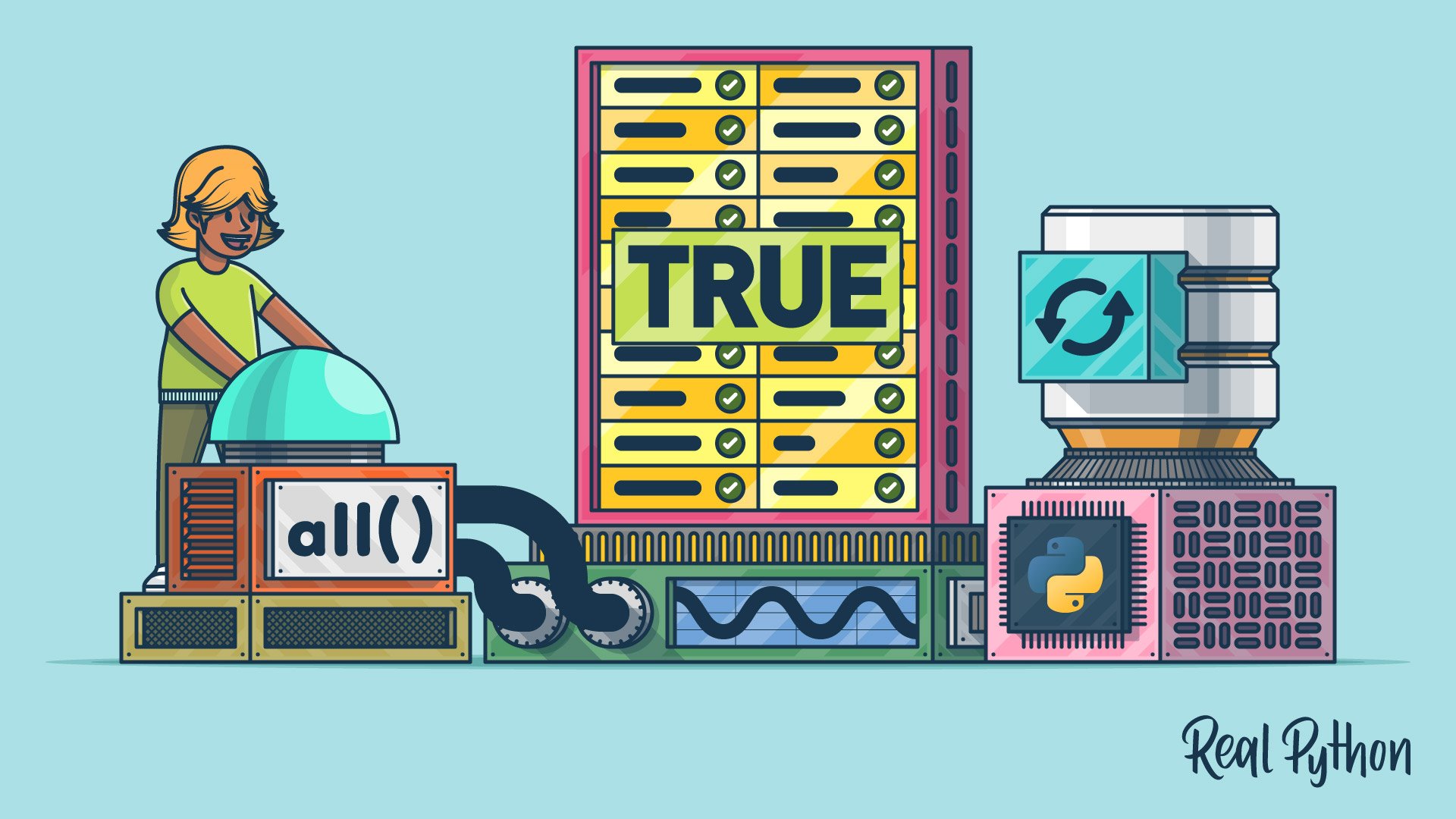
Tutorial
Python's all(): Check Your Iterables for Truthiness
Learn how to use Python's all() function to check if all the items in an iterable are truthy. You'll also code various examples that showcase a few interesting use cases of all() and highlight how you can use this function in Python.
