Basic Configurations
You can use the basicConfig(**
kwargs
)
method to configure the logging:
You will notice that the logging module breaks PEP8 styleguide and uses
camelCase
naming conventions. This is because it was adopted from Log4j, a logging utility in Java. It is a known issue in the package but by the time it was decided to add it to the standard library, it had already been adopted by users and changing it to meet PEP8 requirements would cause backwards compatibility issues. (Source)
Some of the commonly used parameters for basicConfig()
are the following:
level
: The root logger will be set to the specified severity level.filename
: This specifies the file.filemode
: Iffilename
is given, the file is opened in this mode. The default isa
, which means append.format
: This is the format of the log message.
By using the level
parameter, you can set what level of log messages you want to record. This can be done by passing one of the constants available in the class, and this would enable all logging calls at or above that level to be logged.
00:00
We can modify the default logger by using the basicConfig()
method. You might notice that this method is named a little bit differently than most others. That’s because it’s using camel casing, which goes against the PEP 8 standard.
00:15
This is because the logging
module was adopted from a logging utility in Java, and by the time it was formally decided to add it into the standard library, there was already lots of legacy Python code using it, so renaming it would cause backwards compatibility issues.
00:31
I guess that’s what you get when you rely on Java for anything. Anyway, the basicConfig()
method takes lots of different parameters, but I’ll just mention the four most commonly used. level
sets the minimum severity level to log.
00:47
filename
defines a file to write log output to. filemode
defines the mode in which the file should be opened in, which is 'a'
for append by default. And format
defines how the log message should be formatted.
01:03
Here’s an example of using the basicConfig()
method. Here, we can pass the level
parameter a value of logging.DEBUG
so that all event levels DEBUG
or greater are logged.
01:18
The output of this program will look similar to before, except now we’re logging DEBUG
events or higher.
01:26
Here’s an example of how we can use the other three common parameters to log to a file. We’ll call the filename
'app.log'
and give it a filemode
of 'w'
for write.
01:39
This will overwrite the log each time the program is run. If we don’t set this filemode
ourselves, 'a'
for append will be used, which will simply append any new log data to the existing file.
01:52
Finally, we use the format
parameter, which in this case will log the logger name, the level of the event, and the message separated by dashes. Here, name
, levelname
, and message
are all built-in variables that represent exactly what you think they would. As we’ll see later, you can also pass in your own variables in place here.
02:17
And if we run this program, we’ll get this output in our external log file: root - WARNING - This will get logged to a file
.
02:28
Notice how we didn’t include the level
parameter. Remember, the logger’s default level is WARNING
, and so that wasn’t required because our only event in the program is marked as a warning.
02:40
If we had an event marked as less severe, like INFO
or DEBUG
, it wouldn’t be logged in this example.
02:50
It should be noted here that the basicConfig()
function can only be called once. The severity level functions used to assign an event a severity level will implicitly call basicConfig()
if it has not been called before. This means that after the first time one of the severity functions is called, you can no longer call basicConfig()
on the default logger, because those functions would have already called basicConfig()
. In simpler terms, let’s make sure to call basicConfig()
before we start logging any events.
Austin Cepalia RP Team on July 24, 2019
Haha :)
techdiverdown on April 14, 2020
Code Java for many years, even worked for BEA at one time. Python is bringing the joy of programming back that Java extracted from my soul.
sshekhar on Oct. 20, 2022
As someone with 20 years of Java background I felt that Java dig :-)!
andrewodrain on Oct. 17, 2023
Hello,
I ran into an issue around 1:04. I was not getting any output from:
logging.basicConfig(level=logging.DEBUG)
logging.debug('This will get logged')
I discovered that I had to set the root logger to DEBUG this way first:
logging.getLogger().setLevel(logging.DEBUG)
Then I ran this again, and got the output:
logging.basicConfig(level=logging.DEBUG)
logging.debug('This will get logged')
I think it has something to do with the root logger being set to ‘WARNING’ by default, and the fact that basicConfig(level=DEBUG) doesn’t retroactively change the level of other logger instances.
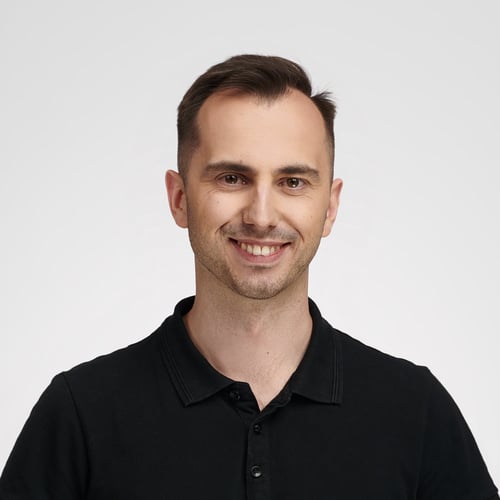
Bartosz Zaczyński RP Team on Oct. 18, 2023
@andrewodrain If you’re finding the standard logging framework in Python confusing, then you might like the third-party loguru package better. It advertises itself as “stupidly” simple.
Become a Member to join the conversation.
Kevin Dienst on July 24, 2019
Love the jab at Java. 😏