Learning the Difference Between a Variable Name and the Object
00:00
Another very common built-in immutable type in Python is the string. Let’s create a string, for example, greeting = "Hello, Pythonistas!"
And you can confirm that greeting
, the string, is immutable by changing for example, let’s try to change the last character.
00:18 Let’s say that you don’t like the exclamation mark, you want to change it with a period or full stop. And if you try to do that, you get a similar error to the one we got in the previous lesson when we tried to make a change to a tuple.
00:32
The TypeError
says: ‘str’ object does not support item assignment. So same issue. We cannot change any value in a string. Why? Because this string is immutable.
00:44
Once you’ve created a string, you cannot change it. Now you might say, well, but is that really true? For example, can’t I do you might be asking, something like this: greeting = "Hello!"
. You hit Enter, no errors.
01:00
And if I find out what greeting
is, it is indeed the new string. So you might be wondering, does that mean that strings can change? And the answer is no, they can’t.
01:12 And what’s happening here is something that’s key to understand in Python. There’s a difference between the object and its name, which we often call the variable.
01:22
There are two string objects that you’re creating here. "Hello, Pythonistas!"
and "Hello!"
. When you create "Hello, Pythonistas!"
, initially you assign it to the variable name greeting
.
01:33
However, when you reassign greeting
to "Hello!"
, that’s a new string. You’re not changing the original object. The original string remains the same.
01:45
It’s immutable, so you cannot change it. Instead, you create a brand new object, the new string, "Hello!"
, and you reassign that to the same variable name.
01:55
So from this point onwards, the variable name greeting
no longer points to the original object, it points to the new object, but importantly, the object itself has not changed.
02:07 Strings cannot change because they’re immutable.
02:11
You can confirm this. Let’s go back to greeting = "Hello Pythonistas!"
.
02:16
And you can find the identity of greeting
. The built-in id()
function finds the identity of an object, not of the name greeting
, but of the object that this name is pointing to.
02:32
So in this case, the string object, "Hello,
Pythonistas!"
has a certain identity. You’ll get a different number when you try it out.
02:39 In fact, you’ll get a different number every time you try it out. It doesn’t matter what this number is. In CPython, which is the most common interpreter in Python, this refers to the memory location.
02:50 What matters is that this number is unique to every object. This object is the only object that can have this number for the duration of my program.
03:01
So let’s try to reassign greeting
to "Hello!"
and find the identity of greeting
again, it’s the same variable name, but it’s not the same object.
03:13
The identity is different. You can see that this number ends in 024, whereas the previous string object, "Hello, Pythonistas!"
03:22 ends in 536. This shows us that even though the variable name is the same, the object is different. You are replacing a string object with another one.
03:33 This difference between the variable name and the object is a really important one in Python. So let’s summarize it. A Python variable name is a label. It refers to a memory location on your computer where objects live.
03:48 The Python object is the concrete piece of information, and that’s what lives in a specific memory location on your computer.
03:57 In Python, everything is an object. You may have heard this phrase many times before, and every object has three core characteristics. Let’s have a look at what they are. There’s the value.
04:08
This is if you have an integer 5
, 5
is its value. Or in the example we’ve seen in this lesson, you had the string, "Hello, Pythonistas!"
, or just "Hello!"
that’s the value of the object.
04:20
Every object has an identity. In this lesson, you’ve also seen one way of looking at this identity using the built-in id()
function, which gives you a number.
04:30 It’s like the address of where this object exists, and every object has a unique identity. So no two objects can have the same identity. They cannot have the same number.
04:41 And finally, the type. The string object is a string. An integer object is an integer. This is an important aspect of an object. The type determines how we can use an object, whether it’s mutable or immutable, and other characteristics that are specific to different data types.
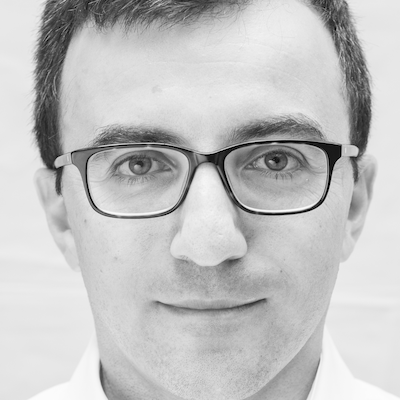
Stephen Gruppetta RP Team on Oct. 4, 2024
Well spotted! Yes, the id is unique throughout the lifetime of the object rather than the program. I think the sentiment there was that this is a value that doesn’t change and can’t be re-used by other objects, but your phrasing is indeed more accurate!
Become a Member to join the conversation.
pylon on Oct. 2, 2024
Hi, for my own learning I got hung up on the quote below.
02:50 - Learning the Difference Between a Variable Name and the Object
It’s not a big deal, but it should be clarified that an object’s id is unique for the lifetime of the object, not the duration of your program.
The following Python 3.12 (CPython) snippet shows that a Python program reuses the ids when the original object is deleted (In CPython, where the id number is somewhat related to the memory address it is obvious that they must be able to be reused when memory is freed).
or more explicit
Why the IDs alternate in the first example and not in the second is due to how GC is invoked when running in the REPL. When put in a file like this:
you get
An aside at the end; in your example here Exploring Mutable Built-in Data Types: Dictionaries & Sets integer values between -5 and 255 are used, in CPython these are interned, so you will get the same id every time. This will be surprising for students exploring the code, as the course content (on immutability) indicate the must be different.
In the following snippet I create a dictionary with some small (interned) and some larger integer values. When looking at the id of the objects they are pointing at they are the same for the interned values and different for the others.
The small id values (think memory offset) also give away the fact that these interned integer objects was created when the interpreter initialized, not at runtime.
(The interpreter will point all keys at the same integer if you add all the keys and values in the dict literal. So for this example to work I add one key/value per line. Same if you run it as a script, the interpreter is smart enough to point the keys to the same integer object)
Liked the course, rock on :)