Object-Oriented Programming (OOP) With Python
Learning Path ⋅ Skills: OOP Fundamentals, Classes & Objects, Methods
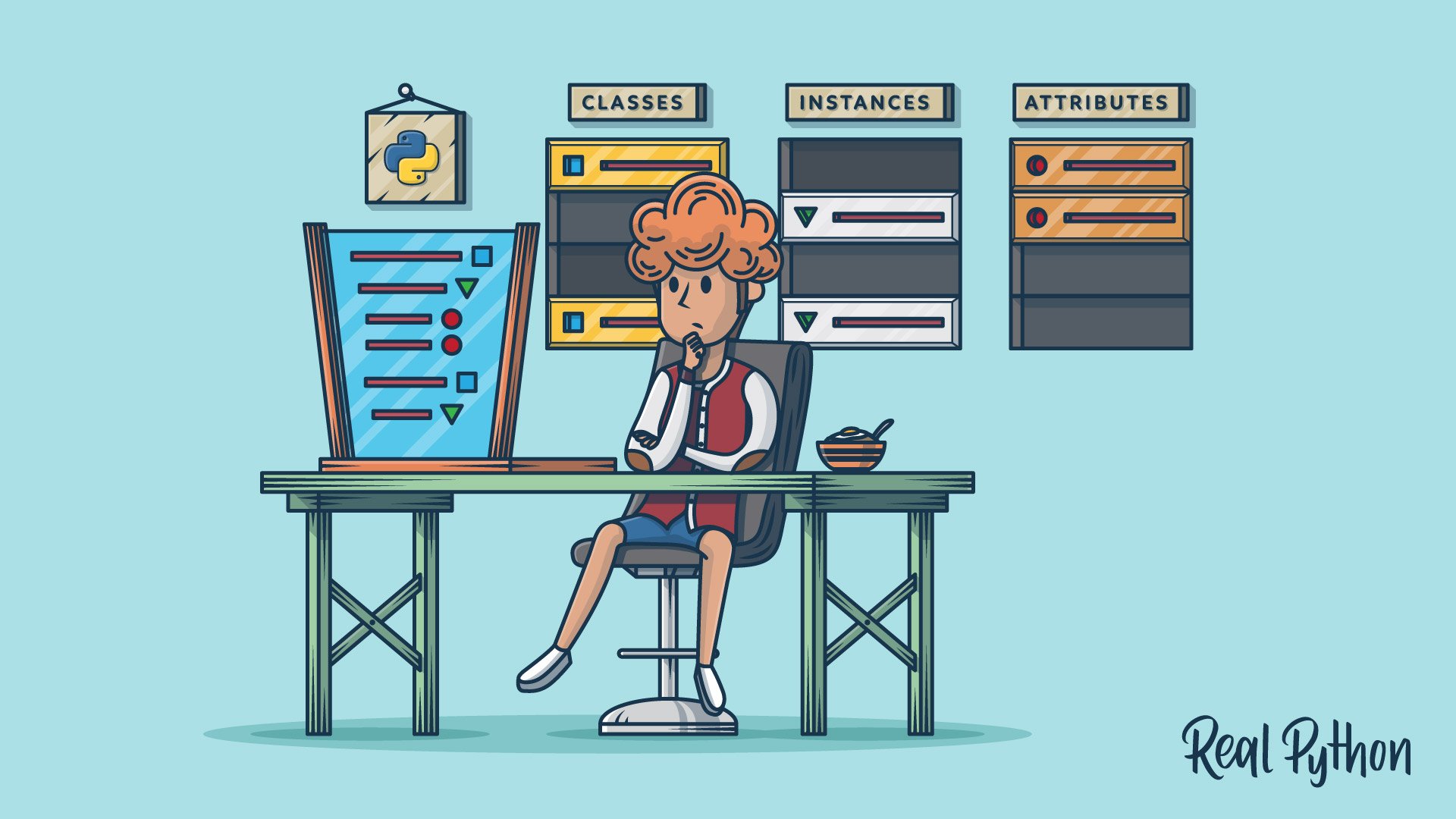
Object-oriented Programming, or OOP for short, is a programming paradigm which provides a means of structuring programs so that attributes (data) and behaviors (methods) are bundled into individual objects.
With this learning path, you’ll get a solid grasp of the fundamentals of OOP in Python. You’ll learn how to use this programming paradigm to make your programs easier to write and maintain.
Object-Oriented Programming (OOP) With Python
Learning Path ⋅ 12 Resources
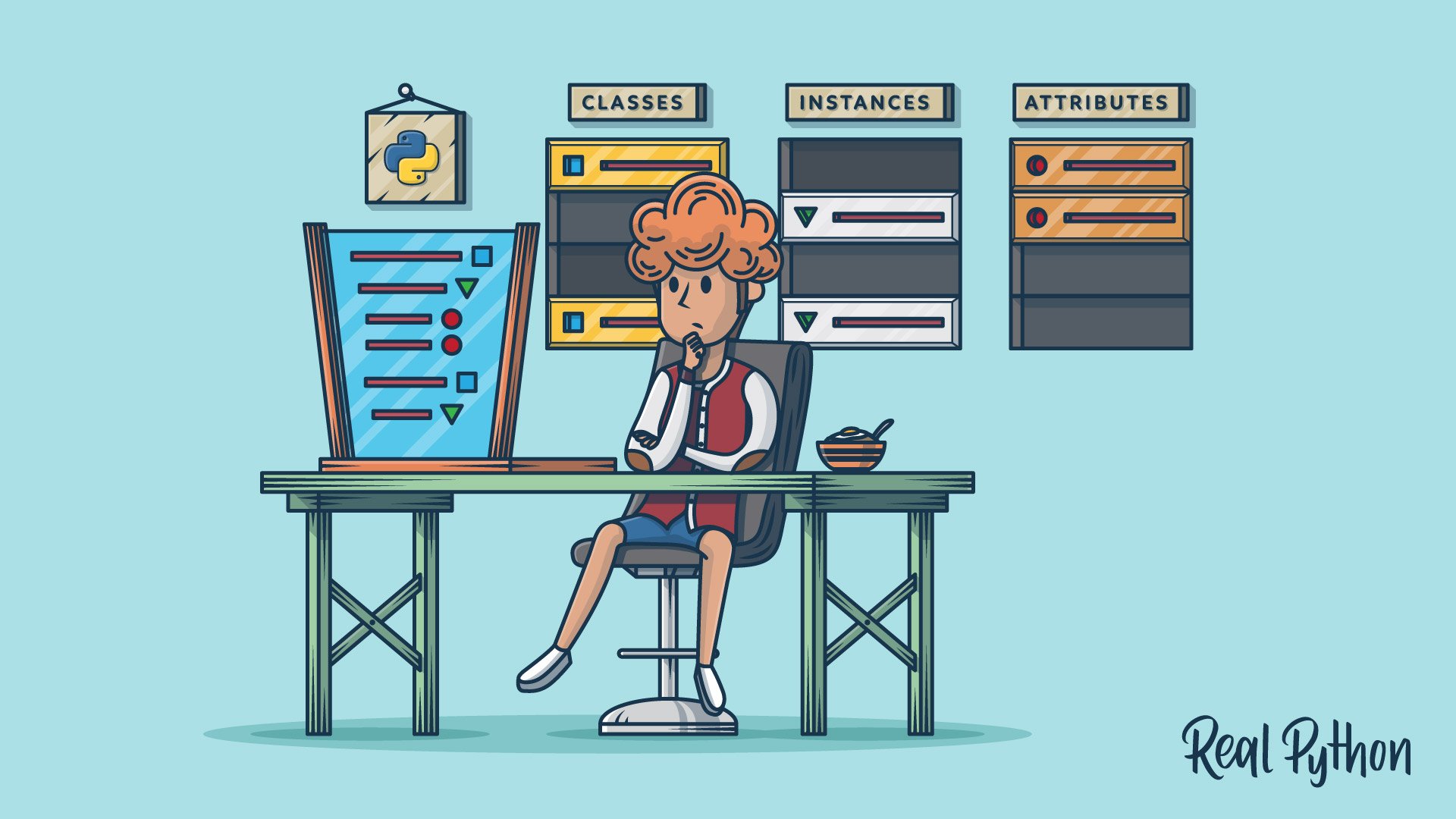
Course
Intro to Object-Oriented Programming (OOP) in Python
Learn the fundamentals of object-oriented programming (OOP) in Python and how to work with classes, objects, and constructors.
