In this lesson, you’ll learn how to write comments in Python. You’ll see that comments are made by putting a “#” symbol before a desired comment. The lesson will also show you how to spread comments over multiple lines as well as how to write comments quickly in your editor using shortcuts,
How to Write Comments in Python
00:00 Welcome back! In this video, we’ll show you how to write comments in Python. Let’s get to it. Let’s write our first comment.
00:10
As mentioned earlier, programming languages have syntax to specify blocks of text to be treated as comments. In Python’s case, a hash symbol (#
) is part of that syntax.
00:23 All texts after the hash symbol through the end of the line is treated as a comment, whether it’s the entire line or just the ending portion.
00:36 For a word about style, PEP 8 advises keeping each line of Python at 79 or fewer characters, while the recommendation for comments is a maximum of 72. Therefore, comments need to be short and sweet.
00:51
If you need extra room to explain your code, you might consider a multiline comment. Python does not have native start and end symbols for multiline comments like other languages do. For example, in this C++ program, we can see that the /*
and the */
allow us to create multiple lines of comments between them.
01:16 So if Python does not provide multiline comment syntax, how do we create comments that might span multiple lines? Well, there are two ways. The first way might be obvious, but it’s to start each line of a multiline comment with a hash symbol.
01:35
Now let’s talk about the second way. The second way might look familiar to you, and that is to surround your multiline comment with triple quotes ("""
).
01:48 “But wait!” you say. “That’s a Python string!” And you’d be correct. This string may not technically be a comment, but it can serve the purpose of one. As a string that’s not stored or referenced by your program, it will be ignored at runtime and won’t appear in the bytecode.
02:05 But there’s a catch. This type of multiline comment, if it’s positioned in your program at certain places—let’s say, as the beginning lines of a class, module, or function declaration—will be treated as a docstring and be associated with that particular object.
02:24
Docstrings are covered more in-depth in another Real Python article, but I’ll quickly show you what I mean. Here’s a triple-quoted comment that’s positioned immediately beneath a function declaration of factorial()
.
02:37
As a result, this string will be read as a docstring and be associated with the factorial()
function. We can see the effect of this by passing the factorial
object to the help()
function. So yes, you will see comments that are surrounded by triple quotes, and that’s mostly okay. But just to be safe, a good rule of thumb may be to stick with the hash symbols unless creating docstrings intentionally.
03:07 Sometimes our coding editors help us out and provide us with some commenting shortcuts. Here are some examples. In some editors, I can hold down my Control, Command, or Option key while left-clicking.
03:21 This has the effect of giving me multiple cursors where I can type simultaneously.
03:29 Another neat trick is to select over lines of code or text and press Control or Command with / (front slash). This will prepend each line with the hash symbol, effectively commenting out that block.
03:44 Many editors will also provide you a way of collapsing your comments to make your code easier to read. The tricks we just covered are not universally adopted in all editors.
03:55 I’m using the latest version of PyCharm, provided by JetBrains. I know that these shortcuts also work in Visual Studio Code, from Microsoft. Now we know why we comment and how we comment. Coming up in the next video, we’ll learn some Dos and Don’ts to keep our code readable and our comments professional. See you there.
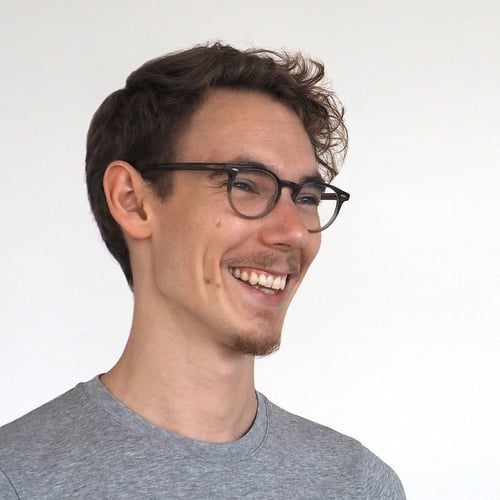
Dan Bader RP Team on March 13, 2019
@Rodrigo: According to PEP 257 (Docstring Conventions), the first variant ("""Text...
) is preferred. But personally I like the second variant better where each """
block is on its own line. It just looks better to me :-)
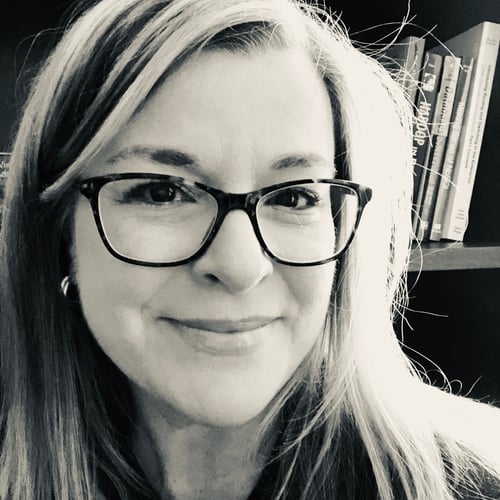
Jackie Wilson RP Team on March 14, 2019
I think the second variant looks better (in code) also. But then again, I prefer camelCase vs underscores.
victorariasvanegas on June 13, 2019
I think the first variant looks good.
Dri on Oct. 9, 2019
Agree, the first variant looks much better
Become a Member to join the conversation.
Rodrigo Vieira on March 13, 2019
Is the
more common than
I particularly prefer the second one. Have you poked around any big project’s source codes to see what is more common?