Alternatives to Standard Classes
00:00 Alternatives to data classes. For simple data structures, you’ve probably already used a tuple or a dictionary. You could represent the queen of hearts card in either of the following ways.
00:30 This does work, but it puts a lot of responsibility on you as a programmer. You need to remember that the queen of hearts variable represents a card. For the tuple version, you need to remember the order of attributes.
00:45
Writing ('Spades', 'A')
will mess up your program but probably not give you an easily understandable error message. If you use the dict
version, you must make sure the names of the attributes are consistent.
01:00
The example shown onscreen would not work as expected. Furthermore, using these structures is not ideal. The tuple does not give us named access, and the dict
version doesn’t give named attribute lookup.
01:27
A better alternative is the namedtuple
. It’s long been used to create readable small data structures, and you can, in fact, recreate the data class example already seen using a namedtuple
as seen onscreen.
01:56
This definition of NamedTupleCard
will give this same output as the DataClassCard
seen earlier on did.
02:25
So why even bother with data classes? First of all, data classes come with many more features than you’ve seen already. At the same time, the namedtuple
has some other features that are not necessarily desirable.
02:39
By design, a namedtuple
is a regular tuple
, which can be illustrated by this comparison seen onscreen. While this might seem like a good thing, this lack of awareness about its own type can lead to subtle and hard-to-find bugs, especially since it will happily compare two different namedtuple
classes.
03:29
The namedtuple
has also come with some restrictions. For instance, it’s hard to add default values to some of the fields in the namedtuple
.
03:37
The namedtuple
is also by nature immutable. That is, the value of a namedtuple
can never change. In some applications, this is an awesome feature, but in other settings, it would be nice to have more flexibility.
04:00
Data classes will not replace all uses of namedtuple
. For instance, if you need your data structure to behave like a tuple, then a namedtuple
is a great alternative! Another alternative, and one of the inspirations for data classes, is the attrs
project.
04:19
With attrs
installed as seen here, you can write a card class as follows.
04:46
This can be used in exactly the same way as the DataClassCard
and NamedTupleCard
examples seen earlier on.
05:17
The attrs
project does support some features that data classes do not, including converters and validators. Furthermore, attrs
has been around for a while and is supported in older versions of Python.
05:30
But attrs
isn’t part of the standard library, and as such, it would add an external dependency to your projects. If you use data classes, similar functionality will be available everywhere without external dependencies.
05:45
In addition to tuple
, dict
, namedtuple
, and attrs
, there are other similar projects, including typing.NamedTuple
, attrdict
, plumber
, and fields
. While data classes are a great feature, there are still use cases where one of the older variants fits better.
06:06 For instance, if you need compatibility with a specific API expecting tuples or need functionality not supported in data classes. Now that you’ve seen what the alternatives may be, in the next chapter, you’ll get back to implementing data classes, starting with a simple example.
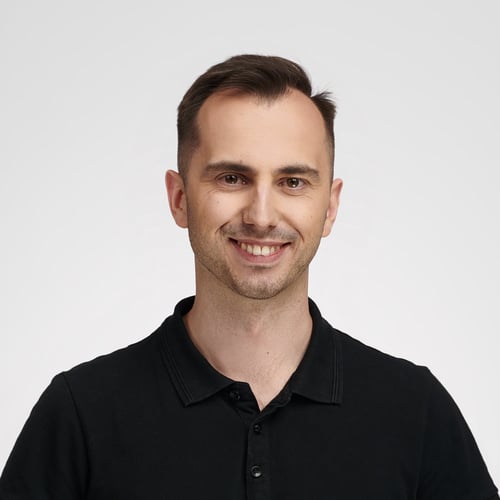
Bartosz Zaczyński RP Team on Sept. 29, 2021
@user45755 The clue is in the name: data classes are suitable for representing objects that mostly carry data with little or no behaviors attached to them. Depending on the context, they’re sometimes called Data Transfer Objects (DTO) or Value Objects (VO). In a nutshell, data classes generate a bunch of boilerplate code that you’d otherwise have to write by hand.
Note: If you’ve done any Java development, then data classes in Python are somewhat similar to record classes introduced in Java 14 or lombok’s @Data annotation.
To give you a quick summary of the built-in data types mentioned in the video, here are their typical use cases:
tuple
: An immutable sequence of elements that you can access by index, e.g.,color[2]
dict
: A mutable collection of key-value pairs that you can access by key, e.g.,color["blue"]
collections.namedtuple
: Liketuple
, but you can give names to indices, e.g.,color.blue
is the same ascolor[2]
typing.NamedTuple
: Likenamedtuple
, but you can annotate elements with type hints for improved type safetydataclasses.dataclass
: LikeNamedTuple
but mutable and highly configurable
I can’t speak of the external libraries like attrs
because I haven’t used them, but I’m sure they all have their uses. Having many tools at your fingertips gives more freedom of choice and allows for choosing the best one for the job.
Become a Member to join the conversation.
user45755 on Sept. 29, 2021
All I took away is data classes make obsolete longer alternatives…
The problem is: what’s the takeaway message? This is aimed at advanced users who have the background to understand the nuance.
“The tuple does not give us named access, [index instead] and the dict version doesn’t give named attribute lookup.” Ok, well, so what? Why would you want these things?