Importing Fundamentals
00:00 In the previous lesson, I gave an overview of the course. In this lesson, I’ll walk you through the most common ways of importing modules in Python. If you’re building a small program, you tend to put it all in one file, but as your programs get bigger, you want a way to organize them, keeping like things together. A namespace in computer science is an abstract container for the parts of your code.
00:22
A namespace might contain variables, class, or function definitions, all with the idea that if I call my function get_name
and you call your function get_name
, we don’t have to worry that we’ve used the same name as long as we have different namespaces.
00:36 Python mostly uses directory structure to define these kinds of groupings, and it uses the term ‘module’ for one of the lower levels of this kind of container.
00:45 It typically corresponds to a single Python file. A ‘package’, on the other hand, is a recursively defined structure rooted at a directory which can contain modules and more directories.
00:57
You indicate that a directory is a package in Python by including a file with a special name __init__
.py
, often pronounced dunder init.py.
01:08 Packages form the backbone of code reuse and can be bundled up for sending to other people. Python also has an extra mechanism that is similar, known as a namespace package.
01:20 This is like a package but is dynamically created, meaning you can have multiple directory structures with the same paths and treat them as a single thing.
01:28 One thing to note about all this vocabulary is that in the real world, people often misuse it. You will frequently hear folks use the term ‘module’ and ‘package’ interchangeably.
01:37 In fact, I tend to only use the word package when I mean a third-party bundle and frequently miscall packages in my own structures, modules. It isn’t strictly correct, but it is quite commonplace.
01:50 Python ships with many modules and packages, which you can take advantage of. And PyPI is a central repository for third-party installable packages and is one of the reasons Python is so popular.
02:02
There’s a package for pretty much everything. The import
keyword is what brings a module or package into your namespace. If you’re writing Python in a file, the import
keyword imports a module or package into the namespace scope of that file.
02:18 Or if you’re using the REPL, it imports it into the general namespace of the REPL. Speaking of the REPL, let’s go there and look at some of the more common types of importing.
02:30
Before importing anything, I want to introduce you to a built-in function called dir()
. The dir()
function lists everything in the current namespace.
02:38 Let me try it out. The default Python namespace already has some stuff in it, but as you’ll note from all those double underscores, it’s internal stuff, so that’s what the almost empty namespace looks like.
02:54
You use the import
keyword to import a module or package. Oh, okay. Let’s just get this out of the way now. I’m going to stop saying module or package every time.
03:03
Remember when I said I tended to use these terms loosely? Well, loose usage is in your future. What running this command has done is import the math
module into the current namespace, which is the REPL’s namespace.
03:16
I can reference things inside of the math
module using dot notation. The math
module contains some constants that are commonly used in math, like pi
.
03:26
Using dot notation like this I can get at all the things in math
, so just what did importing math
do to the namespace? It still has all those dunder things, but the namespace also now has math
tacked on at the end.
03:42
The dir()
function optionally takes arguments. You can pass it a module that you’ve loaded to see what’s in the module’s namespace. math
has a lot of stuff in it, including pi
, the constant I displayed earlier.
03:56 In addition to importing a module, you can import things from a module.
04:04
This notation says to import all the things inside of the math
module into the current namespace. Generally, this isn’t considered good practice as it clutters your namespace up.
04:16
See, the top-level namespace now has all the dunder stuff as well as all the stuff from math
. The more you do this, the more likely you’re going to get a name collision.
04:25 Python allows name collisions. It does shadowing, meaning the last thing defined is what is currently defined. More on that later, but for now, realize that the more things you have in the namespace, the more likely you have clobbered something and won’t be sure what you’re actually using.
04:41 The more common way is to import just the thing you need.
04:47
The calendar
module has constants for each month of the year. This last statement has imported only the JANUARY
constant,
04:56
and since I used the from
format, I don’t need the module name to use JANUARY
, I just use JANUARY
. In fact, I can’t even use calendar
.
05:07
I didn’t import it. I imported JANUARY
from it. Depending on what version of Python you’re using, you’ll get different error messages. Here I’m using Python 3.12, and the last couple of releases have added more helpful errors.
05:20
This one even guesses at what I’m trying to do, and although when I look at JANUARY
in the REPL, it says it is calendar.JANUARY
,
05:30
it actually hasn’t been imported like that. It has to be JANUARY
on its own. You can also rename things when you import them using the as
format of the import statement.
05:44
Here, I’ve imported the cmath
module, but I’ve renamed it complex
instead. Like with math
, cmath
also has constants in it.
05:55
This is similar to what I just did with math.pi
, but this time I’m using cmath.tau
after aliasing cmath
to complex
.
06:04
You can combine the from
syntax with aliasing.
06:13
Here, I’ve imported the MINYEAR
constant from the datetime
module and aliased it as the more readable MIN_YEAR
.
06:22
And like with JANUARY
, I use it directly. Python dates don’t do before Common Era. The minimum year is one.
06:32 Did anyone call FedEx? It’s time to talk packages. Use of the term FedEx or its counterpart, Federal Express, is not meant as an implicit or explicit sponsorship of FedEx.
06:40 Real Python and its affiliates have no relationship with FedEx. Any product names, logos, brands, or trademarks belong to their respective holders. Prolonged use of fine print disclaimers can cause nausea and heartburn. Before trying a disclaimer, you should consult a doctor. Your mileage may vary.
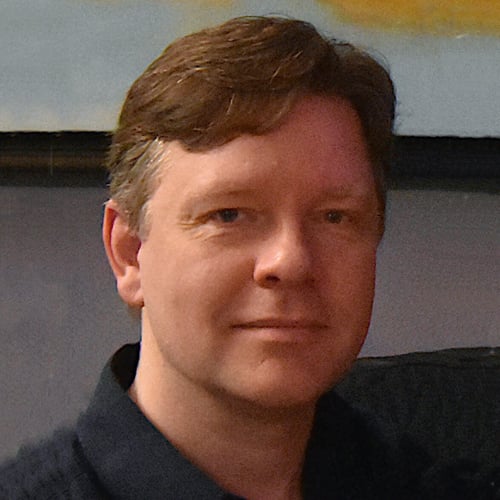
Christopher Trudeau RP Team on Feb. 25, 2025
Yep, you caught me. When I record this stuff I don’t actually use the REPL, I use a tool that helps me present. That way if I flub a line I can back up as if nothing happened. In copy and pasting the dir()
output into my tool, I must have grabbed it from two different sessions that had crap kicking around that shouldn’t be.
Given the sheer volume of output from dir()
I obviously wasn’t looking close enough to catch the mistake. Sorry for any confusion.
Become a Member to join the conversation.
Andras on Feb. 25, 2025
Hi Chris, two questions:
At around 4:30, after you did
from math import *
, what is that'americas'
doing in thedir()
output? It is most likely notmath
related. I guess it must be related to you REPL settings, right?Even more interesting, why did
'math'
disappear from the samedir()
output? I tried the same and I see'math'
on my system. Is it again some Python REPL setting to unload/remove redundant modules?thanks