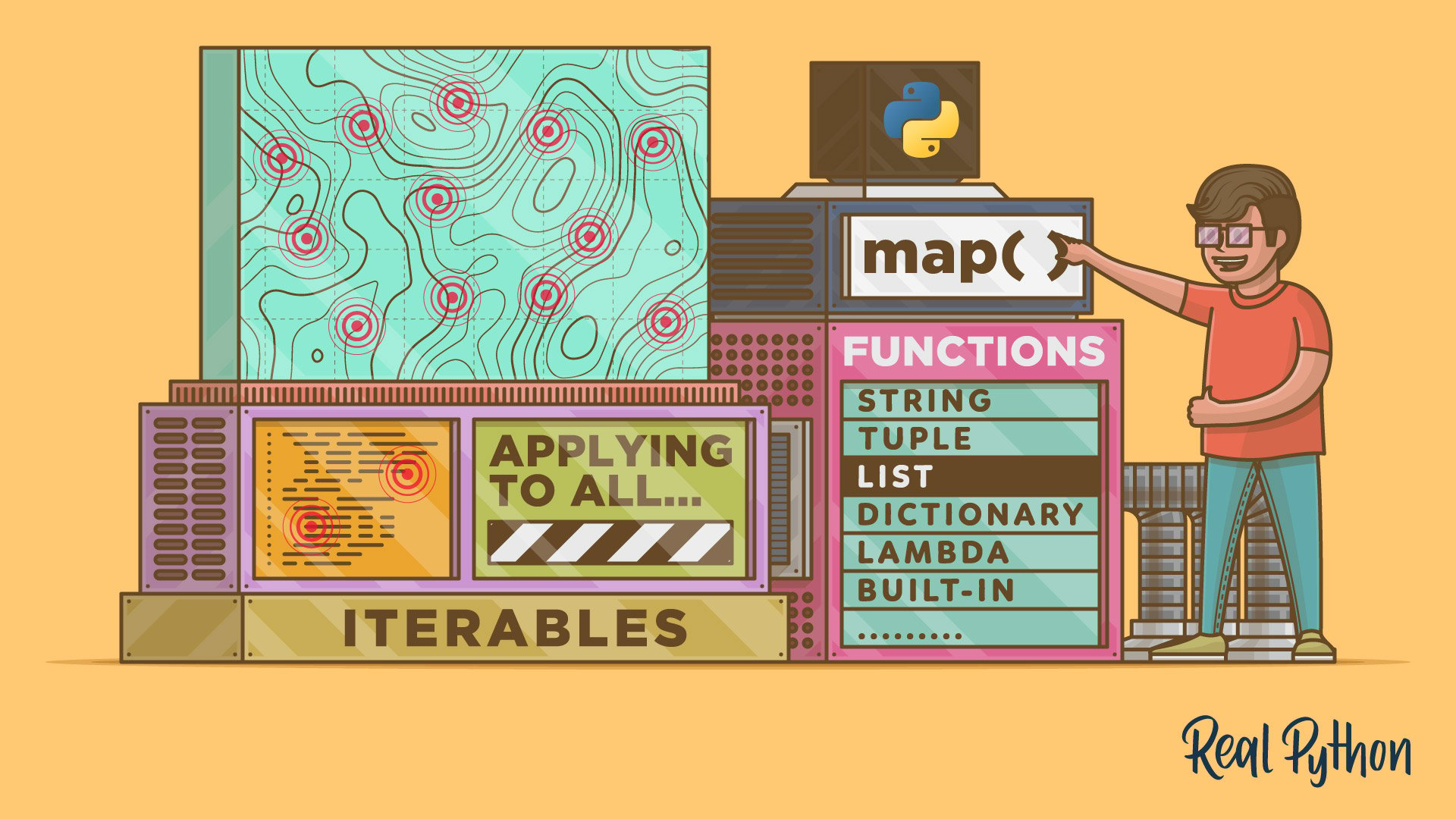
Course
Python's map() Function: Transforming Iterables
Learn how Python's map() works and how to use it effectively in your programs. You'll also learn how to use list comprehension and generator expressions to replace map() in a Pythonic and efficient way.
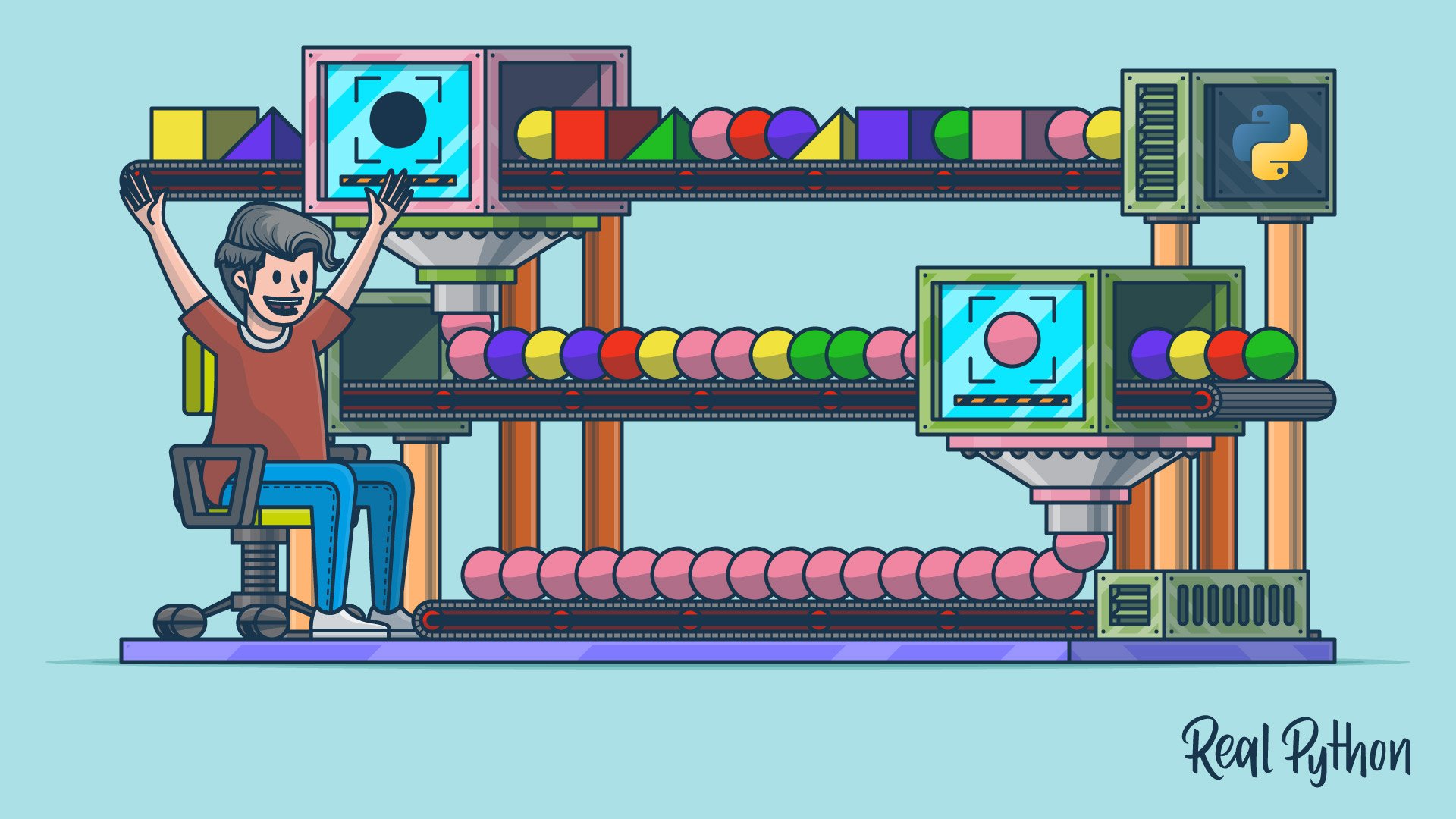
Course
Filtering Iterables With Python