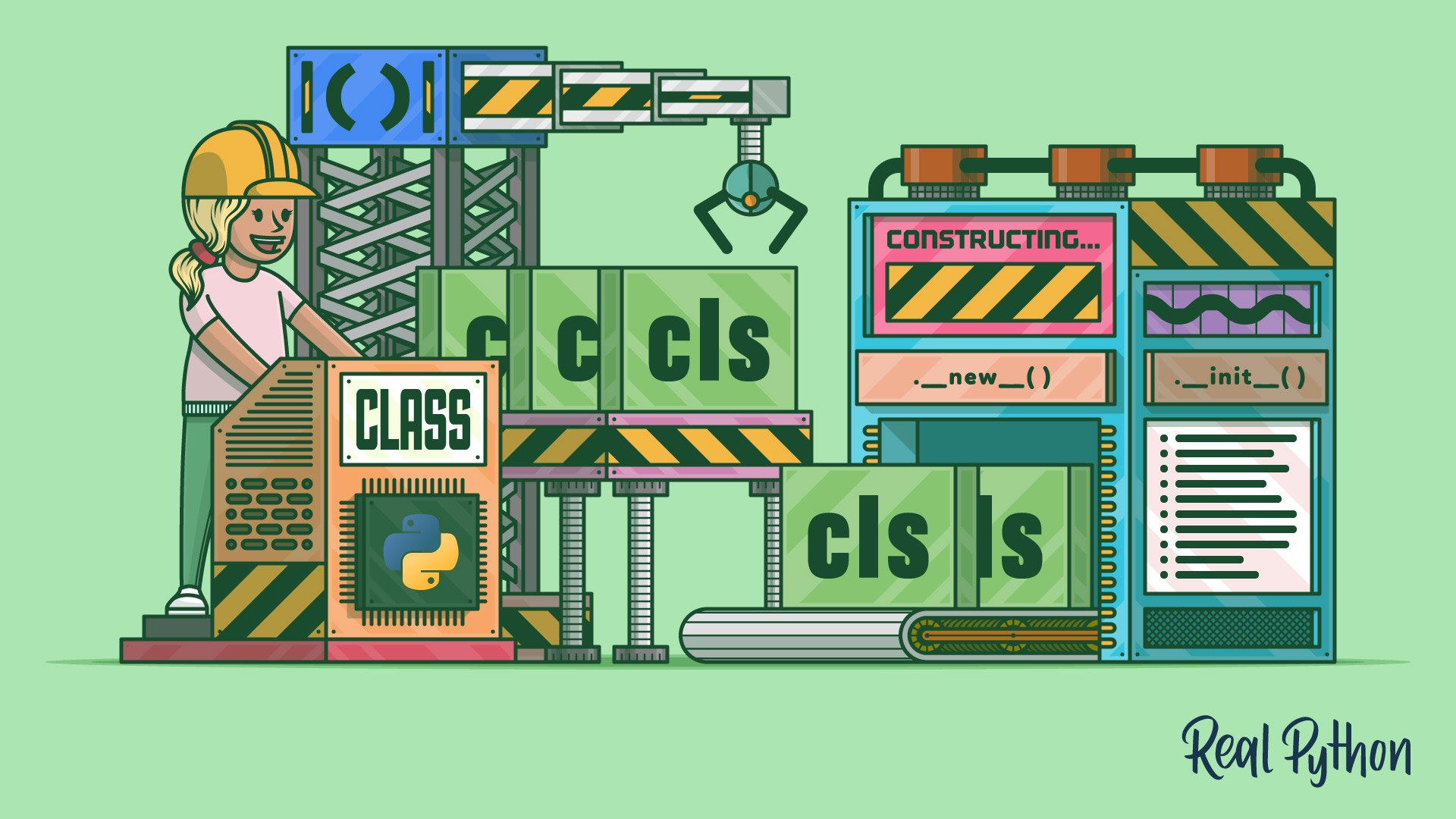
Course
Using Python Class Constructors
Learn how class constructors work in Python. You'll also explore Python's instantiation process, which has two main steps: instance creation and instance initialization.
