Object-Oriented Programming (OOP)
Learning Path ⋅ Skills: Python, OOP, Classes, Data Classes, Getters, Setters, Property, super(), SOLID, Inheritance, Composition, Factory Pattern
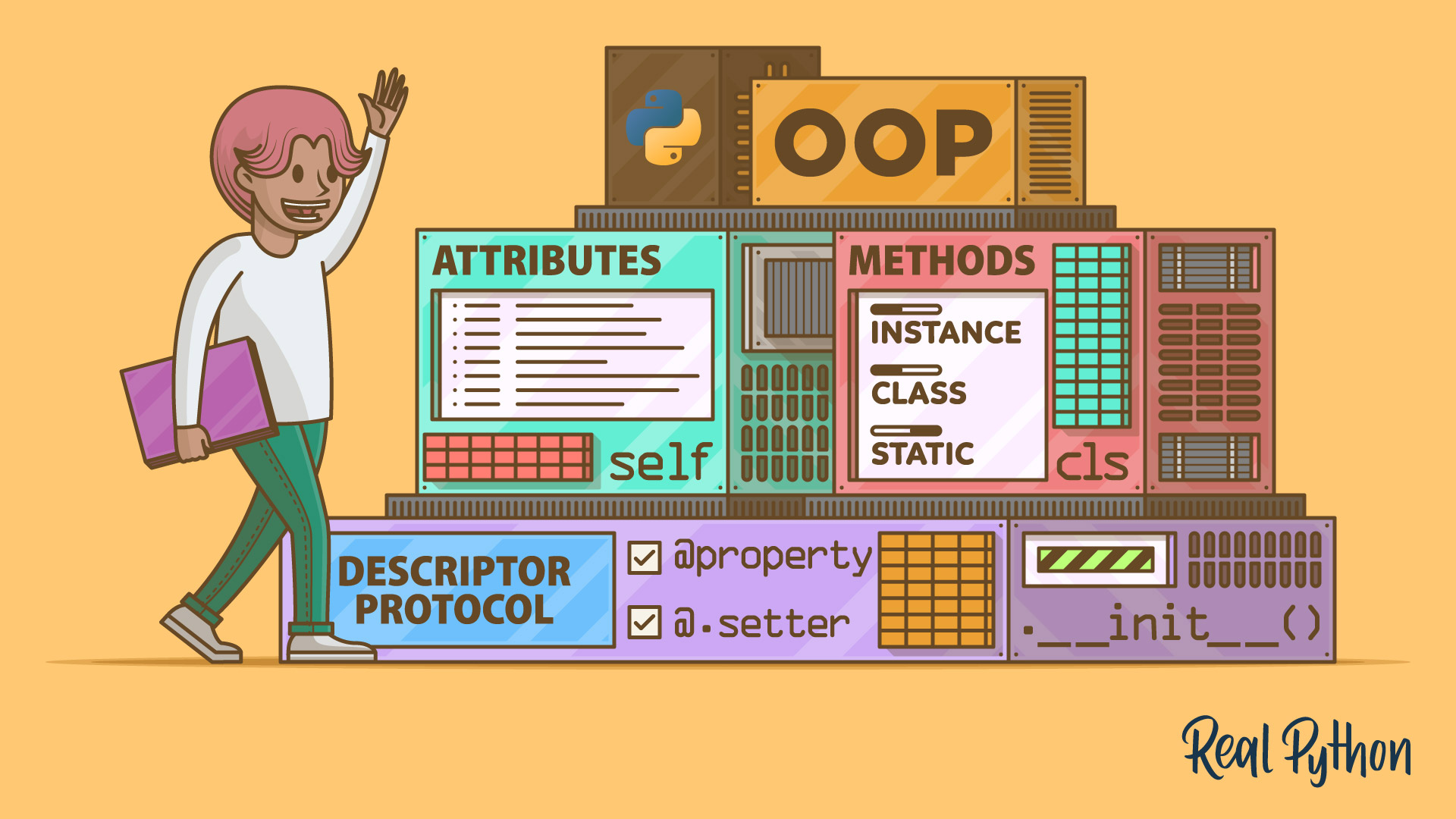
Dive into Python OOP! Learn everything from basic classes to advanced topics like super(), data classes, and design patterns. Enhance your coding with magic methods, managed attributes, and SOLID principles. Start building robust, scalable applications today!
Object-Oriented Programming (OOP)
Learning Path ⋅ 14 Resources
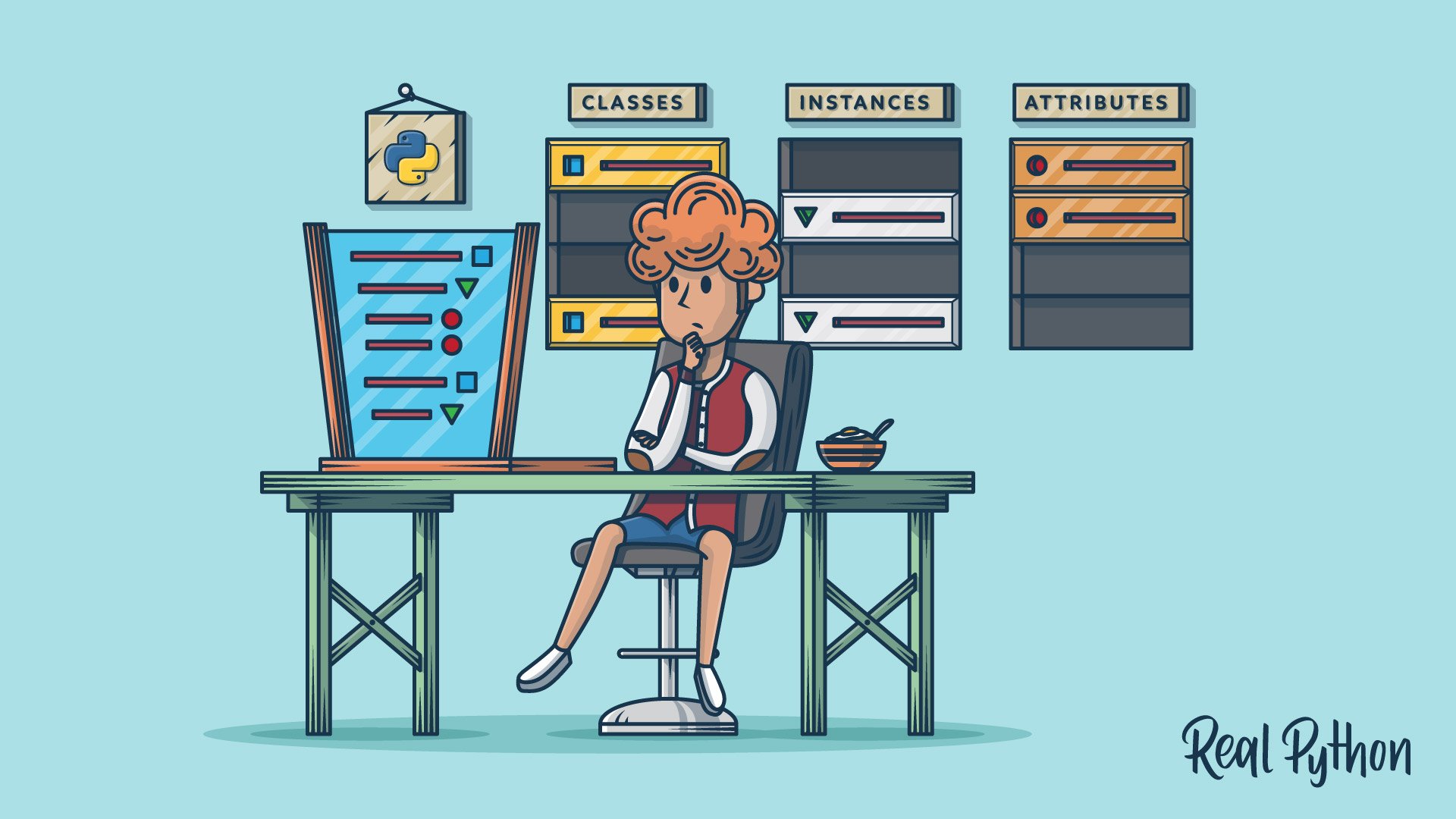
Course
Intro to Object-Oriented Programming (OOP) in Python
Learn the fundamentals of object-oriented programming (OOP) in Python and how to work with classes, objects, and constructors.