Learn how Python's filter() works and how to use it effectively in your programs. You'll also learn how to use list comprehension and generator expressions to replace filter() and make your code more Pythonic.
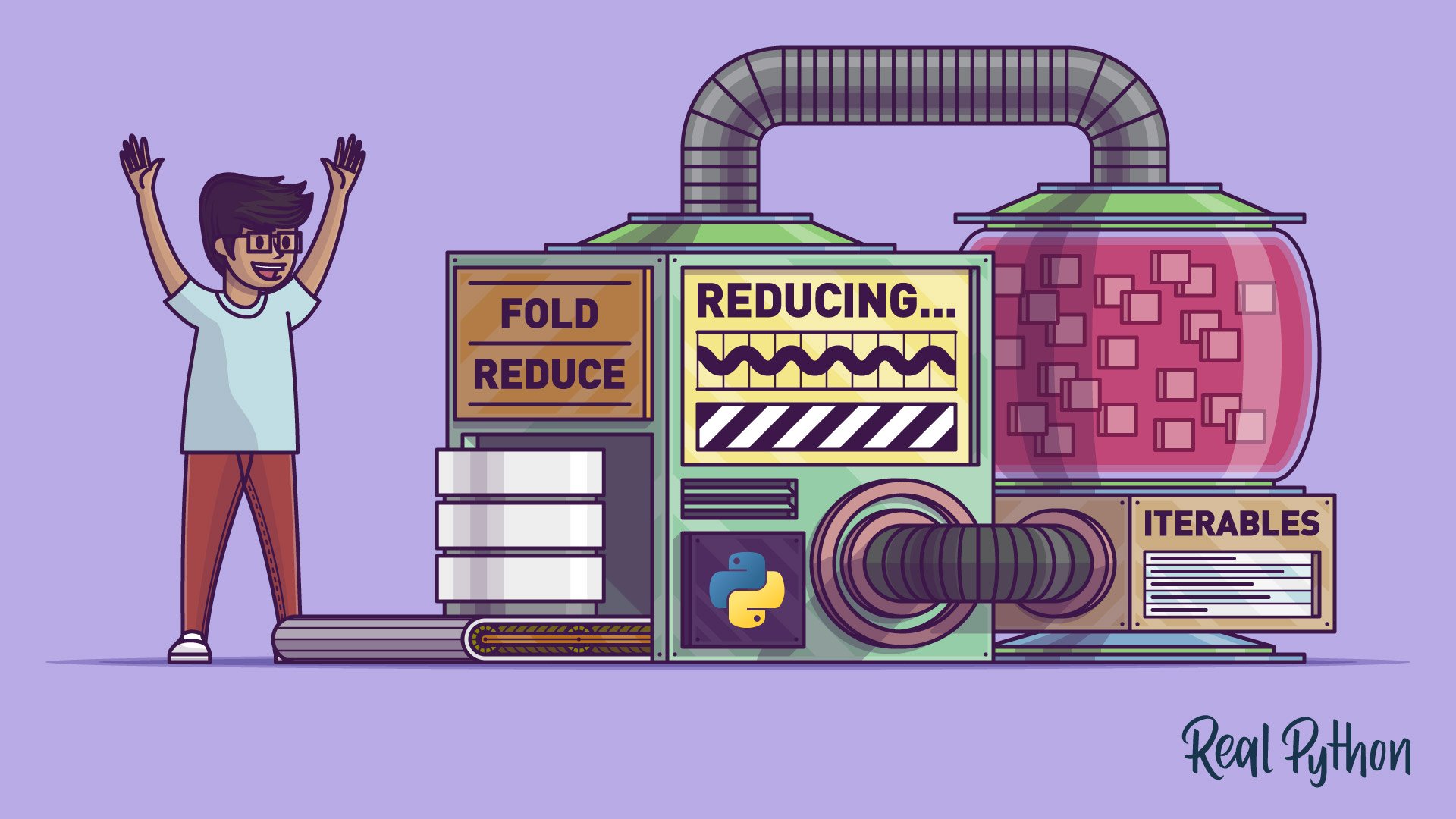
Tutorial
Python's reduce(): From Functional to Pythonic Style
Learn how Python's reduce() works and how to use it effectively in your programs. You'll also learn some more modern, efficient, and Pythonic ways to gently replace reduce() in your programs.