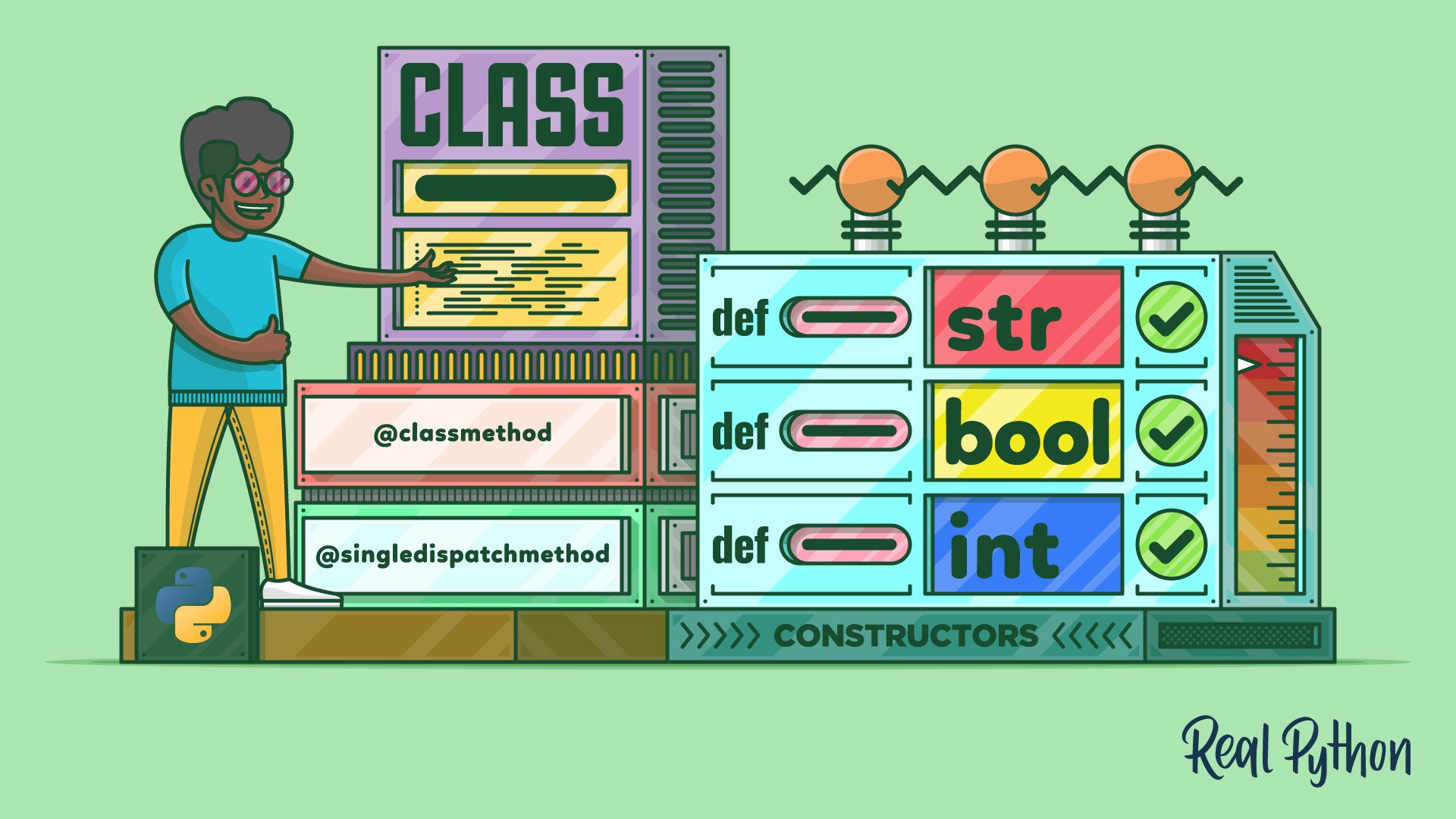
Course
Providing Multiple Constructors in Your Python Classes
Learn how to provide multiple constructors in your Python classes. To this end, you'll learn different techniques, such as checking argument types, using default argument values, writing class methods, and implementing single-dispatch methods.
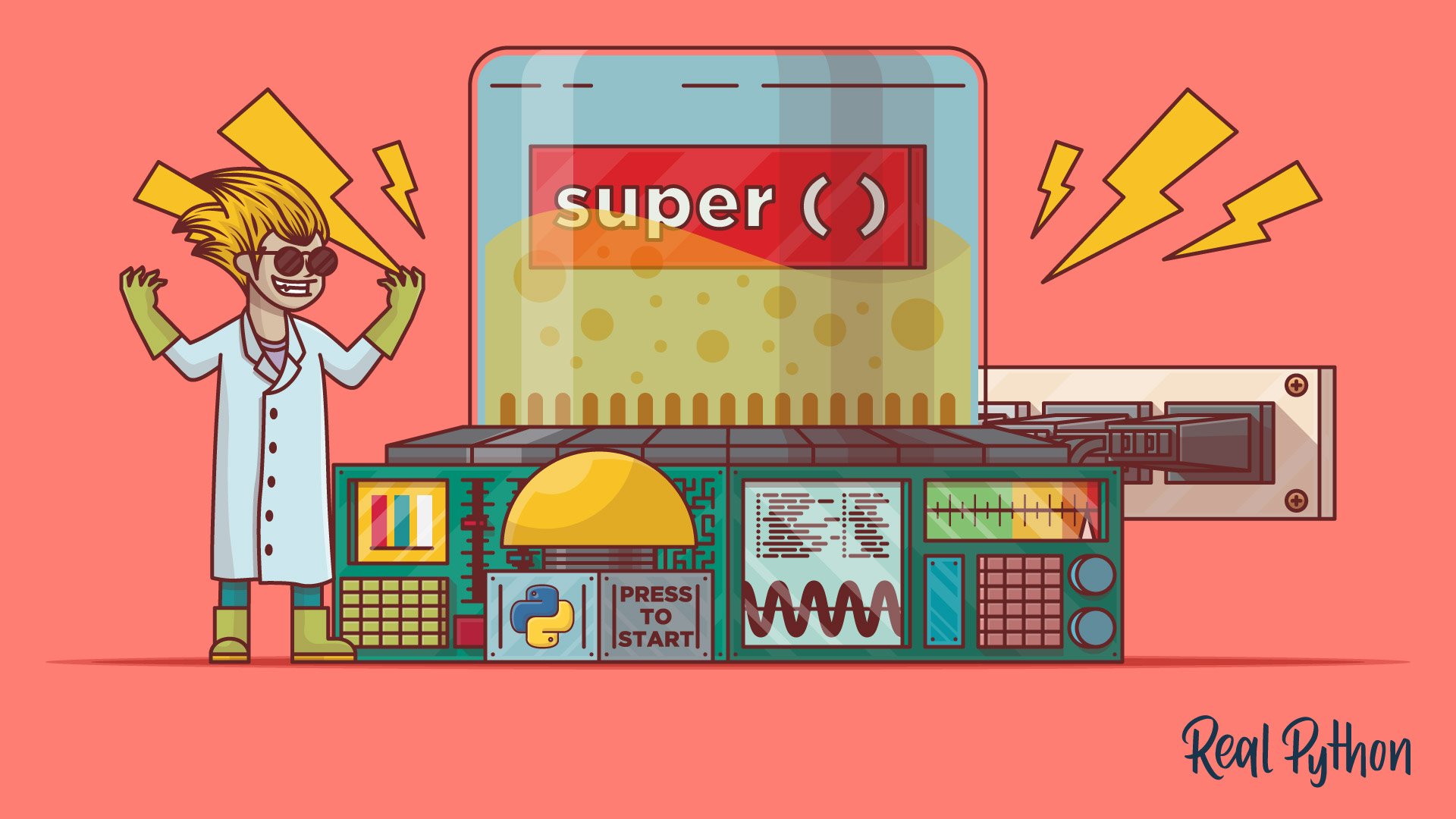
Course
Supercharge Your Classes With Python super()
Learn how to leverage single and multiple inheritance in your object-oriented application to supercharge your classes with Python super().