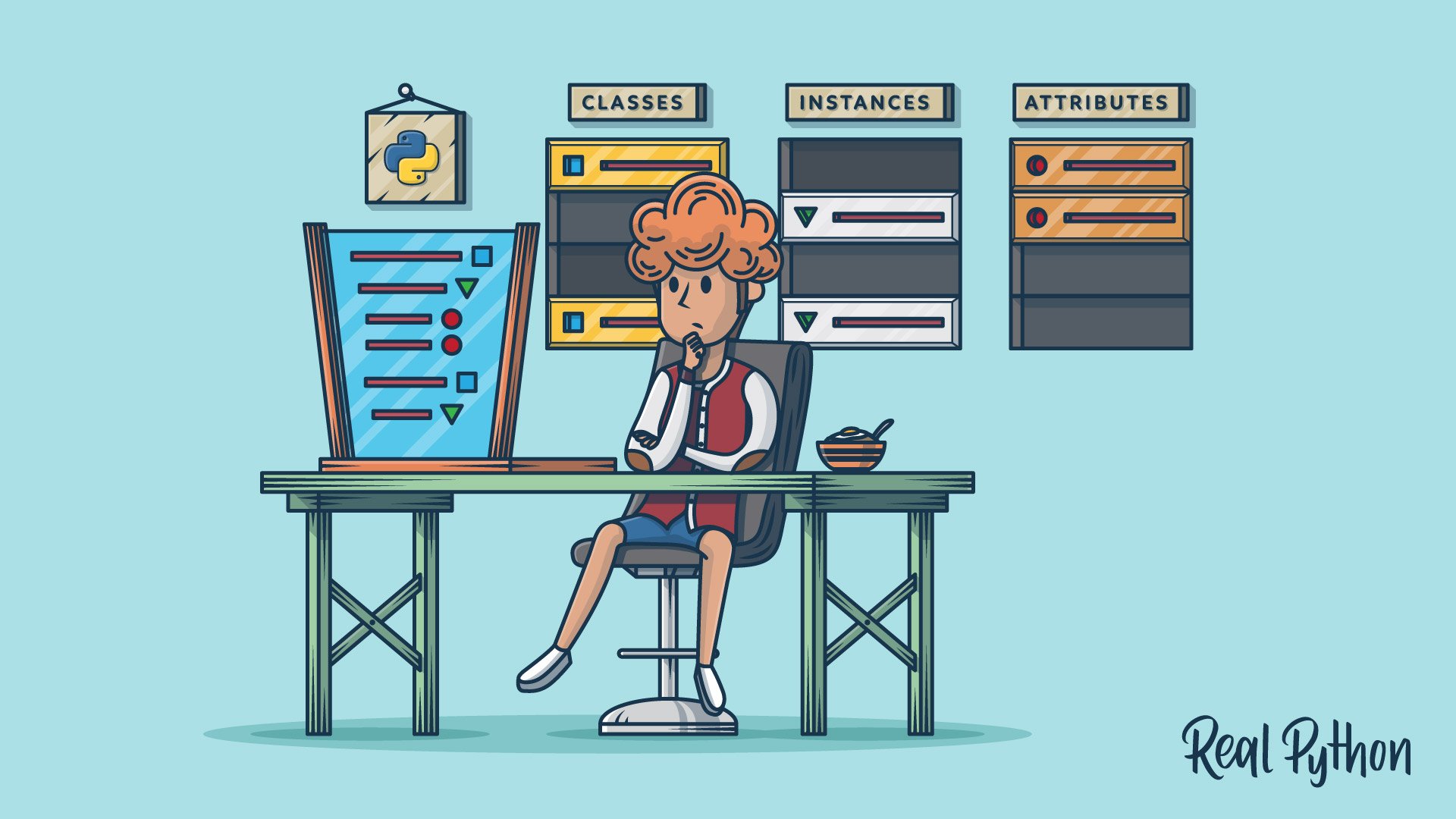
Interactive Quiz
Object-Oriented Programming (OOP) in Python
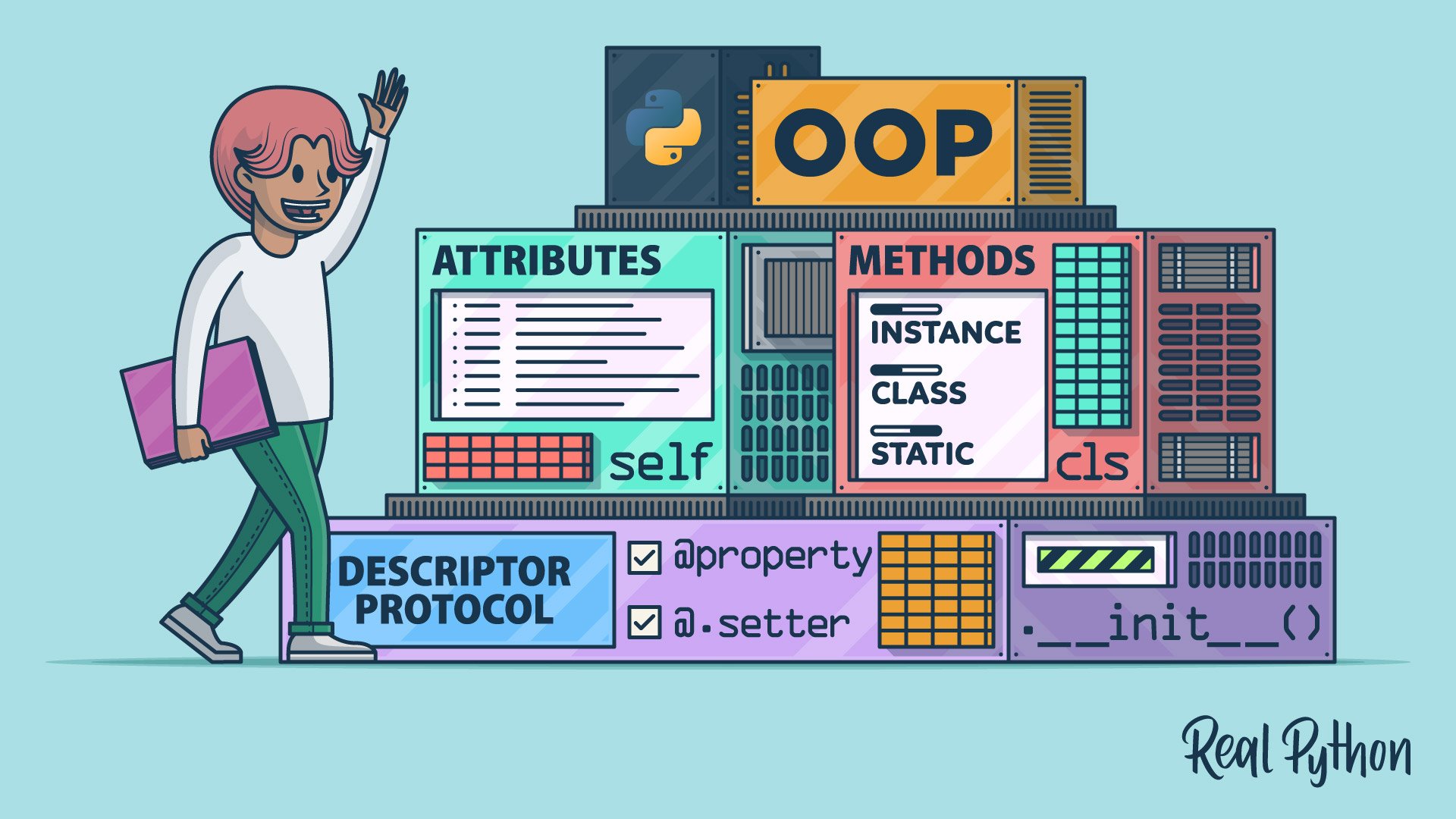
Course
Class Concepts: Object-Oriented Programming in Python
Python uses object-oriented programming to group data and associated operations together into classes. In this video course, you'll learn how to write object-oriented code with classes, attributes, and methods.
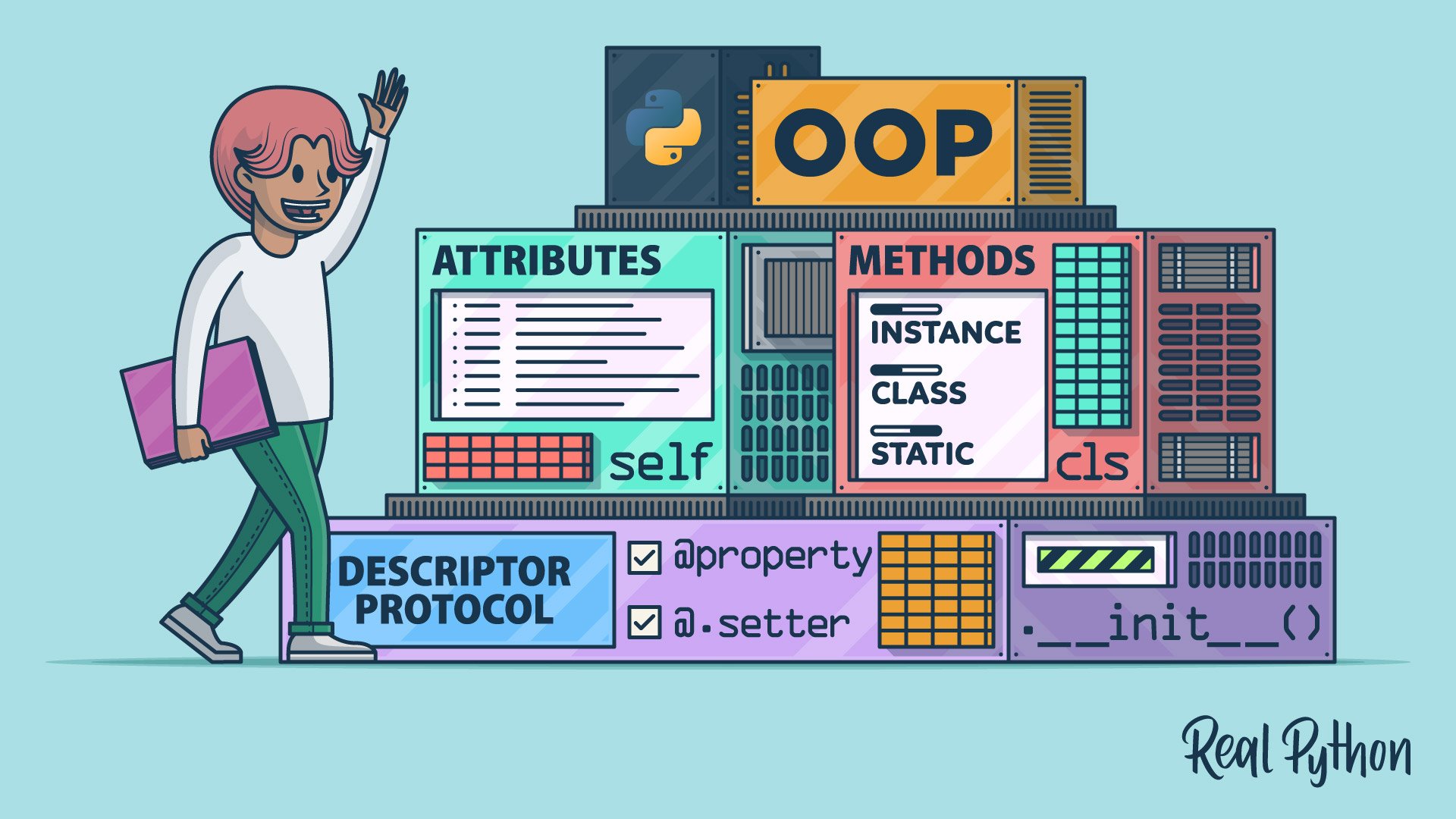
Interactive Quiz
Python Classes - The Power of Object-Oriented Programming
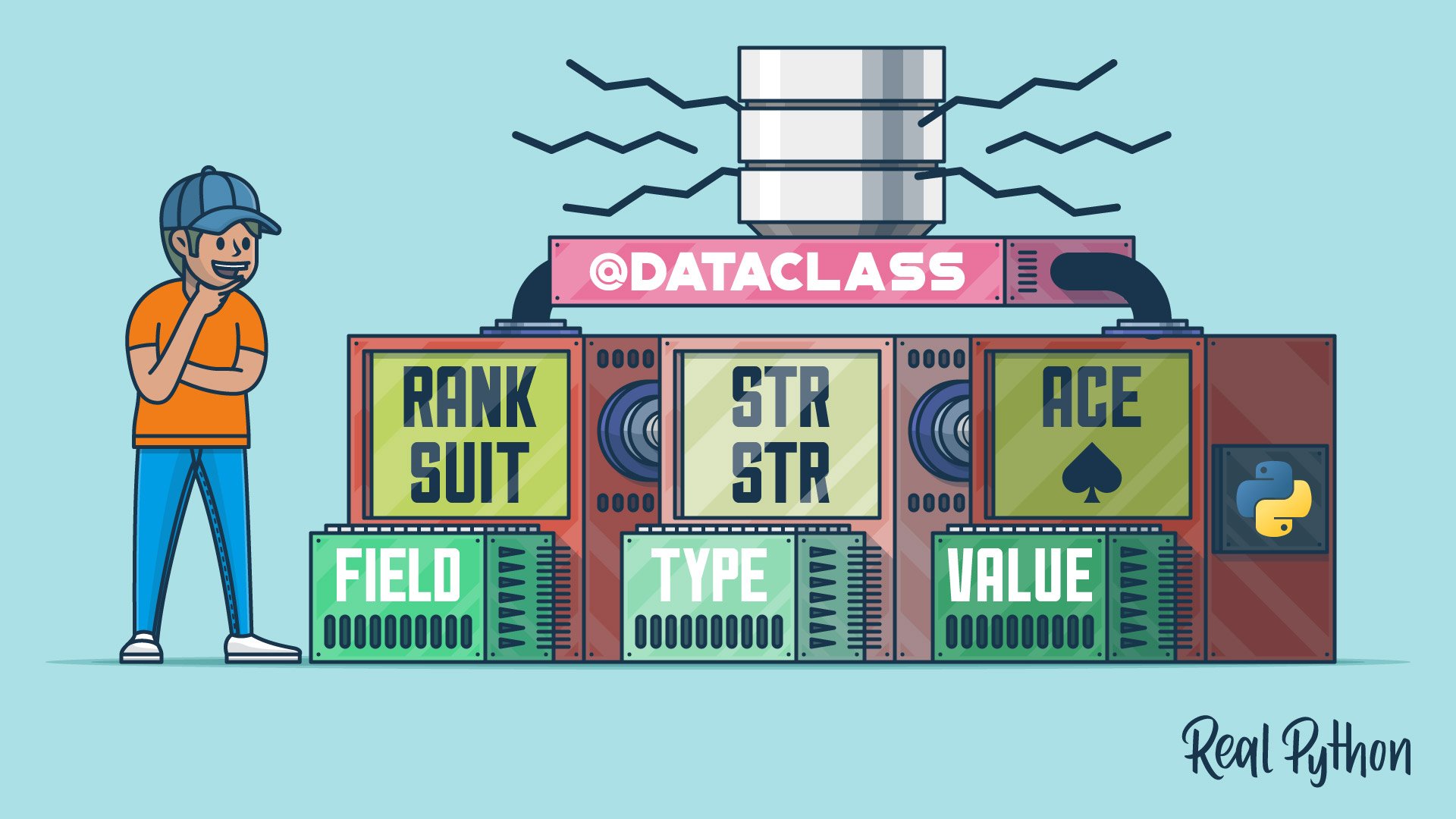
Course
Using Data Classes in Python
Data classes are one of the new features introduced in Python 3.7. When using data classes, you don't have to write boilerplate code to get proper initialization, representation, and comparisons for your objects.