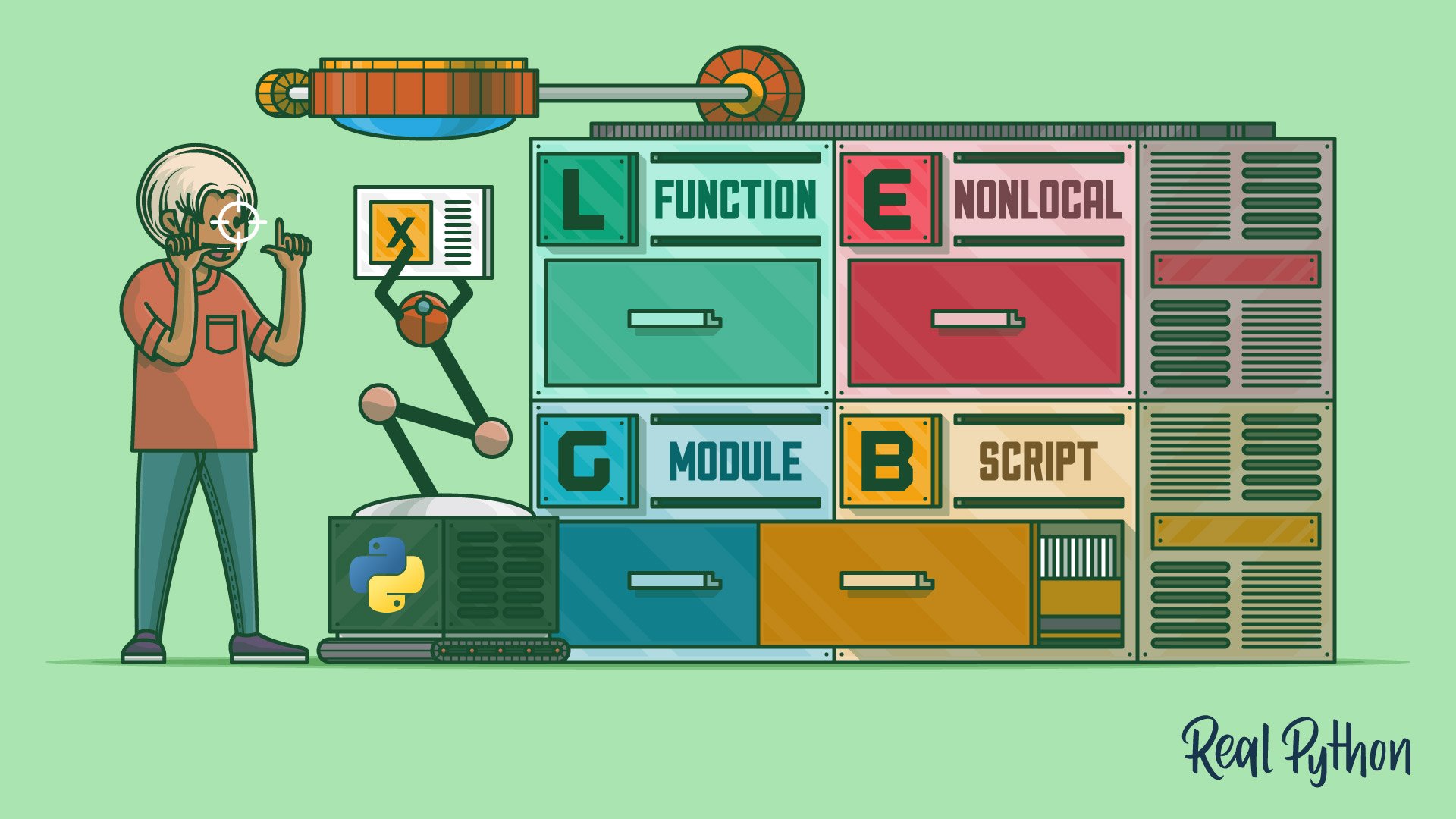
Course
The LEGB Rule & Understanding Python Scope
In this step-by-step video course, you'll learn what scopes are, how they work, and how to use them effectively to avoid name collisions in your code.
