Python Data Structures
Learning Path ⋅ Skills: Python, Strings, Lists, Tuples, Dictionaries, Sets, List Comprehensions, range()
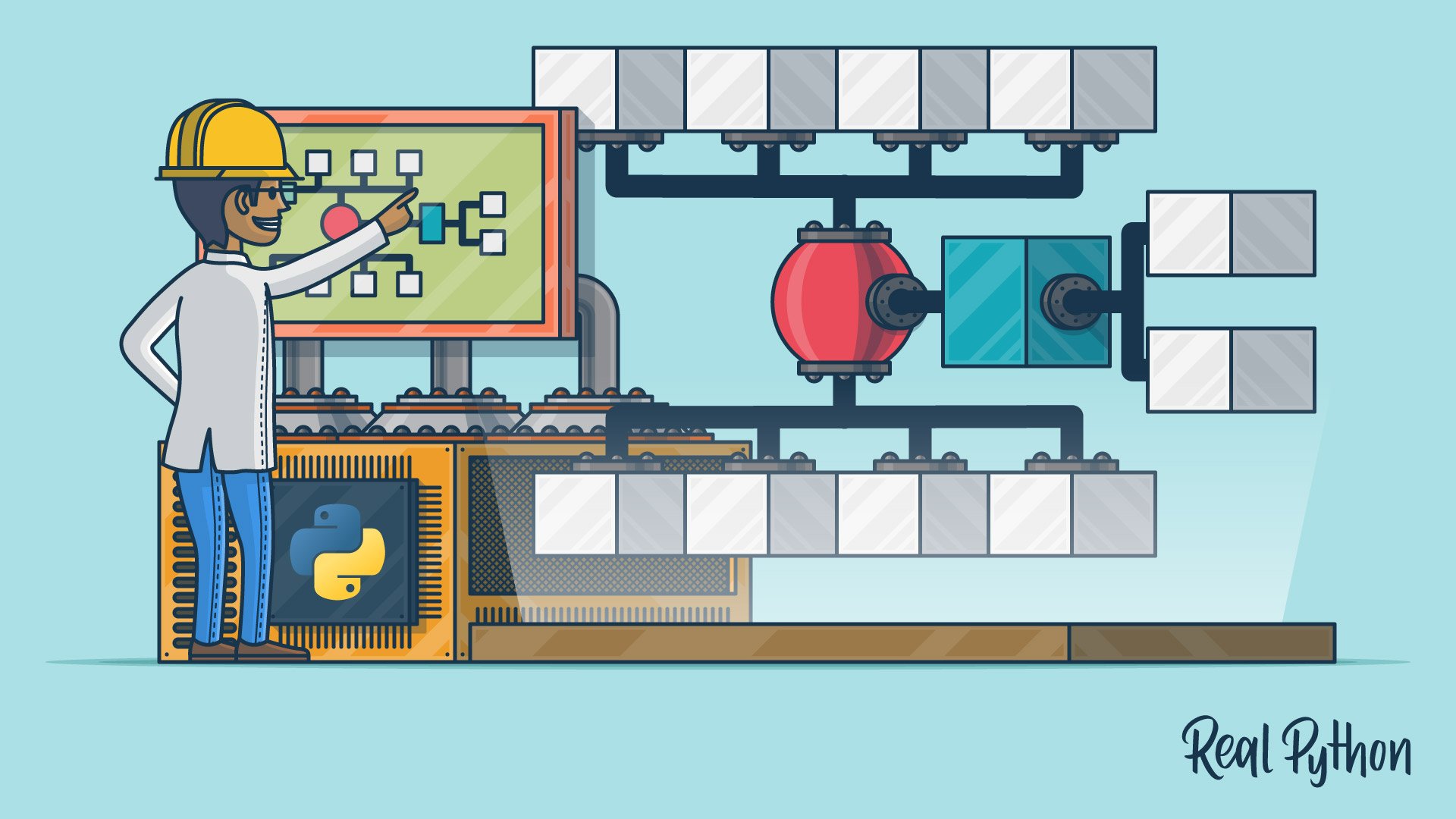
Explore Python’s core data structures: strings, lists, tuples, dictionaries, and sets. Master string operations, list comprehensions, copying objects, sorting, and the range() function. Dive deep into complex numbers, the del statement, and robust assignments.
Python Data Structures
Learning Path ⋅ 23 Resources
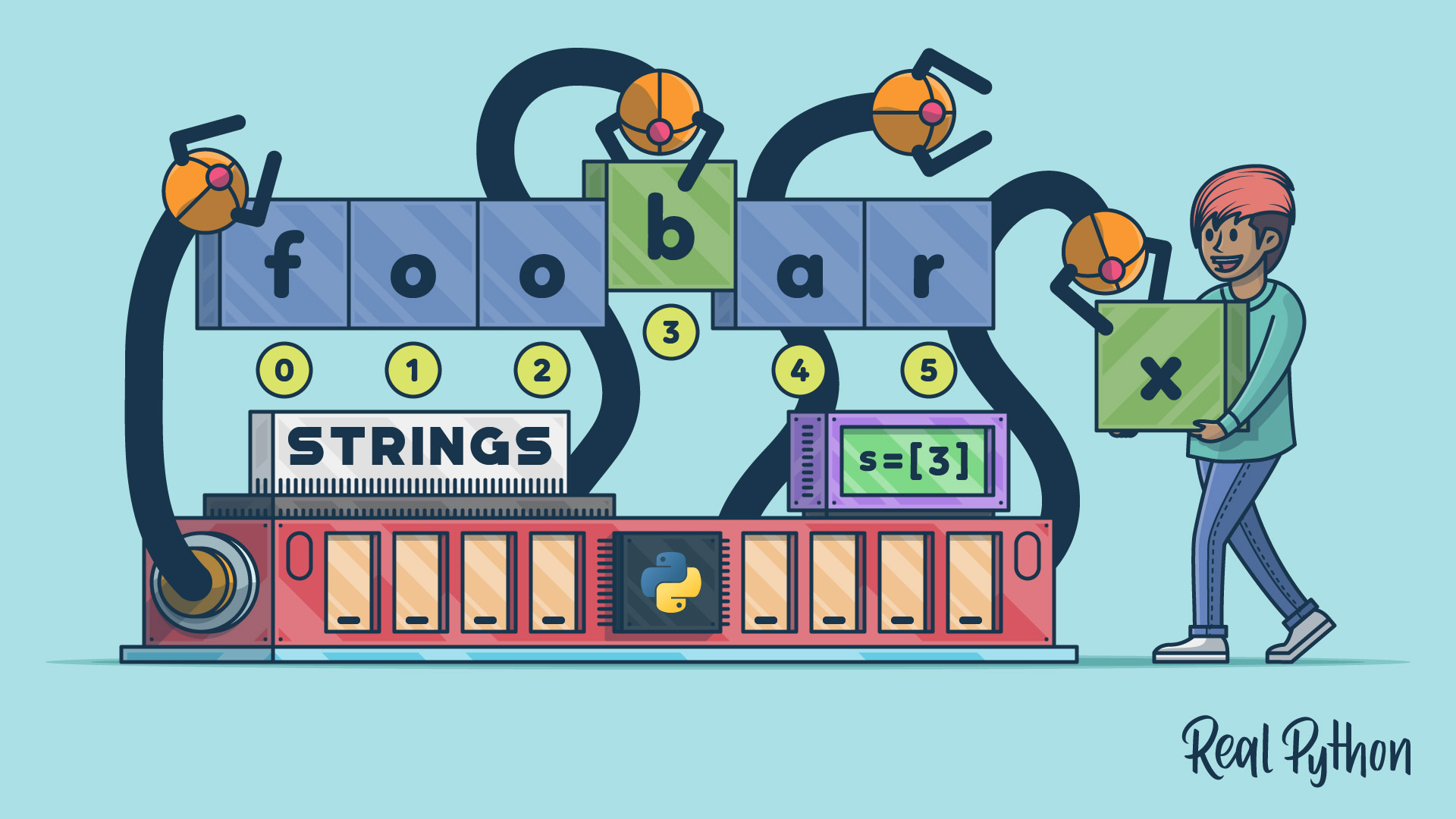
Course
Strings and Character Data in Python
Learn how to use Python's rich set of operators, functions, and methods for working with strings. You'll learn how to access and extract portions of strings, and also become familiar with the methods that are available to manipulate and modify string data in Python 3.
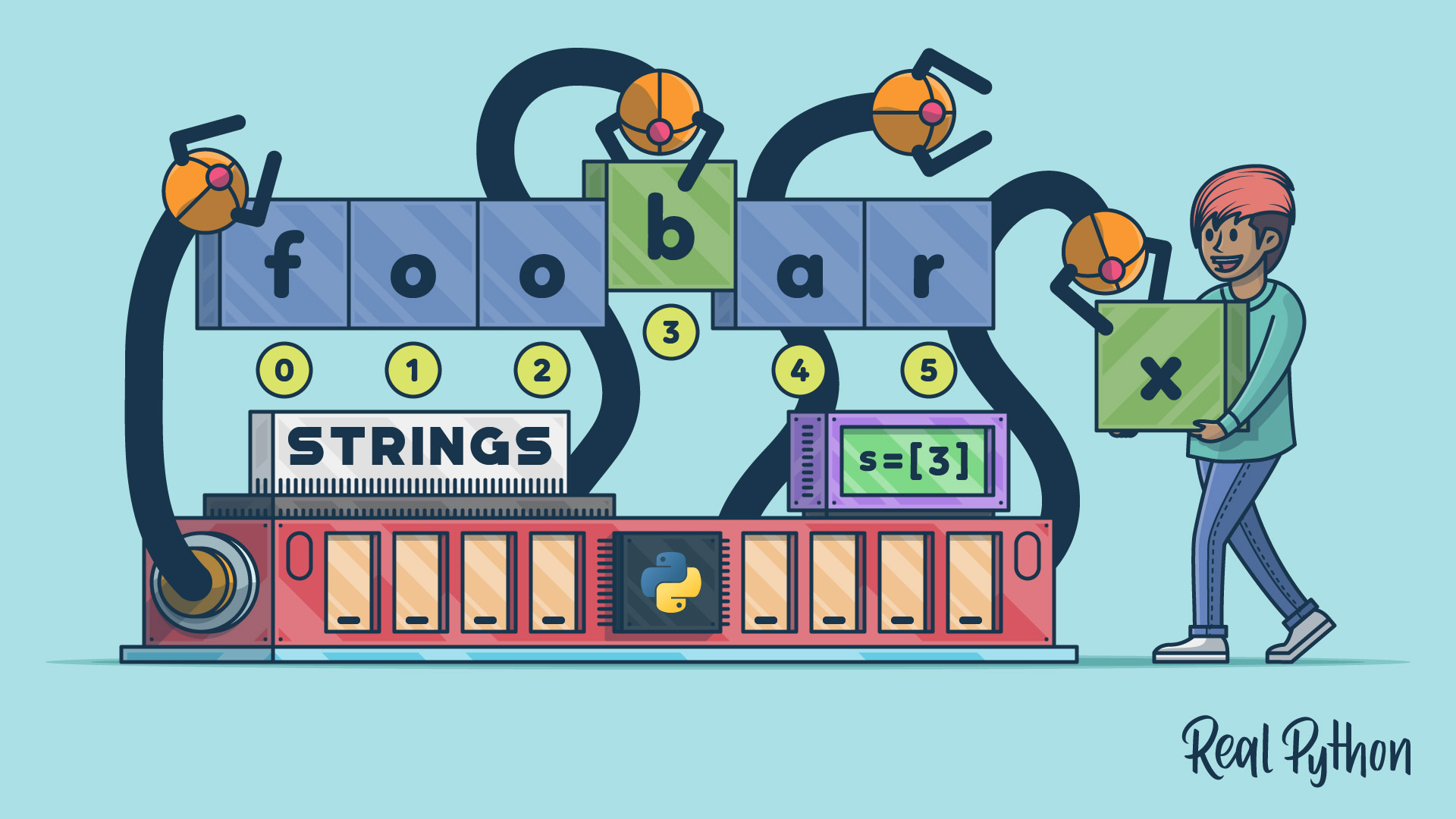
Interactive Quiz
Python Strings and Character Data
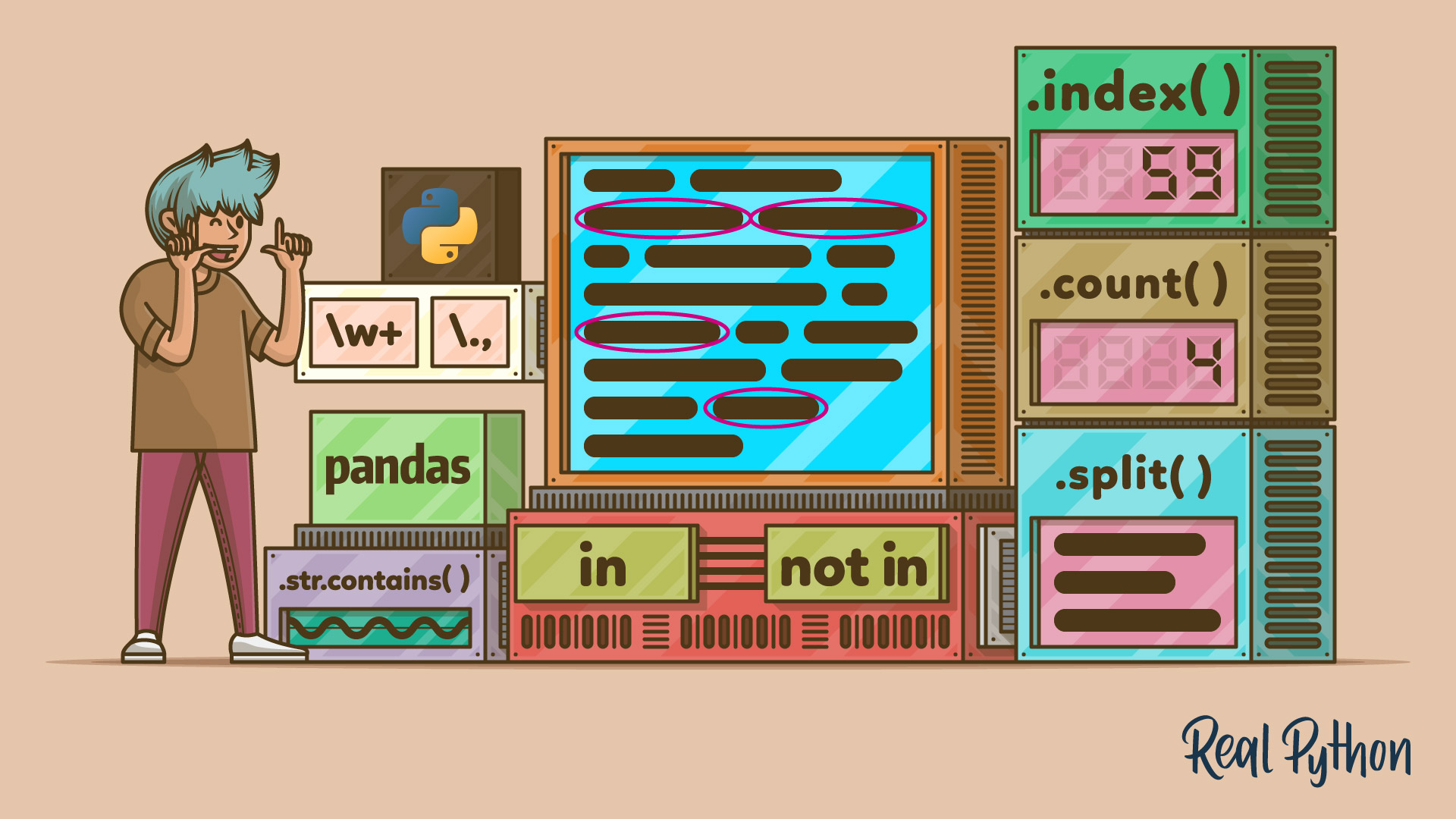
Course
Check if a Python String Contains a Substring
Learn the best way to check whether a Python string contains a substring. You'll also learn about idiomatic ways to inspect the substring further, match substrings with conditions using regular expressions, and search for substrings in pandas.
