Do's and Dont's: Python Programming Recommendations
This lesson covers more recommendations provided by PEP8 to preserve consistency and remove ambiguity.
By the end of the lesson, you’ll know how to check:
- If a Boolean is
True
orFalse
- Whether a list is empty
- Whether or not a variable has a defined value
- That arguments are not None
- Suffixes and prefixes in lists
00:00 By now, you should be familiar with some styling paradigms to make your code more readable per PEP 8. A lot of this has been pretty dry, so in this video, you’ll have some fun by learning a couple preferred ways to do things in Python.
00:13
You can think of these as little Python tricks that you can start using in your code right away to make your code look much better. So first, don’t compare Boolean values to True
or False
using an equivalence operator. You may think that something like this is pretty clear to read.
00:42
And you’d be right. It is pretty clear to read. However, because Booleans take a value of True
or False
, you can instead just omit that and the interpreter will read this the same way.
00:55
If you’re naming your variables correctly, this can read especially well. Let’s just say something like greater_than
and greater_than
.
01:05
So now, if greater_than:
return this, and you can tell that this is greater than. In a similar vein, you can take advantage of empty lists evaluating as falsy in an if
statement.
01:19
So let’s say you have something like my_list
and you want to say if not len(my_list):
print('List is empty!')
.
01:32
Since an (empty) list evaluates as falsy, you can just say if not my_list:
and this will evaluate the same way as before. Falsy or truthy just means that a value evaluates as True
or False
while not explicitly being set to True
or False
.
01:48
Another thing with Booleans that may not be exactly clear is if you want to check if a variable has been set to a value. You may think that you want to do something like if not x is None:
return 'x is set to a value!'
.
02:07
So, if x
has been set to a value, it will not be None
, so this will evaluate as False
, and if not False
—this should be True
.
02:15
But you can actually make this a little more readable and just say if x is not None:
and the interpreter will evaluate this the same way, just in a more readable format for a human. Speaking of not None
, None
is a common default argument for function parameters.
02:35
Let’s say there’s something like function()
and param
is set default to None
. Now if you want to see if that param
variable has a value, you may think that something like this is a good idea,
02:55
but this is assuming that not None
and truthy are equivalent. In most cases they are, but if you remember up here, we said that an empty list is falsy.
03:05
So if you passed an empty list into this function, even though there’s a param
variable set, this would evaluate as False
and this block of code would not run. Instead, you should just check that it is not None
, like so. Finally, you can use the .startswith()
and .endswith()
method to check for what strings start with and end with.
03:31
If you wanted to check if a word started with 'cat'
, you may initially think to do list slicing to do so.
03:45
And this works, because 'cat'
has three characters and this will pull the first 3
characters. But strings have a .startswith()
method that you could use instead.
04:00
This method will evaluate to True
or False
and does the same thing while being a lot more readable. With list slicing, somebody reading your code would have to count how many letters are in what it’s trying to equal, and if you’re looking for really long words, that can be a little tricky to do.
04:16
This becomes extra helpful with the .endswith()
, if you’re looking for certain file formats, like a JPG.
04:29
Now you should be familiar with a couple of little tricks that you can use to make your code a little bit more readable and show that you’re a little more experienced with the language. In fact, checking if Boolean values are equal to True
or False
using an equivalence operator is often used as a quick way to gauge how comfortable somebody is with Python. So just by doing this you can appear a bit more experienced. And that’s it!
04:57 You’re done learning the basics of styling per PEP 8. We’ll finish up in the last video by showing you a couple tools you can use in your editor to make following PEP 8 a breeze. Thanks for watching.
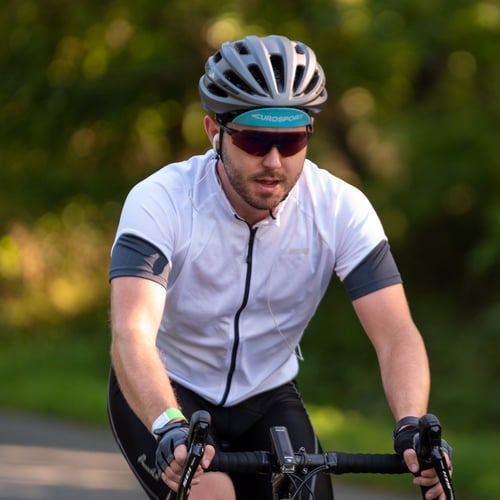
Joe Tatusko RP Team on Dec. 9, 2019
You’re welcome! Returning values can be useful as sometimes you don’t want to print the result to the console, and it also allows you to use the result of a function later on in your code. Let me know if that helps!
Alain Rouleau on Aug. 11, 2020
Really enjoyed this video. Good tips and advice, thanks.
Become a Member to join the conversation.
victorariasvanegas on Dec. 1, 2019
I don’t understand because you are using the return keyword instead of using the print function, thanks for the tutorial.