Responses: Status Codes
What are HTTP status codes? Which status codes do exist? These questions are answered in this lesson of the Making HTTP Requests With Python course.
You’ll learn about the different status code ranges and what they mean internally.
Additionally, you’ll learn how to handle different status codes in multiple ways, e.g. by using if
-statements or try-except
-blocks.
00:00 Status codes are issued by a server in response to a client request. Here’s a few examples. In the 100 series, it’s information. 200 series indicates success—like the request was received, understood, and accepted. 300 means a redirection. Somewhere in the 400 series means a client error, and the 500 series means a server error.
00:22 There’s a lot more information that can be found on Wikipedia with an entire list of the HTTP status codes. Using status codes, we can make decisions inside of our code.
00:33
Let’s create a script to work with. I’m using Visual Studio Code. Inside of here, I’m going to make a new file. I’m just going to call it script
, save it as a Python file.
00:46 I’m going to change my window layout a little bit, and I’m going to exit out of the REPL here. Great. You can see that I’ve already changed my directory, so I’m inside of that folder.
01:02
So, for our script we need to import requests
, we need to set up the URL we’re going to go to, which we can change later if we’d like.
01:14
We’ll keep using the Root Rest API. We’re going to create a response
. Let’s save our GET
request into it. So down here, we’ll do a bit of logic, though.
01:27
If the .status_code
equals 200
, we’ll print to the console 'Success!'
elif
… the .status_code
is 404
, we’ll say that the resource is not found.
01:47 I’m going to save, so down here in my terminal I can run my script.
01:59
It’s a Success!
Let’s try changing it to that invalid URL.
02:08
Save it again, and we can run it again, and this time it comes back with a 404
, Not Found.
The requests
library goes one step further in simplifying the process for you.
02:19
The Response
instance has a conditional expression inside of it that will evaluate to True
if the .status_code
is between 200
and 400
—or it’ll just be False
, otherwise. So we can simplify our last script inside of the if
statement.
02:34
We can simply say if response
—meaning if response
is True
—then it’s a success, else
… we’ll change it to say an error occurred.
02:52
We can run our script again after saving. And in this case, since it’s an invalid URL, it’s coming back with An error has occurred
. Change it back to the valid one—just getting rid of the statement here… and save. Let’s try running it again.
03:13
Success!
Keep in mind that this method is not actually verifying the .status_code
is equal to 200
. It’s simply just saying it’s within the range between 200
and 400
.
03:24
There are codes that come back like 204
—that means that there’s no content—or 304
would indicate that something’s not modified.
03:30 So just make sure that this is just a shorthand to indicate that there was a successful return, but it doesn’t necessarily mean that you got content back.
03:37 What may work better, in this case, for having errors come back—let’s see if we can look at what the errors are. So we’re going to change our code a little bit here.
03:47
We’re going to keep with the import of requests
, and then from requests
we’re going to add something additional—a set of exceptions.
03:56
In this case, one called the HTTPError
. We’re going to create a little for
loop.
04:07 We’ll have, in this case, a list with our valid and our invalid versions of the address.
04:22
Okay. This looks a little bit cleaner. import requests
, from requests.exceptions
we’re going to import HTTPError
. We’ll set up a for
loop—for a URL with the first API, which is the standard one that we’ve been using, and then we’ll have a second one, which is going to the invalid one. From there, inside the for
loop—oh, I need to put a colon on the end—we’ll try
and we’ll look at our response
here.
04:52
So it’ll try each url
. I’ll put a note here, # If the response is successful, no Exception will be raised
. Here, we’ll use .raise_for_status()
.
05:13
We’ll save the HTTPError
as a variable called http_err
. Make a little bit of room here. And we’re going to print this out using f-strings.
05:30 Note that f-strings are only available in Python 3.6 or higher. There are additional tutorials about using f-strings available on the Real Python site, so you can search there if you have questions about it.
05:45
And then if it’s not the HTTPError
, we’ll except as an Exception
, save that as a variable err
and print out that error.
05:58
else
, if we make it all the way through the try
statement, we can print 'Success!'
Okay, I’m going to save, go down here to terminal, and we’ll run my script.
06:10
So, the very first time it came through and it was a Success!
