Creating a Calculator App
00:00 Creating a Calculator App To round off your knowledge of PyQt, you’ll develop a calculator GUI app using the model-view -controller design pattern. This pattern has three layers of code, with each having different roles.
00:15 The model takes care of your app’s business logic. It contains the core functionality and data in the calculator app. The model will handle the input values and the calculations.
00:26 The view implements your app’s GUI. It hosts all the widgets that the end user needs to interact with the application. The view also receives a user’s actions and events.
00:38 In this example, the view will be the calculator window on screen. The controller connects the model and the view to make the application work. Users, events, or requests are sent to the controller, which puts the model to work.
00:52 When the model delivers the requested result or data in the right format, the controller forwards it to the view. In the calculator app, the controller will receive the target math expressions from the GUI, ask the model to perform calculations, and update the GUI with the result.
01:11 Here are the steps the GUI calculator will take to function. The user performs an action or request on the GUI. The view notifies the controller about the user’s action.
01:23 The controller gets the user’s request and queries the model for a response. The model processes the controller’s query, performs the required computations, and returns the result.
01:35 The controller receives the model’s response and updates the view accordingly, and the user finally sees the requested result on the view. So now you know how the MVC design pattern will work in practice.
01:49
Let’s get started building the calculator. To start off, you’ll implement a minimal skeleton for the application in a file called pycalc.py
.
01:58
And remember, you can get the completed version of the calculator and all the other codes you’ve seen in this course in the course materials. Start by creating pycalc.py
in your current working directory and open it up in an editor or IDE.
02:14
Then enter the code as seen. First, you import sys
. This module provides the exit function, which you’ll use to cleanly terminate the app. These lines import the require classes from PyQt6.QtWidgets
.
02:30 You’ll be importing more classes later so the line is structured to allow this future expansion to be handled cleanly.
02:38 Here you create a Python constant to hold a fixed window size in pixels for the calculator app.
02:46 This creates the PyCalcWindow class to provide the app’s GUI. Note that this class inherits from QMainWindow.
02:55
These lines define the class initializer and call __init__
on the superclass for initialization purposes.
03:05 Here you set the windows title to PyCalc.
03:10
This uses setFixedSize
to give the window a fixed size. This ensures that the user won’t be able to resize the window during the app’s execution.
03:22
These lines create a QWidget
object and set it as the windows central widget. This object will be the parent of all the required GUI components in the calculator app.
03:35
The next lines will define the calculator’s main()
function. Having a main()
function like this is best practice in Python as it provides the application’s entry point. Inside main()
, you perform the following: create a QApplication
object named pycalcApp
.
03:53 Create an instance of the app’s window called pycalcWindow,
03:59
show the GUI by calling show()
on the window object,
04:04
and run the application event loop with exec()
. The last line calls the main function to execute the calculator app using the `__name__ == ‘__main__’ convention.
04:18 When you run the script, you’ll see the application on screen and while this clearly isn’t the most exciting of applications at this point, it is the foundation for the GUI calculator.
04:30 So with this groundwork complete, you’re ready to continue building the project and that’s what you’ll do in the next section of the course.
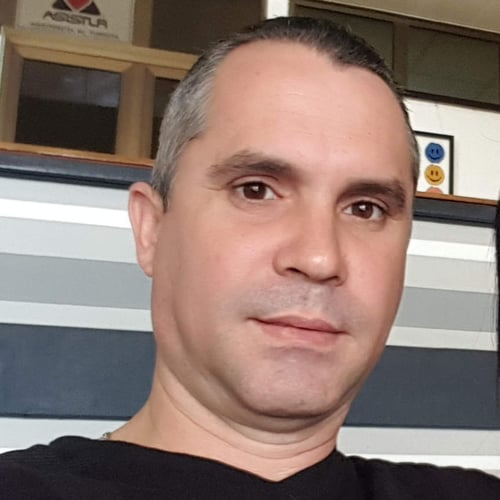
Leodanis Pozo Ramos RP Team on Aug. 13, 2024
@Ariba S Hey! In this case, main()
isn’t a method but a top-level function. Your linter may be complaining because of a wrong indentation? The main()
function isn’t a method of PyCalcWindow
, so it must be a top-level function in the module.
Become a Member to join the conversation.
Ariba S on Aug. 12, 2024
Hello, my Pylint complains about the main method not having any arguments. Should be this re-written as a static method?