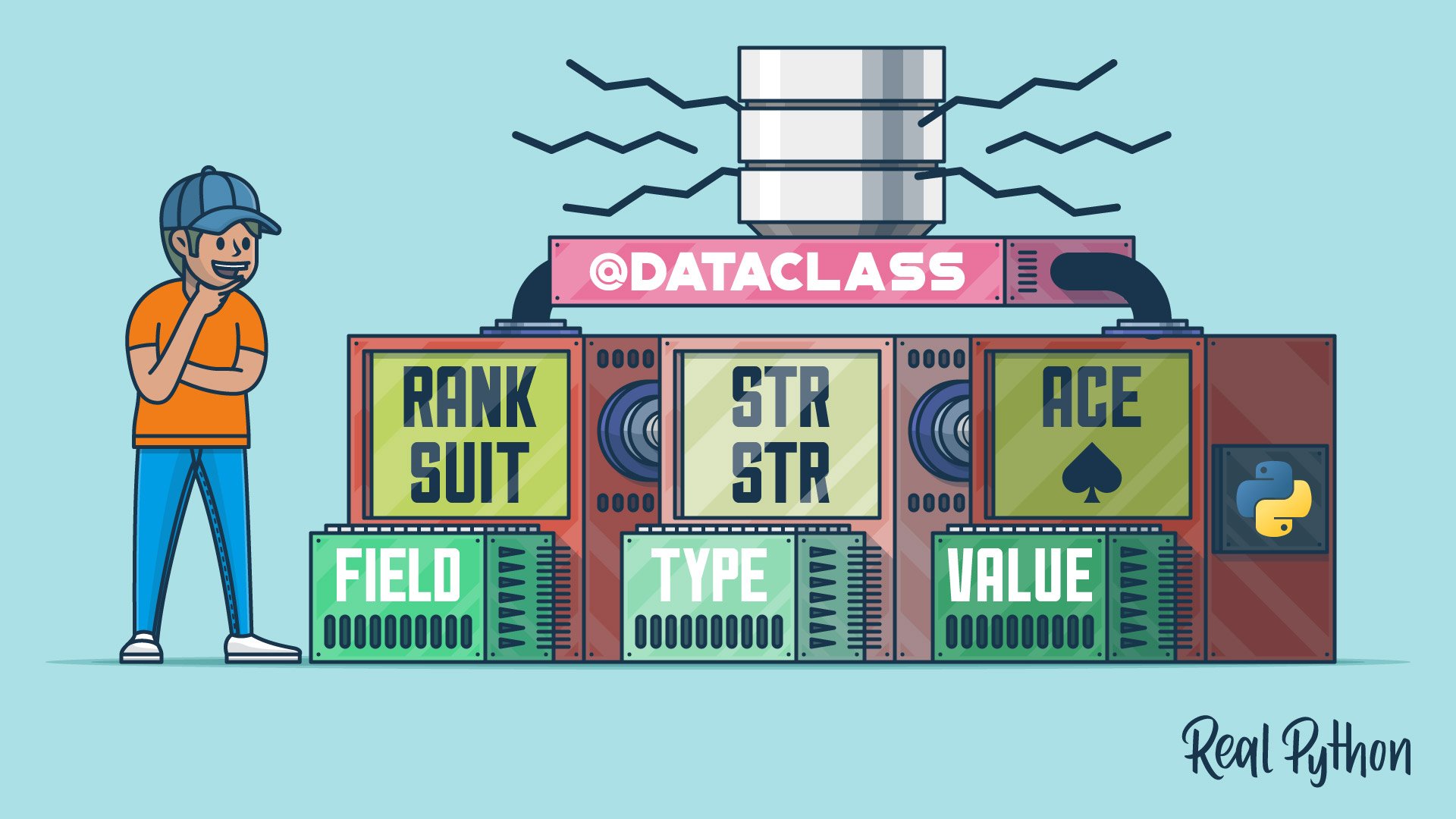
Interactive Quiz
Data Classes in Python
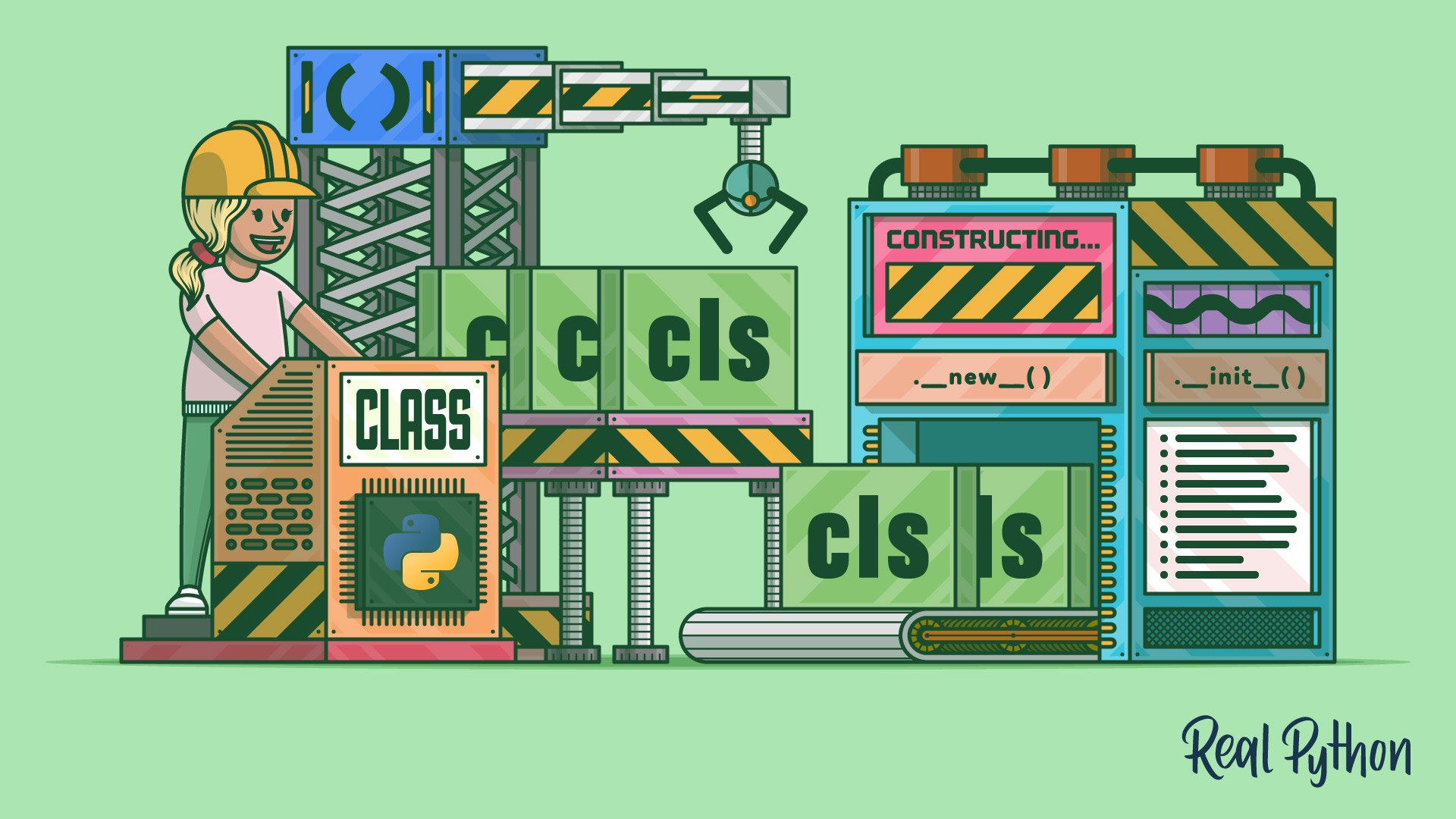
Course
Using Python Class Constructors
Learn how class constructors work in Python. You'll also explore Python's instantiation process, which has two main steps: instance creation and instance initialization.
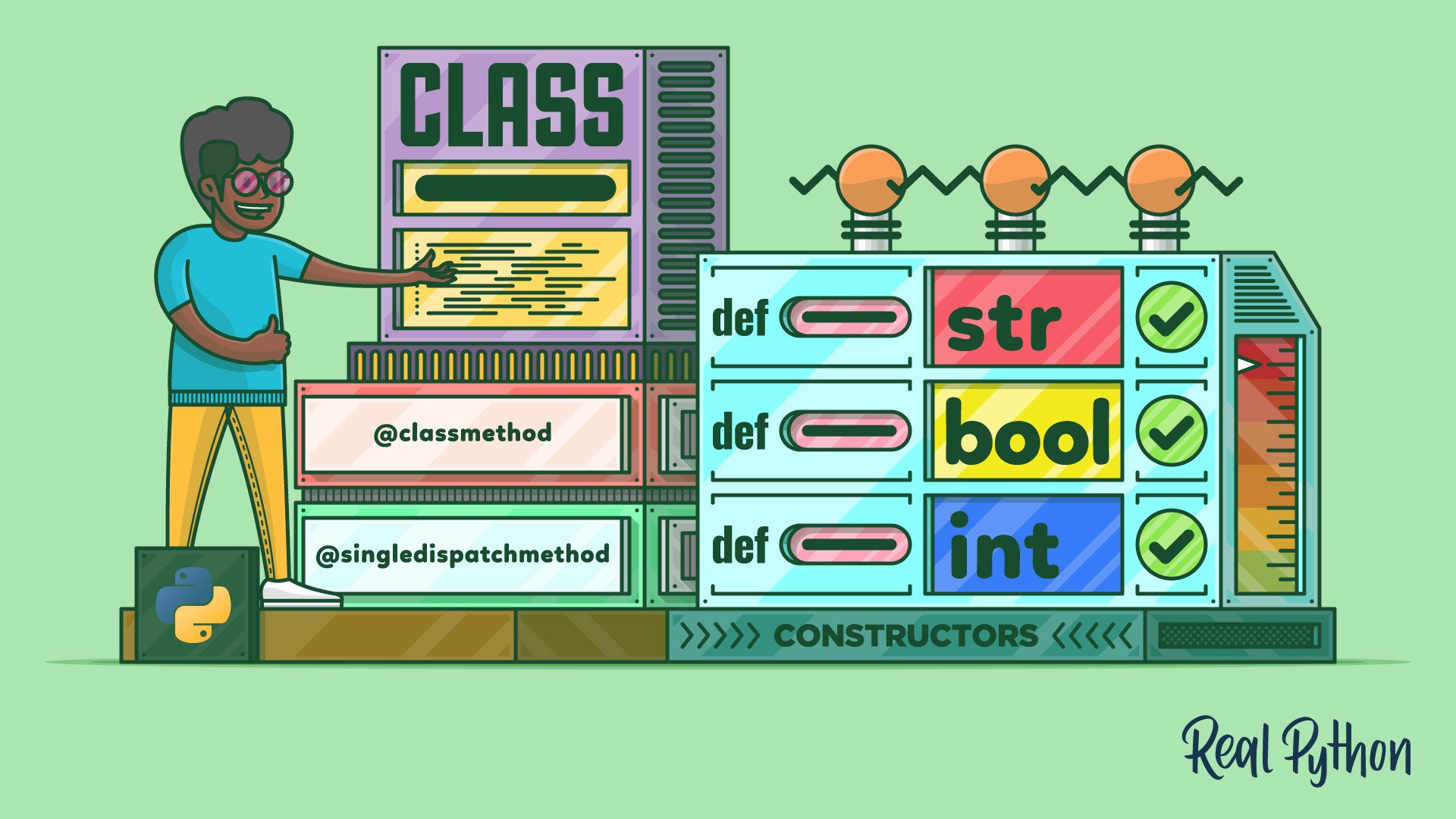
Course
Using Multiple Constructors in Your Python Classes
Learn how to provide multiple constructors in your Python classes. To this end, you'll learn different techniques, such as checking argument types, using default argument values, writing class methods, and implementing single-dispatch methods.
