List Comprehensions and Built-In Functions on Lists
In this lesson, you’ll learn how to use list comprehensions to construct various lists, and different list methods to manipulate lists.
List comprehensions are a useful way to construct lists on the fly. They use the following syntax:
[<expr> for <elem> in <lst> if <cond>]
Here’s an example:
>>> [(lambda x: x *x )(x) for x in [1, 2, -5, 4]]
[1, 4, 25, 16]
They’re used in place of functions like map()
and filter()
. To learn more, check out Using List Comprehensions Effectively.
There are also some useful built-in functions that can be applied to lists such as max()
, min()
, any()
, and all()
:
max()
finds the maximum value of an iterable using the built-incmp()
method, or thekey
param.min()
finds the minimum value of an iterable using the built-incmp()
method, or thekey
param.any()
returns whether any of the elements in the iterable are atrue
value.all()
returns whether all of the elements in the iterable aretrue
values.
To learn more, check out Python Docs Built-in Functions.
00:00 In this video, you’ll learn about list comprehensions and some useful built-in functions that you can apply to lists. Well, let’s get started with the list.
00:08
Let’s do lst = [1, 2, -5, 4]
.
00:16
Let’s define a square()
function that will just take in x
and return x * x
. Let’s say you want to apply the square()
function to each element in the list.
00:27
Well, you can use the built-in map()
function, which takes in our function square()
and lst
and returns a map
object, which we then have to cast into a list.
00:40
And there you go! You’ve got to list with each number squared. Let’s do this with a for
loop. So, result = []
. for num in lst
result.append(square(num))
.
00:57
Cool, that worked too. Let’s use list comprehensions, which are a very Pythonic way to do exactly this. We would do a bracket and then we would call square()
on the num
, for num in lst
.
01:11 So, here are three ways to do the exact same thing, but really, the last way—using list comprehensions—is the most Pythonic way and can show your interviewer that you know syntax that is used very frequently in Python.
01:26
Let’s look at another example. Let’s define a is_odd()
method, which will just return if x % 2
is 1
, basically because modulo takes the remainder, and if the remainder of dividing a number by 2
is 1
, then it is odd.
01:45
Let’s filter out elements from our list that are not odd. We could use our filter. filter()
passes in the function, and then the lst
. Then, we have to convert that to a list
02:00
and there we get [1, -5]
. Here’s how you do it using a list comprehension. [num for num in lst if is_odd(num)]
.
02:12
Let’s go through a more complicated example that you would see in many interviews. Many questions include some sort of grid or some sort of matrix, so maybe, let’s have a two by three matrix just filled with 0
’s, and define it
02:29 using lists. Here, we can see this would be row one, this would be row two, and they are both elements of a list. Well, how would you build that dynamically?
02:40
Let’s say if you were just given a variable num_rows = 2
, and num_columns = 3
. How would you build that? Well, let’s make our grid
an empty list, and use a for
loop, and then use a list comprehension. So you’d say for
blank in range(num_rows)
and then you have your current row, which is a list, and you say for _ in range(num_columns)
.
03:08
Then, you would append to the current row a 0
, and then you would append your current row to the grid
.
03:22
There we go! So, how do we do that using list comprehensions? Well, grid = [[0 for _ in range(num_columns)
for _ in range(num_rows)]]
.
03:39
Forgot a bracket. Oops. This is actually a very good bug. What happened here? Well, we did one list comprehension, [[0 for _ in range(num_columns) for _ in range(num_rows)]]
.
03:55
This executed num_columns
times, and then we did that num_rows
times, and then all the 0
’s were in one long list. What we really want is lists of lists, so we just forgot a bracket, there, and then we added an extra one at the very end.
04:17 Cool. It’s always good to verify your output looks correct before just jumping to the next part of the problem, just to make sure you don’t have a silly bug like this.
04:28
Let’s move on to some useful built-in functions. max()
takes in some numbers and finds the max number, but it also can take in a list. So, looking back at that lst
we created at the beginning, max(list)
would be 4
. What if we want to find the number that has the max square? Well, we pass in a key
param to lst
.
04:51
key=square
—that would work, but I’m actually just going to redefine it as a lambda, just to show that you can do this all in one line and make it really clean, maybe in an interview or something like that.
05:04
This will output -5
, because -5
has the largest square. min()
is the same, but as you can imagine, it finds the minimum value. It takes in a list as well, and a key
.
05:20
1
. Cool! any()
is a useful function that takes in a iterable and returns True
if any of the values in the iterable are a True
value, and it returns False
if none of the values in the iterable are True
values. So, what does that mean?
05:37
Well, any()
of our list is going to look through our list [1, 2, -5, 4]
, call bool()
on them, which is this method bool()
, which converts that to True
or False
depending on if it’s a True
value or a False
value, and then any()
will return True
—yeah, based on if any of them are True
, and False
if none of them are True
.
06:00
So any()
of our list is going to return True
because 1
, 2
, -5
, and 4
are all True
values.
06:07
This might make more sense if we make a new list, like [True, False]
or [False, False]
. Let’s use any()
to check if any of our values in our list are odd. Well, how would we do that? any()
of our list, and then key=is_odd()
—no, lambda
…
06:30
like this, just do it all nice and in one line. Oh! any()
, for whatever reason, doesn’t take in a key
argument, not sure why. min()
and max()
do, but this does not work. So, we have to sort of do a weird shortcut.