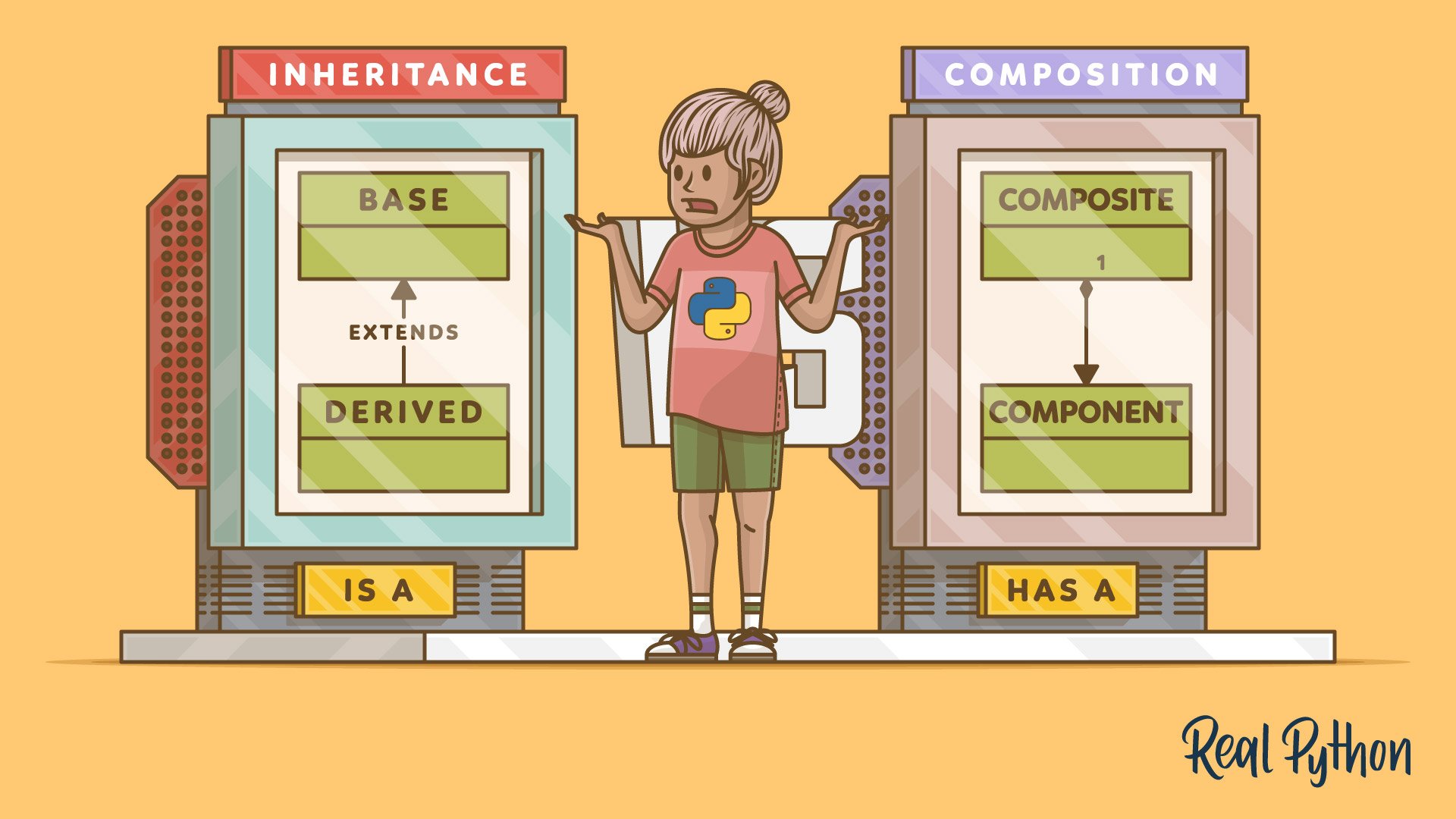
Course
Inheritance and Composition: A Python OOP Guide
Learn about inheritance and composition in Python. You'll improve your object-oriented programming (OOP) skills by understanding how to use inheritance and composition and how to leverage them in their design.
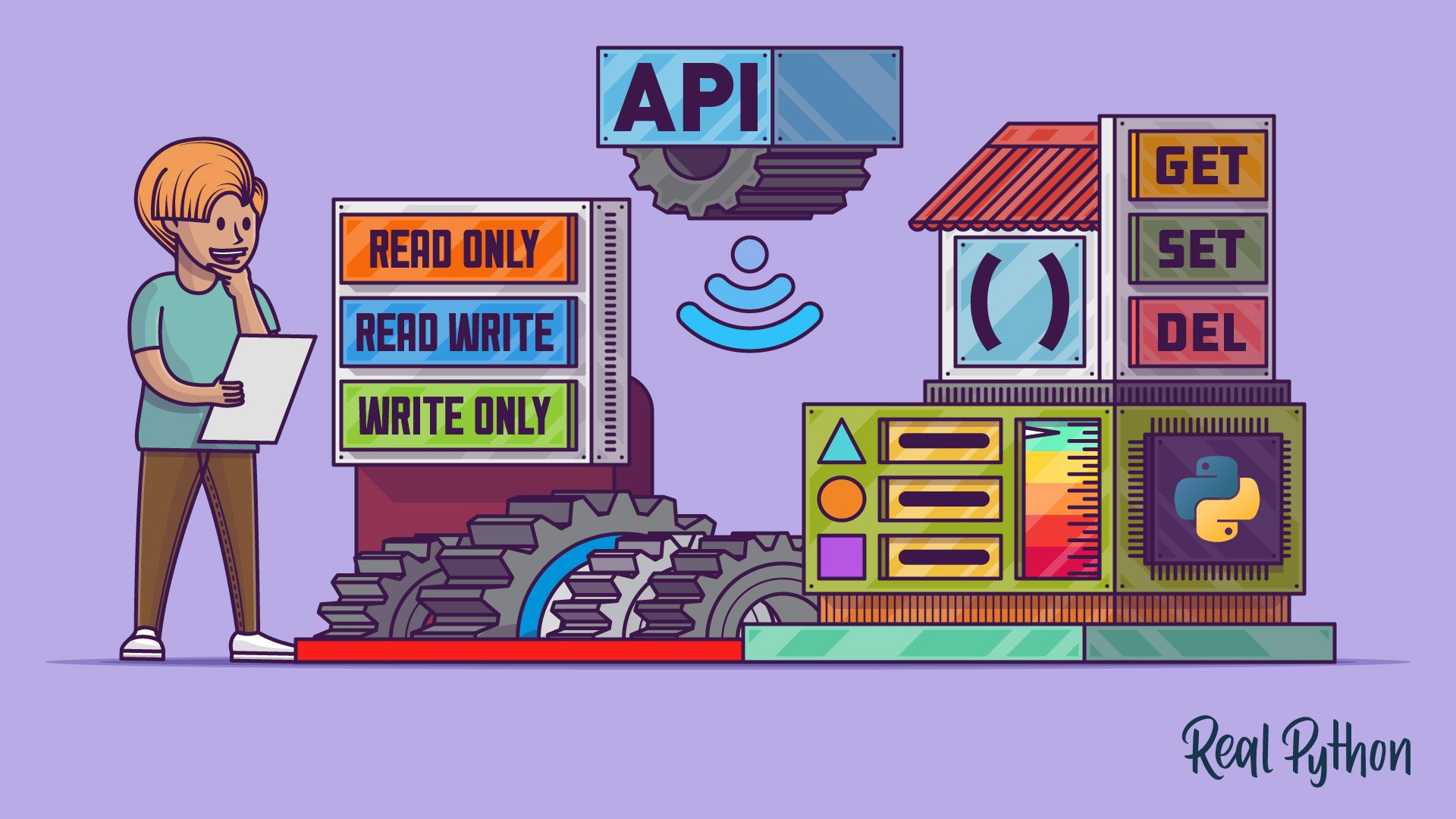
Course
Managing Attributes With Python's property()
Learn how to create managed attributes, also known as properties, using Python's property() in your custom classes.
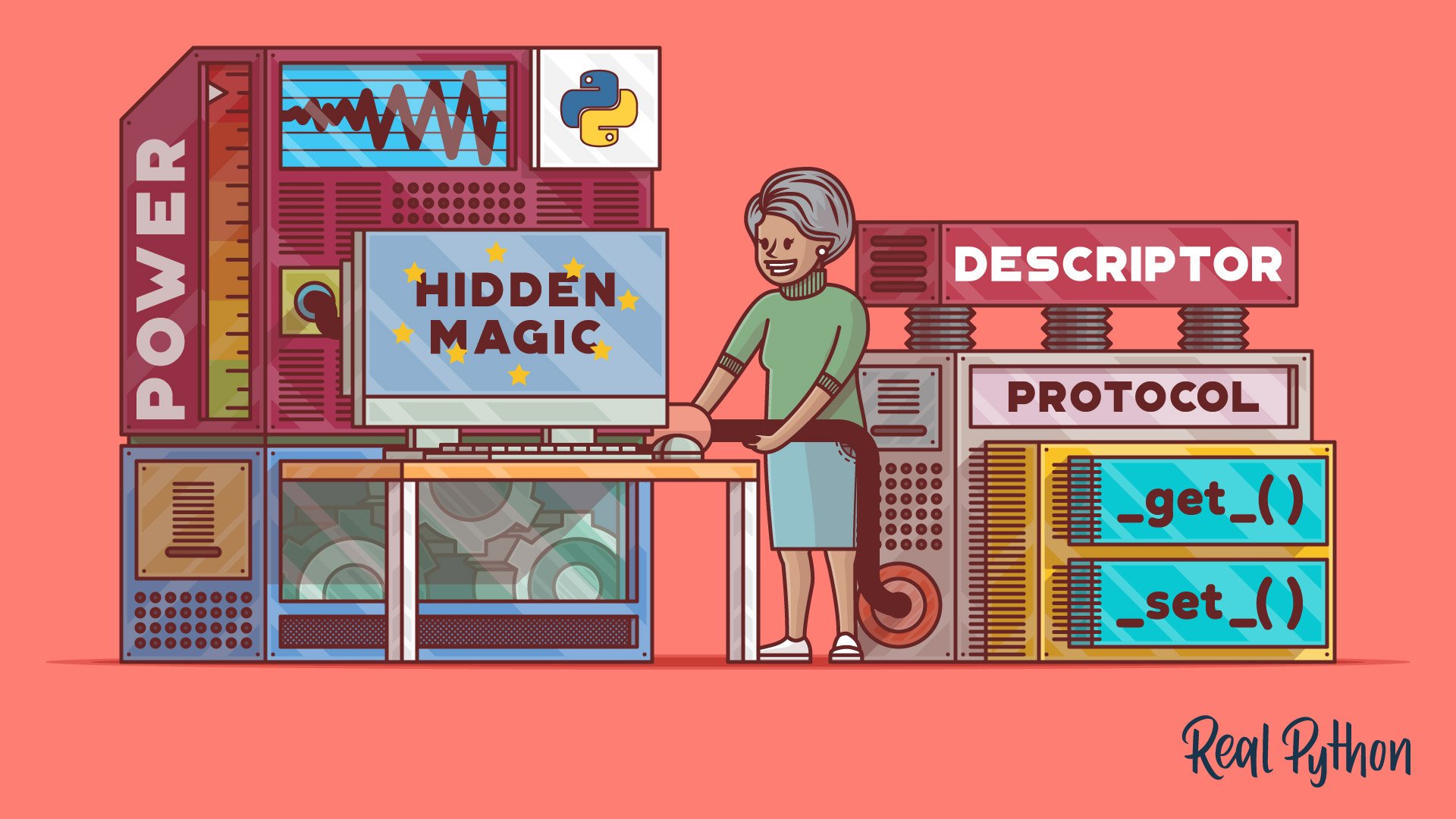
Tutorial
Python Descriptors: An Introduction
Learn what Python descriptors are and how they're used in Python's internals. You'll learn about the descriptor protocol and how the lookup chain works when you access an attribute. You'll also see a few practical examples where Python descriptors can come in handy.
