Pick Apart Your User's Input (Solution)
00:00
Here we are. I created a new file called first_letter.py
and just took a quick note of the task, which is to return the uppercased first letter entered by the user.
00:11
That means I’m going to have to collect the user input again. I will again assign that to a variable called user_input
00:18
and then just call the input()
function with a prompt, "Tell me your password: "
.
00:28 Add a space to make it display nicer here.
00:33
That should collect the user input, so whatever the input is going to be saved to the variable user_input
. And then I need to get the first letter of that.
00:42
I’ll be explicit and assign the first letter to a new variable that I’ll call here first_letter
, and then I will use user_input
, which will hold the string that the user input and then use indexing to get the first character by using square brackets and putting in 0
.
01:03
So I want the character at position zero. That’ll be the first letter. And then the task is to display that back with a short message. So again, I will use the print()
01:17
function and the string in here that says "The first letter you
entered was"
, put a colon and a space, and then we’ll concatenate this with my variable, the first_letter
variable, but it will also uppercase it, .upper()
.
01:39
So I could also uppercase it here. I could say user_input[0].upper()
. That would work as well, but that’s a bit less readable, especially because for now I just called the variable first_letter
.
01:53
I could change it to calling it first_letter_uppercase
and then add the .upper()
right after getting the first letter using the index.
02:06 But anyway, so there are always different types of solutions. If you solved it in a different way, that’s fine as well. I’m going to stick with this, where I uppercase the first letter before printing it out.
02:19
Okay, let’s see whether it works. I’m going to press F5 to run the script. Tell me your password:
and I will say No
, and The first letter you entered was: N
.
02:30
Let’s try it again with a different input. Tell me your password: PaSSworD
, and it tells me The first letter you entered was: P
.
02:42 Let’s see whether the uppercasing works. I actually haven’t tested it because both of the inputs already had an uppercase. I will run it yet another time.
02:51
Tell me your password:
and I’ll start with lowercase pwd
, and The first letter you entered was: P
, so it uppercased it correctly.
03:00 Great. So this is a working solution, and I’m satisfied with it. Did you get something else? If you did, then post it down in the comments.
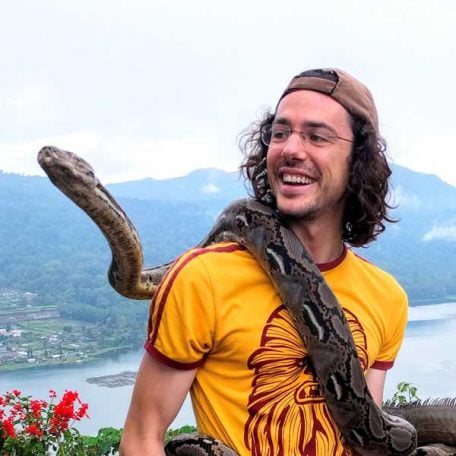
Martin Breuss RP Team on Jan. 3, 2024
@JulianV nice solution, and a good use of an f-string :)
tracyelliott79 on Feb. 18, 2024
Hi Martin :)
I coded it this way:
user_input = input(“Tell me your password: “)
print(“The first letter you entered was: ” + user_input[0].upper())
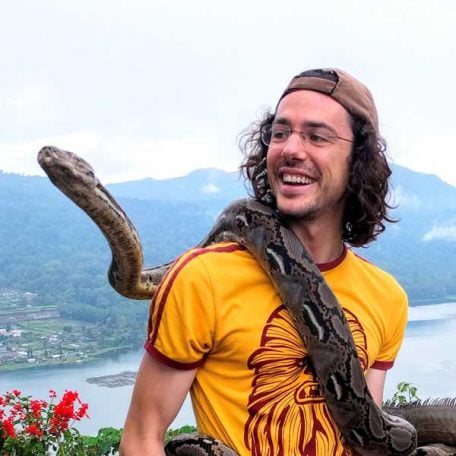
Martin Breuss RP Team on Feb. 19, 2024
Hi @tracyelliott79, and nice solution :D
rombaut1977 on Jan. 19, 2025
My solution was:
user_input = input("Tell me your password: ")
print(user_input[0].upper())
Is it really necesaary to use another variable?
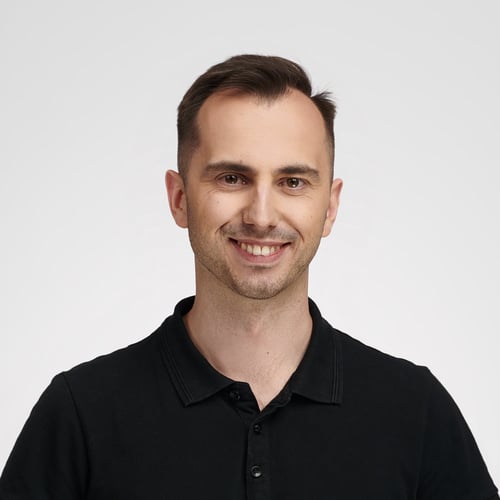
Bartosz Zaczyński RP Team on Jan. 20, 2025
@rombaut1977 Your solution looks perfectly fine, and you’re right that you don’t really need a variable here. However, declaring a variable can sometimes help improve the readability of your code, allowing you to give the variable a meaningful name and break a long line of code.
Become a Member to join the conversation.
JVargas on Dec. 29, 2023
Hi Martin and RP Team,
I coded this way:
Cheers and happy coding.