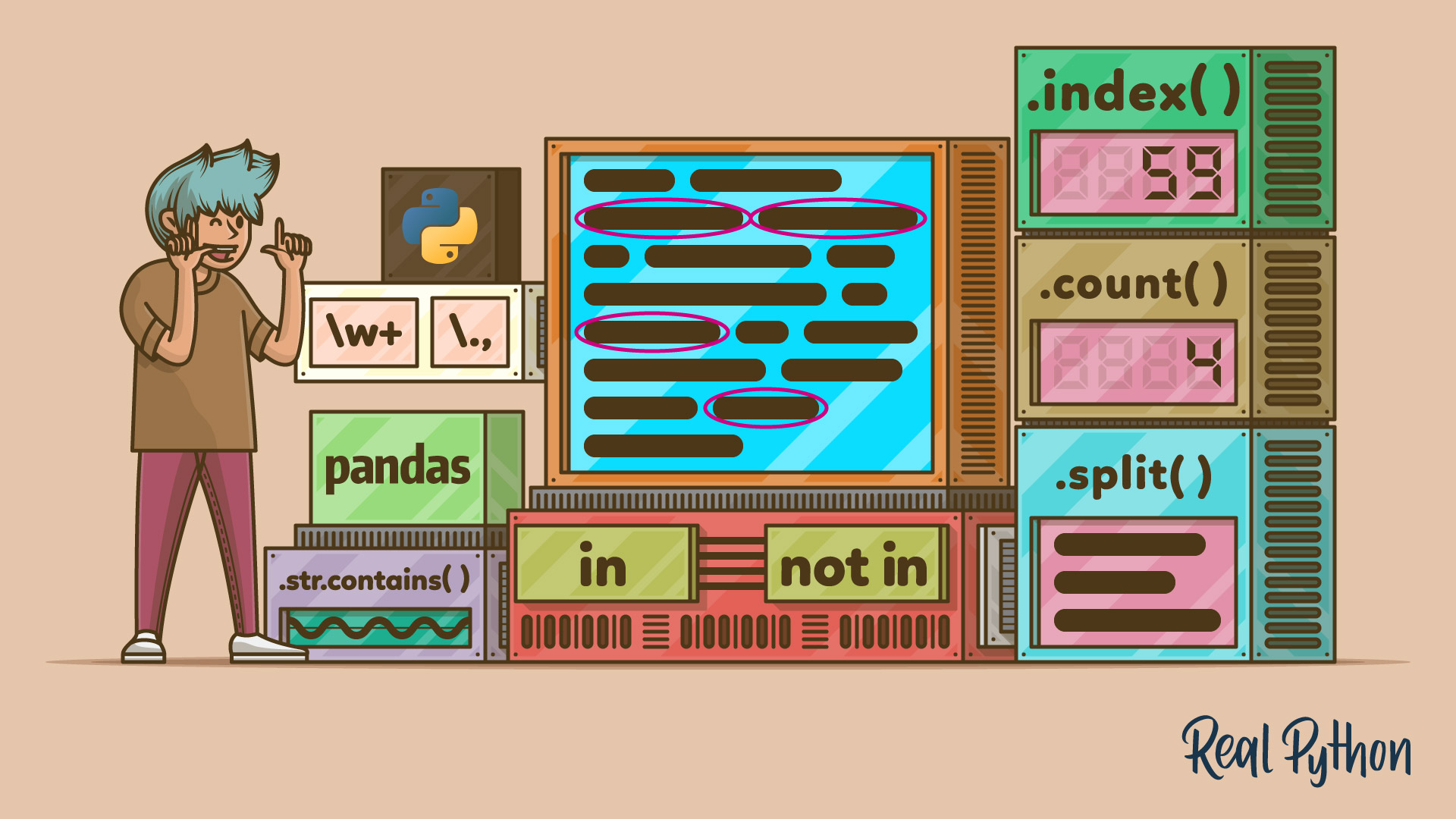
Interactive Quiz
How to Check if a Python String Contains a Substring
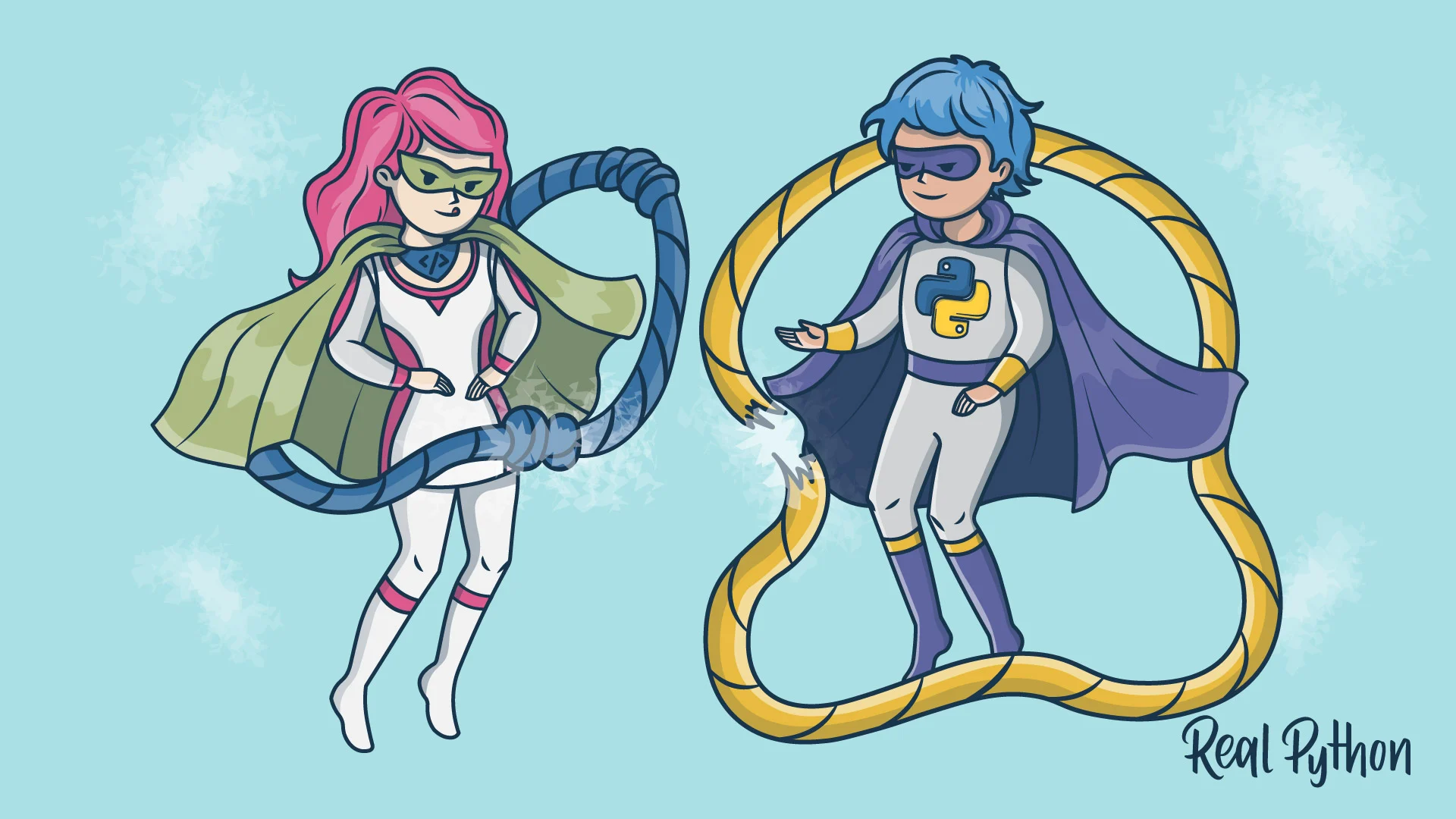
Course
Splitting, Concatenating, and Joining Python Strings
In this course you'll some of the most fundamental string operations: splitting, concatenating, and joining. Not only will you learn how to use these tools, but you’ll walk away with a deeper understanding of how they work under the hood in Python.
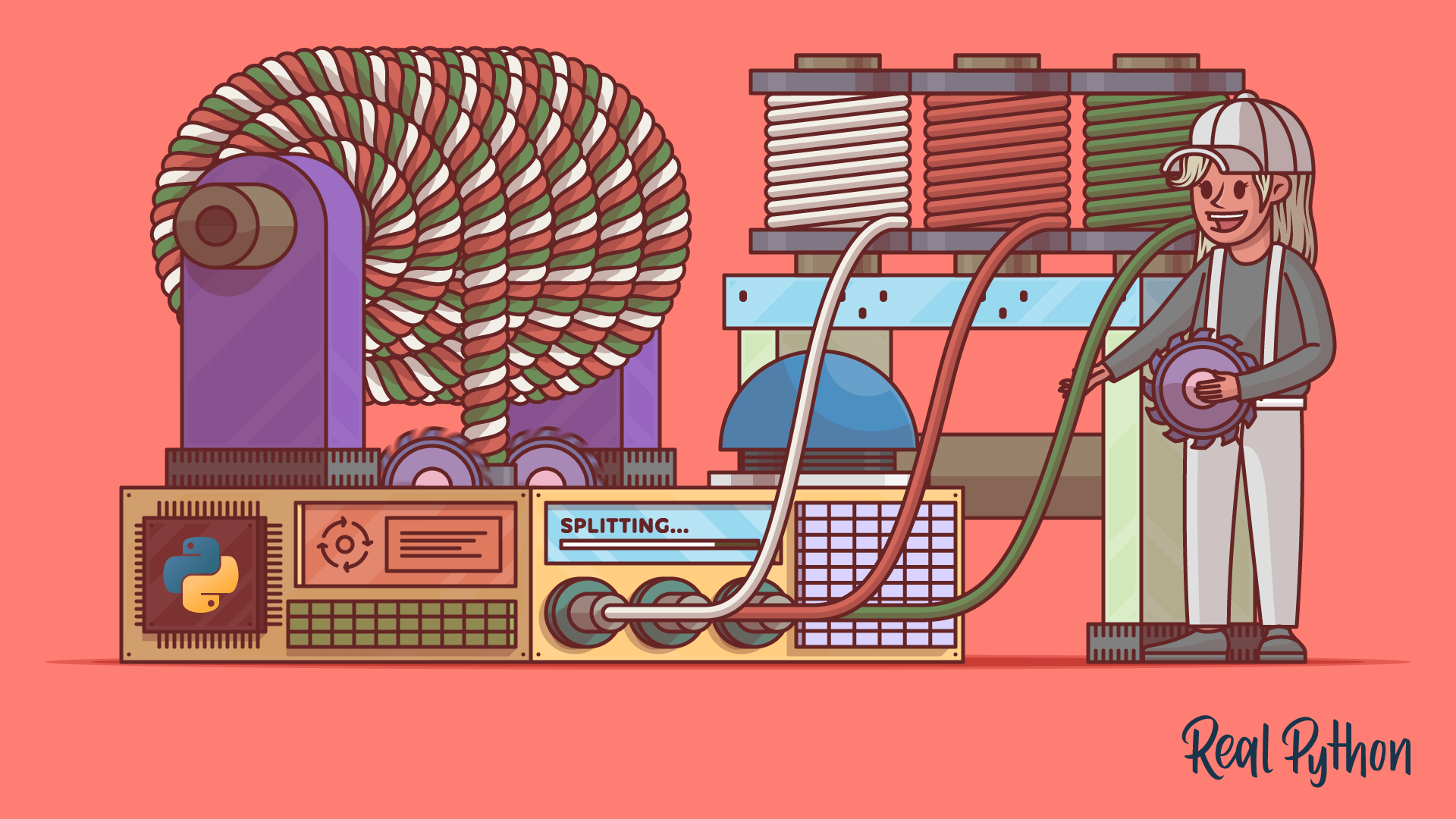
Tutorial
How to Split a String in Python
This tutorial will help you master Python string splitting. You'll learn to use .split(), .splitlines(), and re.split() to effectively handle whitespace, custom delimiters, and multiline text, which will level up your data parsing skills.
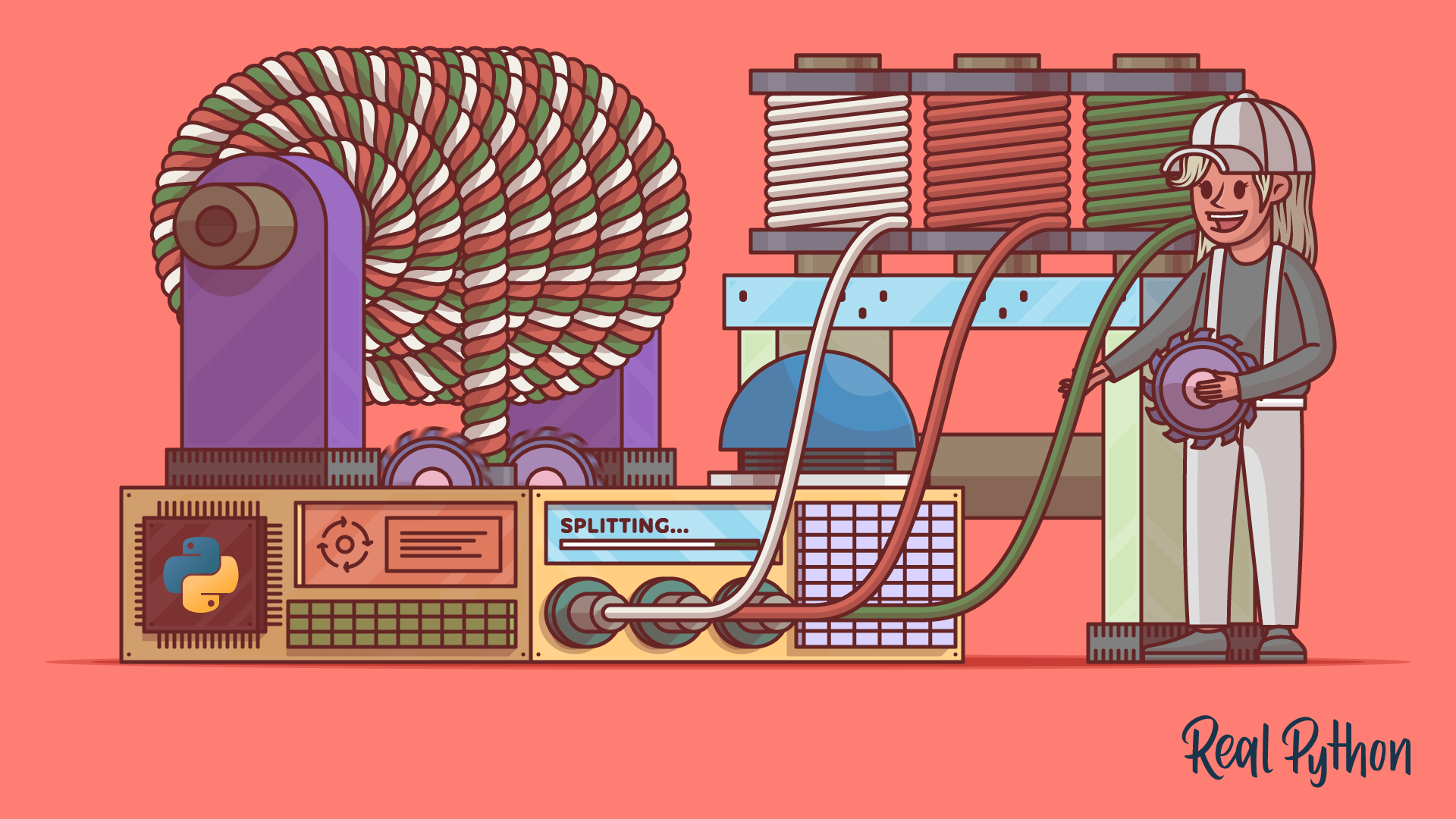
Interactive Quiz
How to Split a String in Python
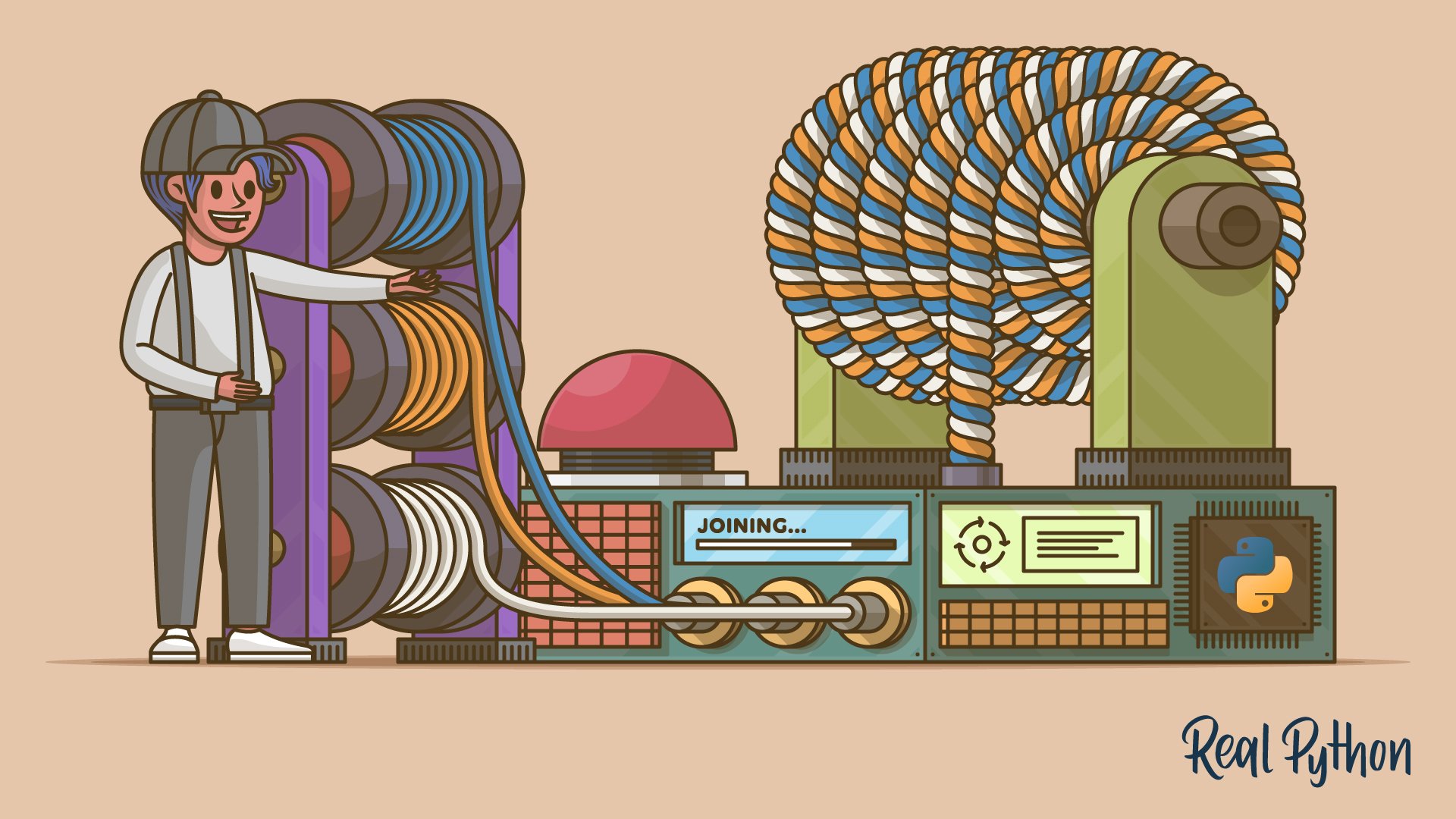
Tutorial
How to Join Strings in Python
Learn how to use Python's built-in .join() method to combine string elements from an iterable into a single string with a specified separator. You'll also learn about common pitfalls, and how CPython makes .join() work efficiently.