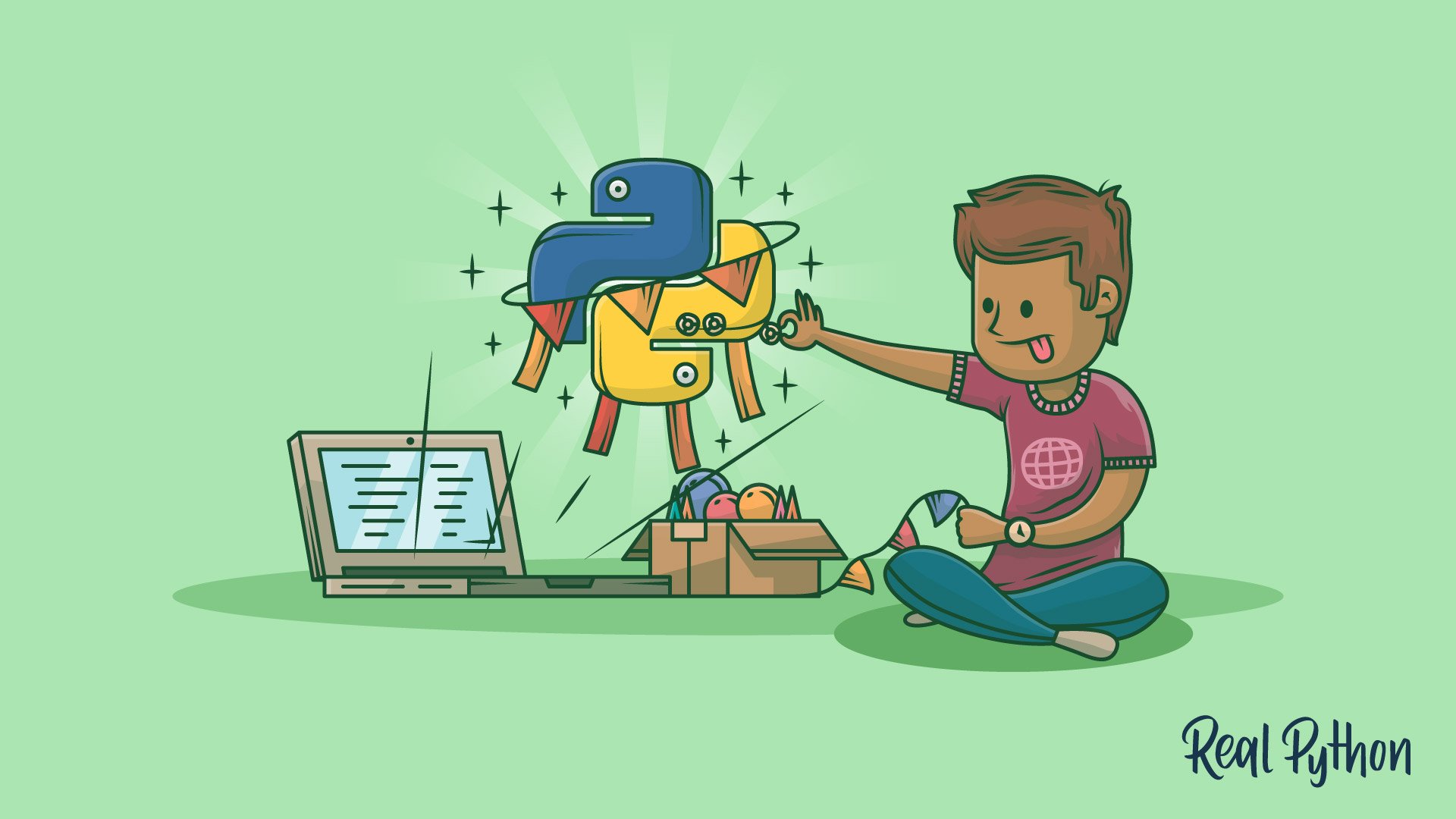
Course
Python Decorators 101
In this course on Python decorators, you'll learn what they are and how to create and use them. Decorators provide a simple syntax for calling higher-order functions in Python. By definition, a decorator is a function that takes another function and extends the behavior of the latter function without explicitly modifying it.
