Built-in Namespace
00:00 In this lesson, you will have a closer look at the built-in namespace. The built-in namespace is always available when Python is running.
00:11
You can see all the Python goodness that is available to you, right from the builtins by typing dir(__builtins__)
in the Python REPL. You may see types here that you recognize, like 'bool'
, 'int'
, or 'str'
(string).
00:29
And you may see functions that you recognize like 'print'
, 'len'
, or 'list'
. But where is the value of __builtins__
defined? To figure that out, you can run the following command: __builtins__.str.__module__
.
00:50
That means that the value of the __builtins__
name is actually the builtins
module. This is indeed the module where all builtins are located, as you can see when you import the builtins
module and check whether __builtins__
equals builtins
, which shows you that it is!
01:13
You will probably never need to import the builtins
module yourself but it is still good to know where all those types and functions you can use without ever importing them come from.
01:27 In the next lesson, you will learn about the global namespace.
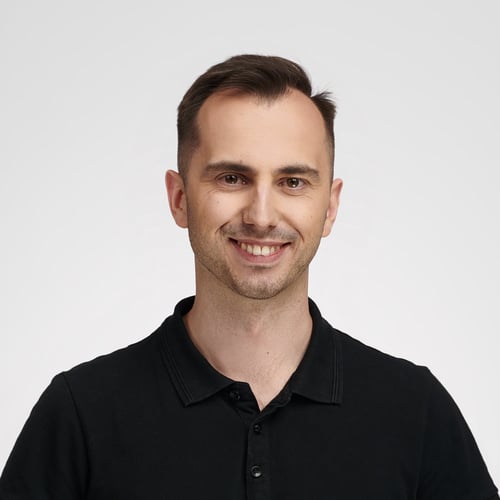
Bartosz Zaczyński RP Team on April 13, 2022
@Cindy The word “dunder” is a common abbreviation for “double underscore” or __
, which has a few special meanings in Python. In most cases, it’s used as a prefix and a suffix in special method names, such as .__str__()
or .__len__()
, to make them visually distinguishable from your own methods. When used as a prefix on your custom methods, the double underscore enables the name mangling mechanism, which has a purpose in multiple-inheritance scenarios.
__builtins__
is a special module that contains all Python built-in functions, types, etc. that you don’t typically need to import:
>>> type(__builtins__)
<class 'module'>
>>> __builtins__
<module 'builtins' (built-in)>
>>> __builtins__.len("Hello")
5
>>> len("Hello")
5
For example, you can call the global len()
function directly or more explicitly by prefixing its name with the __builtins__
module it belongs to.
__module__
is an attribute containing the name of the module:
>>> len.__module__
'builtins'
>>> __builtins__.len.__module__
'builtins'
Become a Member to join the conversation.
Cindy on April 12, 2022
Hi Johan, thank you for the lecture. I am wondering what is the function of
__
(dundas) in Python? And could you explain about the syntax:__builtins__.str.__module__
?