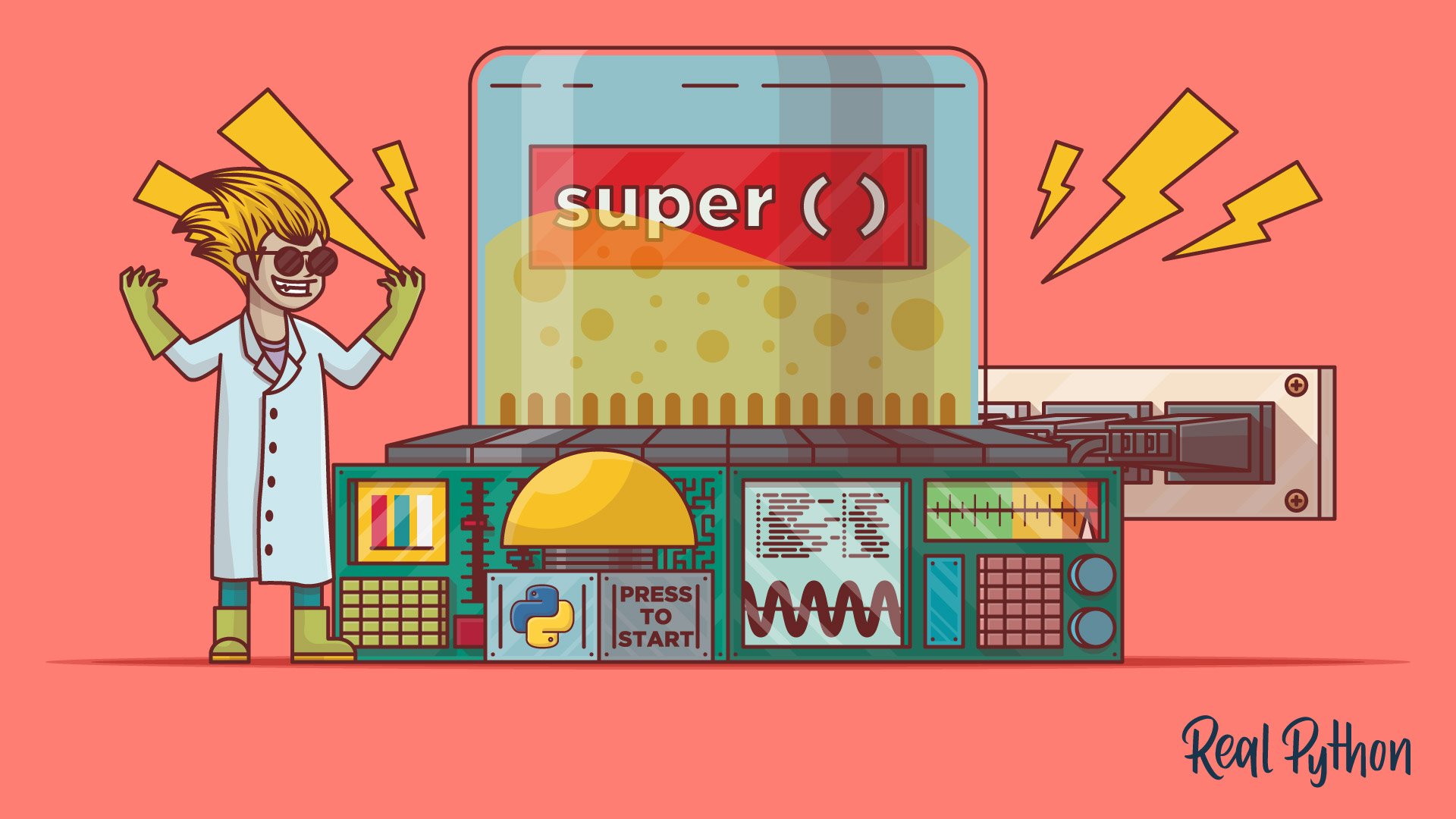
Course
Supercharge Your Classes With Python super()
Learn how to leverage single and multiple inheritance in your object-oriented application to supercharge your classes with Python super().
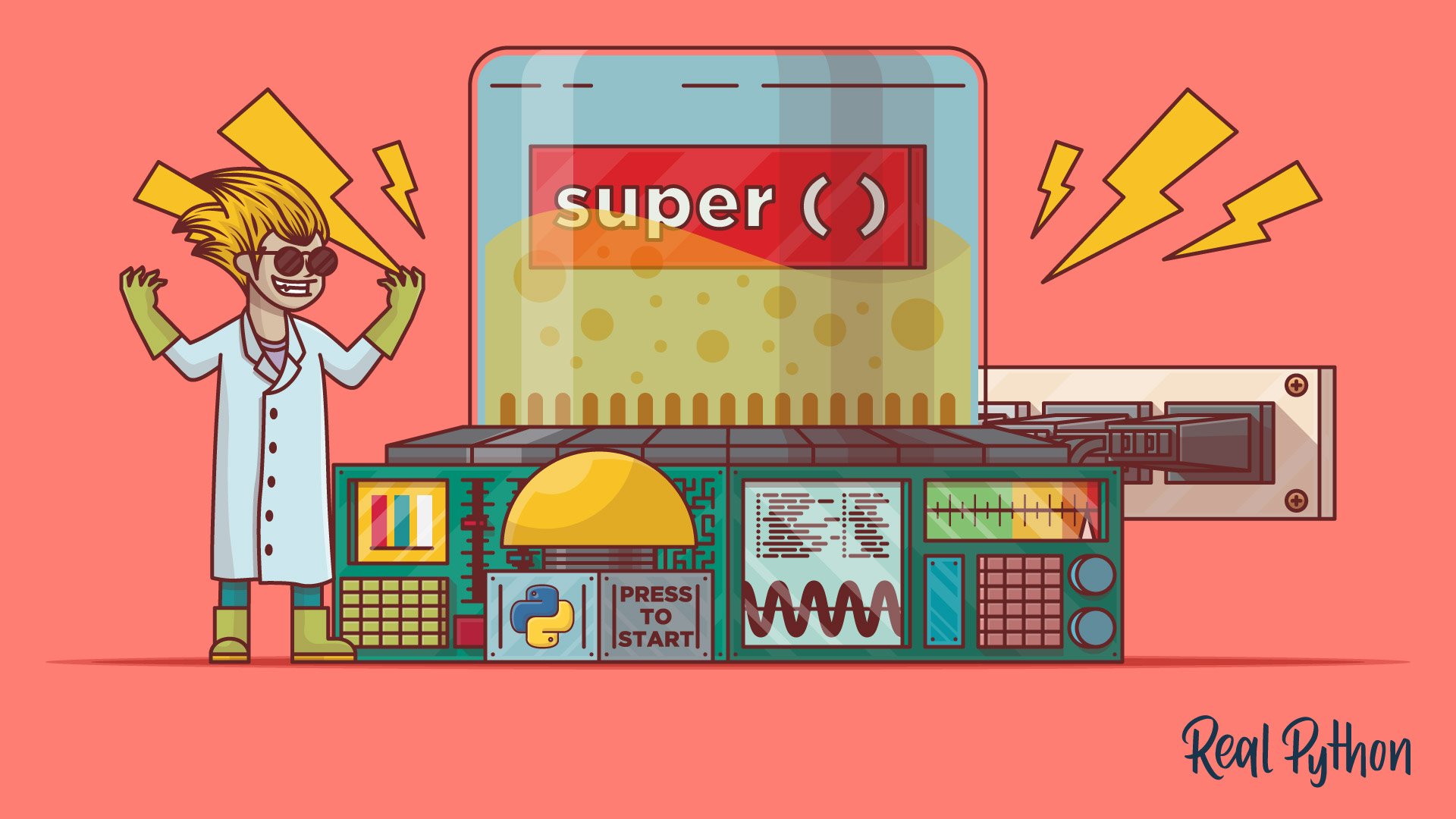
Interactive Quiz
Supercharge Your Classes With Python super()
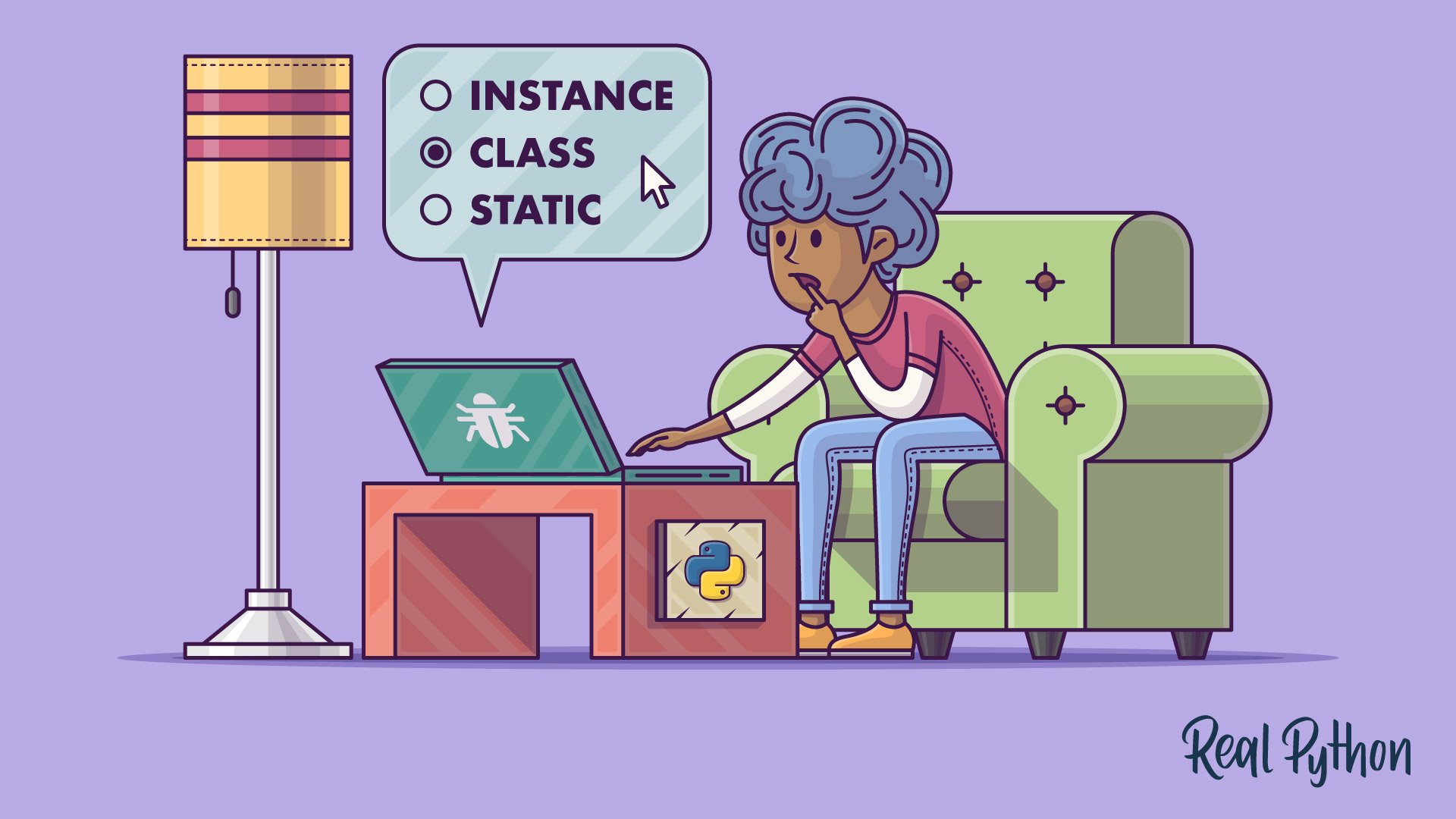
Course
OOP Method Types in Python: @classmethod vs @staticmethod vs Instance Methods
What's the difference between @classmethod, @staticmethod, and "plain/regular" instance methods in Python? You'll know the answer after watching this video course.