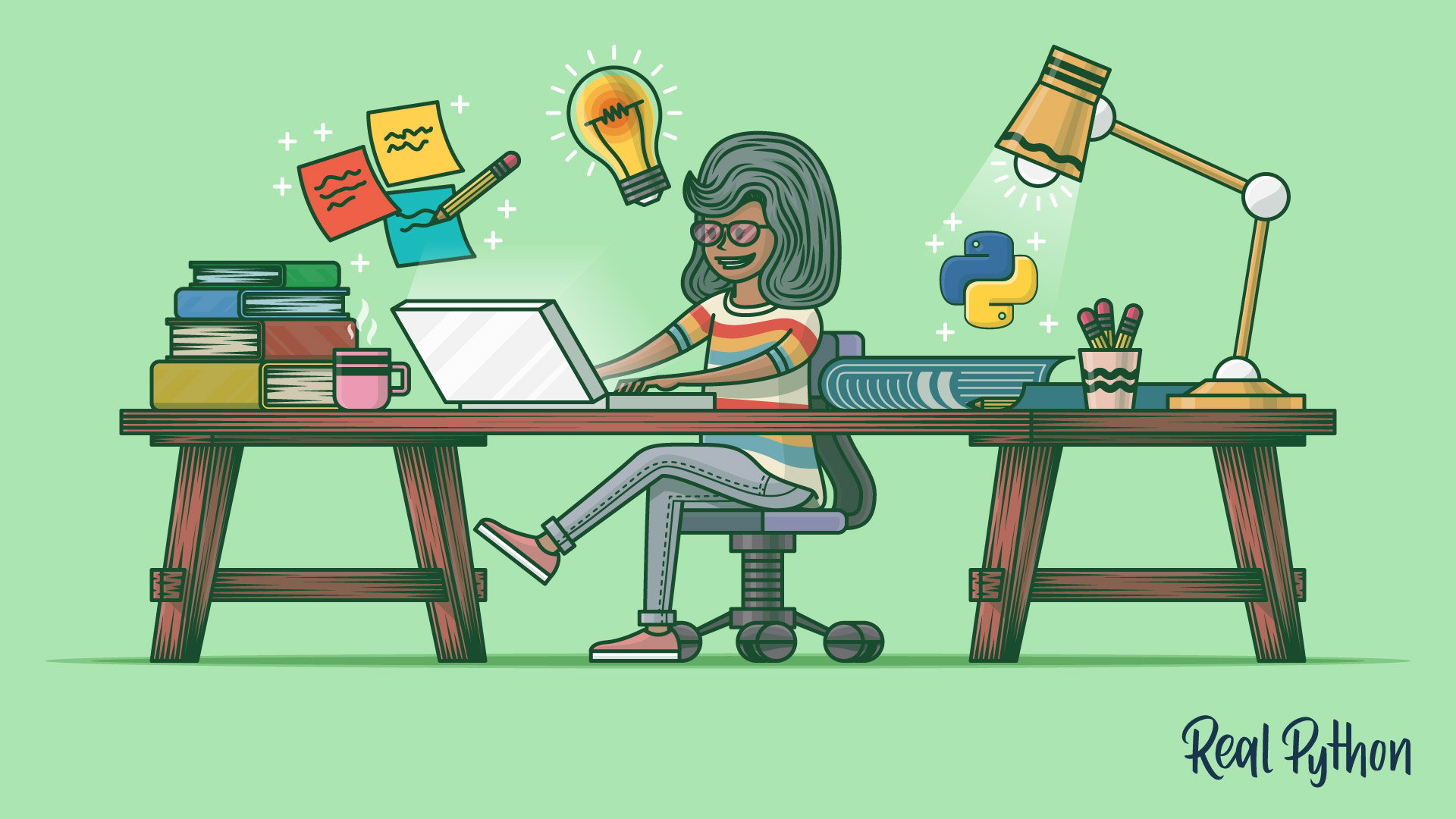
Course
Pythonic OOP String Conversion: .__repr__() vs .__str__()
In this tutorial series you'll do a deep dive on how Python's to-string conversion using the .__repr__() and .__str__() "magic methods" works and how you can add implement them in your own classes and objects.
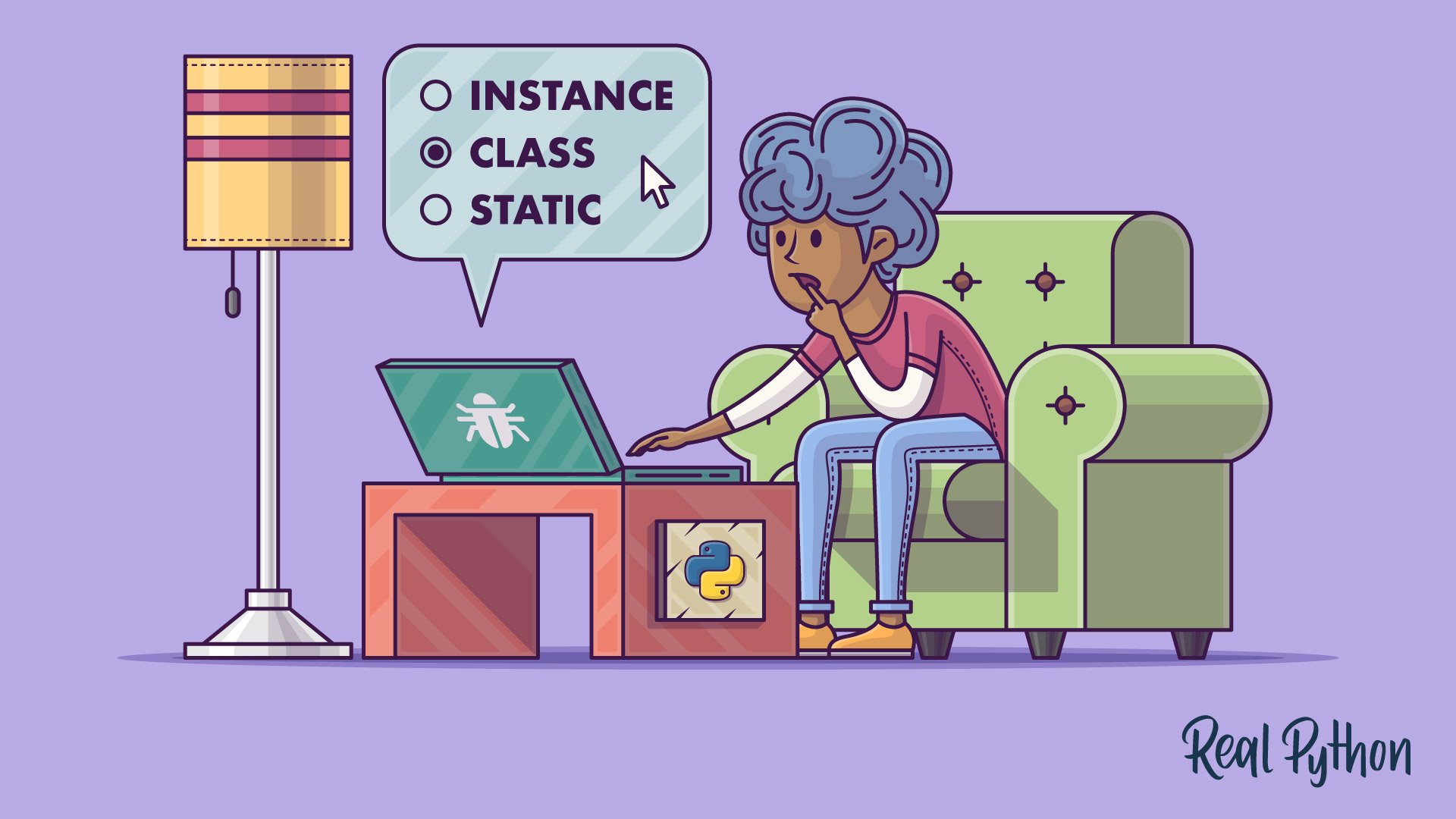
Course
OOP Method Types in Python: @classmethod vs @staticmethod vs Instance Methods
What's the difference between @classmethod, @staticmethod, and "plain/regular" instance methods in Python? You'll know the answer after watching this video course.