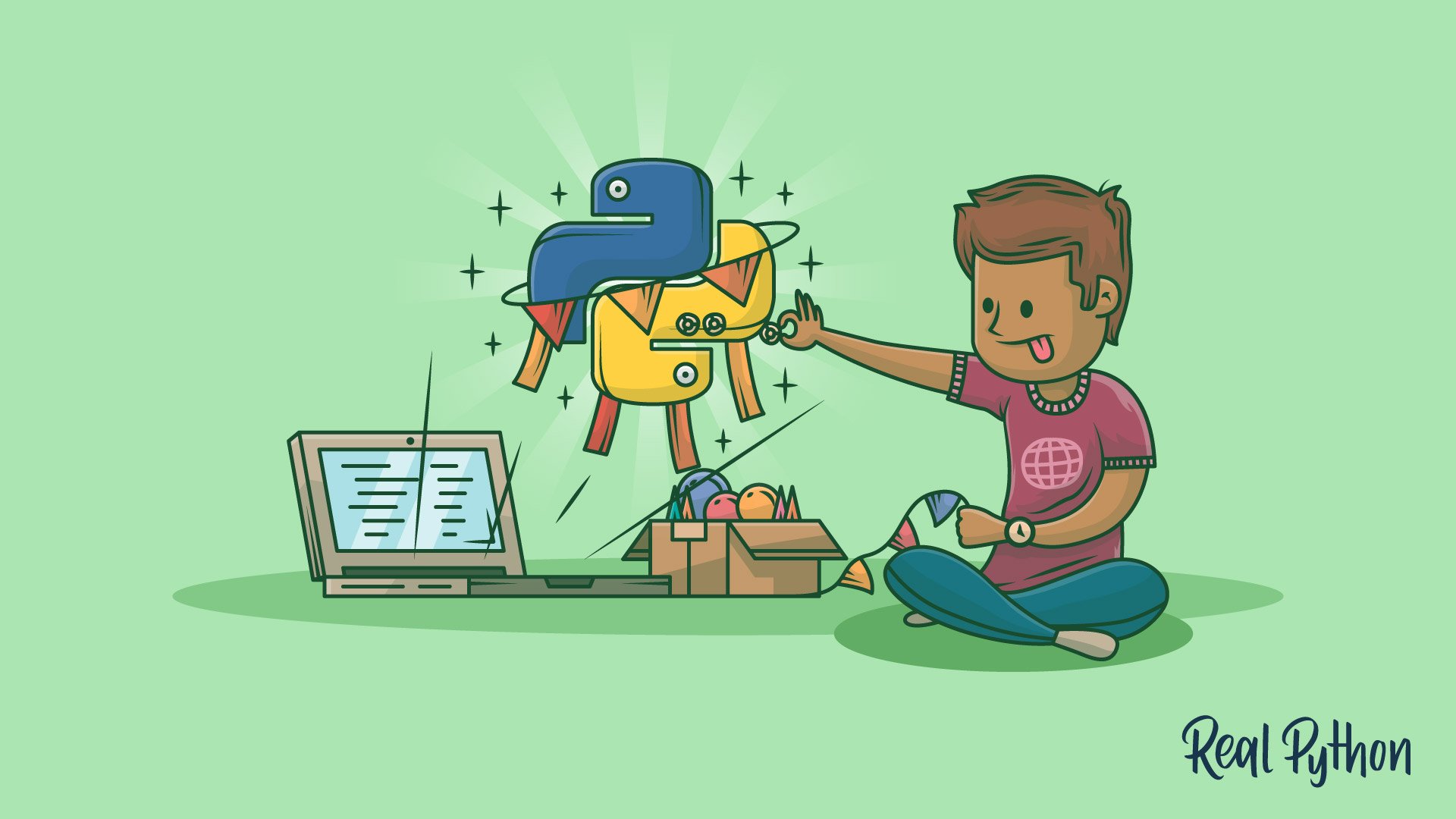
Interactive Quiz
Decorators
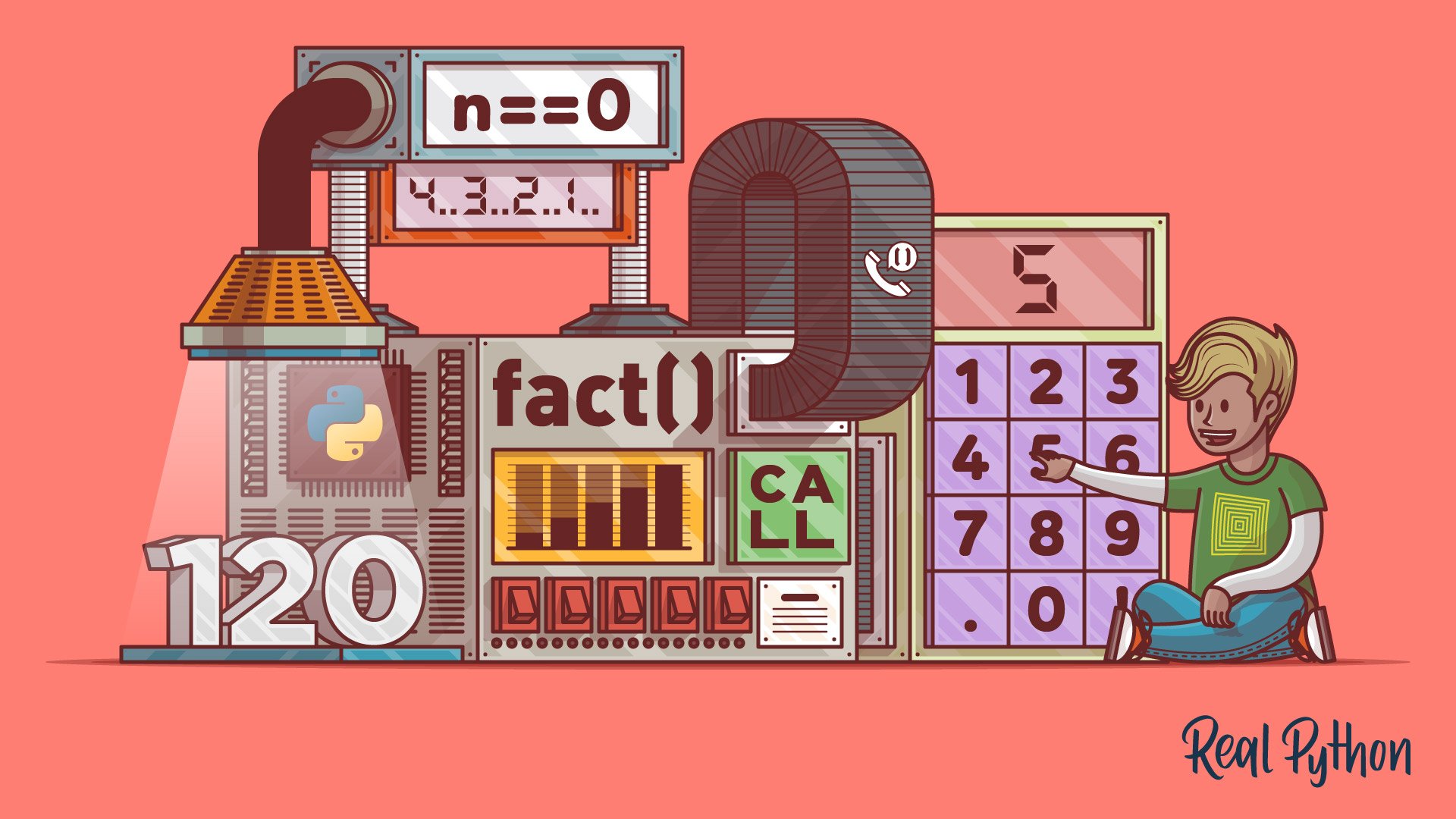
Course
Recursion in Python
A recursive function is one that calls itself. In this video course, you'll see what recursion is, how it works in Python, and