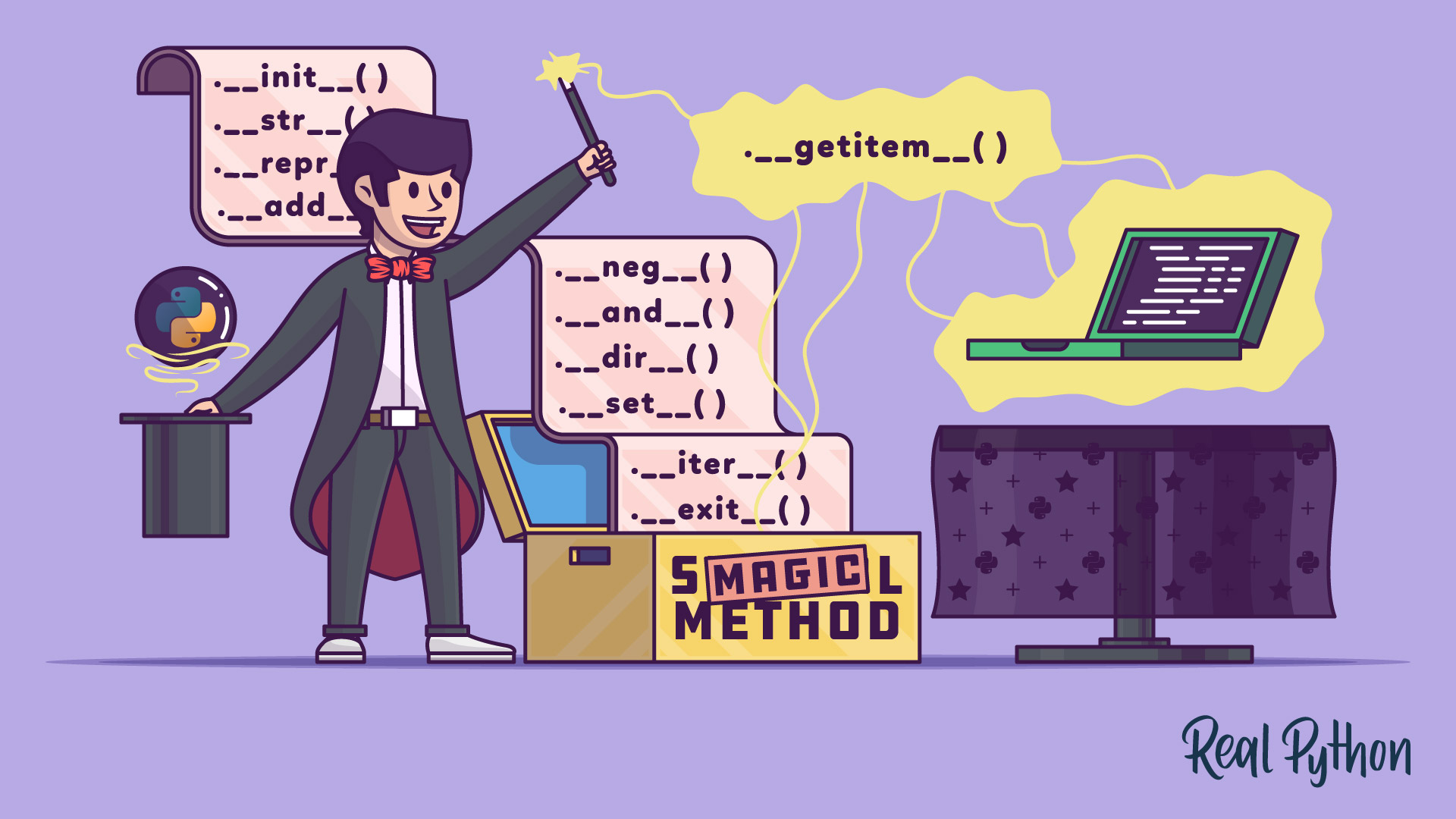
Course
Python's Magic Methods in Classes
Learn what magic methods are in Python, how they work, and how to use them in your custom classes to support powerful features in your object-oriented code.
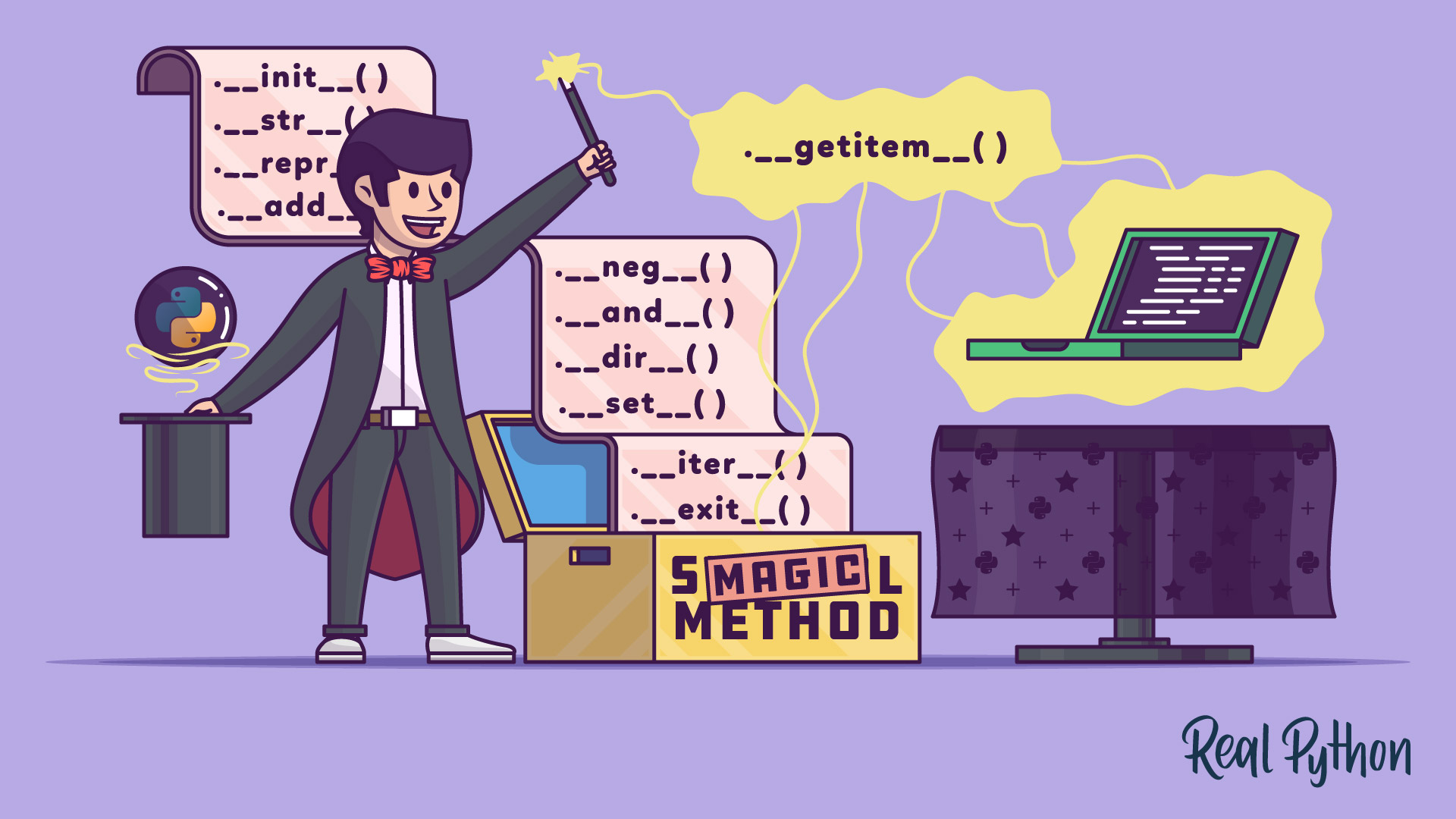
Interactive Quiz
Python's Magic Methods: Leverage Their Power in Your Classes
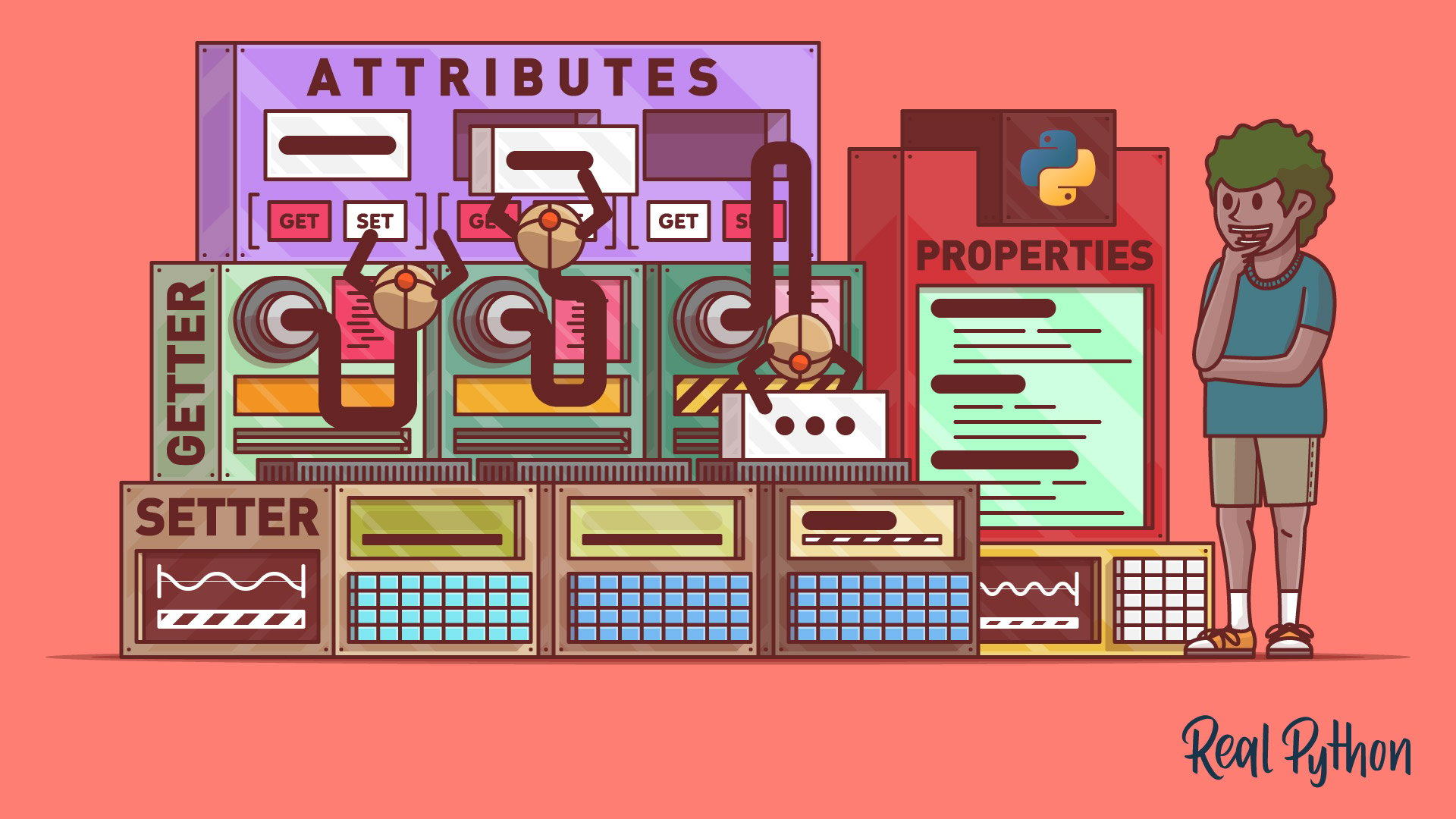
Course
Getters and Setters in Python
Learn what getter and setter methods are, how Python properties are preferred over getters and setters when dealing with attribute access and mutation, and when to use getter and setter methods instead of properties in Python.