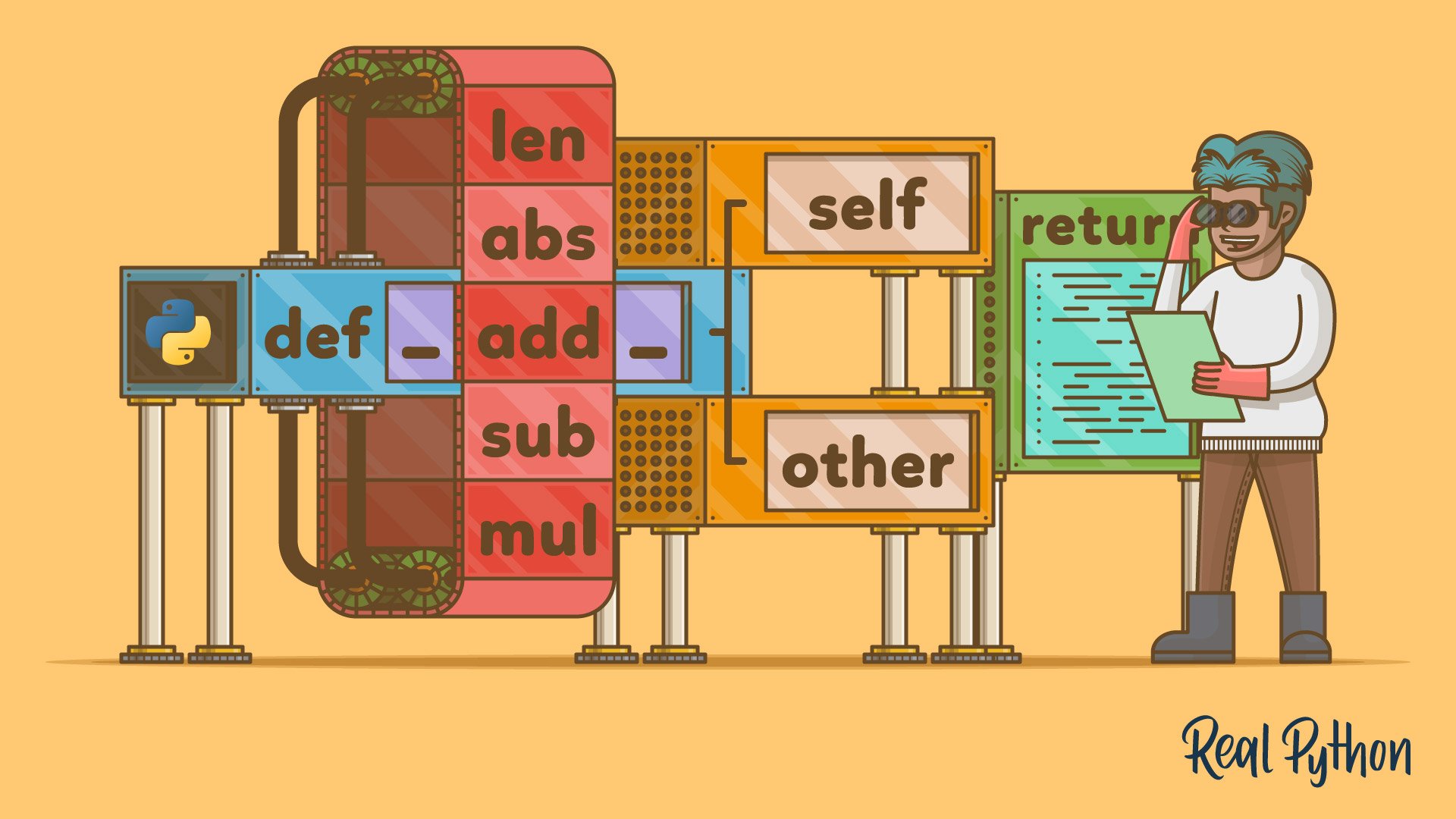
Tutorial
Operator and Function Overloading in Custom Python Classes
How to overload built-in functions and operators in your custom Python classes in order to make your code more Pythonic.