under what circumstances you should use it.
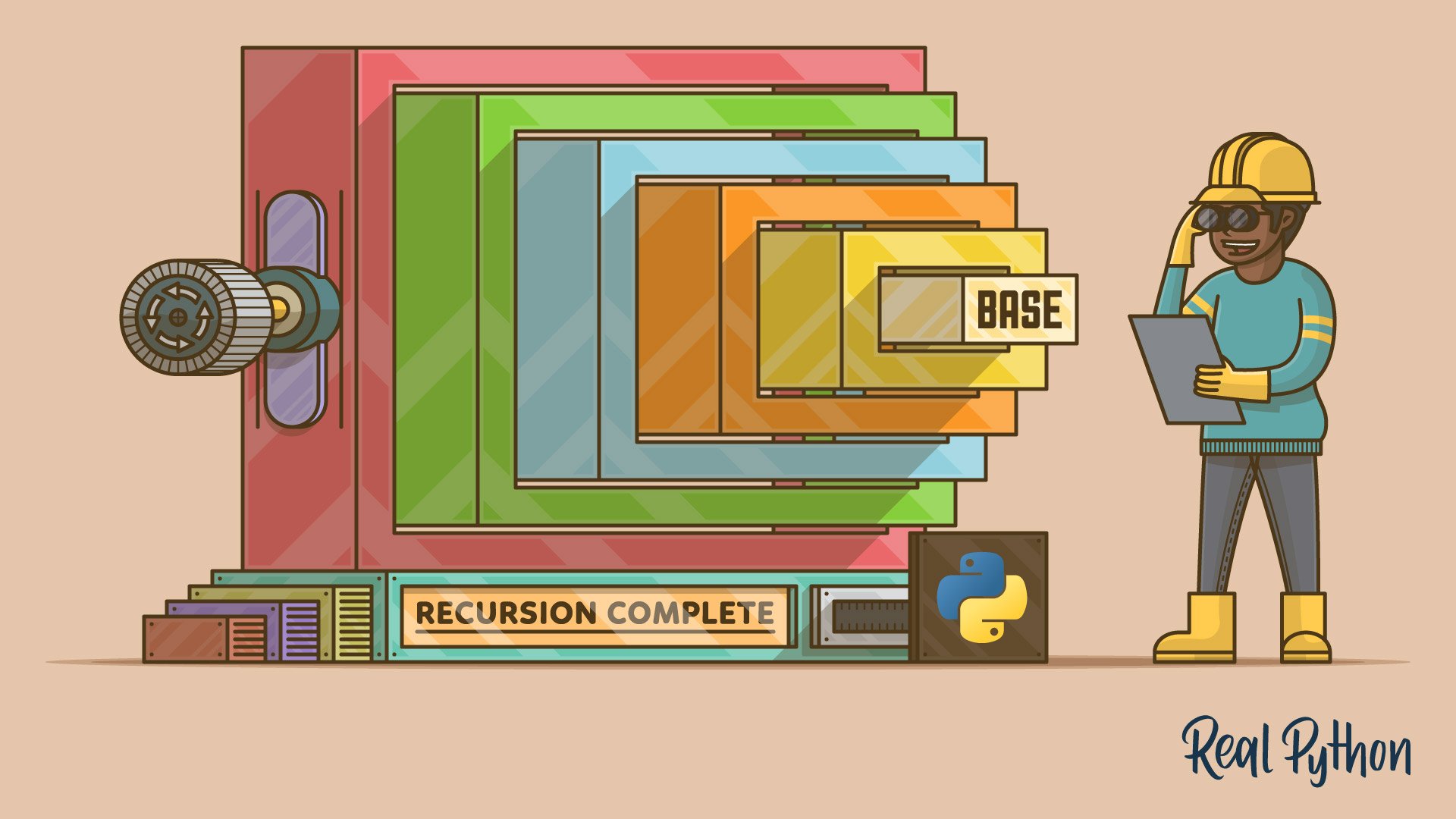
Course
Thinking Recursively With Python
Learn how to work with recursion in your Python programs by mastering concepts such as recursive functions and recursive data structures.