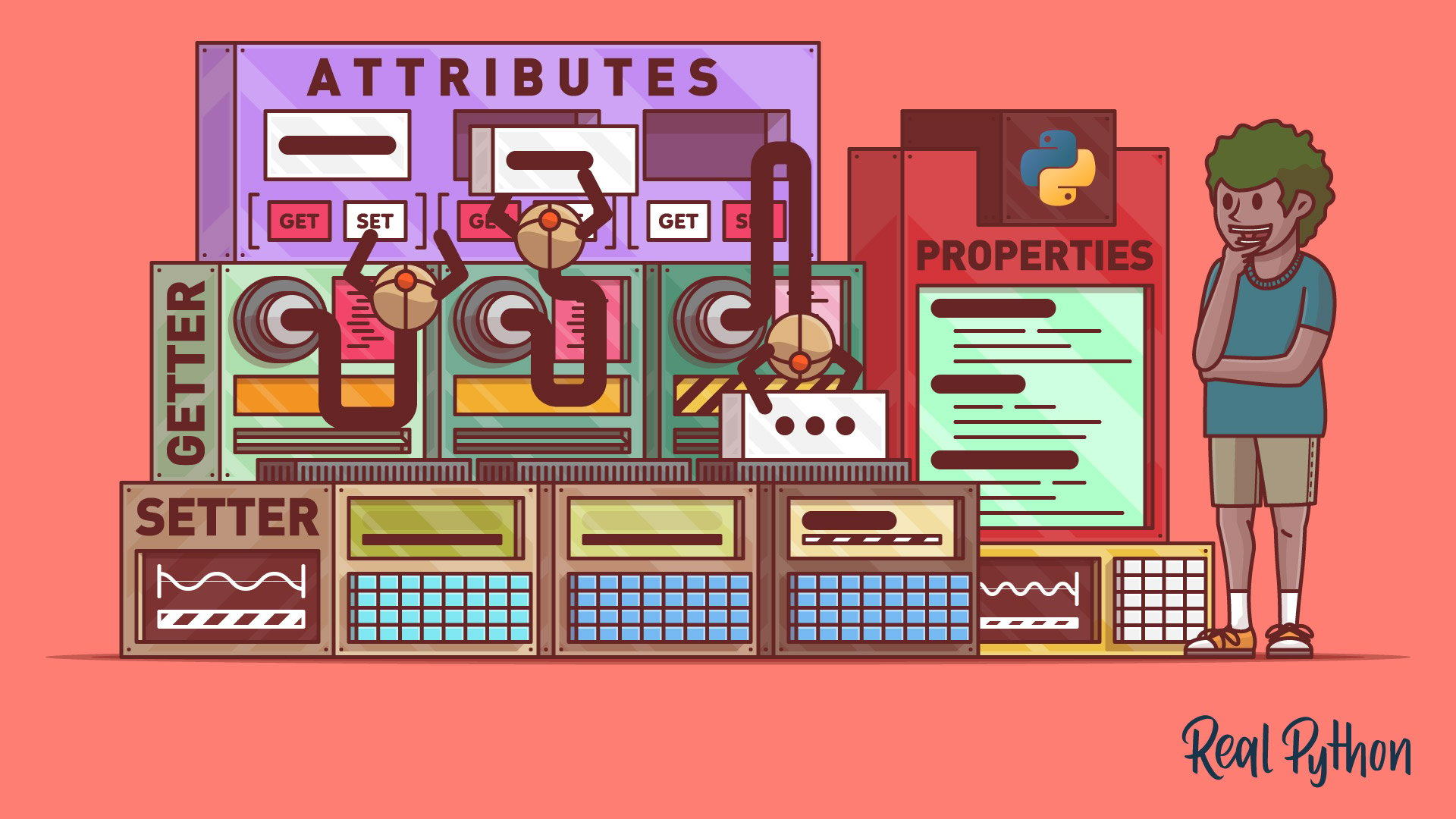
Interactive Quiz
Getters and Setters: Manage Attributes in Python
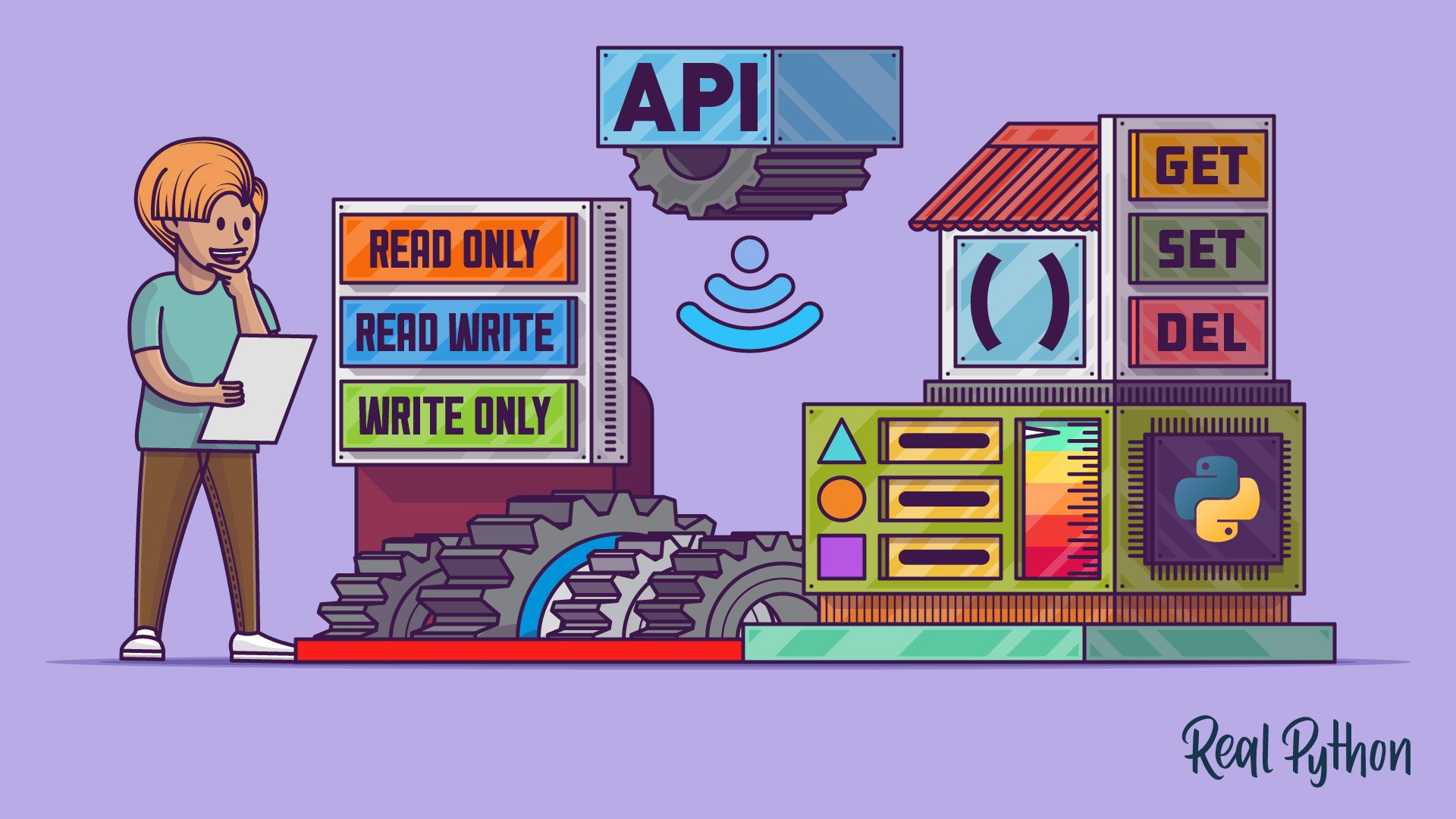
Course
Managing Attributes With Python's property()
Learn how to create managed attributes, also known as properties, using Python's property() in your custom classes.
