What Are These Different Method Types Good for in Python?
Now that you’ve learned about the basic concepts behind instance, class and static methods, the current lesson let’s you run the code you have written to see the actual results of calling the different method types. Furthermore, limits of certain types are revealed.
00:00 So, the first thing I want to show you here is that when I create an object instance based on this class, I can actually call any of these method types on that object.
00:15
So, I can call a plain method—and I kind of structured these methods in a way that they return a string that kind of explains what’s going on. All right, so when I call obj.method()
, we can see here that, well, we called the instance method and we had this self
object pointing to an instance of MyClass
.
00:36
Now, I can do the same thing with a class method, and now when I call this, you can see here that, again, we’re calling this .classmethod()
method, and this time we also have access to this class object.
00:52
Now, the difference is that with the instance method, we actually had access to the object instance, right? So, this was an instance of a MyClass
object.
01:03
Whereas with the class method, we have access to the class itself, but not to any of the objects because the class method doesn’t really care about an object existing. However, you can both call .classmethod()
and .staticmethod()
on an object instance.
01:23
This is going to work—it doesn’t really make a difference. So again, when you call .staticmethod()
here, it’s going to work and it’s going to know which method you want to call, but really, the key difference now is going to be that we can also say, okay, MyClass
—and I’m not creating an instance of this class—and I can go in and say .classmethod()
, for example. This is going to work fine.
01:46
And I can also go ahead and call the .staticmethod()
. This is also going to work fine. But of course, when I try and call .method()
, that’s going to fail because we didn’t actually call it with a class instance.
02:01 So, I hope this makes this distinction between regular methods and static and class methods a little bit more clear. Now, of course, the big question is, “Okay, why…why do we need that? Why is that a thing?” And I want to go over some examples here of what you can use these methods for, because I think they’re actually a really powerful concept, or a really powerful tool, for you to structure your code in a way that makes the most sense.
Zarata on April 20, 2020
OH!!! The DECORATORS are more than just pretty things. Aha! Too bad there’s no comment erase! :)
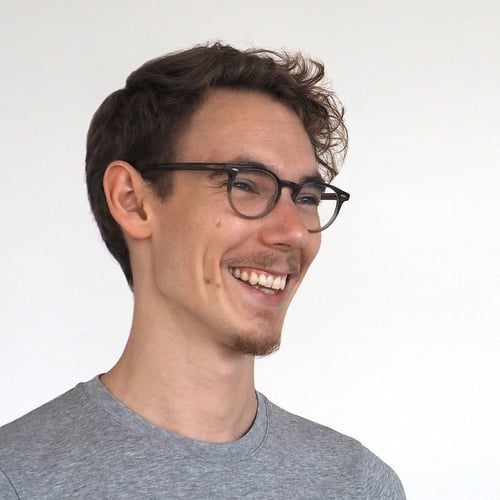
Dan Bader RP Team on April 21, 2020
Are
self
andcls
true “magic” variables, even moreso than the dunders?
You’re on the right track! The position of the self
or cls
parameter is what’s important, not the name.
Naming the parameter self
or cls
is just a convention (and one we should stick to for stylistic reasons.)
So you could call the parameter ego
, clazz
, foobar
instead of self
and your code would still work, as long as that parameter is in position #1 in the function definition.
More info here: stackoverflow.com/questions/7554738/python-self-no-self-and-cls
debjan on Feb. 16, 2021
I think this should have be slightly better then it is as great, in regular Python interpreter instead BPython (just nitpicking, sorry):
>>> obj = MyClass()
>>> obj.method()
('instance method called', <__main__.MyClass object at 0x7fd5b495b280>)
>>> obj.classmethod()
('class method called', <class '__main__.MyClass'>)
>>> obj.staticmethod()
'static method called'
even in IPython we don’t have console dunder:
In [1]: obj = MyClass()
In [2]: obj.method()
Out[2]: ('instance method called', <__main__.MyClass at 0x7f9f964cd220>)
In [3]: obj.classmethod()
Out[3]: ('class method called', __main__.MyClass)
In [4]: obj.staticmethod()
Out[4]: 'static method called'
Become a Member to join the conversation.
Zarata on April 20, 2020
At this point I’m wondering about how Python views the terms “self” and “cls” when used as method arguments. These aren’t keywords, and yet they are automatically being interpreted with different understandings: one to symbolize “this” instance, one to symbolize the class. Before I saw this example, I thought “self” could be any group of characters … “Selbst”, “selbt_gleich”, or “soi” or “autobus” for that matter. Are “self” and “cls” true “magic” variables, even moreso than the dunders?