Creating Your Own Functions
To learn more about Python’s style guide, check out How to Write Beautiful Python Code With PEP 8.
00:00
In the previous lesson, you learned how to execute functions. Now it’s time to create your own function. You’ll start by creating a print()
function that also returns its input value.
00:11 Before we hop over to our code editor again, let’s think of a name for our function. Just like with variable names, function names must follow some rules.
00:21 The rules for naming a function are the following. A function name can contain letters, numbers, or underscores. A function name, however, must not start with a number.
00:35 Let’s keep this rules in mind and start programming.
00:40
When you define a function, you start with the so-called function signature The function signature always starts with the def
keyword, which is short for define.
00:52
After the def
keyword comes the function name. With Python’s rules about naming functions in mind, let’s call it shout_and_return
. Oops, and there should be an underscore of course.
01:12
Then you add a pair of parentheses. When your function accepts arguments, you provide a list of parameters. Our shout_and_return()
function should only accept one argument, which we name input_string
.
01:31 A parameter is sort of like a variable, except that it has no value. It’s a placeholder for actual values that will be provided whenever the function is called with one or more arguments. At the end of your function signature, you must put a colon. When you hit enter, you’re inside the function body.
01:53
You can see that IDLE shows three dots at the beginning of the line, and my cursor is indented. This is vitally important. Every line that is indented below the function signature is understood to be part of the function’s body. We’ll play around with the indentation a bit later. For now, let’s continue with the function body. Define a variable called loud_input
, which is equal to input_string
—that’s the parameter that we are passing in—.upper()
.
02:29
The .upper()
method converts every character in the input string to uppercase.
02:35
Press Enter. You can see on the three dots that you are still in the function body. And now you call the print()
function with loud_input
as argument. If we would stop now, then our function would return None
, exactly the same as the print()
function. To return loud_input
, we must close the function body with a return
statement.
03:01
So press Enter again and then use the return
keyword and after it, loud_input
. So the shout_and_return()
function returns loud_input
in the end.
03:15
When you define a function in IDLE’s interactive window, you need to press Enter twice after the line containing return
for Python to register the function. So if I hit Return, as you can see, IDLE is smart enough to jump out of the function body after the return
statement, but there are still three dots on the beginning of the line.
03:37
So I have to press Enter another time to actually jump out of the function body. You can see with the three carets (>>>
) at the beginning that we are now outside of the function body, and Python is waiting for our input.
03:52
Awesome. Let’s try out our new function. You call a user-defined function just like any other function. Type the function name followed by a list of arguments enclosed by parentheses. So for our function, it’s shout_and_return
, and with an opening parenthesis, you can see that IDLE already recommends that we put an input_string
as an argument.
04:19
So let’s put "hello"
in lowercase in it as a string, close the parentheses, and press Enter.
04:29
Now IDLE shows HELLO
in uppercase two times. One time, it’s the side effect of the print()
function we are calling inside of shout_and_return()
.
04:38
And the second time is the return value that IDLE shows. Let’s try to assign the return value to a variable. Let’s call the variable my_return
and set it equal to shout_and_return
, and this time let’s use "hi"
as a lowercase string, close the parentheses at the end, and press Enter.
05:07
Okay, that looks good. So now HI
is printed one time. Again, that’s the side effect of the print()
function. And if we are typing my_return
, Enter, then you see that my_return
now contains the value 'HI'
in uppercase letters. That’s pretty cool, but there is a caveat. Unlike built-in functions, user-defined functions are not available until they’ve been defined with the def
keyword.
05:35 So if you wouldn’t have defined the function at the top of this window, it wouldn’t be there. So you must define the function before you can call it. To explore a bit further what I mean by this, let’s move to the editor window.
05:52
Okay, on the right side, you now see the IDLE editor window. I have created a file called functions_and_loops.py
, and I saved it on the desktop.
06:05
Now let’s see what happens when we just call the shout_and_return()
function in the IDLE editor window without doing anything else. So I type shout_and_return()
and type "hello"
and close it off.
06:23 I save the file … and now I go to the menu and say Run Module. Later, I will shortcut this with F5.
06:36
And when I run the file, I get a NameError: name 'shout_
_and_return' is not defined
.
06:44
Although you defined the shout_and_return()
function in the IDLE prompt window before, it’s not available in your Python files by default. So again, that’s different to Python’s built-in functions that are available for you.
07:00 So to actually be able to use the function, let’s copy the code from the left window to the right window.
07:13 All right. I now copied the code to the editor window. I saved the file. And now I press F5 to run it … and still an error.
07:26
As you can see in the window on the left side, the error is exactly the same like the error before. Although we now have our shout_and_return()
function in our functions_and_loops
file, the issue is this: we call the function shout_and_return()
in line 1, but we define the function only in line 3, so Python doesn’t know about the function yet when it executes the shout_and_return()
function in line 1—or, to be more precise, when Python tries to execute shout_and_return()
in line 1. Let’s switch
08:04
them. I cut the function call from line 1, put the function definition there, and then I paste the shout_and_return()
call into line 6. I save the file and run it.
08:18
And now you see that on the left side, it prints HELLO
in uppercase letters. It works. As you can see, order is important.
08:29
This also holds true for the function body. And you need to pay special attention to the return
statement at the end of the function body. Once Python executes a return
statement, the function stops running and returns the value.
08:46
If any code appears below the return
statement and is indented like it’s part of the function body, then it will never run. For example, if you put the print()
function
09:00
after the return
statement
09:04
and save and run the file again, then Python doesn’t complain. It restarts the file, but the print()
statement is never executed. But it’s still valid Python, so there is no error.
09:20
So you really have to remember that the order is important and that you put your return
statement at the end of your function body. Oh, and speaking about order, when we first defined the shout_and_return()
function in the interactive window, I mentioned that the indentation is important.