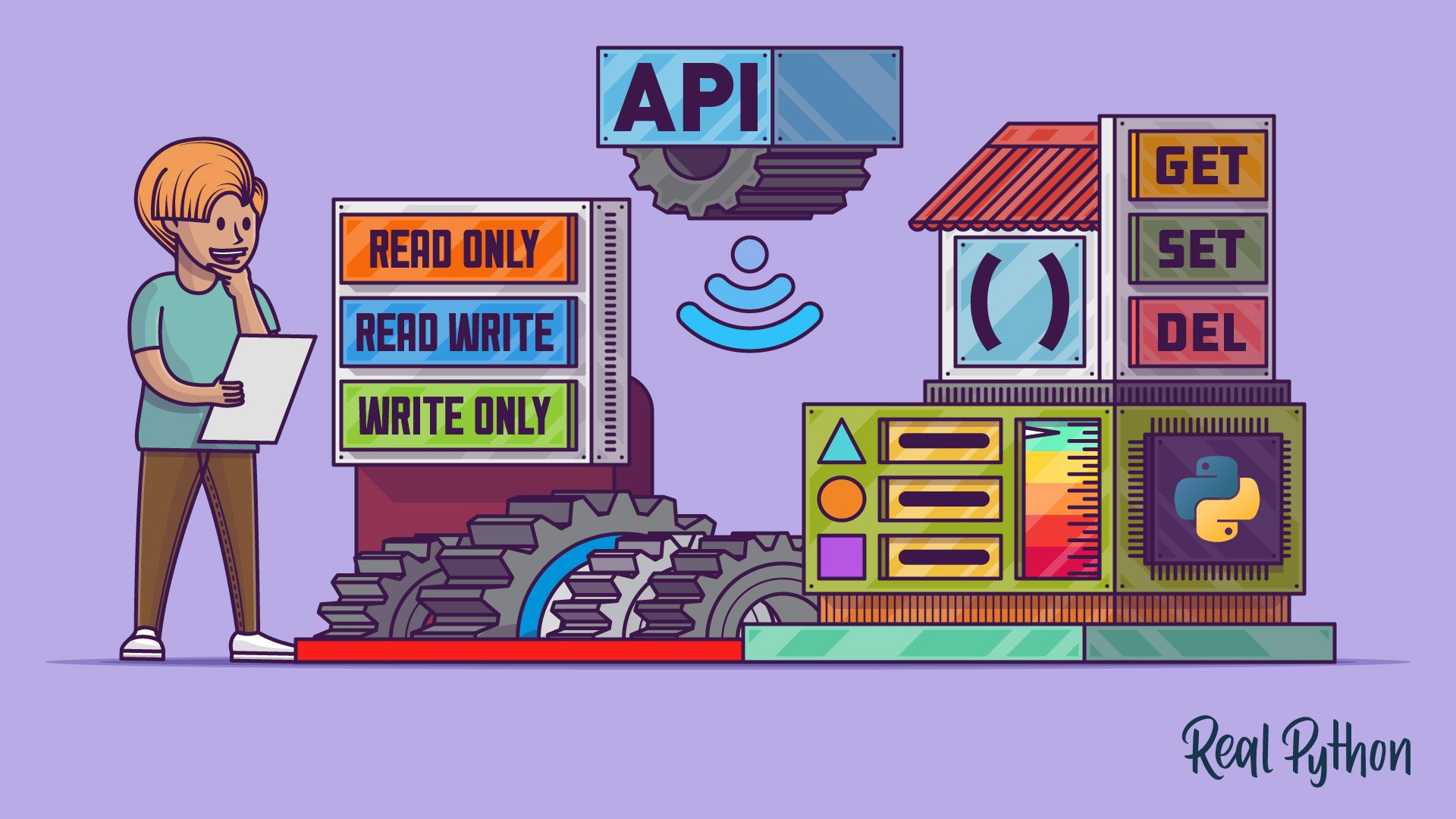
Interactive Quiz
Python's property(): Add Managed Attributes to Your Classes
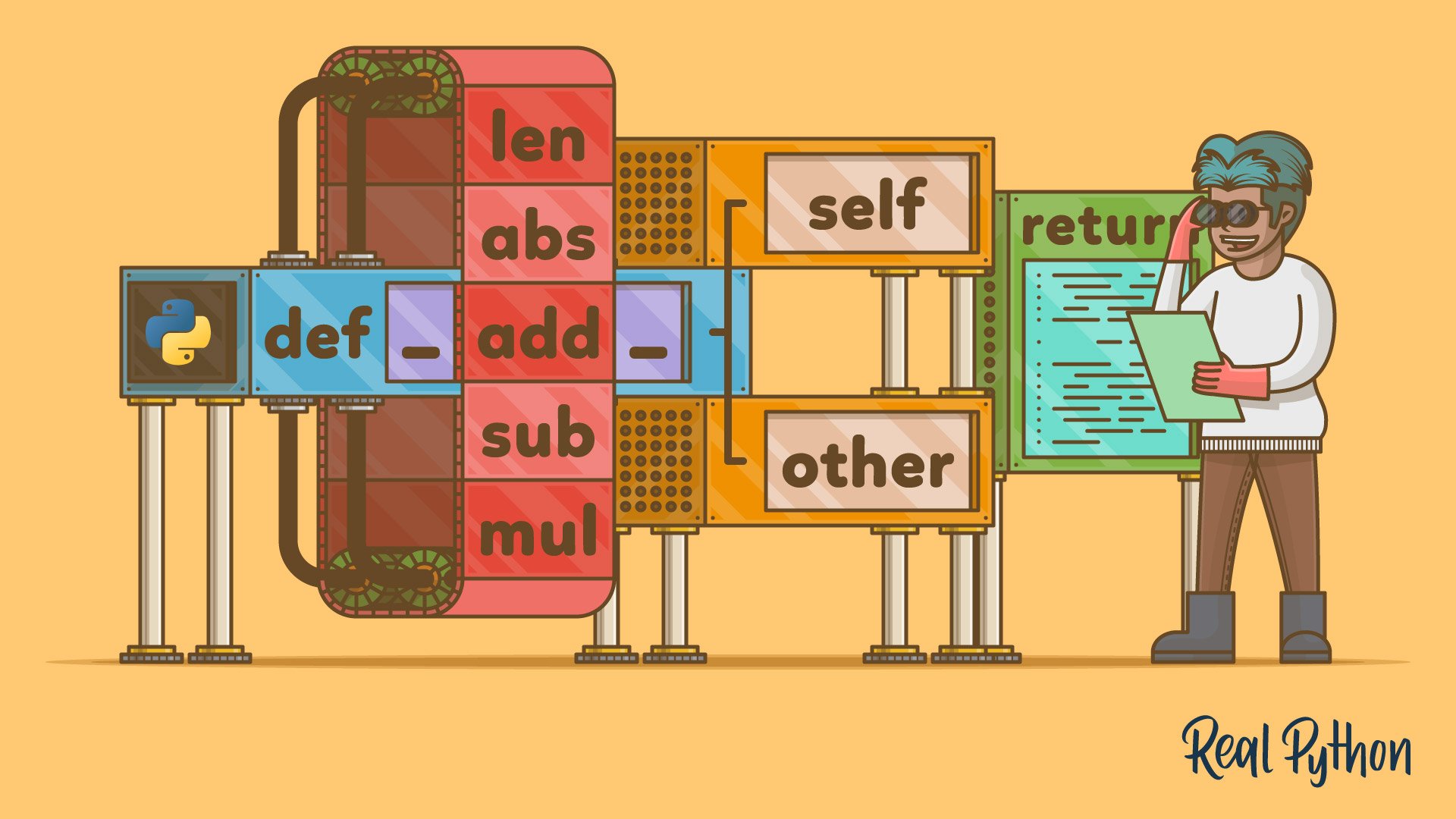
Tutorial
Operator and Function Overloading in Custom Python Classes
How to overload built-in functions and operators in your custom Python classes in order to make your code more Pythonic.
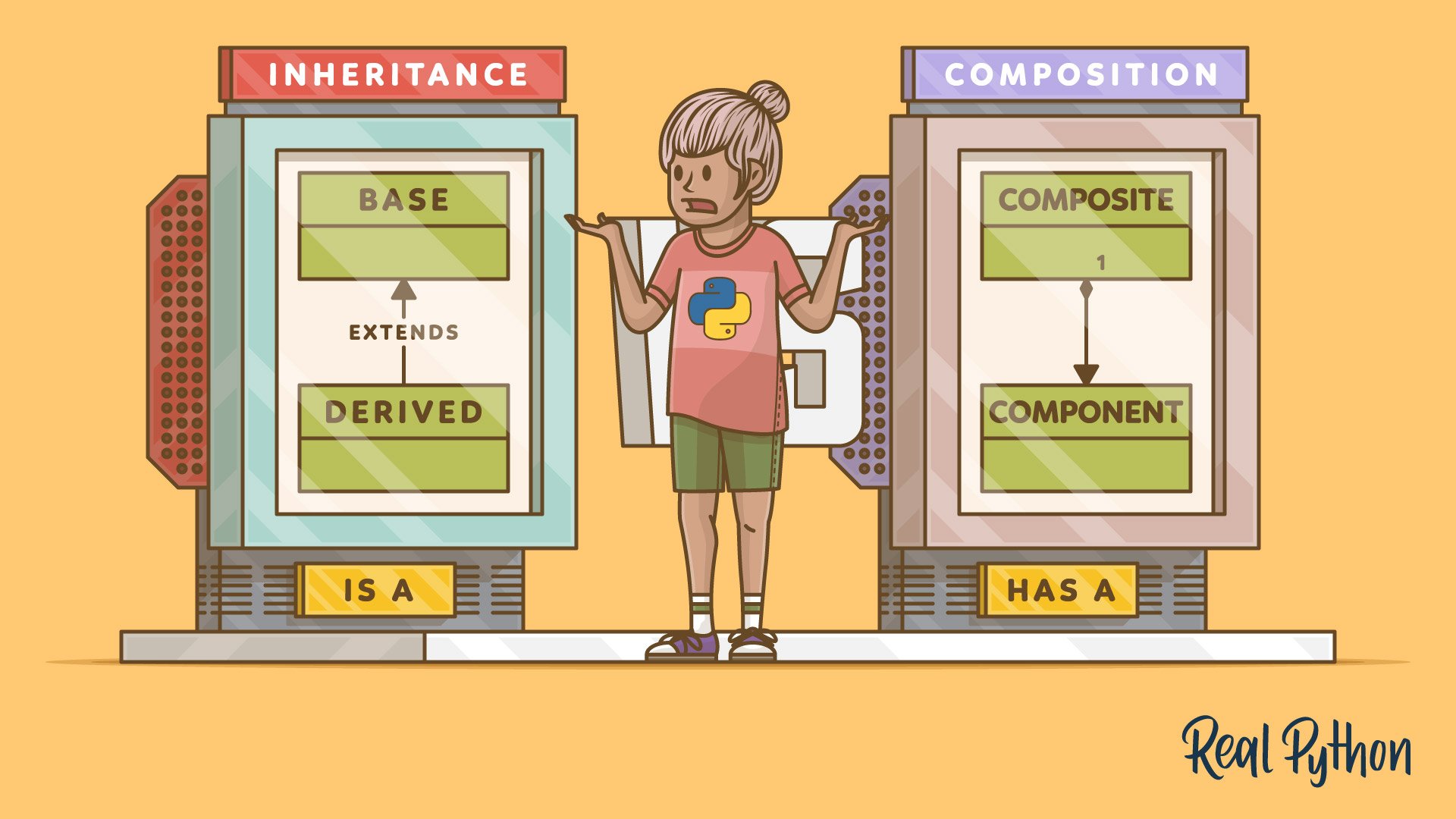
Course
Inheritance and Composition: A Python OOP Guide
Learn about inheritance and composition in Python. You'll improve your object-oriented programming (OOP) skills by understanding how to use inheritance and composition and how to leverage them in their design.