06:47
We have to use list comprehensions to basically apply this function to each one of our values in our list, and then check if any of those values are True
. So here—and I’m going to do something sort of interesting here—I’m going to do it all in one line in case, you know, you have to do a one-line, or you have to complicate things, or you didn’t have time to define it beforehand.
07:12
Something like this, where we would do (num) for num in lst
. That would return [True, False, True, False]
because 1
is odd—yeah, you can see.
07:26
And then, we would call any()
on that resulting list, and that would basically return, “Are any of our numbers odd?” Okay, you might be wondering, is there an all()
one that checks if all of them are odd? all()
.
07:44
all()
is the same as any()
, except all()
returns True
if all of the numbers in the list evaluate to a True
value.
07:50
Otherwise, it returns False
. So, this concludes the video on list comprehensions and list methods. In the next video, you’ll learn different ways to debug in Python.
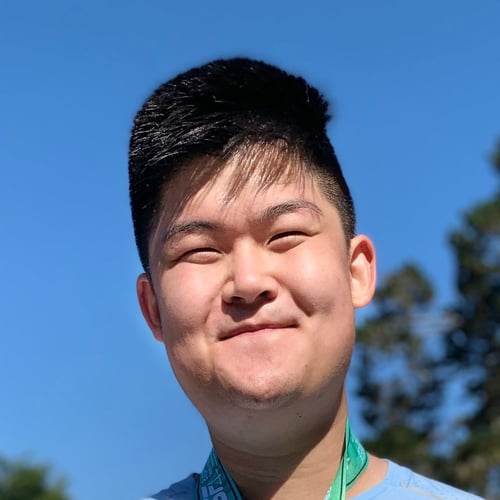
James Uejio RP Team on April 26, 2020
@Piotr Good point! Either is fine in my opinion, bool() will assume that the user knows that 0 is a False value in Python (some languages it might be a True value), while lambda is more explicit.
MichaelKareev on July 12, 2020
Probably it’s even more Pythonic for squaring list’s elements:
[x**2 for x in lst]
sanketkamat10 on Feb. 1, 2022
Using the following code as well gets the same result instead of using lambda:
any([i % 2 == 1 for i in lst])
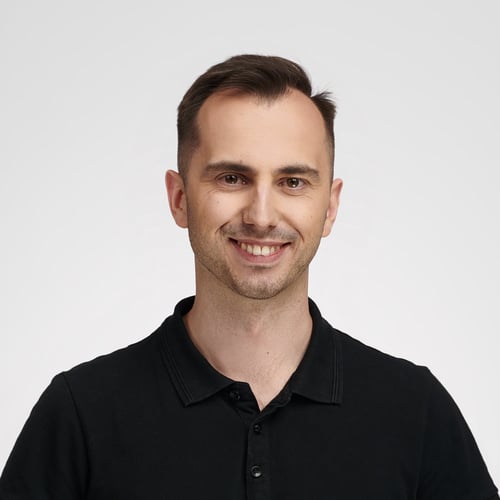
Bartosz Zaczyński RP Team on Feb. 3, 2022
@sanketkamat10 As long as you’re only interested in a simple yes-no answer, then you can simplify your example even further by dropping the square brackets:
any(i % 2 == 1 for i in lst)
Such syntax declares a generator expression instead of a list comprehension.
Rinkalaone on March 9, 2022
In case of using a boolean function with the built-in filter as in :
def is_odd(x):
return x % 2 == 1
lst = [1, -2, 9]
oddlist = list(filter(is_odd, lst))
I wonder if there is a way to pass the boolean inverse of that function to the built-in filter? Something like:
evenlist = list(filter(not(is_odd), lst))
…which is bringing the error “bool is not callable”.
Thanks James! Great course.
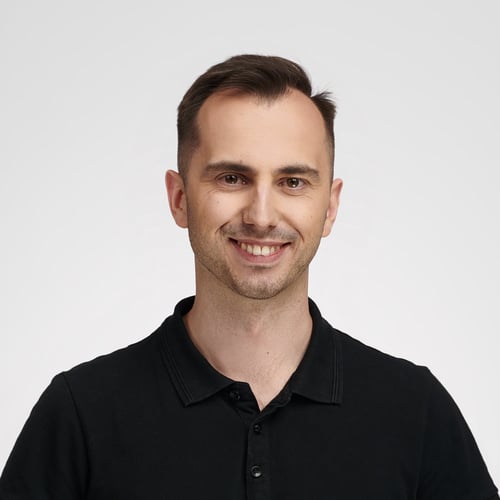
Bartosz Zaczyński RP Team on March 9, 2022
@Rinkalaone You could try using a decorator like this:
>>> def is_odd(x):
... return x % 2 == 1
>>> def not_(predicate):
... def wrapper(x):
... return not predicate(x)
... return wrapper
>>> numbers = [1, -2, 9]
>>> list(filter(is_odd, numbers))
[1, 9]
>>> list(filter(not_(is_odd), numbers))
[-2]
Rinkalaone on March 10, 2022
Awesome! Thank you Bartosz.
Become a Member to join the conversation.
Piotr on April 25, 2020
I wonder what’s the benefit of using
over simply
?