09:41 Indentation is the space before your text. By default, that’s four spaces in IDLE and should also be four spaces in your editor of choice if you are using another editor.
09:57 Python is not strict with it. So you could also put all the code of your function body in with two spaces. Let me quickly fix that part here as well. So our function should work now again.
10:14
I put the print()
function on line 3 again and the return
statement on line 4 again, so the order is correct. But now I have two spaces in front of the function body.
10:24
I save the file, and now I run the file, and as you can see on the left window, HELLO
is printed in uppercase letters, so it works although we only have two spaces at the beginning of the function body.
10:39 Generally, you should stay with four spaces. That’s a convention that’s defined in PEP 8. That’s the style guide for Python. It says that it’s recommended to indent your code with four spaces.
10:53
While this is not a strict rule, there is one rule that you must follow when indenting code in a function’s body: every line must be indented by the same number of spaces. For example, if I only put two spaces here and I save the file and I try running it, then IDLE already shows a window: unindent does not match any outer indentation level
.
11:20 So you can’t even run this file with IDLE.
11:27 You also see that here there is a red line marking the error. So let’s put this in order again. What happens if we indent line 2 more? Let’s put three spaces in there now. I save the file.
11:42
I try to run it, and again the same error: unindent does not match any outer indentation level
.
11:51 Okay. Here, line 3 is marked again although our error is in line 2. The reason for this is that Python only recognizes in line 3 that something went wrong. Let’s put it back in order.
12:05 Let’s save it and run it. And now it works again because the indentation level is consistent.
12:14
There is also a case where IDLE probably won’t complain. And this is when we take the print()
statement out of the function and put it in line 4, although this time we don’t indent it.
12:31
I put a line in between so we can see it better. So now, on line 5, it says print(loud_input)
, but it’s indented without any spaces, so it’s basically not indented, in comparison to the situation before where it was indented just like the function body with four spaces.
12:50
Let’s see what happens now. I save the file and run it, and now we get a NameError: name 'loud_input' is not defined
. That’s because loud_input
right here is actually really not known to Python because that’s the variable that we are having inside of the function, but outside it’s not known.
13:12
So you have to be careful where you put your statements and references to variables inside of a function body. Again, you have to indent it correctly and also take good care about where in the order of the function body you put your function calls or statements. Again, return
should always be at the end of your function. Okay, let’s clean the code up a little bit so we don’t have so many blank lines sitting around here.
13:45
I save the file just to be sure. Let me run it. It works. It prints HELLO
in uppercase letters on the left side. That’s fine.
13:54 And we are almost finished with our own function. I may not point out the indentation level every time in the next lessons, so be careful at which position your cursor is when you’re programming and when you’re following along. All right. And that’s it for now, but your very own function is missing one last detail.
14:17 You wonder what it is? Well, you will find out in the next lesson.
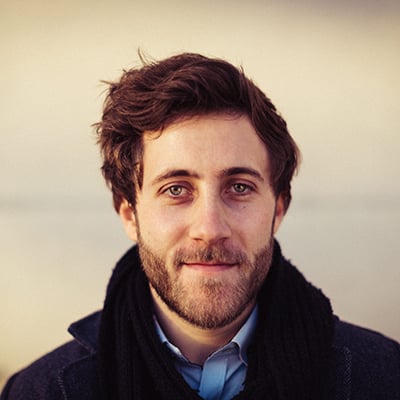
Philipp Acsany RP Team on Oct. 5, 2022
@aholt That’s interesting!
In the video, I’m using the IDLE shell. So some of the output is displayed because of the shell behaviour. When you save the code as a script, you won’t see any output, unless you’re calling print.
So, my_return = shout_and_return(“hello”)
will show HELLO
as long as you have an active print call inside of shout_and_return()
. Once you comment the print call out, you’ll see indeed nothing.
Only when you actively use print(my_return)
, then you’ll see the calue of my_return
, which is HELLO
.
Realblogger on Aug. 25, 2023
Why didn’t the function return two HELLO
strings as output from the editor: one from the print()
function and the other from the return
statement?
At 08:19
Can you please clarify?
Thanks
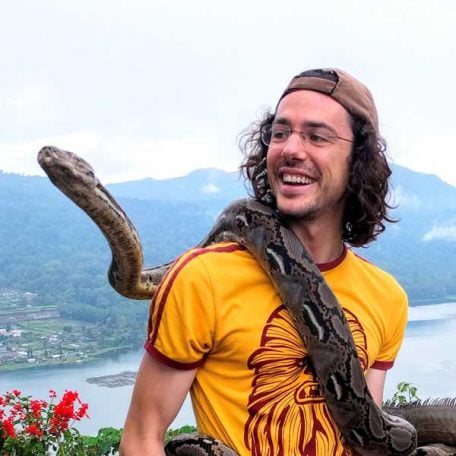
Martin Breuss RP Team on Aug. 29, 2023
@Realblogger the return
statement doesn’t display any output, it only hands a value back from within the function’s scope.
You’ll only see a return value displayed in your terminal if you wrap your function call into a call to print()
.
Can you change the code displayed in the lesson so that it’ll print HELLO
twice?
Become a Member to join the conversation.
aholt on Sept. 30, 2022
HI. i’m a bit confused on the return function. I’m using vscode and the inbuilt terminal to code. When i use your code as an example and then run the program in terminal, i only see the print function returning the uppercase word. I can prove this by commenting out the print function, running the program and seeing nothing returned.
However, when i assign the function to a variable eg my_return = shout_and_return(“hello”), and then add print(my_return), it does print in fact print the return function as well as the print function. I’m just wondering why i don’t see on the first part?