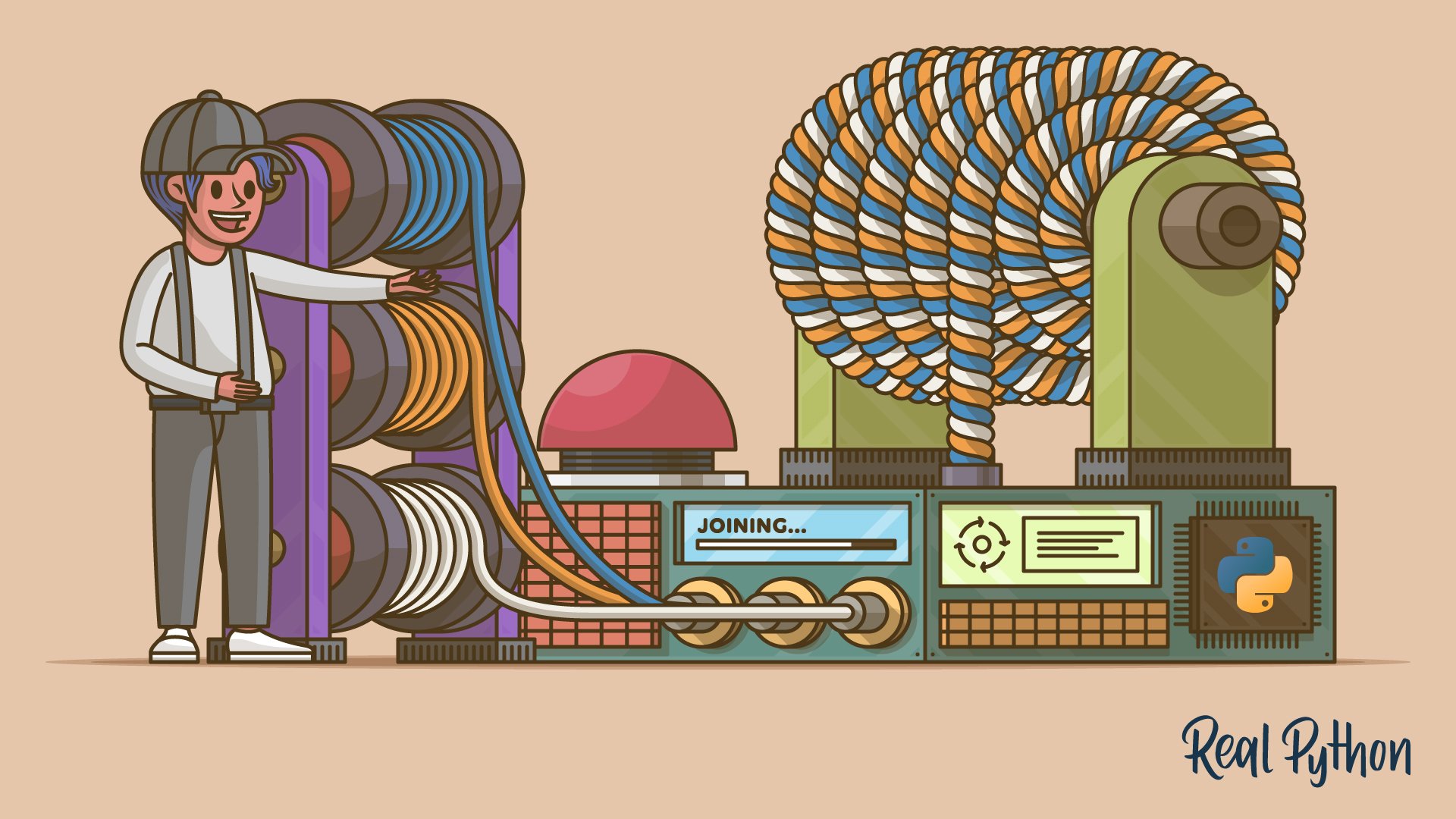
Interactive Quiz
How to Join Strings in Python
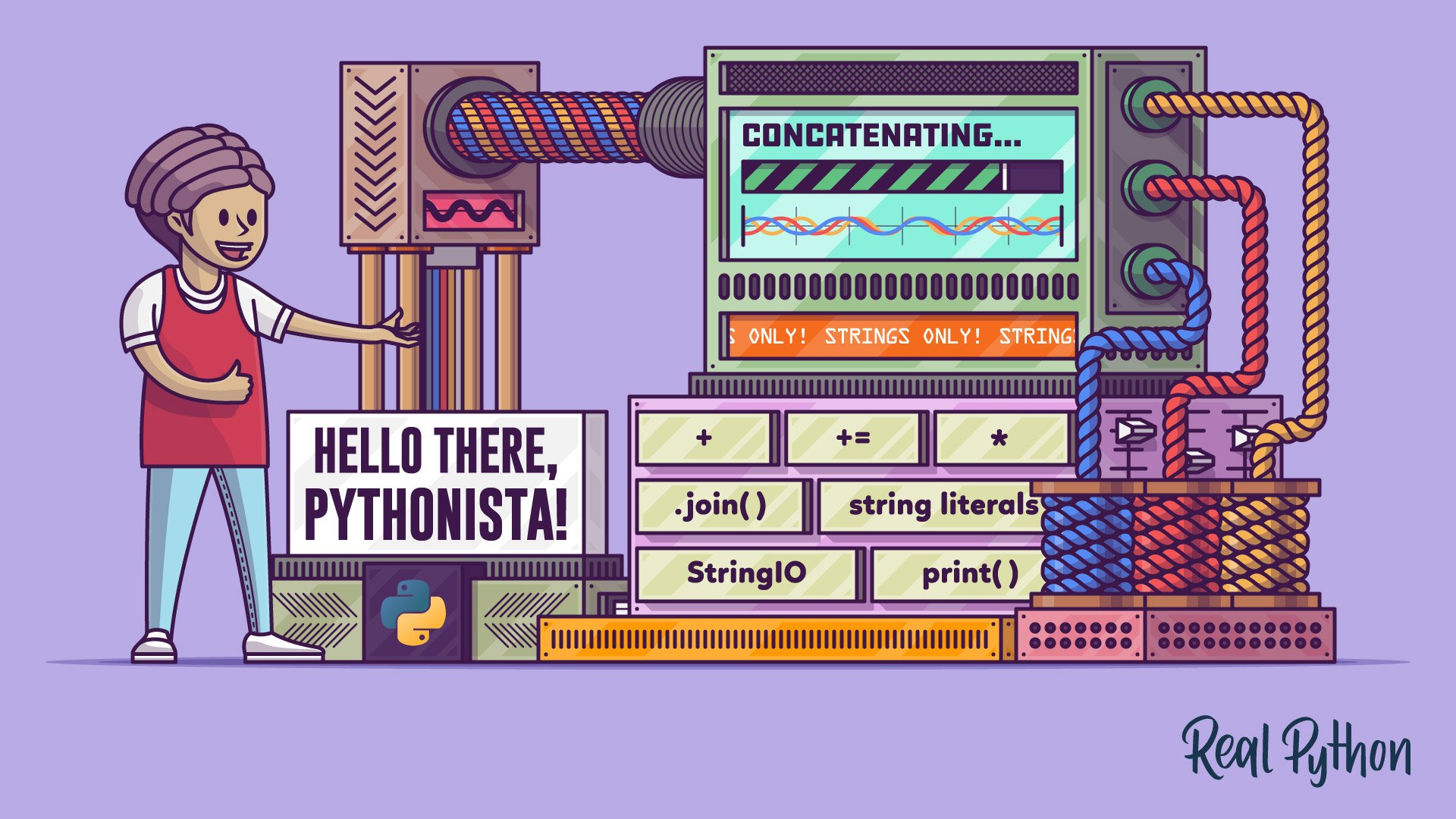
Course
Concatenating Strings in Python Efficiently
Learn how to concatenate strings in Python. You'll use different tools and techniques for string concatenation, including the concatenation operators and the .join() method. You'll also explore other tools that can also be handy for string concatenation in Python.
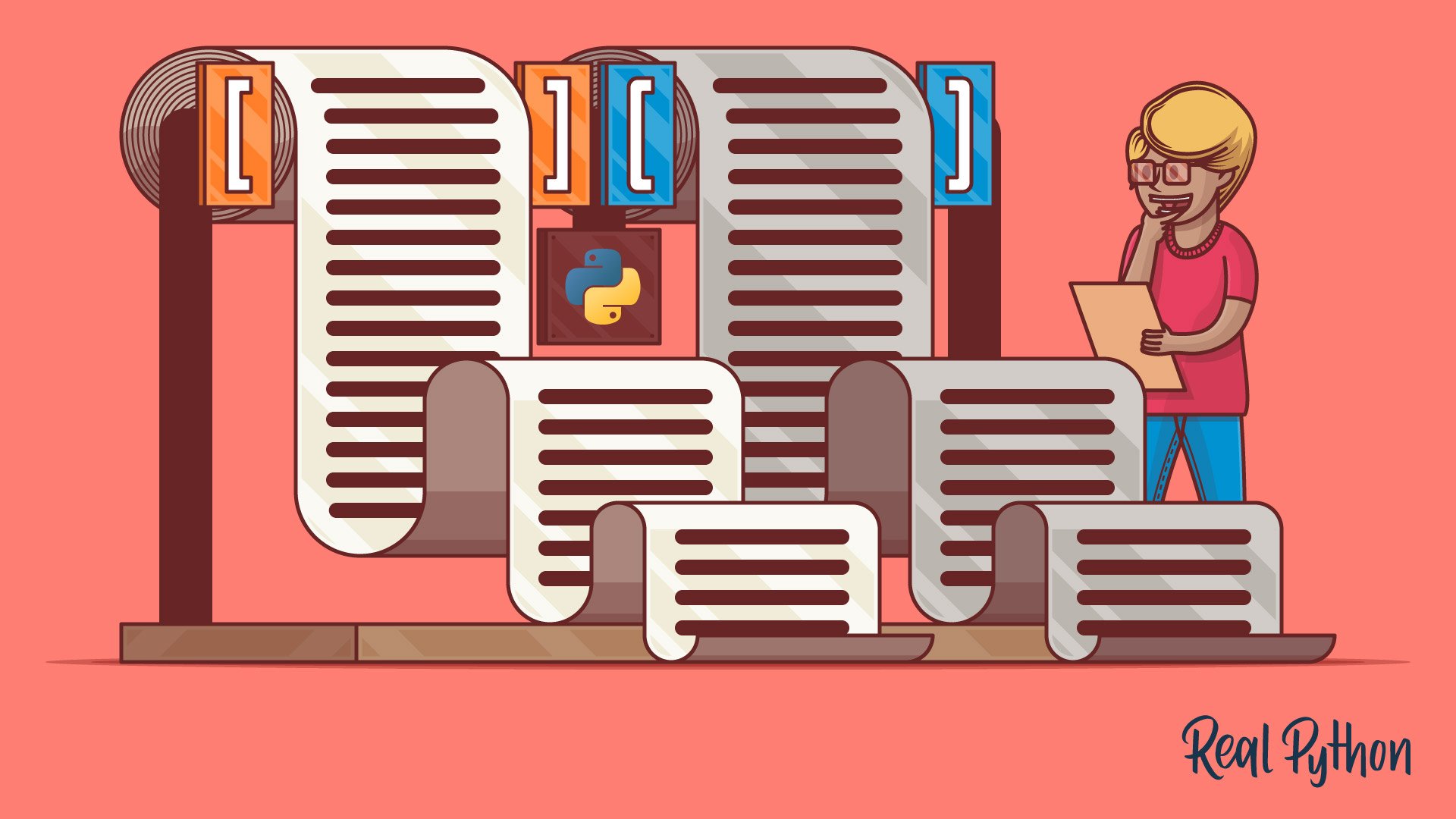
Course
Lists and Tuples in Python
See the important characteristics of lists and tuples in Python 3. You'll learn how to define them and how to manipulate them. When you're finished, you'll have a good feel for when and how to use these object types in a Python program.
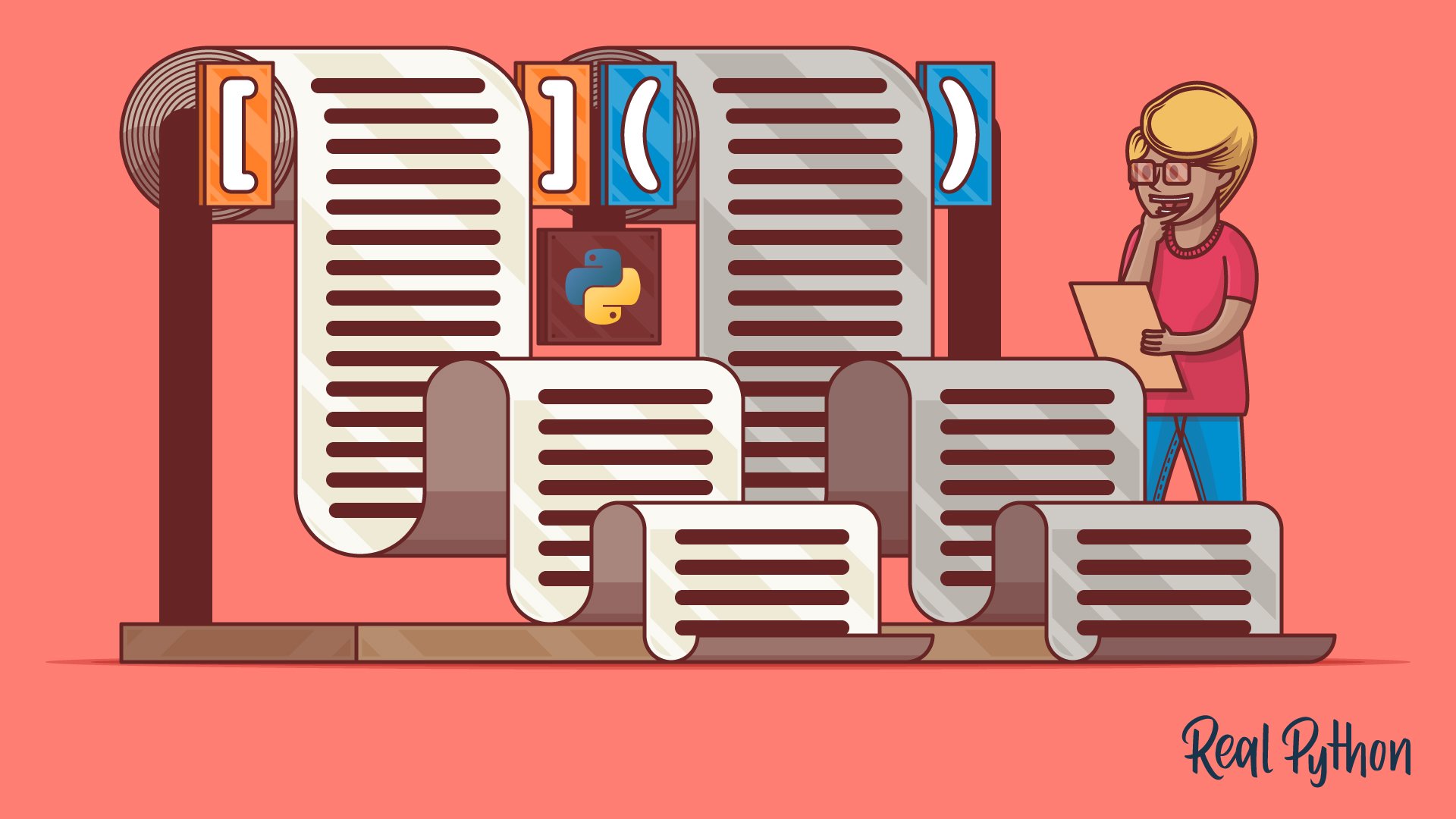
Interactive Quiz
Lists vs Tuples in Python
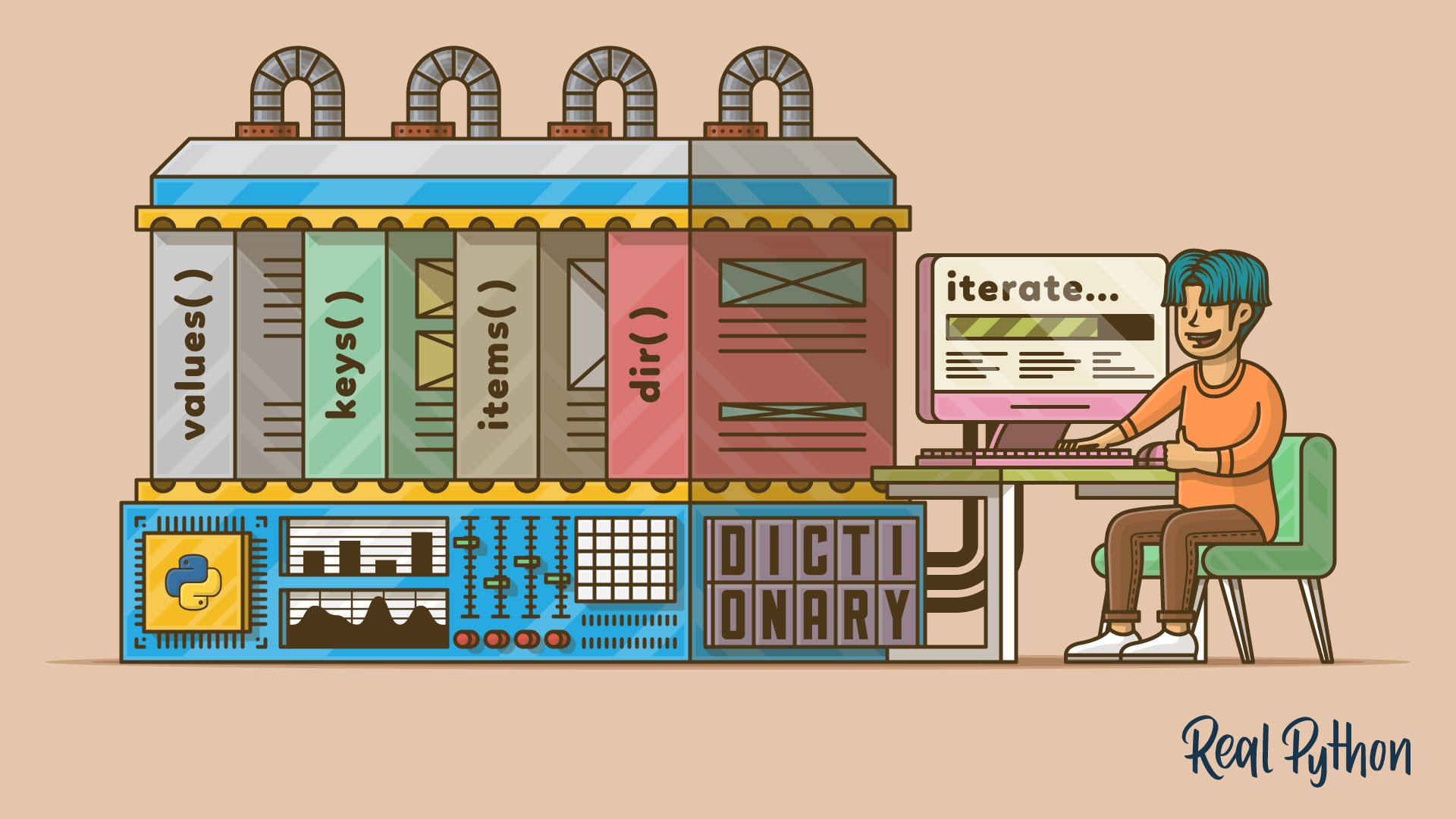
Course
Using Dictionaries in Python
In this course on Python dictionaries, you'll cover the basic characteristics of dictionaries and learn how to access and manage dictionary data. Once you've finished this course, you'll have a good sense of when a dictionary is the appropriate data type to use and know how to use it.
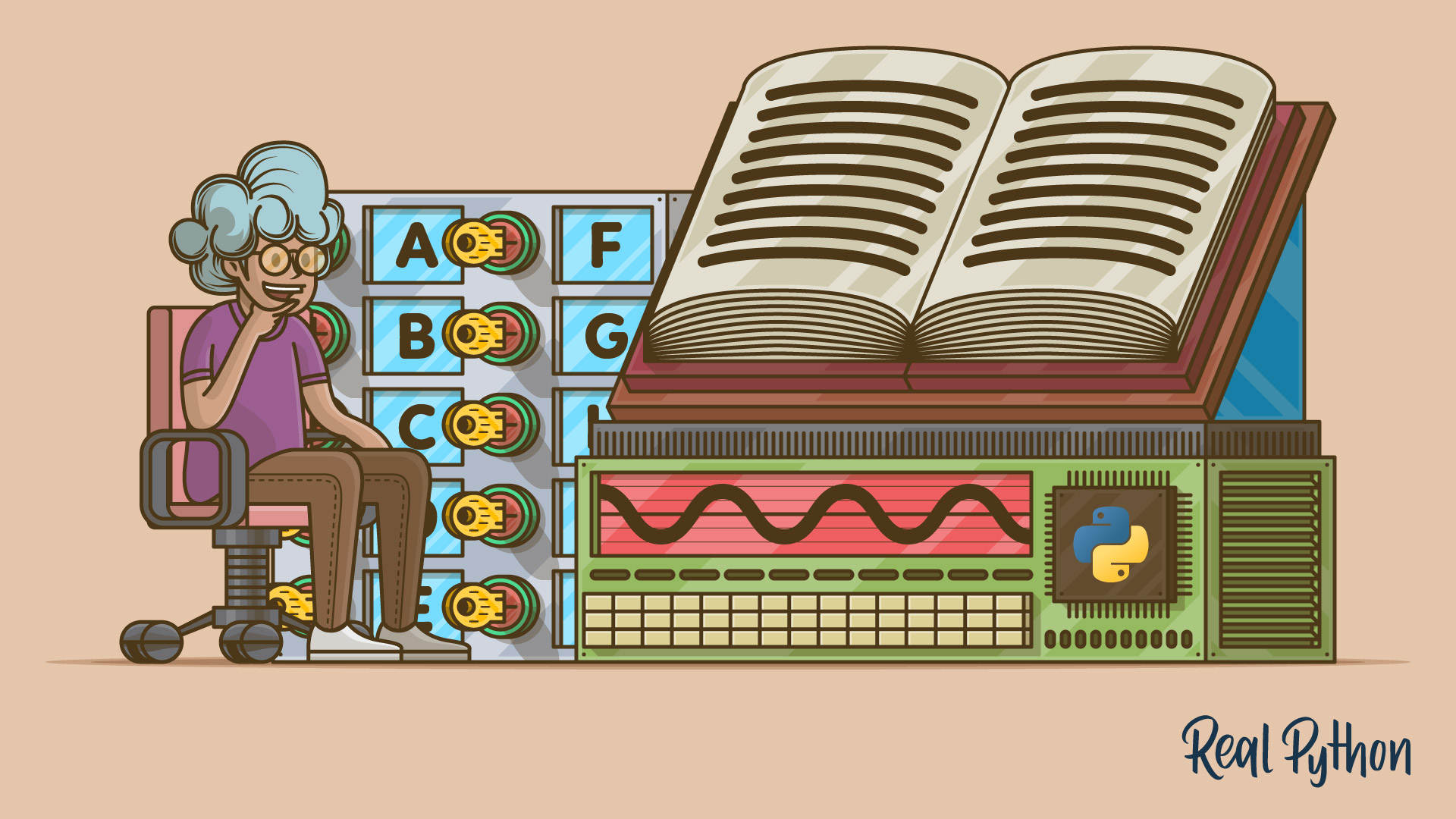
Interactive Quiz
Using Dictionaries in Python
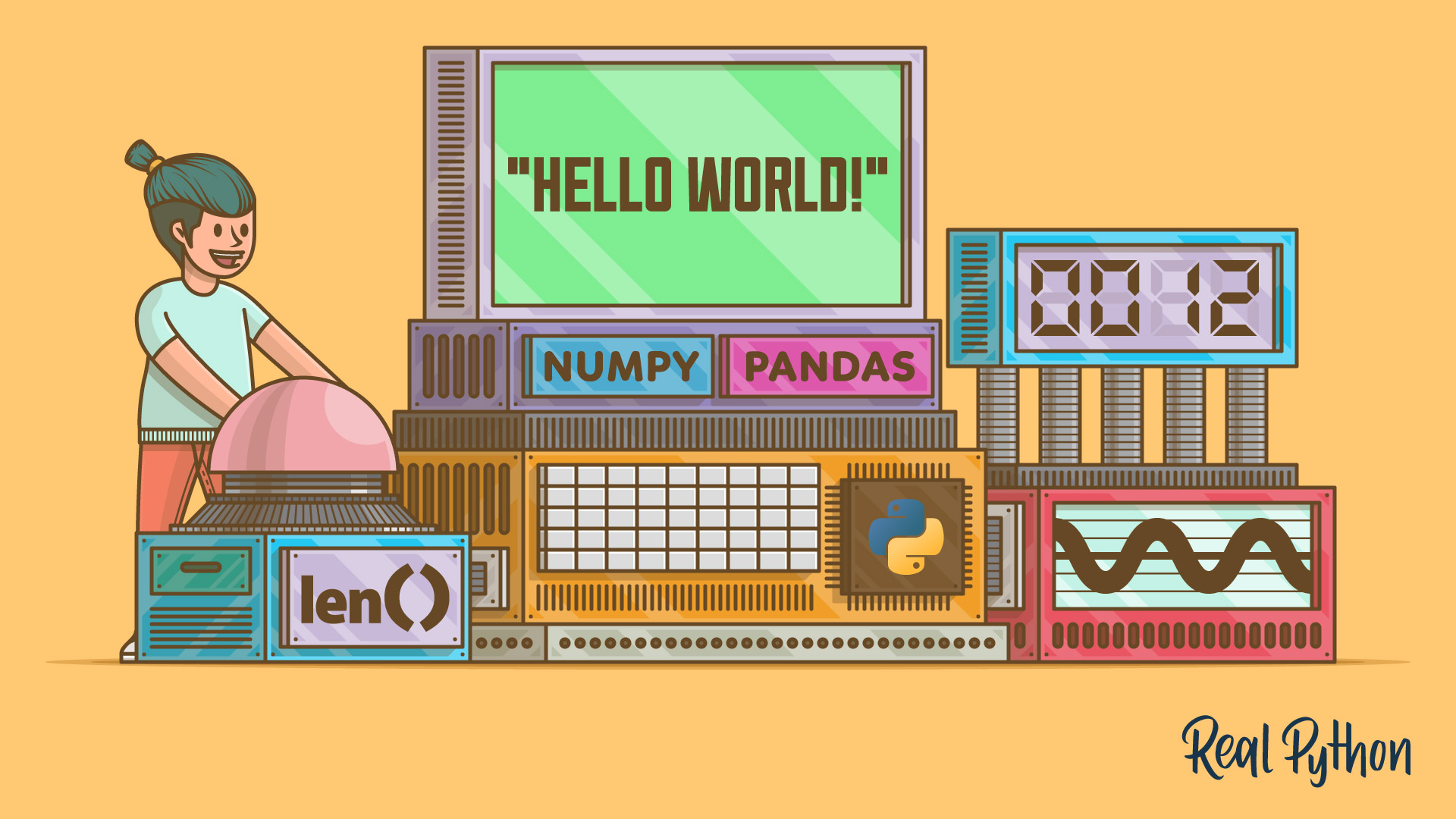
Course
Python's len() Function
Learn how and when to use the len() Python function. You'll also learn how to customize your class definitions so that objects of a user-defined class can be used as arguments in len().
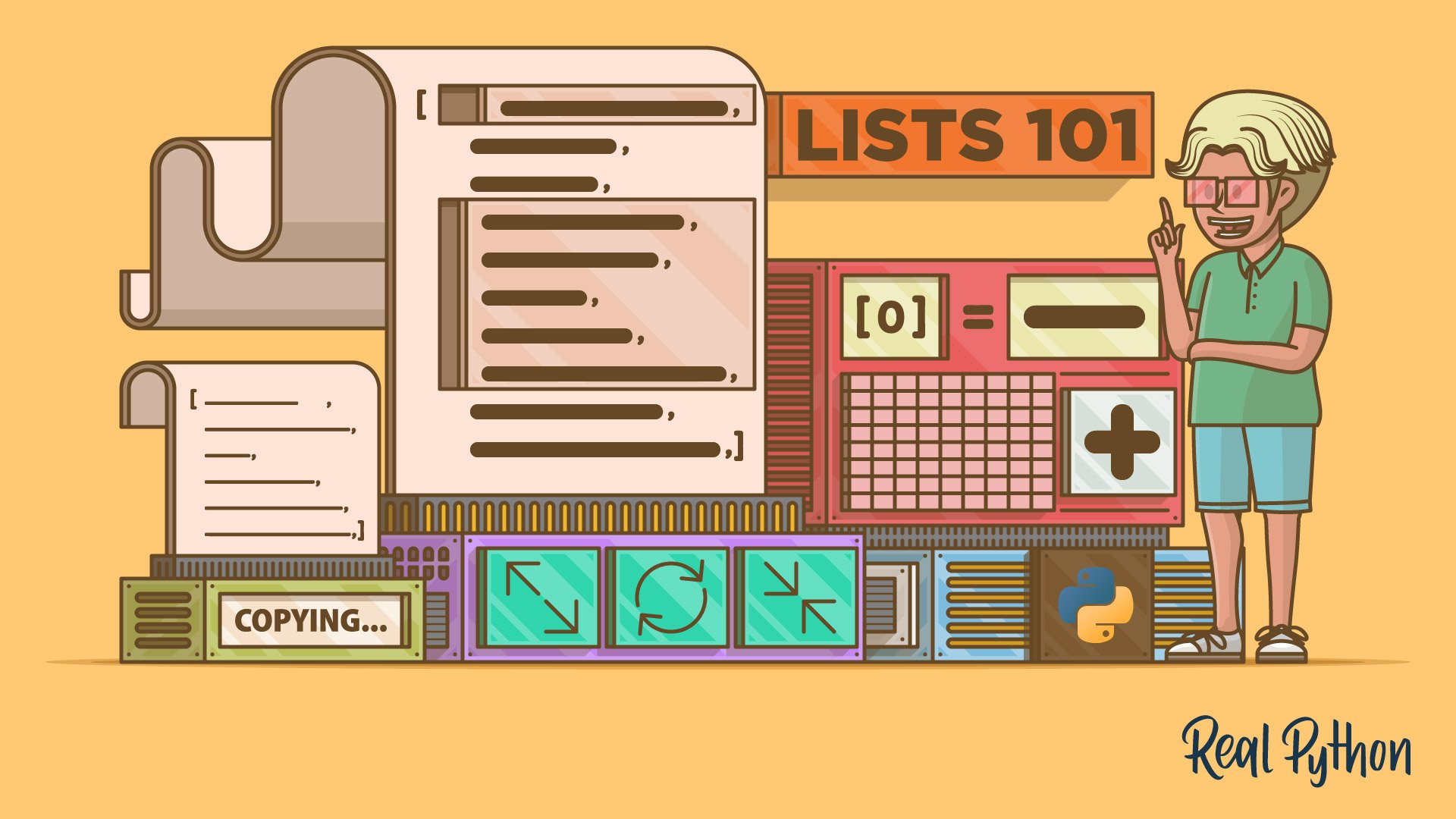
Course
Exploring Python's list Data Type With Examples
In this video course, you'll dive deep into Python's lists: how to create them, update their content, populate and grow them - with practical code examples.
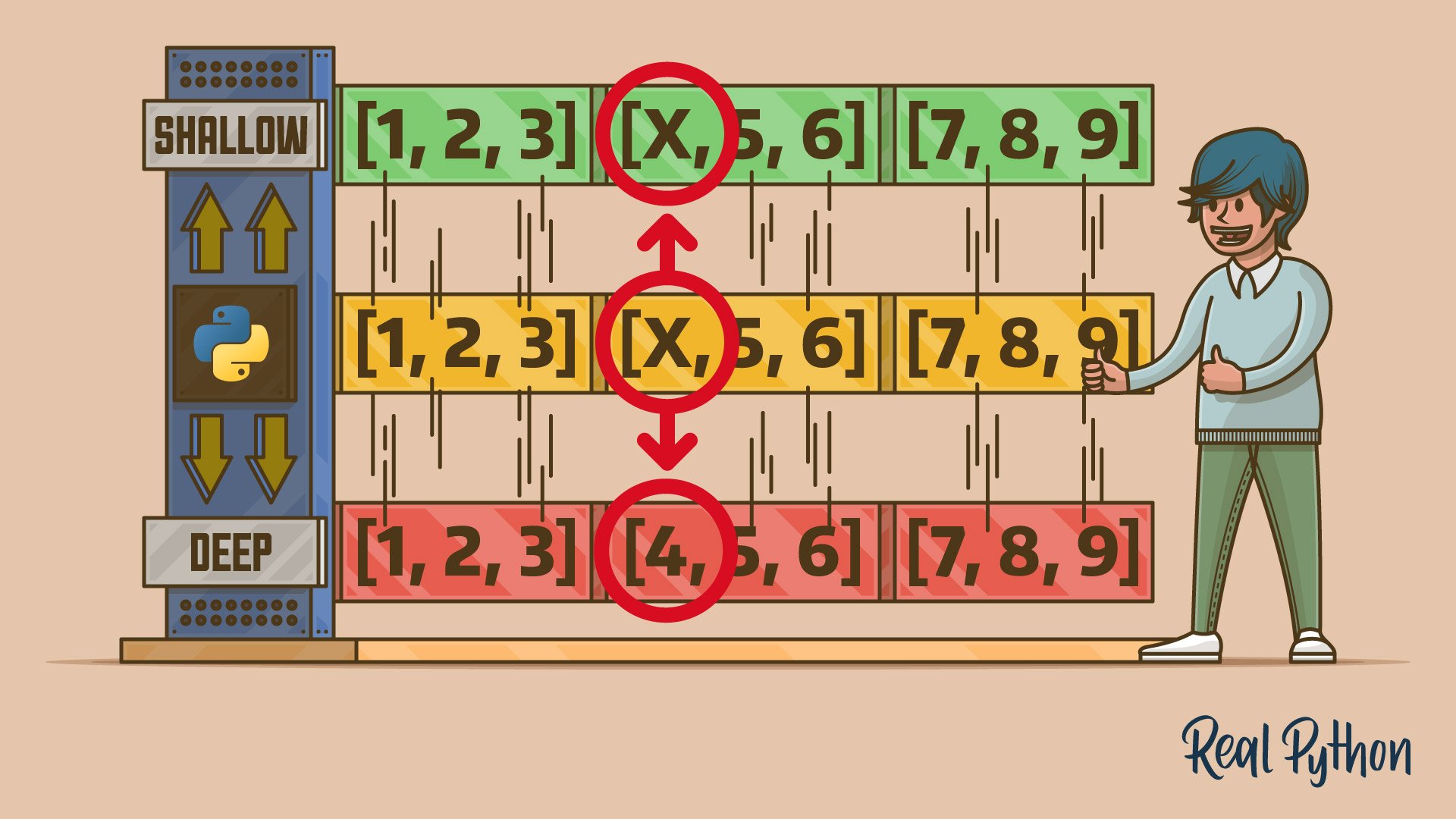
Tutorial
How to Copy Objects in Python: Shallow vs Deep Copy Explained
Understand the difference between shallow and deep copies in Python. Learn how to duplicate objects safely using the copy module and other techniques.
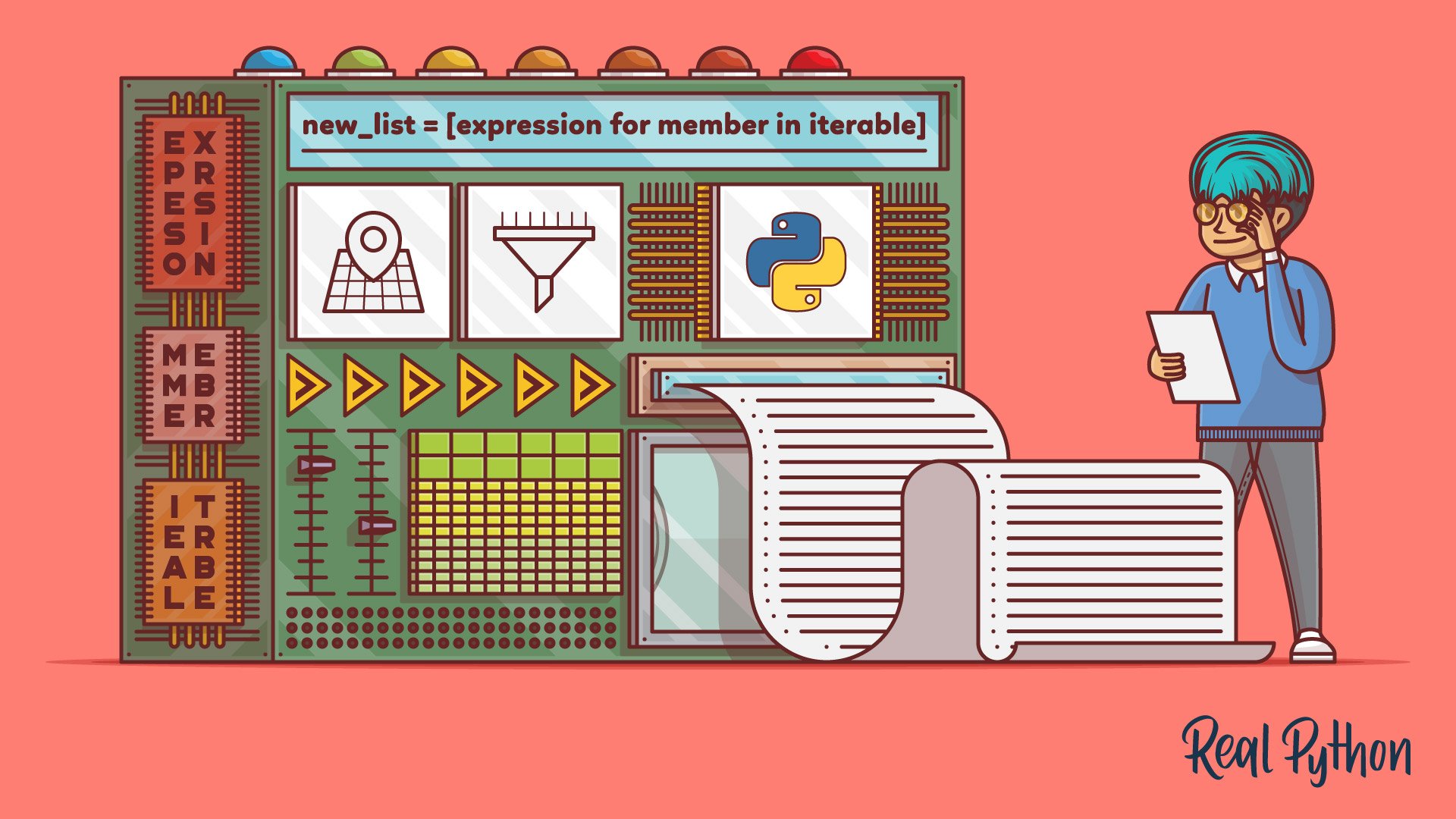
Course
Understanding Python List Comprehensions
Python list comprehensions make it easy to create lists while performing sophisticated filtering, mapping, and conditional logic on their members. In this course, you'll learn when to use a list comprehension in Python and how to create them effectively.
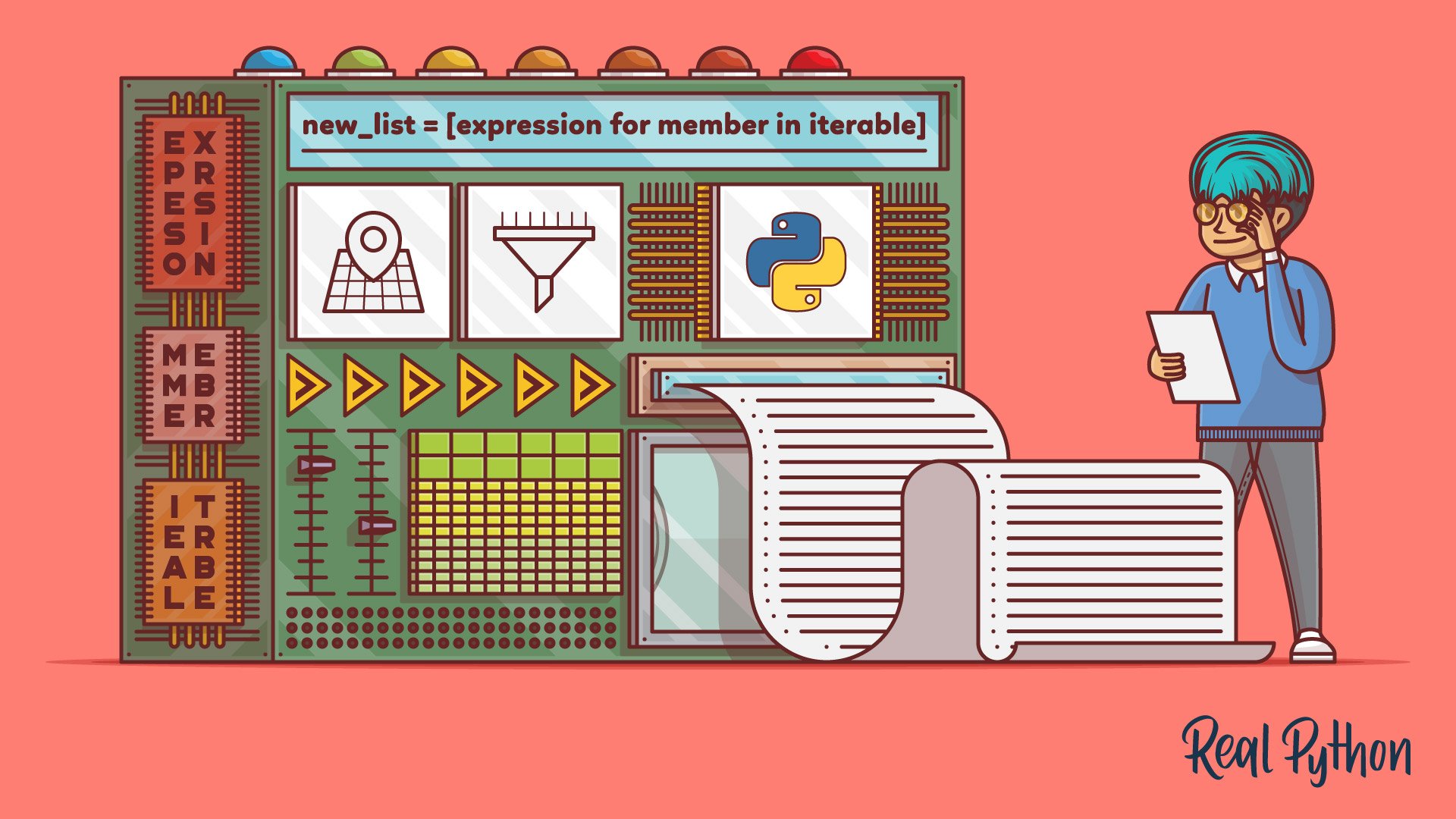
Interactive Quiz
When to Use a List Comprehension in Python
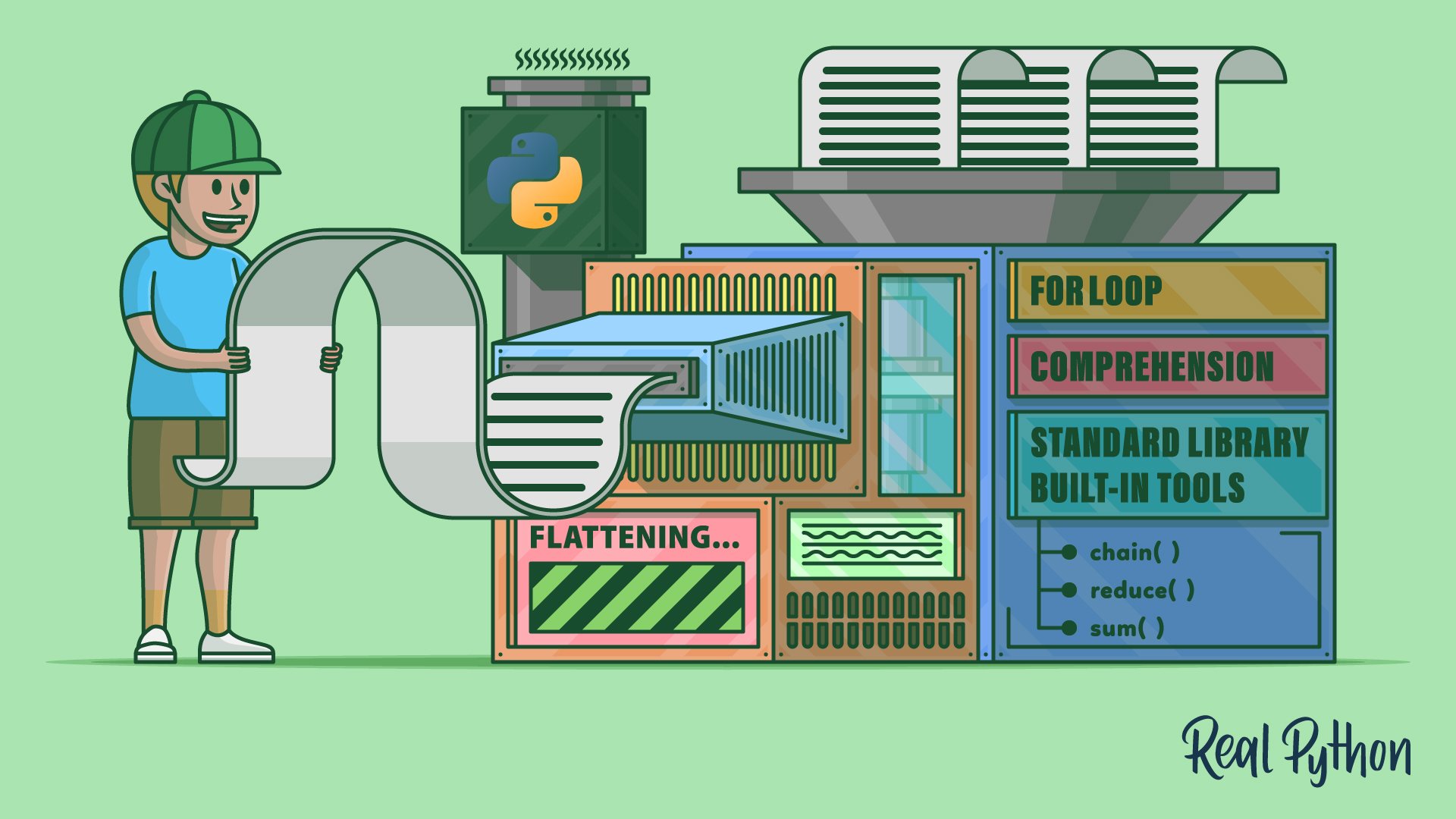
Course
Flattening a List of Lists in Python
Learn how to flatten a list of lists in Python. You'll use different tools and techniques to accomplish this task. First, you'll use a loop along with the .extend() method of list. Then you'll explore other tools, including reduce(), sum(), itertools.chain(), and more.
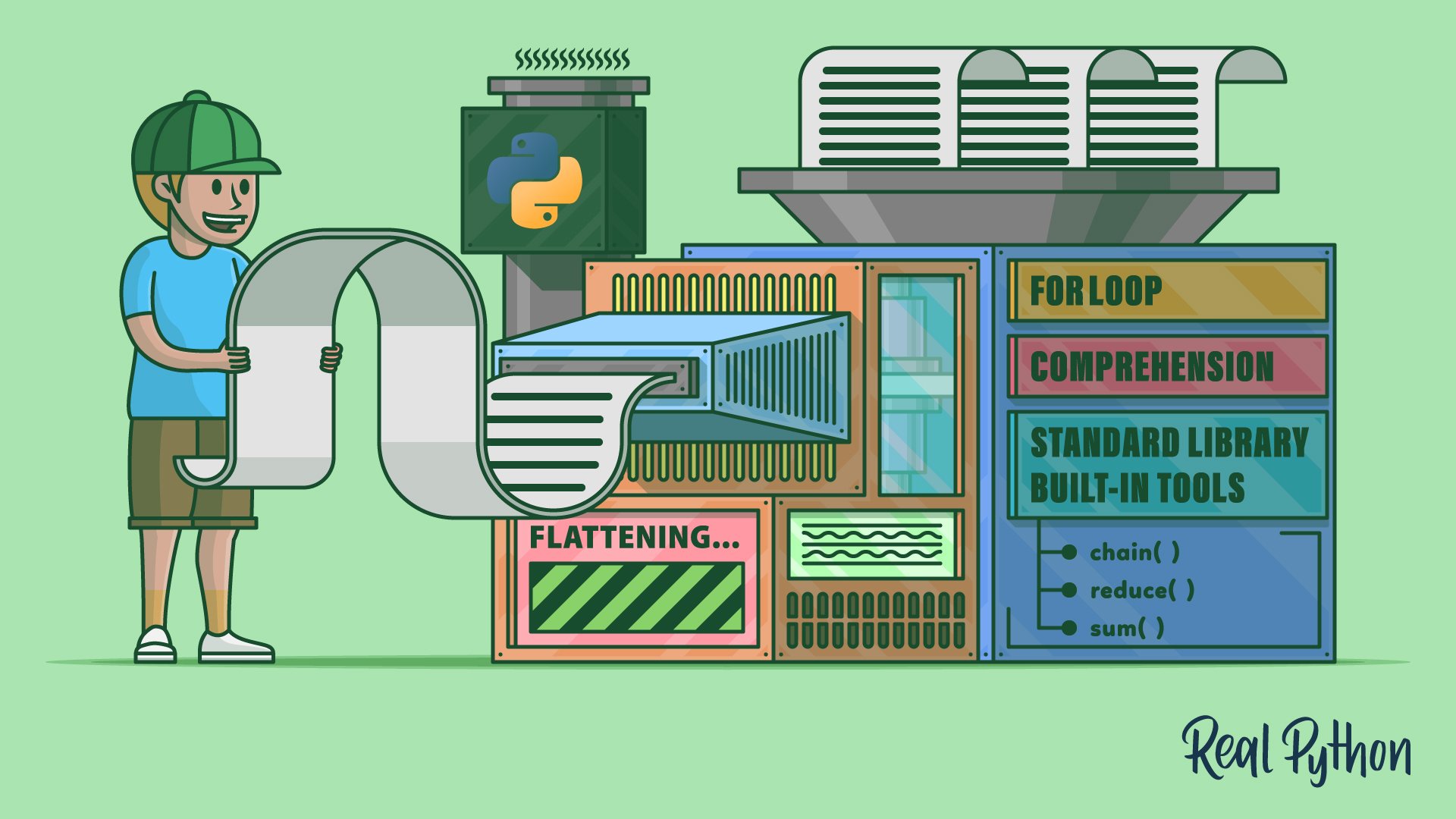
Interactive Quiz
How to Flatten a List of Lists in Python
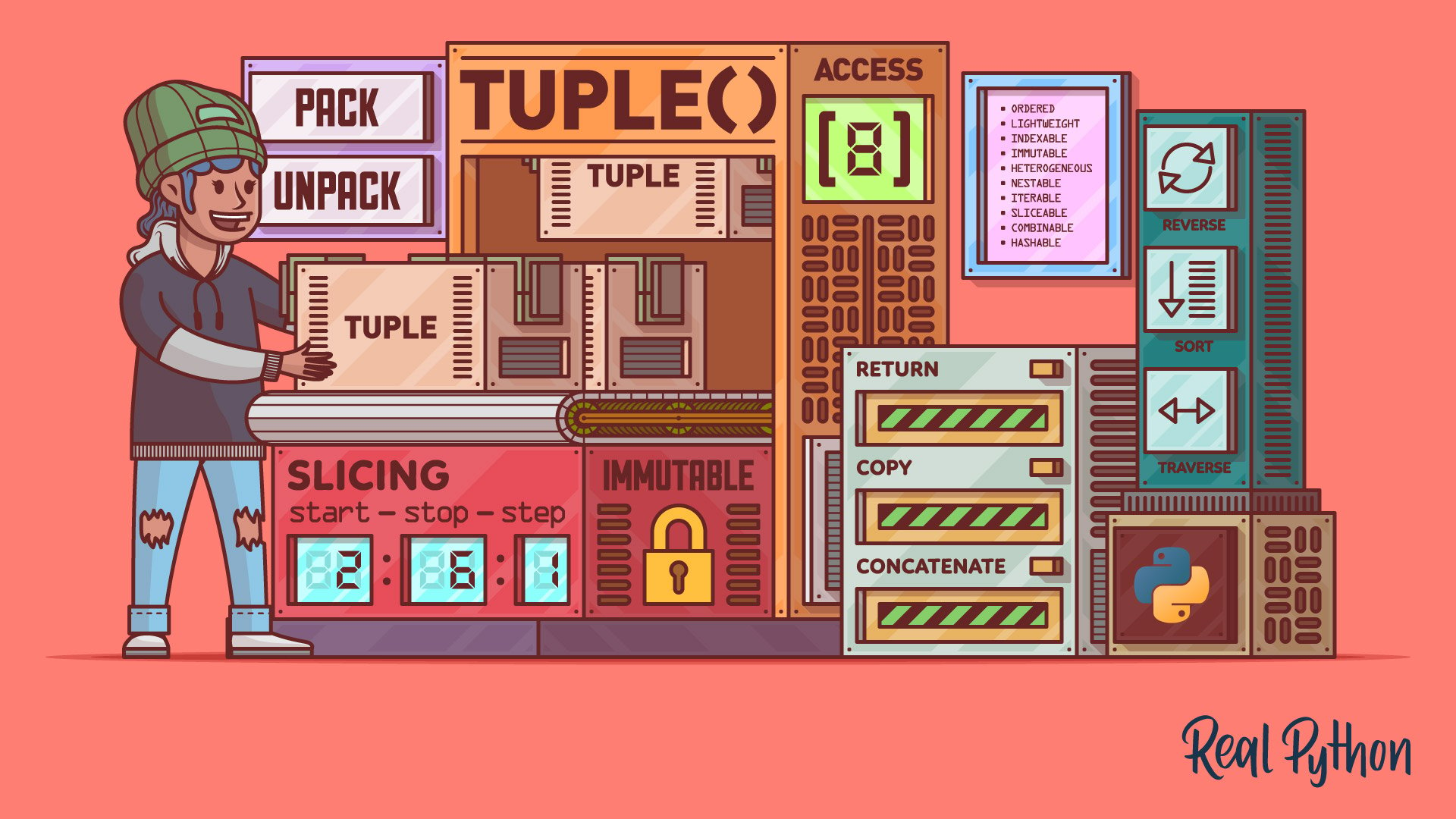
Course
Exploring Python's tuple Data Type With Examples
In Python, a tuple is a built-in data type that allows you to create immutable sequences of values. The values or items in a tuple can be of any type. This makes tuples pretty useful in those situations where you need to store heterogeneous data, like that in a database record, for example.
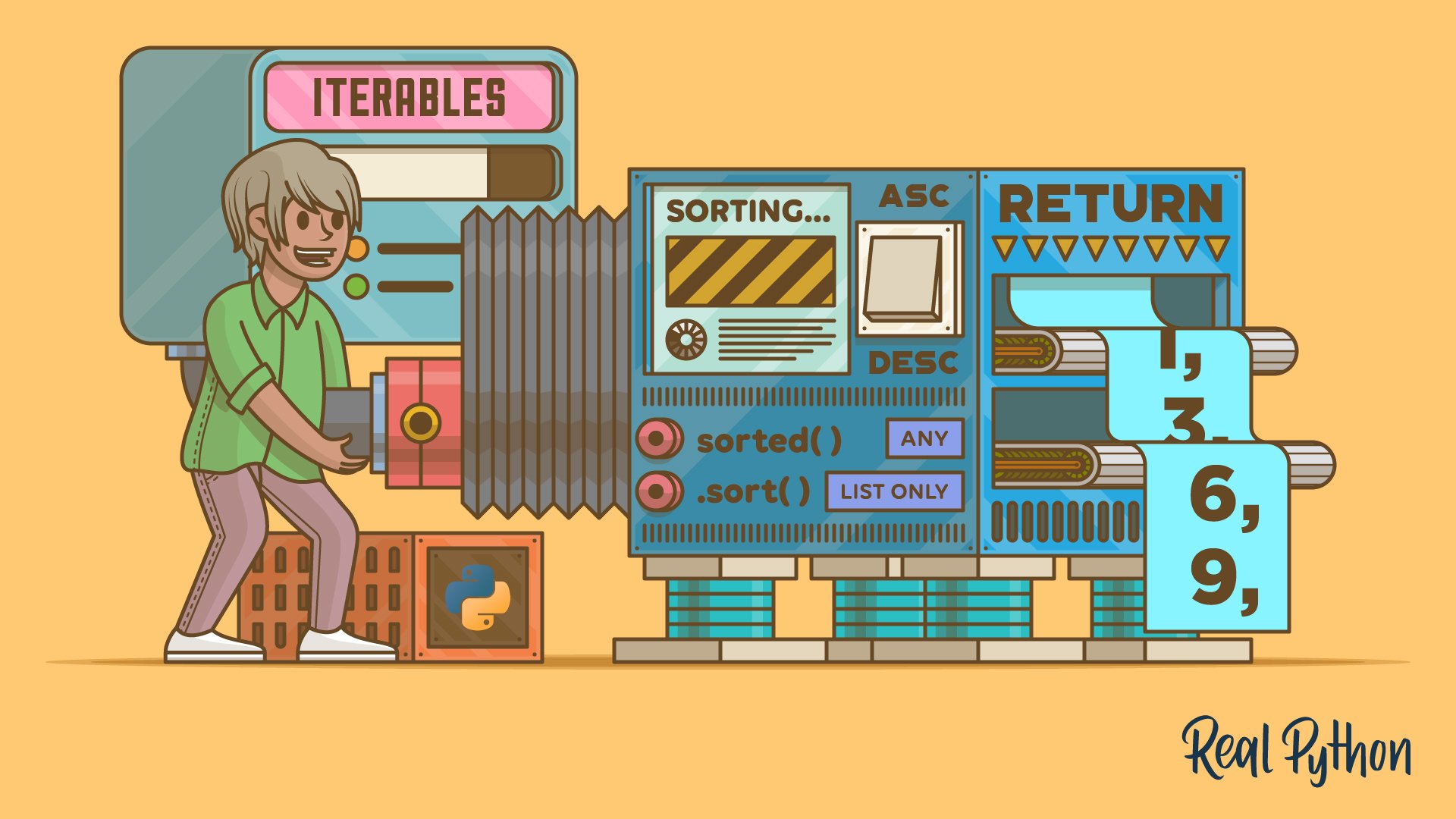
Course
Sorting Data With Python
In this step-by-step course, you’ll learn how to sort in Python. You'll know how to sort various types of data in different data structures, customize the order, and work with two different ways of sorting in Python.
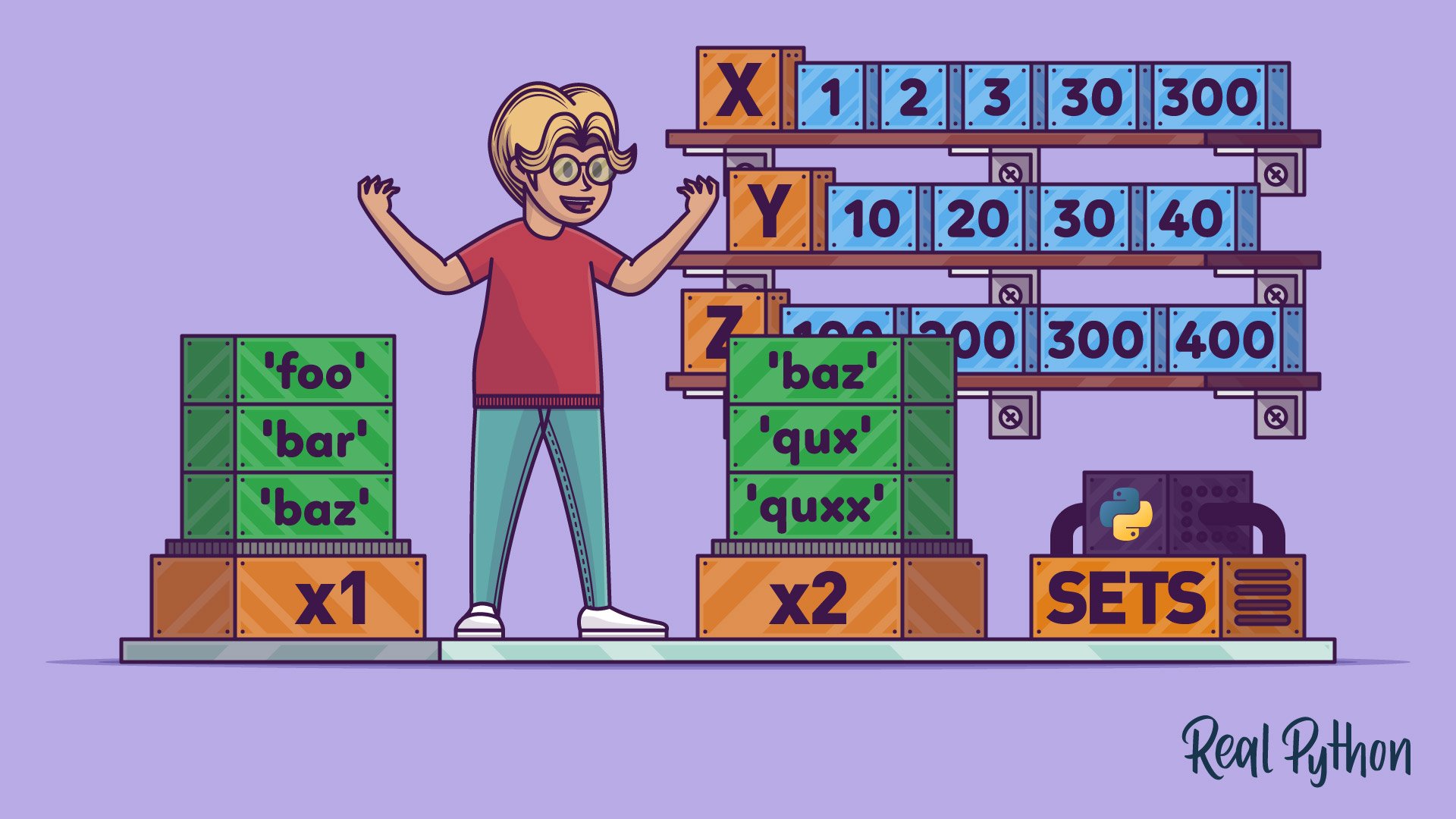
Course
Using Sets in Python
Learn how to work with Python's set data type. You'll see how to define set objects in Python and discover the operations that they support. By the end of this course, you'll have a good feel for when a set is an appropriate choice in your own programs.
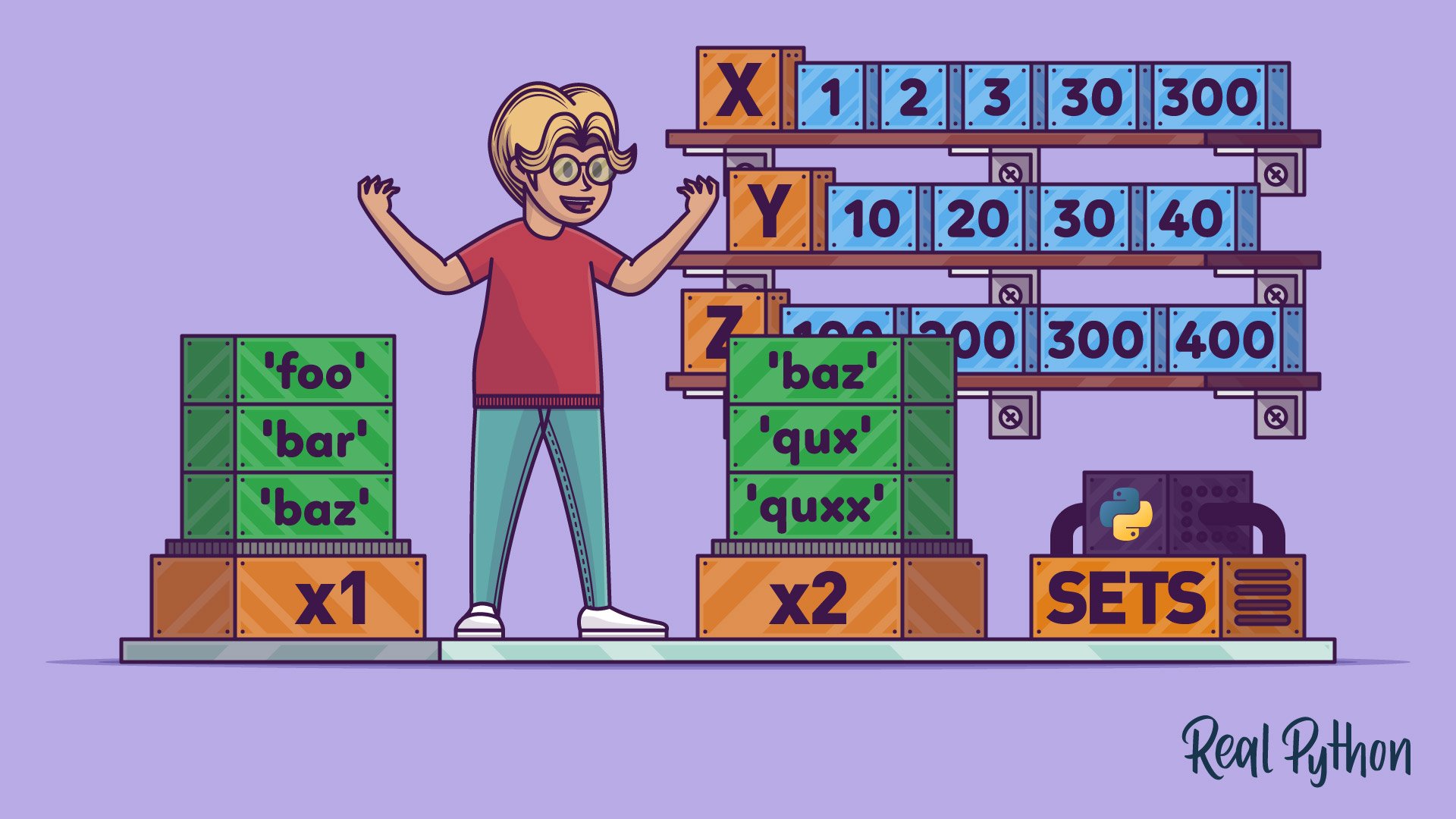
Interactive Quiz
Python Sets
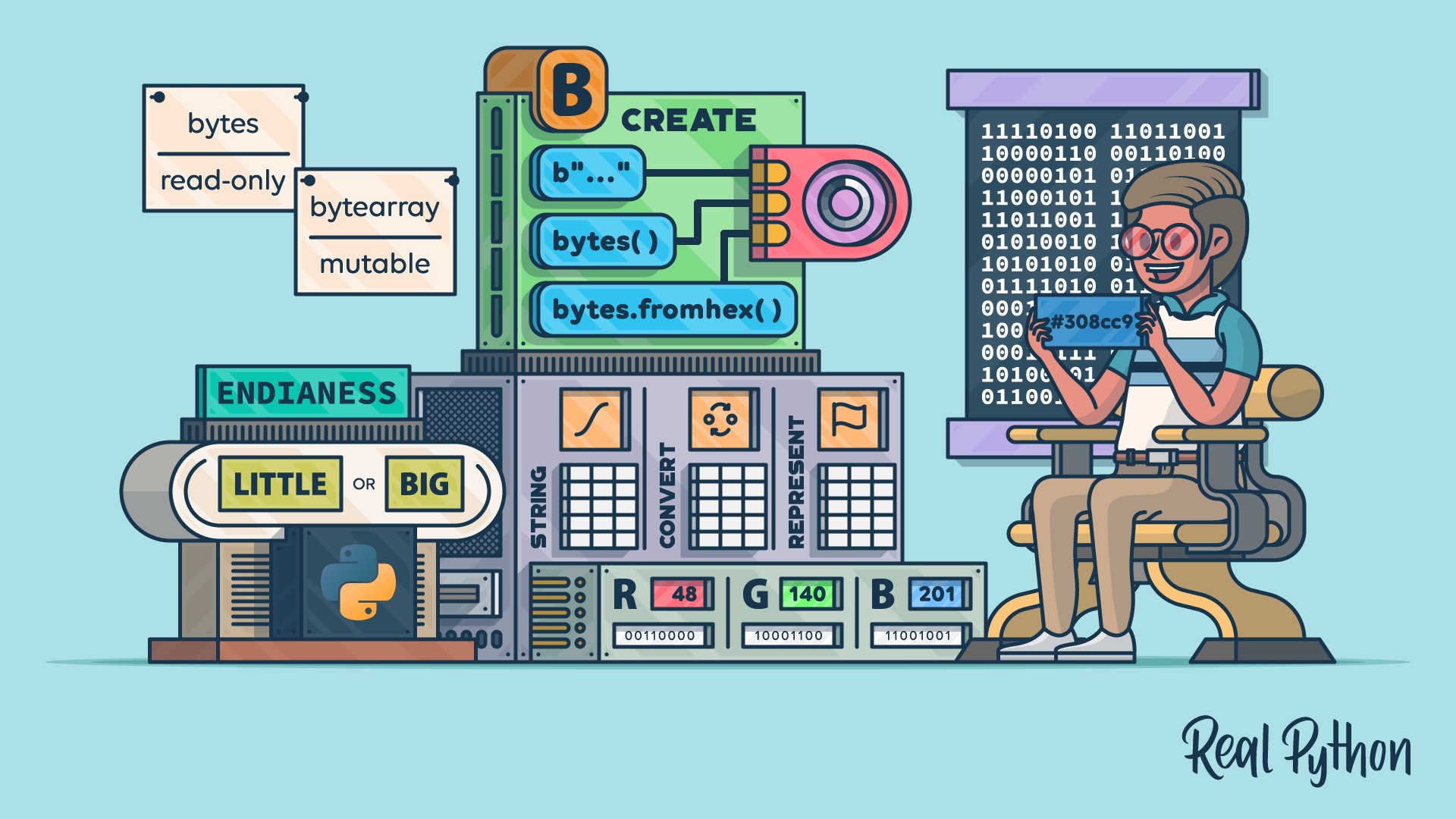
Tutorial
Bytes Objects: Handling Binary Data in Python
Learn about Python's bytes objects, which help you process low-level binary data. You'll explore how to create and manipulate byte sequences in Python and how to convert between bytes and strings. Additionally, you'll practice this knowledge by coding a few fun examples.
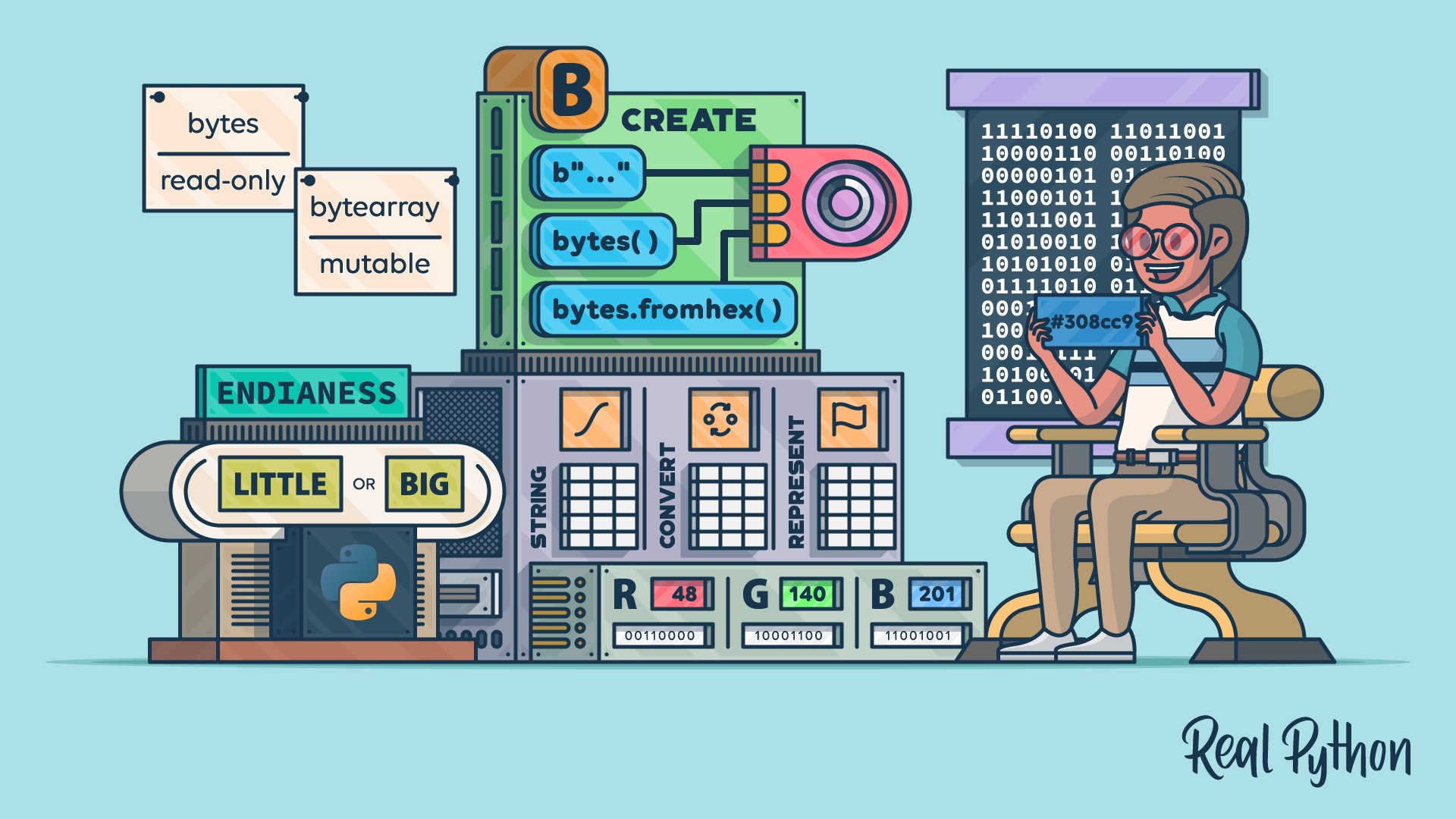
Interactive Quiz
Python Bytes
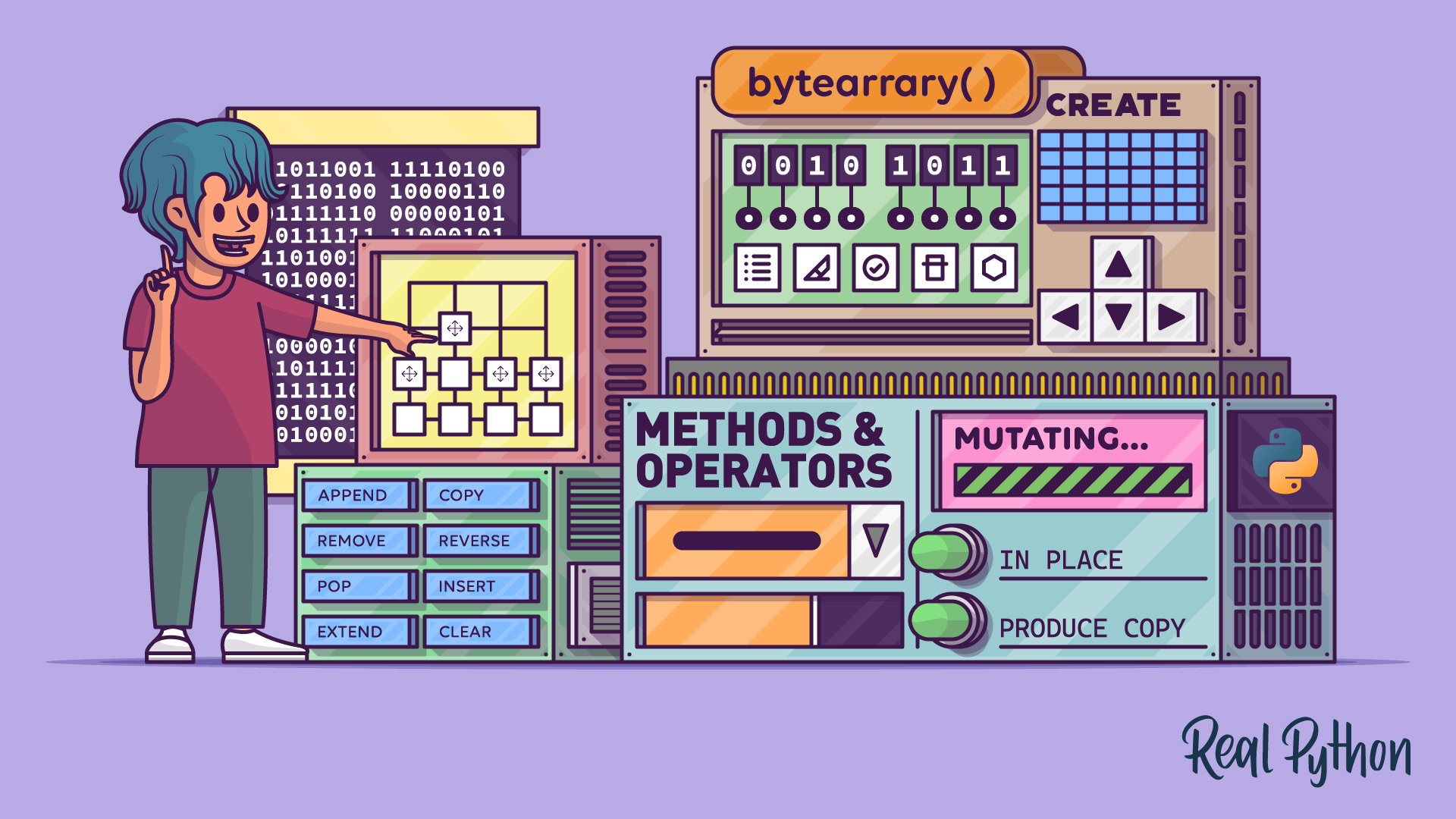
Tutorial
Python's Bytearray: A Mutable Sequence of Bytes
Learn about Python's bytearray, a mutable sequence of bytes for efficient binary data manipulation. You'll explore how it differs from bytes, how to create and modify bytearray objects, and when to use them in tasks like processing binary files and network protocols.
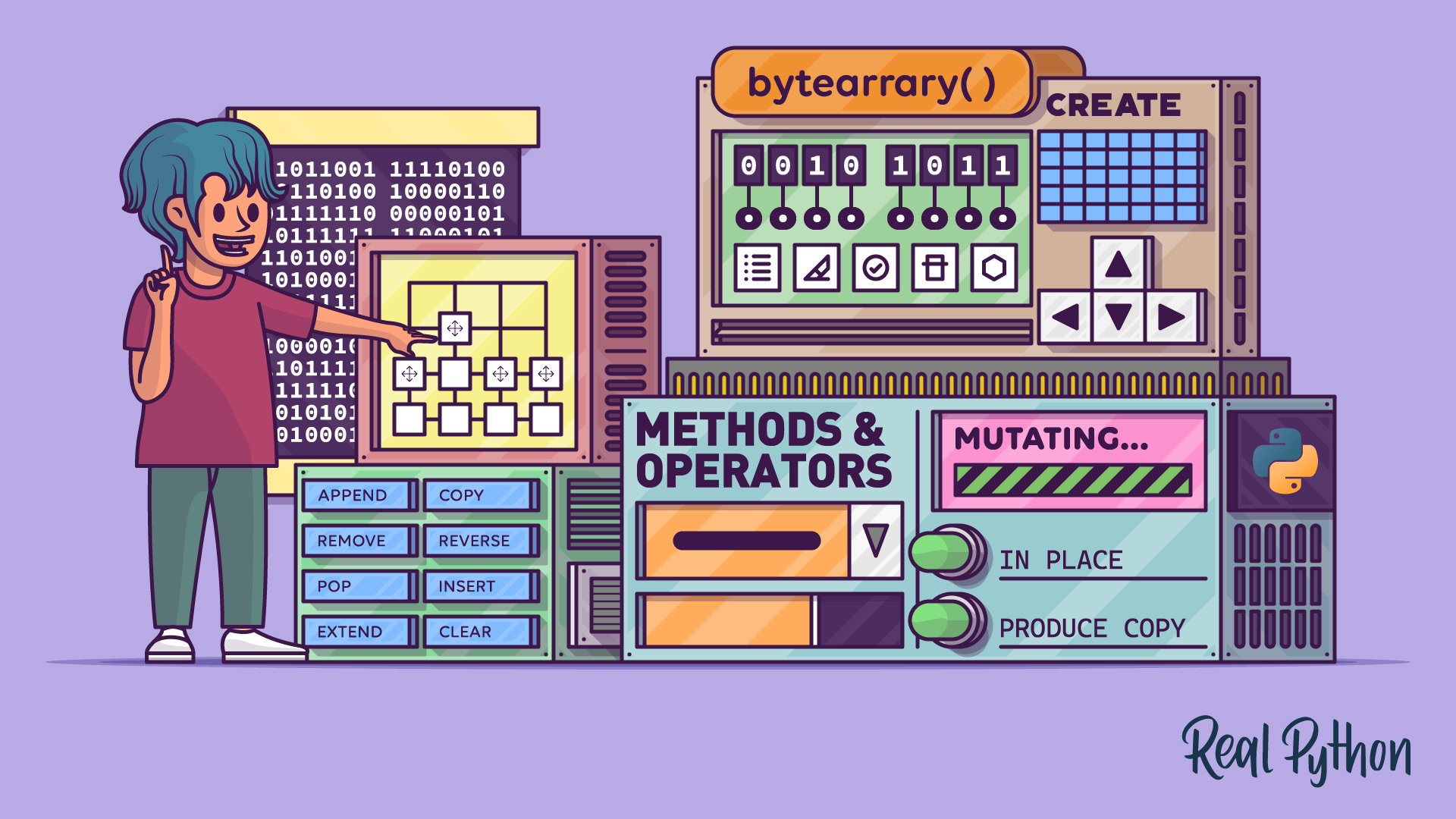
Interactive Quiz
Python's Bytearray
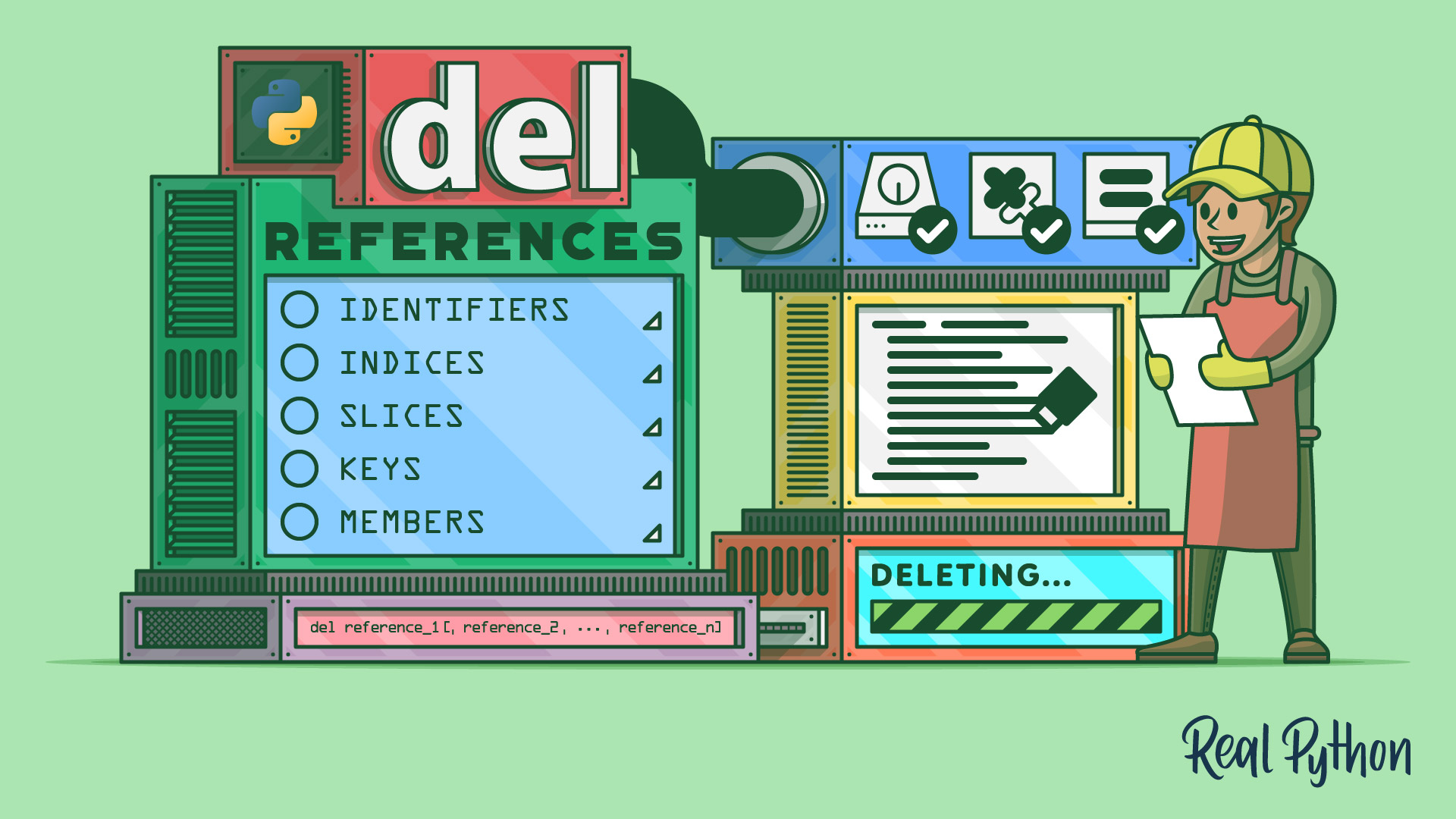
Tutorial
Python's del: Remove References From Scopes and Containers
Learn how Python's del statement works and how to use it in your code. This statement will allow you to remove references from different scopes, items from lists, keys from dictionaries, and members from classes. This will lead to potentially memory-efficient code.
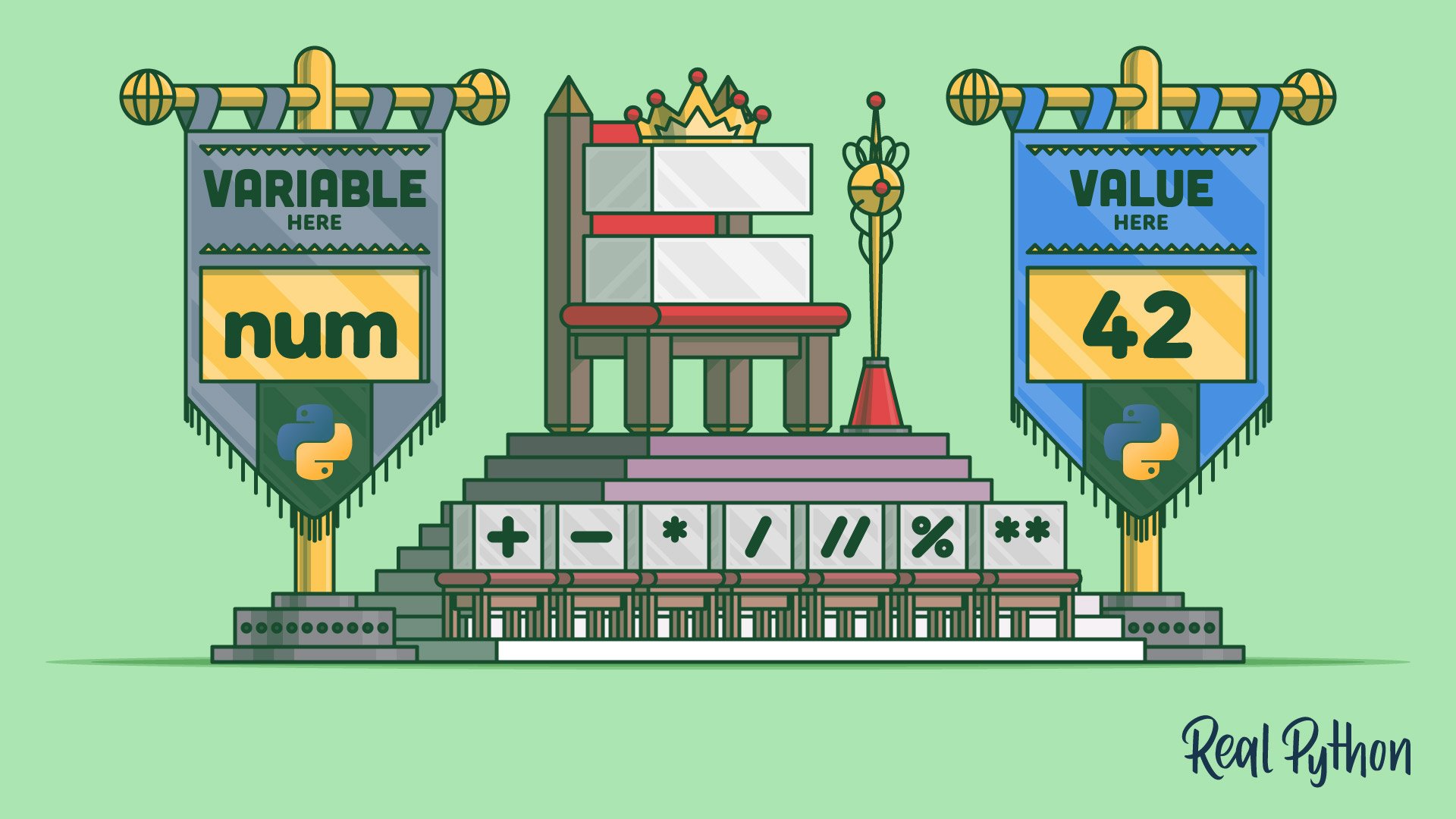
Tutorial
Python's Assignment Operator: Write Robust Assignments
Learn how to use Python's assignment operators to write assignment statements that allow you to create, initialize, and update variables in your code.
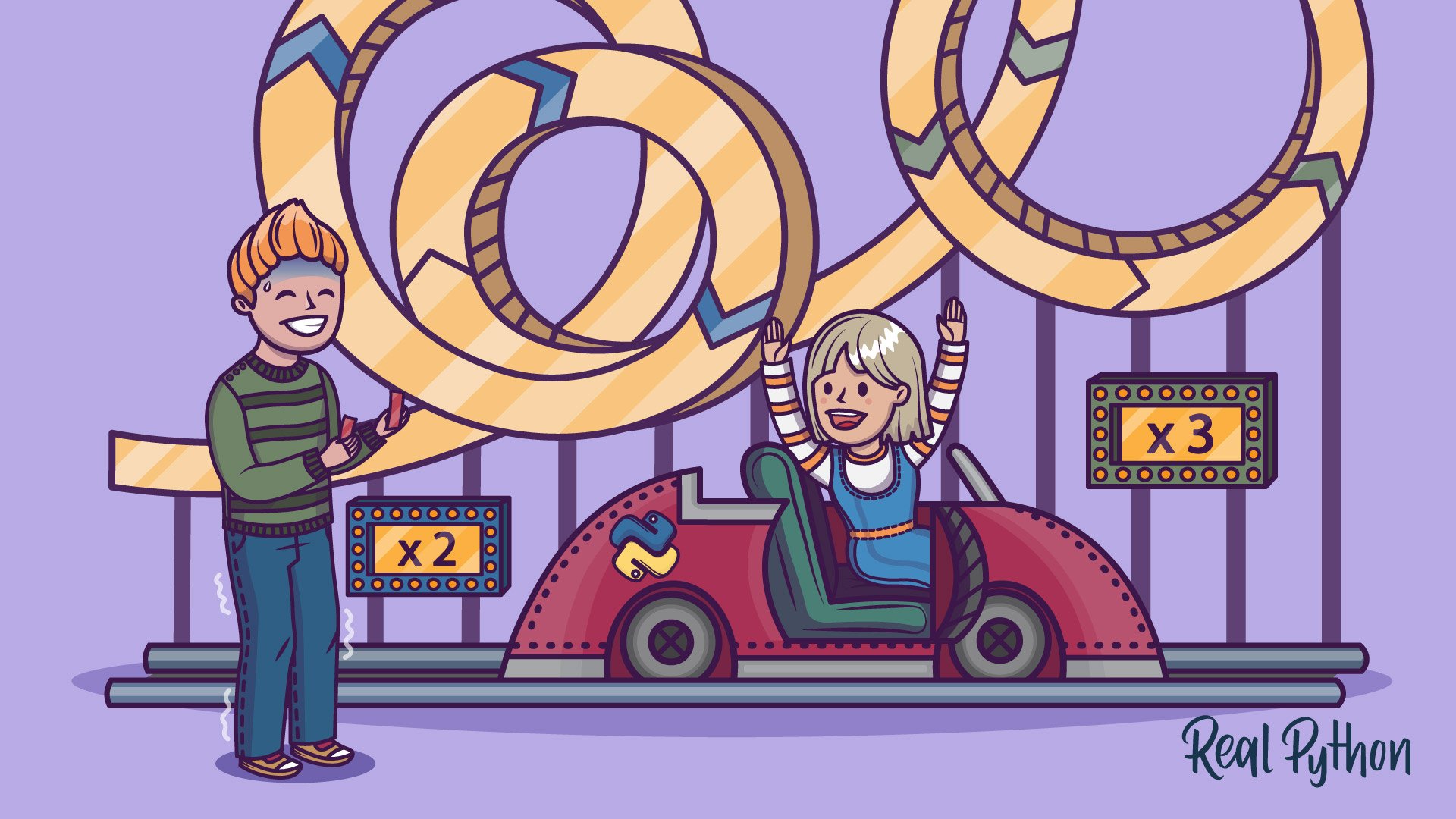
Course
The Python range() Function
In this step-by-step course, you'll master the Python range() function, learn how its implementation differs in Python 3 vs 2, and see how you can use it to write faster and more Pythonic code.
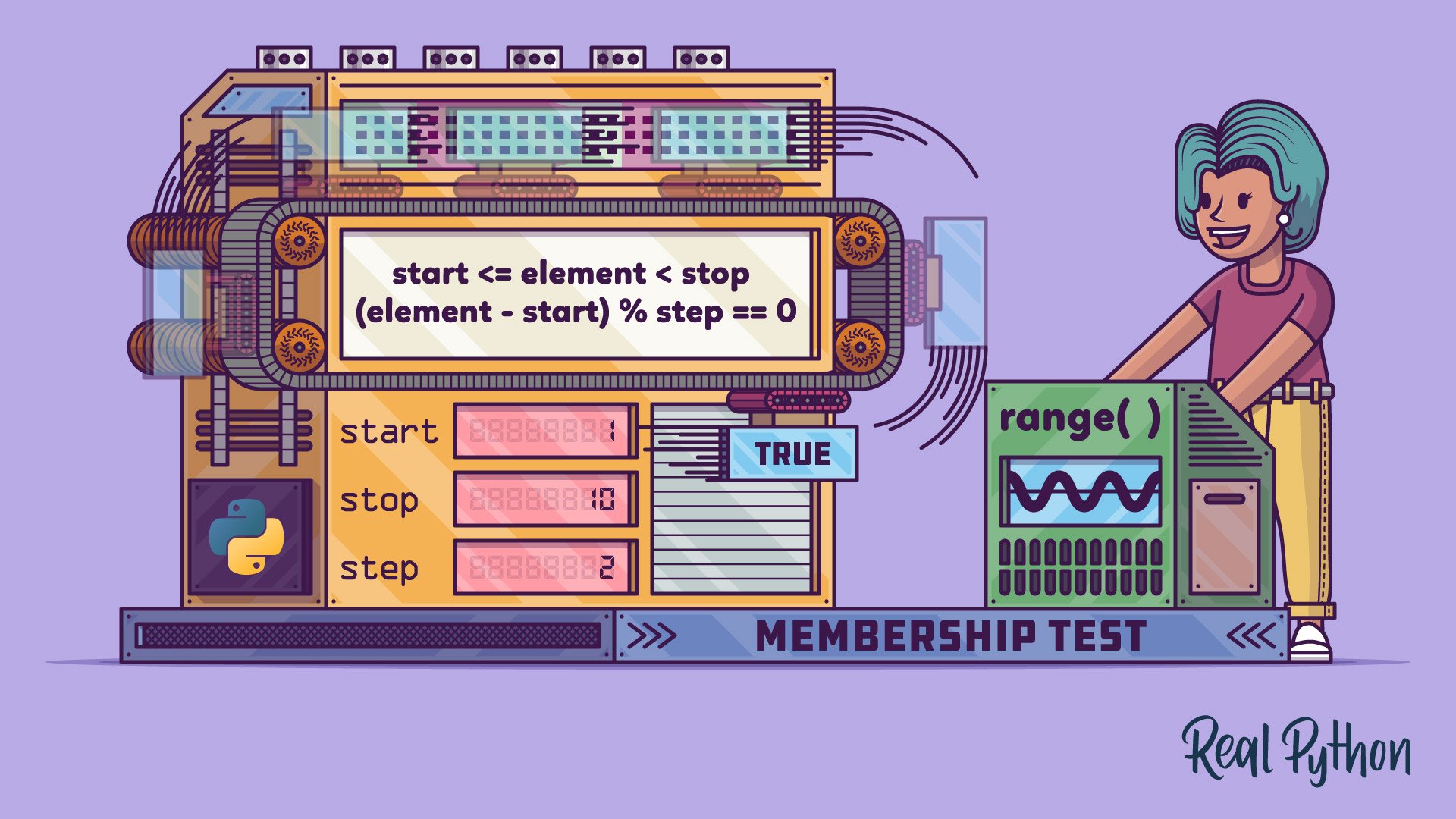
Tutorial
Why Are Membership Tests So Fast for range() in Python?
In Python, range() is most commonly used in for loops. However, ranges have some other use cases too, as they share many properties with lists. In this tutorial, you'll explore why it's so fast to perform a membership test on a Python range.
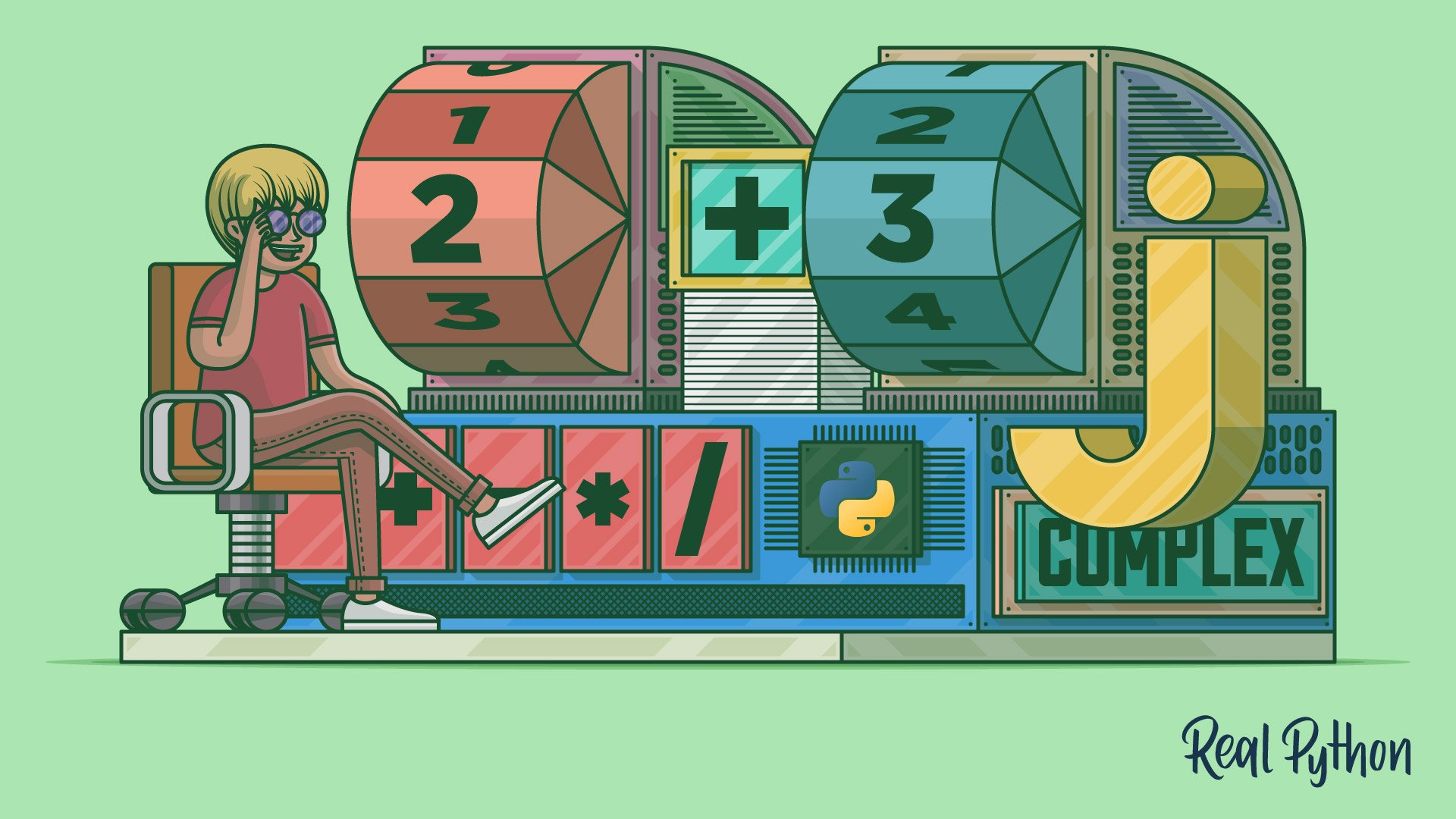
Tutorial
Simplify Complex Numbers With Python
Learn about the unique treatment of complex numbers in Python. Complex numbers are a convenient tool for solving scientific and engineering problems. You'll experience the elegance of using complex numbers in Python with several hands-on examples.
Got feedback on this learning path?
Looking for real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session. Happy Pythoning!