What Is Object-Oriented Programming (OOP)?
In this video, you’ll learn what object-oriented programming (OOP) is and why it’s used.
Lots of popular frameworks and libraries are written using OOP, so it’s a good tool to have in your Python toolkit.
OOP is a programming paradigm, or a specific way of designing a program. It allows us to think of the data in our program in terms of real-world objects, with both properties and behaviors. These objects can be passed around throughout our program.
Properties define the state of the object. This is the data that the object stores. This data can be a built-in type like int
, or even our own custom types we’ll create later.
Behaviors are the actions our object can take. Oftentimes, this involves using or modifying the properties of our object.
00:00 Hi. I’m Austin Cepalia with realpython.com, and I welcome you to our series on object-oriented programming in Python 3. This is one of the biggest and most important subjects in all of programming and as you can see, we have a lot to cover.
00:16 This series will provide you with a basic conceptual understanding of object-oriented programming so that you can take your Python programming skills to the next level. To fully appreciate the subject, we have to first understand why we need it. Programs are very complex things and there’s a hundred different ways that you could define what a program is formally, but basically, all programs are a set of instructions for manipulating data in some meaningful way. When I teach Python to kids, I always have them write a program that calculates how many days old they are, and that’s a great example of a program that follows these three steps. First, the program has to ask the user for their birth date.
00:59 That’s the data it’s going to accept. Then, it manipulates the data by subtracting the current date from the user’s birth date. Finally, the program displays the result of this calculation on the screen.
01:12 That’s a great starter program, but it’s pretty trivial. How do we manage large programs with lots of data to keep track of, like a video game? As you might have guessed, that’s where object-oriented programming comes in.
01:26 Object-oriented programming, or OOP for short, is a very common programming paradigm. Informally, this means that it’s a specific way of designing programs, like a style that’s widely used in software projects.
01:41 Not every program should be written with OOP but many are, and lots of languages support it. A lot of the Python code you interact with on a daily basis will be written using OOP. Understanding this is a fundamental part of becoming a great Python programmer.
01:57 It opens the door to creating really cool software. At the heart of OOP is the idea that a program is composed of lots of individual objects. You can think of these objects as entities, or things, in your program.
02:12 Oftentimes, they are the nouns in your project, such as a person, a house, an email, or any other entity like that. These objects are like little bundles of data that are passed around throughout the life of your program. For example, a person might have properties like name, age, and home address.
02:33
This is the individual data that the object stores throughout its life in the program. When we create this Person
object, we’ll make sure to give it a name, an age, and an address. Objects also have behaviors. These are the actions that the object can take.
02:50
Our person should be able to walk, talk, and breathe, for example. Oftentimes, these behaviors will need to reference the properties of the object. For example, if we make our talk behavior print the name and the age of the person, then our Person
object would need to know what its name and age is—those properties we gave it earlier before.
03:12 If this all seems a little bit confusing right now, don’t worry. When I started programming, this was the first real hurdle I ran into, and it just took some dedication and practice until I finally understood it.
03:22 We’ll make heavy use of objects throughout the rest of this series and you’ll love the feeling you get when it just clicks for you.
03:30 In the next video, we’ll learn how to define our own objects using Python classes. I’ll see you there.
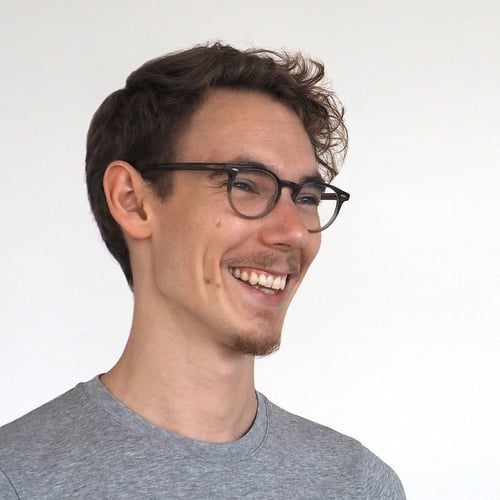
Dan Bader RP Team on March 22, 2019
Thanks for your feedback Peter, that’s great to hear. Stay tuned for more Python OOP tutorials and video courses in the future :)
Austin Cepalia RP Team on March 22, 2019
Thanks for the kind words Peter, I’m glad to hear the course was helpful for you.
arnautovdmitry2015 on April 1, 2019
It’s very helpful course. Absolutely nice. Thanks a lot!
Sillikone on Aug. 4, 2021
Hello ! Thanks for the course ! I was exercising and wanted to create an instance attribute of type ‘Wear’ for my dogs. For it to work I had to explicitly name the class in the init of Dog():
def __init__(self, name, age, cloth=Wear):
I’m affraid that I won’t be able to understand… but why this doesn’t work like the other types?
def __init__(self, name, age, cloth):
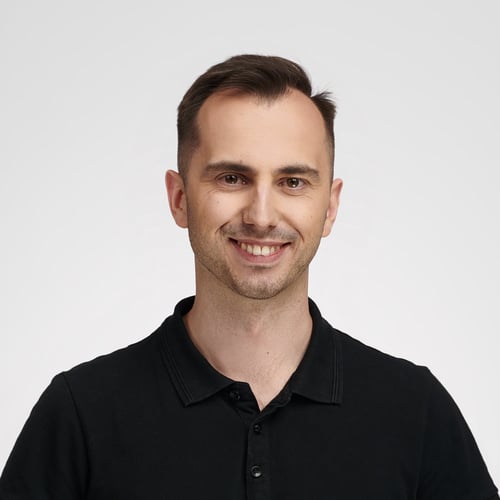
Bartosz Zaczyński RP Team on Aug. 5, 2021
@Sillikone Hi, there. Can you clarify your question, please? I’m afraid folks over here may not be able to understand it correctly.
If you want to create an instance variable of a specific type, then you should state that explicitly in your .__init__()
method:
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
self.cloth = Wear() # <--- Here
However, your function accepts the cloth
as a parameter, so perhaps this is what you meant instead?
class Dog:
def __init__(self, name, age, cloth):
self.name = name
self.age = age
self.cloth = cloth
Sillikone on Aug. 5, 2021
@Bartosz Zaczyński Hi ! Sorry for the trouble, yersterday this wasn’t working:
class Wear:
def __init__(self, color):
self.color = color
class Dog:
def __init__(self, name, cloth):
self.name = name
self.cloth = cloth
shirt = Wear('red')
dog = Dog('Luigi', shirt)
print(f'{dog.name} is wearing a {dog.cloth.color} cloth')
I had to specify this for it to work:
class Dog:
def __init__(self, name, cloth=Wear):
self.name = name
self.cloth = cloth
Rewritting all from scratch to clarify, I could not reproduce the behavior.
Become a Member to join the conversation.
Peter T on March 22, 2019
Austin did an excellent job of clearly explaining the various OOP topics in all 7 videos, without leaving any gaps or questions. Now, I’m anxiously waiting for the next set of OOP videos to continue to this course & I hope they show up soon. IMHO, there aren’t enough OOP topics in realpython.com or dbader.org, but there are tons of other Python-related topics in both sites!