The Basics of the any() Function
00:00
In this lesson, you’re going to cover the basics of any()
. There are only a few things that you need to grasp to use any()
in its most basic form. The subtleties of the any()
function and how it works will be covered in more detail as you progress through the course. So the basics are it accepts one argument, and that is an iterable, so that means it must be a list ([]
), a set ({}
), a dictionary ({k:v}
), or a tuple (()
).
00:24
And it returns one Boolean value. That is, it must be True
or False
.
00:31 To illustrate, you’re going to look at this fictional situation of a list of cities or selection of places that may be hot spots or not, and you’re interested to know whether any of these places are hot spots.
00:43
As you can see, this data has one True
value, so our expected result is that this should be True
.
00:53
The first example is a list. There is just one True
value, and the way you use this is you call the any()
function, which is built in, so you don’t need to import it or anything.
01:05
And then you just put in this variable, which in this case is a list, so it’s valid. And it will return True
because there’s at least one True
value in this list.
01:17
The same also works for a set. So now we’re overwriting the is_hotspot
variable with this new set, which has the same values. In this example, using a set doesn’t make much sense because a set can only have unique values.
01:35
So now it’s being condensed to False
and True
. That said, you can still use the any()
function on it, and since there is at least one True
value there, it will return True
.
01:51 This is also true of a tuple,
01:56
which is indicated by the normal brackets (()
). This will return True
again because there is at least one True
value in this tuple.
02:08
You can also use any()
with dictionaries. Take this fictional dictionary. You have just one True
value, Fraggle Rock
, which is a hotspot.
02:18
All the rest of the values are False
. So where you’d want to use any()
in this case is to return True
if any of the values are true. A thing to remember, though, with any()
, is that if you pass it in a dictionary, as it’s iterable, it will evaluate the keys and not the values.
02:36
So if you’re going to use any()
with a dictionary, you have to make a call to .values()
. The .values()
method will return an iterable of the values of the dictionary. If you don’t do this, then you’ll still get a True
value in this case, even though all the values are False
.
03:07
As you can see, you get True
even though all the values are False
, and this is because it is evaluating the keys of the dictionary.
03:16
How exactly any()
evaluates different values into Boolean values will be covered in a later lesson. And there you have it. That’s the basics of using the any()
function.
03:30
It accepts one iterable, and it returns one Boolean value. Next up, you’re going to learn how to manage many or
conditions.
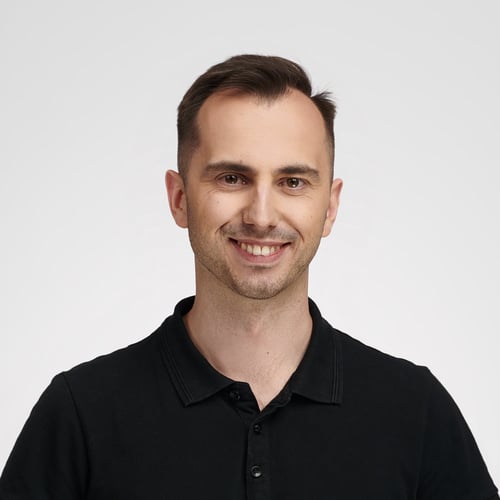
Bartosz Zaczyński RP Team on Feb. 22, 2022
@lafralafrovic The built-in function any()
expects an iterable object as an argument. When you pass a dictionary to it, it will turn it into an iterator and use that iterator to loop over the elements:
>>> d = {"a": False, "b": False}
>>> any(d)
True
>>> any(iter(d))
True
>>> any(list(iter(d)))
True
>>> list(iter(d))
['a', 'b']
Iterating over a dictionary in Python is equivalent to iterating over its keys. In this case, "a"
and "b"
are both truthy, so any()
returns true.
On the other hand, to explicitly iterate over the values, you need to call the .values()
method on a dictionary:
>>> any(d.values())
False
>>> any(list(d.values()))
False
>>> list(d.values())
[False, False]
This will supply a list-like view of the values stored in the dictionary.
Become a Member to join the conversation.
lafralafrovic on Feb. 22, 2022
This simple piece of code gives me True
I need to do
print(any(d.values()))
to get False. What am I doing wrong?