Because Python f-Strings are evaluated at runtime, you can put any valid expression into them. You’ll learn how to use them to do pretty nifty things such as evaluating expressions, calling functions and even calling methods from them.
Embedding Arbitrary Expressions in F-Strings
00:00 Now that I’ve shown you the simple syntax for f-strings, let’s talk about arbitrary expressions. f-strings are evaluated at runtime. That means that you can put any and all valid Python expressions inside of those curly braces. It lets you do some pretty neat things.
00:14 Let me show you some examples. Now that you’re back in the REPL, let me have you try a few arbitrary expressions. You could do simple math inside of your curly braces.
00:24
It will evaluate the expression. If you had a variable, like the few that we used before—like name
—you could do something with that also. You can also call functions.
00:37
I’ll have you make a very simple function, to_lowercase()
, that returns whatever input
we give it with the function .lower()
.
00:46
So, we already have our name
. Actually, let me have you spell the name out a little further. In our f-string, you can call the function, sending in the variable name
and complete the rest of your string also.
01:01
If you wanted to, you could call methods on strings directly inside there, or methods on anything. Let’s say name.upper()
.
01:18 Let me have you do something a little more advanced.
01:23
For this, I’ll have you create a class. The class will be Comedian
. When you initialize, you’ll set the attributes .first_name
, .last_name
and .age
. And just below, we’ll set the .first_name
to first_name
, .last_name
to last_name
, and same for .age
. The interesting part here is the representations that we will make for when we reference our class.
01:49
These two double underscore (__
), or dunder, methods for .__str__()
will print out the .first_name
and the .last_name
, with a space between, is this .age
, basically printing out the comedian’s name and their age as a string, explaining who they are. In this case, a human-readable version. For the representation one, we will do f"Comedian"
and then inside of parentheses, we’ll put in all of these parameters, f"({self.first_name}, {self.last_name}, {self.age})"
. What I want to show you is—so if I hit Return here—now make a new object, new_comedian
, and enter in the attributes for .first_name
, "Erik"
, and .last_name
, and .age
.
02:41 Now that you’ve done that, what if you use an f-string to print
02:47
our object, new_comedian
? Which method will it use to print that out?
02:54
It used .__str__()
. If we were to type in new_comedian
here,
03:01
in that particular case it will do .__repr__()
. What if we wanted the f-string to use the .__repr__()
version? With the f-string, you actually would need to invoke that by using the conversion flag !r
inside of the parentheses for our expression.
03:21
So when that is done, it will use the .__repr__()
version for Comedian
. The .__str__()
version is the informal string representation for the object—again, being human-readable. And the representation here is more of how could you recreate this. Just for you to remember, when using f-strings is that it will use the .__str__()
by default. For more information about creating classes or working with those dunder methods of .__str__()
and .__repr__()
, check out other articles on realpython.com. Next, I’ll take you through multiline f-strings.
Perryg on July 24, 2020
This is excellent.
Very clear and concise.
Thank you!
P Moz on March 6, 2023
Instead of f”{new_comedian!r}” could you use f”{repr(new_comedian)}”?
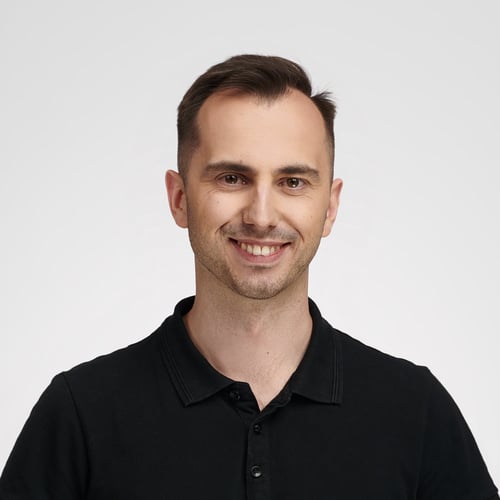
Bartosz Zaczyński RP Team on March 8, 2023
@P Moz Both expressions would produce the same result, so yes, you can call repr()
, but using the formatting syntax !r
looks more compact.
Become a Member to join the conversation.
Andras Novoszath on March 16, 2019
Lol, this is ridiculously good! :)