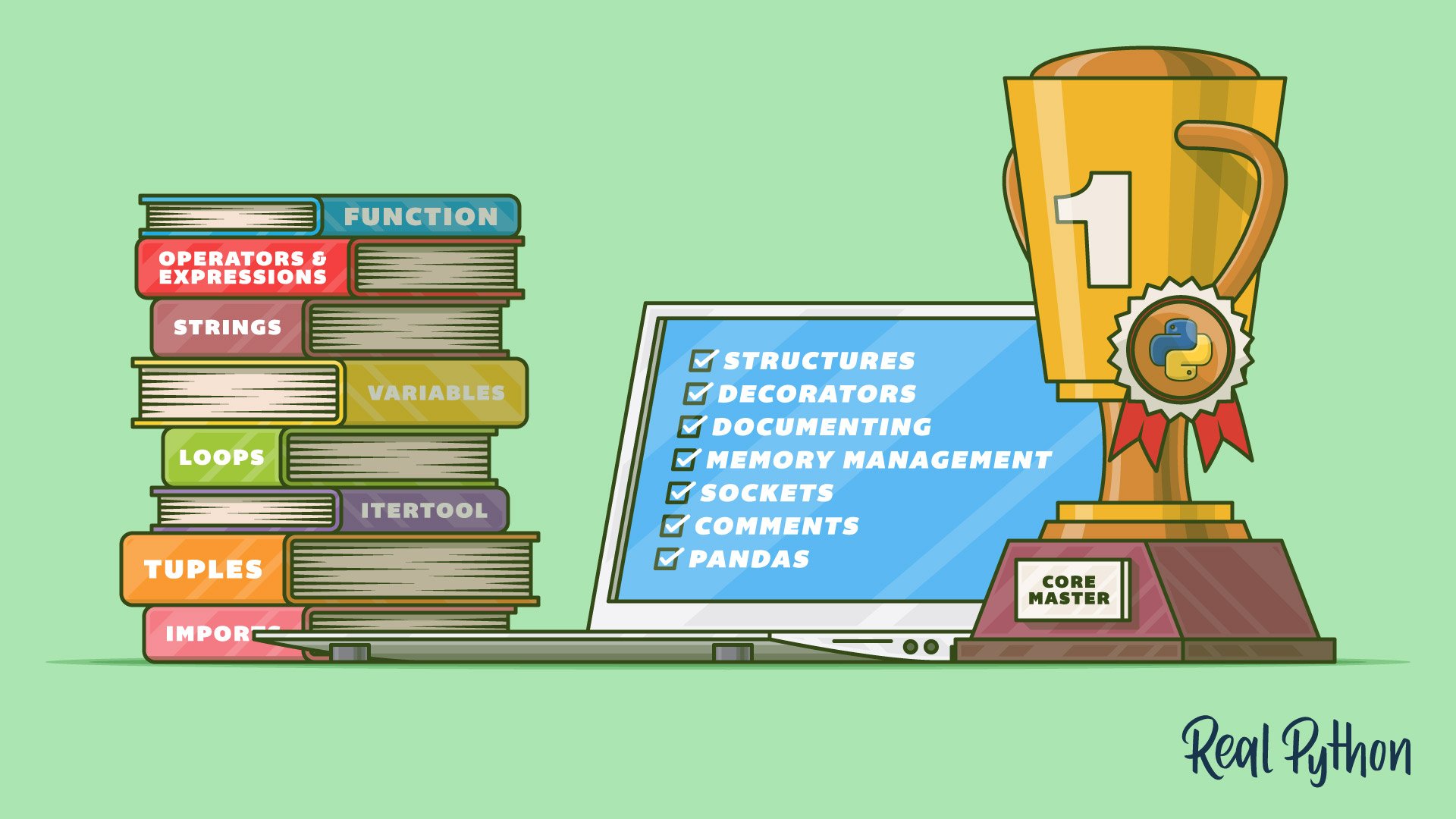
Course
Functional Programming in Python
Learn how to approach functional programming in Python. You'll cover what functional programming is, how you can use immutable data structures to represent your data, as well as how to use filter(), map(), and reduce().
Got feedback on this learning path?
Looking for real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session. Happy Pythoning!