That was going here, to the normal api.github.com
. And the second time, HTTPError occurred: 404 Client Error
.
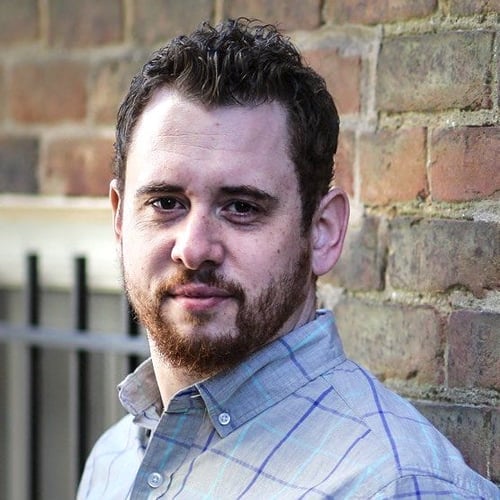
Ricky White RP Team on Feb. 8, 2020
This is used so that no exception is raised on a successful request. This will allow you to capture unsuccessful requests based on the exception type returned and deal with them accordingly.
idosegev on Feb. 12, 2020
I don’t get any response from my if-elif loop and i don’t know why. maybe it has to do with python38 ?
import requests
url = ‘api.github.com’ response = requests.get(url)
if response.status_code == 202: print(‘Success!’) elif response.status_code == 404: print(‘Not Found.’)
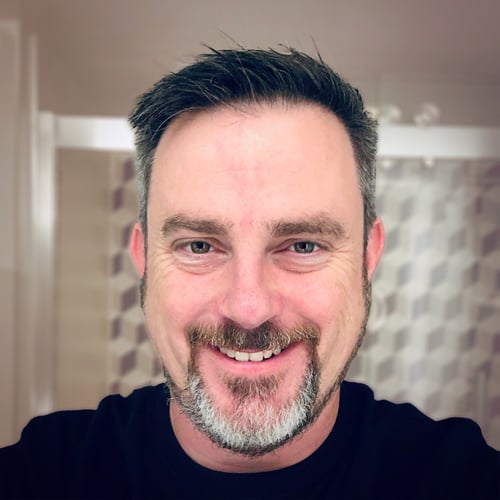
Chris Bailey RP Team on Feb. 12, 2020
Hi @idosegev,
There are a couple of differences from your code in the comment you posted and the example in the video lesson:
The url
should be url = 'http://api.github.com'
The first if
should be if response.status_code == 200:
and not 202
. 200 would mean success and 202 would mean something different, accepted. Because the code I’m demonstrating is an incomplete example, I don’t have a fall back of else:
which would catch some other result. I guess you could have it print the response, such as
else:
print(f'Status Code was {response.status_code}')
Using an f string. I hope this helps with your question.
idosegev on Feb. 13, 2020
@Chris Bailey thanks for the quick response!
B S K Karthik on April 19, 2020
@Chris Bailey, Hi Chris Can you please let me know what color theme have you used in visual studio code editor. Thank you.
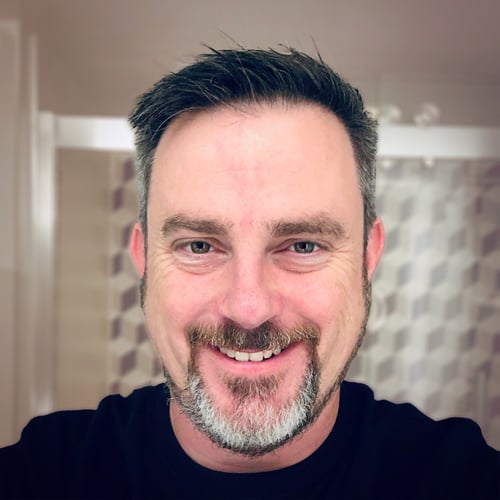
Chris Bailey RP Team on April 19, 2020
Hi @B S K Karthik, I use Dainty - Material Theme Ocean, in VSCode. Its part of the Dainty theme package. I love the contrast.
B S K Karthik on April 20, 2020
Super Thank you Chris.
mkpf on May 1, 2020
Thanks for this!
Become a Member to join the conversation.
balakumaranrk on Feb. 8, 2020
In this video what is the purpose of writing :
can you please explain ?