Apply String Methods (Solution)
00:00
I’ve already assigned the four strings here to save a bit of time, and now the task is to make them all return True
when calling .startswith()
passing in "be"
as an argument.
00:19
For string1
, we can see that it’s capitalized, so it starts with an uppercase "B"
, which is why initially calling .startswith("be")
on it returned False
.
00:26
So I will just lowercase this one saying string1
.lower()
and then call .startswith("be")
. And you can see that this returns True
. Note also that in this case I didn’t print the output,
00:41
which if you’re running this in a script, you would have to, but since I’m in a REPL, I can skip the print()
call and just instead see the output displayed here in the REPL directly. string2
.
00:52
Well, here I don’t need to do anything, so I can just say .startswith("be")
, and I’ll get True
. And I’m going to go and copy this because otherwise I’m going to keep making mistakes typing it out.
01:17
Now we’re looking at string number three, which is an all-uppercase word that is "BEAR"
in this case. So again, I will need to lowercase it so I can say string3
.lower()
and then .startswith("be")
.
01:31 And as you can see here, I’m kind of, like, chaining these method calls on it. This doesn’t always work, but it works with strings because strings are immutable.
01:41
So all of these methods always return a new string. So string3.lower()
actually returns a new string. It’s not the same string3
, but it’s 'bear'
but lowercase.
01:56
So you get 'bear'
back. And then because string3.lower()
returns this lowercase string 'bear'
, I can then call .startswith("be")
on this lowercase string.
02:08
So I’m not actually calling .startswith("be")
on the uppercase "BEAR"
but on the new string that gets created when I run string3.lower()
.
02:17 after another with a dot in between is something that’s called method chaining.
02:24
Again, we’ve got one more to go, string four. string4.
I will need to get rid of the whitespace, so I’m going to say .lstrip()
and get rid of the whitespace on the left side.
02:39
There’s none on the right side. And then I will also need to lowercase it, so .lstrip().lower()
, and then I’m going to do .startswith("be")
,
02:52
and I get True
as a return value. So this is kind of interesting to look at, right? You can see all these dots and then method calls after it, like I explained with the one before. You’re doing the same here.
03:07
Just there’s one additional step. So in this case, you’re first creating a string that has the whitespace removed on the left side, so it’s still this weirdly cased "bEautiful"
with the with the capital "E"
and the rest lowercase.
03:21
Then you create a new string with also adding .lower()
at the end of this, which then gets rid of the uppercase "E"
. Well, it converts it to a lowercase "e"
.
03:32
And then finally, you’re calling .startswith("be")
on this string, on the lowercase "beautiful"
string. And because of that, it returns True
, and that’s it.
03:43
If you did this with intermediate variables, that’s completely fine as well. Like I explained the steps here, you could have done that as well. You could have said string4_without_whitespace
and assigned it to string4.lstrip()
and then say string4_without_whitespace.lower()
is going to be s4_lower
.
04:16
And then you could say s4_lower
.startswith("be")
.
04:23
Oh no, I got a double dot in there accidentally. s4_lower.startswith("be")
, and then you get True
. So, you don’t need to do this using method chaining in one line.
04:36 You can do it with intermediate variables, and that’s totally fine, but it also works like this, but maybe it’s interesting to see. Exercise solved.
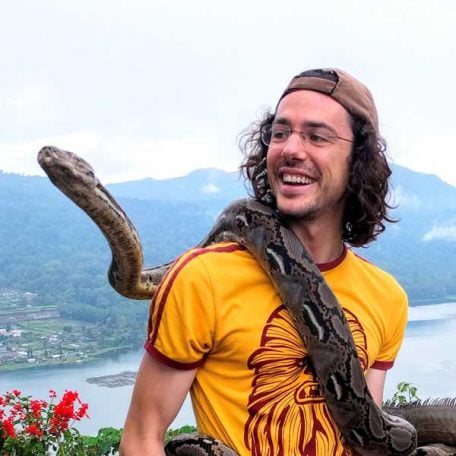
Martin Breuss RP Team on Dec. 20, 2023
DimaG nice work! I like your use of .casefold()
here, which makes it even more explicit that you’re doing the case-transformations for comparison’s sake. And more stable, even though for English words (AFAIK) .lower()
suffices.
For learners who are wondering about DimaG’s use of a list and a for
loop, you’ll cover these topics further down the line of the Python Basics Learning Path :)
Become a Member to join the conversation.
DimaG on Dec. 19, 2023
Here is my version using a list and a
for
loop: