Adding a Constructor
00:00
Being able to manually create nodes and link them together is fine, but it’s a bit of a pain and it’s easy to mess up. Instead, let’s make it so that we can instantiate a new LinkedList
object by passing in a list of strings, similar to how collections.deque
works.
00:21
The LinkedList
class will automatically create nodes for each string and link them together for us. To do that, I’m going to modify the constructor to the LinkedList
class.
00:33
It should take an optional parameter of nodes
, which is the list of strings the user can supply. If no nodes
list is supplied, then the linked list will start out as empty, just like before. First, we’ll check to see if the user supplied a list.
00:51
If they did, we should iterate through the list, creating nodes and linking them together. I’ll say node = Node(data=nodes.pop(0))
.
01:06
This will get the first string in the nodes
list and use it to create a new Node
. Since this is the first node in our linked list, we set the .head
of the LinkedList
to point to this Node
.
01:18
All that’s left to do is iterate through the remaining strings in the nodes
list, using them to create a new node for the linked list we’re building.
01:30
Since we’re creating new nodes and linking them to the .next
attribute of the current node we’re on, it’s important that we set node
equal to node.next
before the next iteration of
01:43
this for
loop. Let’s try this out. With my module imported, I’ll start by creating a new list of strings. Now, I can pass that list into a new LinkedList
object
01:59
and finally, inspect the LinkedList
. As you can see, working with linked lists can be tricky. However, building out these methods for utilizing the linked list is important.
02:12
It abstracts all the complex code of managing references away from the end user of the LinkedList
object so they don’t accidentally mess up the whole list and lose all the data.
02:24
In the next few videos, we’ll create more methods that provide a nice interface for our LinkedList
. This is great practice.
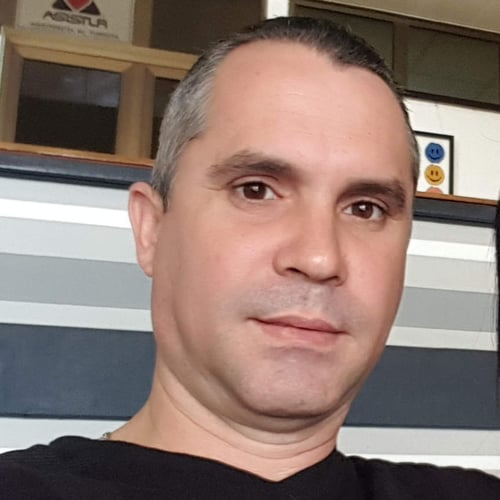
Leodanis Pozo Ramos RP Team on May 18, 2022
Hey @penguin! I’d say that more than a convention, this is a recommended practice. Using keyword arguments in function/method calls makes clear what argument you’re passing the value to.
So, whenever possible, apply this recommendation in your code. It’ll definitely makes the code easier to understand, maintain, and also more Pythonic.
Become a Member to join the conversation.
penguin on May 17, 2022
Is it a Python convention to write node = Node(data=nodes.pop(0))
instead of node = Node(nodes.pop(0))
in line 13 and similarly in line 16?