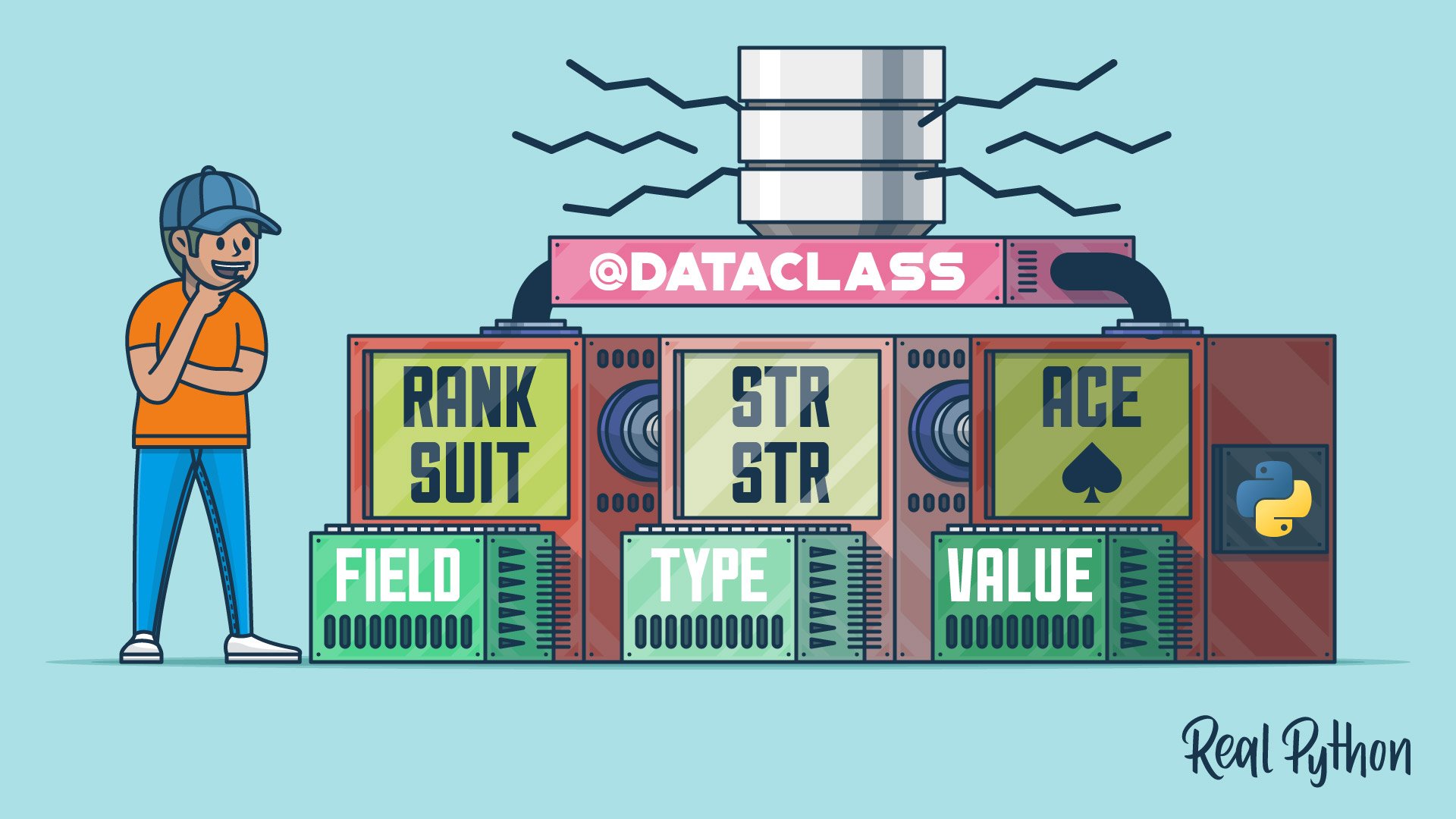
Course
Using Data Classes in Python
Data classes are one of the new features introduced in Python 3.7. When using data classes, you don't have to write boilerplate code to get proper initialization, representation, and comparisons for your objects.
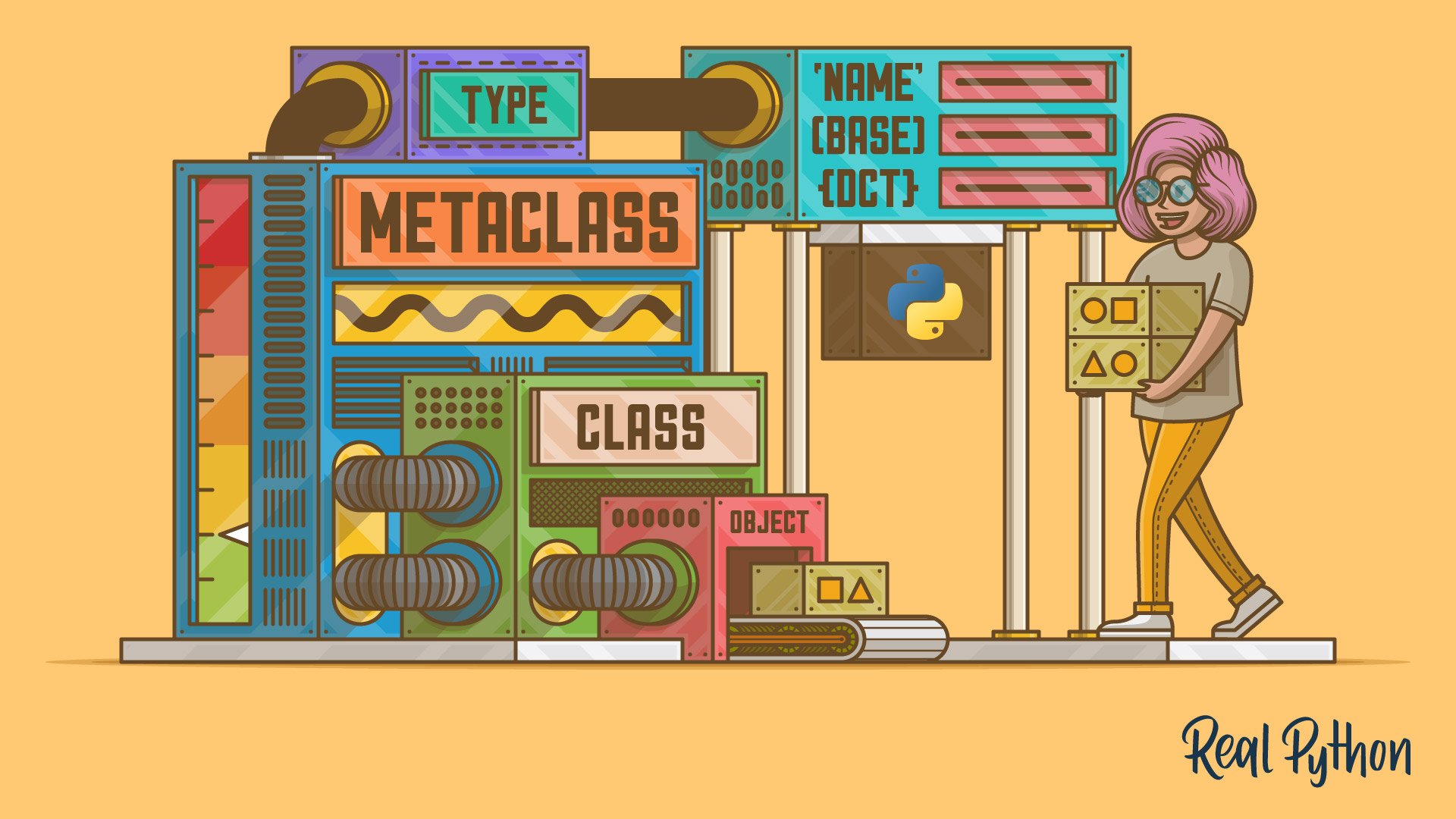
Course
Metaclasses in Python
Metaclasses are an important but mysterious behind-the-scenes mechanism for instantiating classes in Python. In this video course, you'll learn how Python's metaclasses work in object-oriented programming.
Got feedback on this learning path?
Looking for real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session. Happy Pythoning!