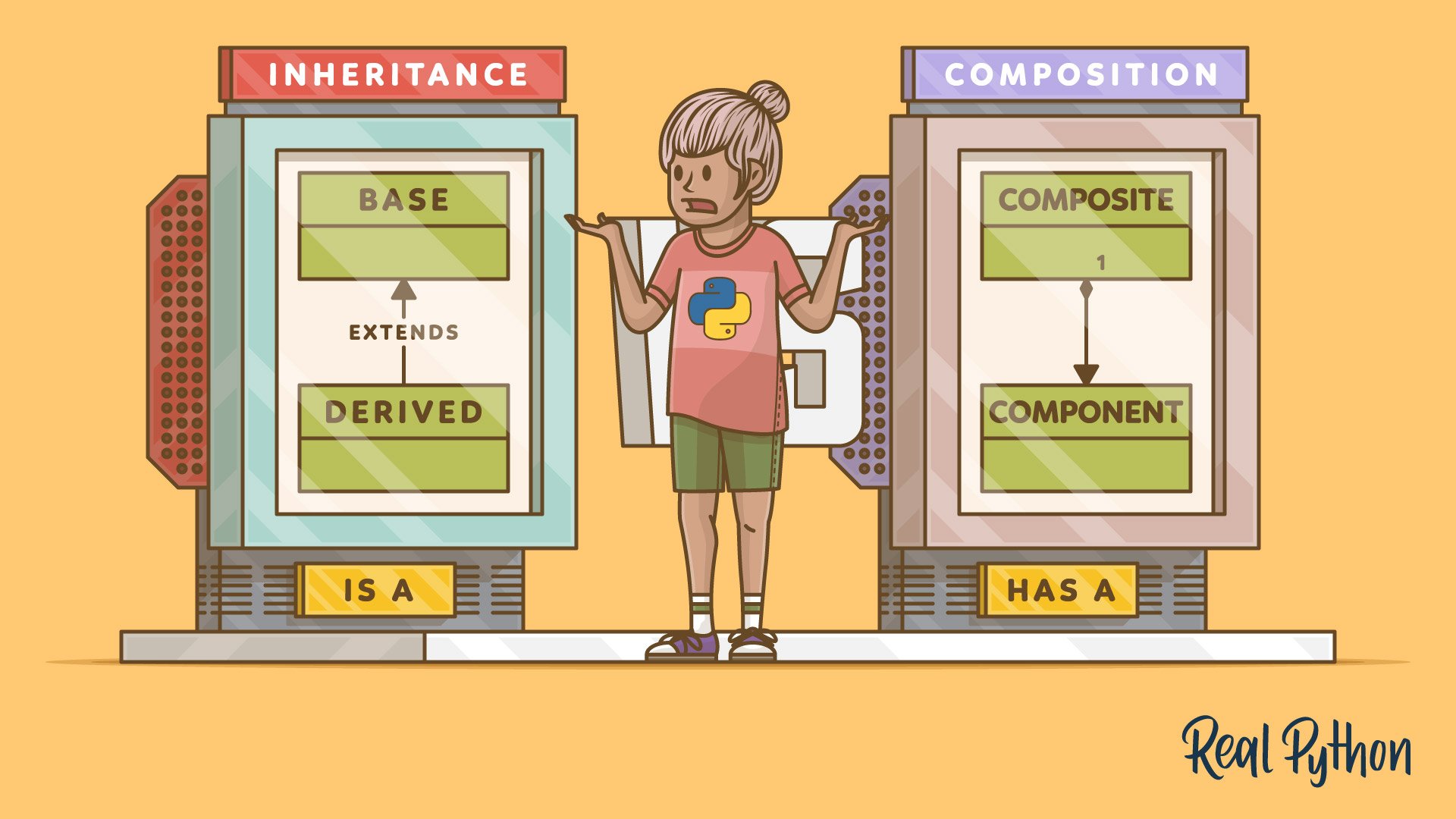
Interactive Quiz
Inheritance and Composition: A Python OOP Guide
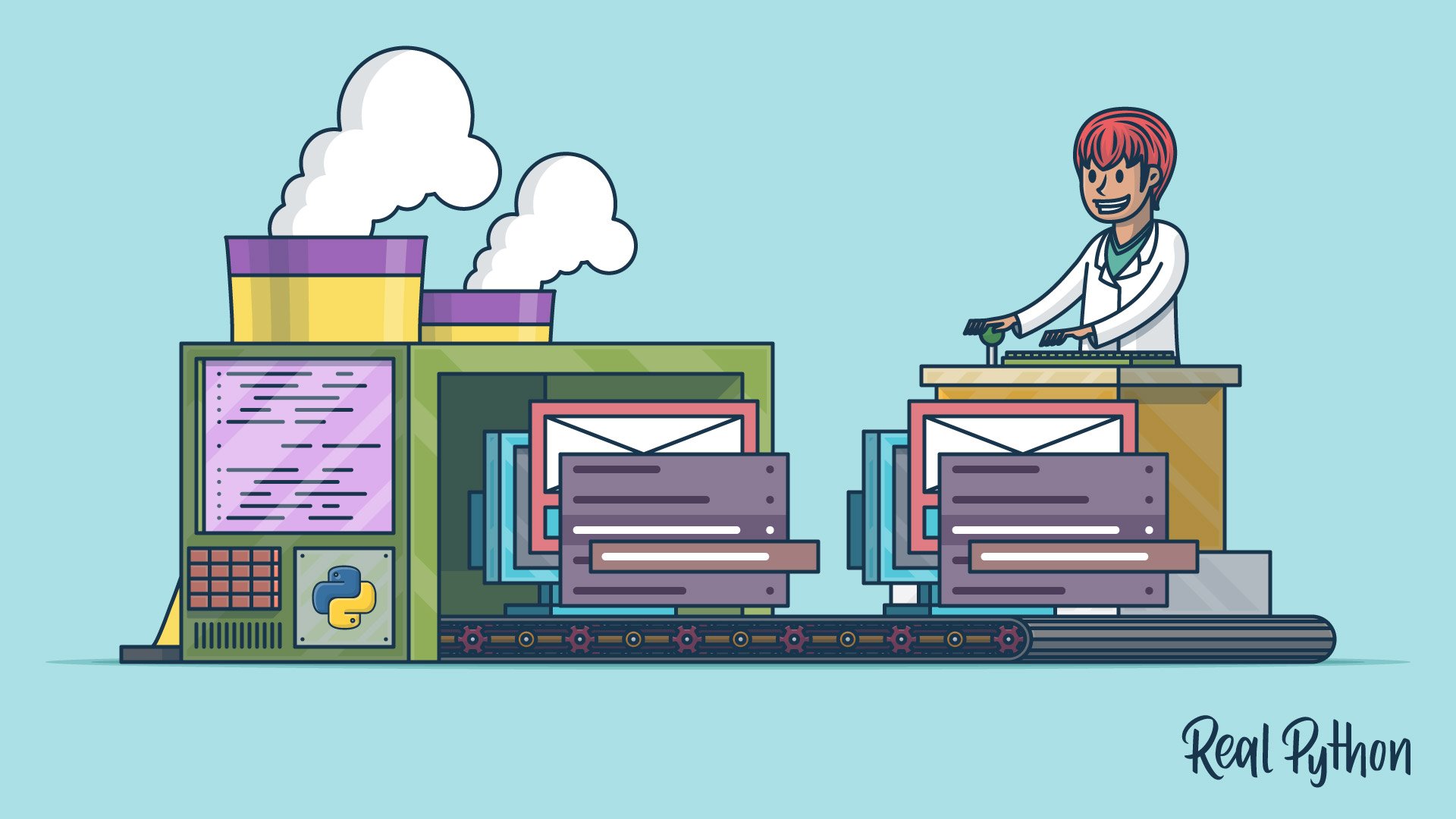
Tutorial
The Factory Method Pattern and Its Implementation in Python
Learn about the Factory Method design pattern and its implementation. You'll understand the components of Factory Method, when to use it, and how to modify existing code to leverage it. You'll also see a general purpose implementation of Factory Method in Python.
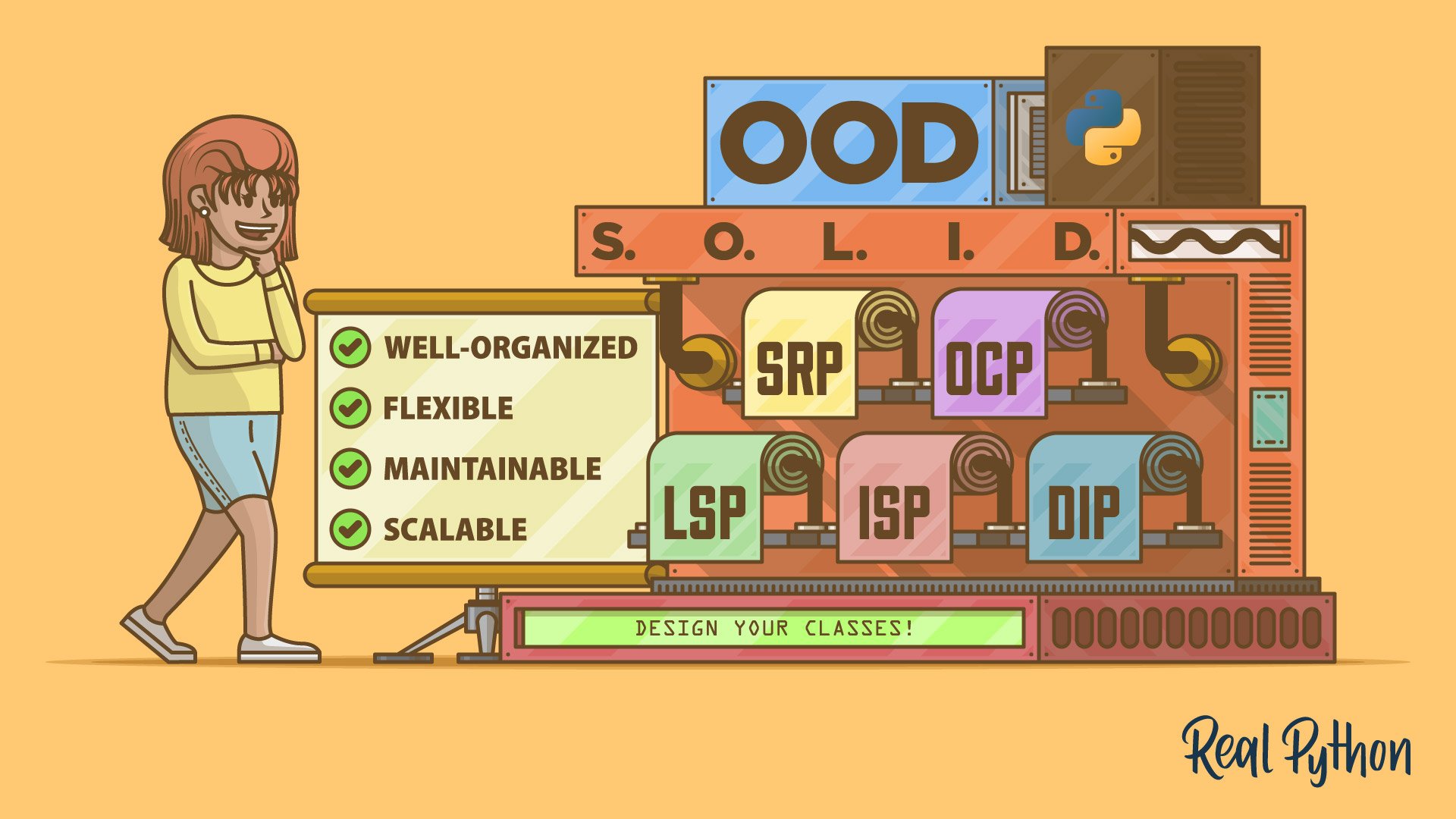
Course
Design and Guidance: Object-Oriented Programming in Python
Learn about the SOLID principles, which are five well-established standards for improving your object-oriented design in Python. By applying these principles, you can create object-oriented code that is more maintainable, extensible, scalable, and testable.
Got feedback on this learning path?
Looking for real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session. Happy Pythoning!