Mutable Data Structures: Lists and Dictionaries
In this lesson, you’ll see how you could approach this data set using mutable data structures, like lists and dictionaries. When you use mutable data structures, their contents can be modified. So if you use them to hold your data, then you run the risk of messing up your data set, since it can be changed.
If you want to have a multithreaded program and do parallel processing, then using immutable data structures would allow you to not have to worry about locking the data structures because there would be no way to update them.
00:00
When I started thinking about this tutorial, my first hunch was, “Okay, if I want to bring this into Python, I’d probably have a list of dictionaries.” So I could say, okay, scientists =
and then I would just have a list of dictionary objects—I should probably indent that—and I would put different keys, like 'name'
,
00:25 and I would put the respective field for the person,
00:31 and I would put the year they were born,
00:37 and then I would have some kind of flag that tells me whether or not they won the Nobel Prize. I would do something like that, right? And then just have a list of those.
00:49 And you can imagine that there would be some more here, so let me just copy and paste that. This is a little bit quicker. There will be more data here. And eventually, we close that list. Now, we have this data inside Python and we can work with that. This looks kind of nice, right?
01:11 We have individual items here. They’re represented by dictionaries, which is kind of neat. The only thing that I don’t really like about this is that, A, we’ve got a mutable
01:25 data structure here, so we have a list of these dictionaries—and by mutable,
01:33
I mean that I can just reach in here and I can say, “Okay, we’re going to grab the first scientist here.” I’m just going to reach inside this data structure, and I’m going to rename Ada, give her a different name, right? And now when I print this out, we actually modified the 'name'
of the scientist and it kind of screws up the data. In the beginning, I said, you know, one of the core tenants of functional programming is actually that you mainly work—or ideally, always work with immutable data structure.
02:11 You want a data structure that can’t be modified that way because if you represent your data using immutable data structures, it has some really interesting properties. For example, if you wanted to have a multithreaded program—you want to do some parallel processing there—you wouldn’t have to worry about locking the data structure because there was no way to update it. You know, all of these reads can happen in parallel, and we’d never have to worry about changing the state of this data structure while another thread is accessing it.
02:38 So, that would be one advantage of not allowing mutability here. What it leads
02:45 to if you use immutable data structures that cannot be modified, like this—So, this example here, this was a mutable data structure that I could modify at will, you know, at any time. With an immutable data structure, I couldn’t do that.
02:58 And that, a lot of times, leads to a cleaner conceptual model. I’m forced to do my calculations in a different way and it’s very easy, also, to come up with a history of the calculations that I do, you know, because I can’t just reach in and modify this existing object, but instead what I’d have to do is I’d have to create a full copy of this data structure that I can modify.
03:22 And so now, there exist two copies that I can use in some kind of history, and I could trace back, you know, that I made these changes, here. And of course, all of that takes up more memory, at least if you do it in Python. In other programming languages, they have better immutable data structures.
03:36 But the gist of it is that in a more functional programming style, you’re trying to go after immutable data structures and then make them, like, the core parts of your program.
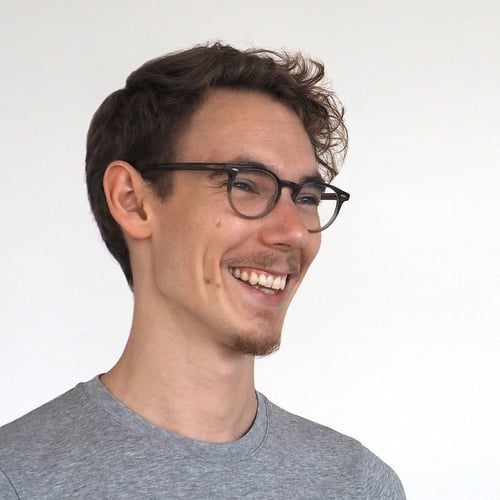
Dan Bader RP Team on July 24, 2019
@senatoduro8: Cheers, I just added the code sample to the end of section 1 :)
senatoduro8 on July 25, 2019
Thanks Dan. This tutorial is great! It did help me learn new was to use the map, reduce and apply functions creatively.
Pygator on Jan. 18, 2020
I thought you were going to reach in and change the name to ‘Dan Bader’ :)
molecule61 on April 19, 2020
The reason you want to use immutable data structures is not that “you run the risk of messing up your data set, since it can be changed”, but that it lets you write code that works through side effects. Also, it’s hazardous to pass mutable data structures to other parts of your program, since changes made through one reference to that data can then affect otherwise unrelated parts of your code. I’m sure you can communicate those reasons better than I can. But, one way or the other, accidentally “messing up” your data is just a wierd thing to suggest people worry about.
jameslangbein on May 7, 2020
Actually, Tom, “accidentally messing up your data” is not a weird thing to suggest people worry about. It’s very easy to cause unintended consequences in a complex system that uses mutable data structures. Once the code-base is large enough, it can be very difficult to keep a mental model of how a piece of data or object is changing as the system affects it.
That said, there can be very good intended consequences from using mutable data structures. For instance, if you have a master set of objects of the same class, and you want to work on a subset but have any changes reflected automatically in the master set, then a mutable structure is great.
I think the main thing to keep in mind is to be intentional when choosing a data structure, choose the right one for the right reasons. This can be difficult when just starting out though.
luckjanning on June 2, 2020
Hey!!! Wich editor are you using? I use VSC but it is way over my head & I just want something more simple.
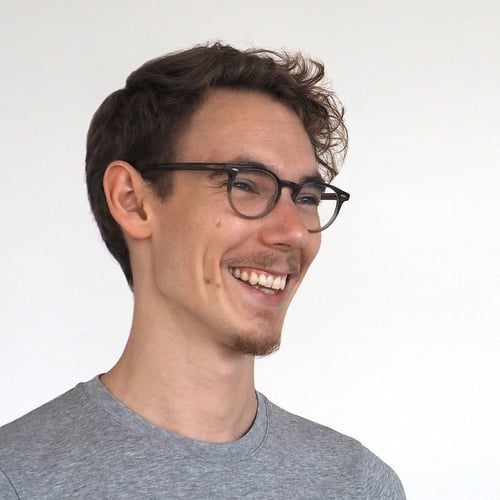
Dan Bader RP Team on June 2, 2020
I’m using an alternative Python REPL called bpython
in my videos. You can learn more about it here: bpython-interpreter.org. If bpython
is difficult to install I can also recommend ptpython.
confiq on July 3, 2020
pip install bpython
! I’ve replace the iPython!
Become a Member to join the conversation.
senatoduro8 on July 24, 2019
I wish the data used in this tutorials is provided like it is in the asyncio tutorials.