What Is the map() Function?
In this lesson, you’ll see that map()
is a built-in function that is one of the functional programming primitives (or building blocks) available in Python. It’s useful in a number of contexts.
map()
takes two arguments: an iterable (like a list) and a function. It then applies that function to all of the elements in the iterable. It outputs an iterator of items that are the result of the function called on the items in the first iterator.
00:00
So, again, that’s another built-in function that’s available in Python. You can just go map()
—I’m on Python 3, here. Python 2—some of the stuff might be slightly different.
00:11 You’re usually going to get a list object back instead of an iterable—or, an iterator. So, this is maybe something you’ll have to adjust for, but that shouldn’t be too hard. Let’s take a look at the docstring here.
00:22
The map()
function returns an iterator that computes the function we pass in—
00:30
this bit here, func
—[…] using arguments from each of the iterables
. So, basically, we’re passing in an iterable, like a list of something, and then map()
is going to apply our function on each item in this list and it’s going to create an output stream, or an output iterator of items that are the results of this function func
called on one of the items.
00:55 And we’re stopping once we’re done here. And actually, I think you can pass a number of iterables here and they will all kind of be chained together. We’re not going to be doing that in this tutorial. Okay.
01:05 So, I know this stuff sounds super confusing. The first time I read this, I was like, “Okay, I have no idea what this will be good for and when I would ever use this,” and yeah, I don’t know. It made me feel pretty stupid.
01:19 So what I’m going to do next is I’m going to try and make sure you get some real examples of how this would work, how this would be useful. So, I just wanted to show you this data set that we used in previous installments
01:34
of the series. We have this data set of scientists, these are all namedtuple
objects so that they’re immutable. The whole thing is actually stored inside a tuple so that the whole structure is immutable. That’s why I didn’t use a plain list, for example.
01:51 And by immutable, it just means, you know, we can’t reach in and modify these objects. If we wanted to modify one, we’d have to make a copy, and that way we can trace these changes and we can make sure that this list is always going to be the same.
02:04 So, immutability is kind of not having these side effects. It’s kind of a big part of functional programming. Yeah, so this is the dataset we’re working with.
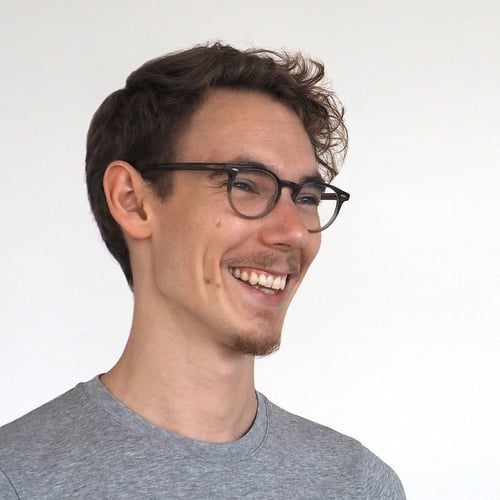
Dan Bader RP Team on Sept. 26, 2020
Check out the definition of map()
in Python 3:
map(function, iterable, ...)
Return an iterator that applies function to every item of iterable, yielding the results. If additional iterable arguments are passed, function must take that many arguments and is applied to the items from all iterables in parallel. With multiple iterables, the iterator stops when the shortest iterable is exhausted. (Source)
It means that you can pass more than one iterable to map()
and in each execution step, map()
takes the next element from each iterable and then calls the map function on them.
When one of the iterables has fewer elements than the others, map()
will stop “mapping” as soon as the shortest/smallest iterable is exhausted. (“exhausted” meaning that all of its elements have been processed.)
Probably easier to see what I mean in an example:
>>> iterable1 = ["a", "b", "c"]
>>> iterable2 = ["1", "2"]
>>> list(map(lambda a, b: a + b, iterable1, iterable2))
['a1', 'b2']
As you can see, the end result of the map()
call in the example above is ['a1', 'b2']
and no exception was raised, even though iterable2
contained fewer elements than iterable1
.
I hope that clears it up :) Also check out our dedicated tutorial on Python’s map()
function.
samhassan1010 on June 20, 2021
Please how different is the map() from list comprehension
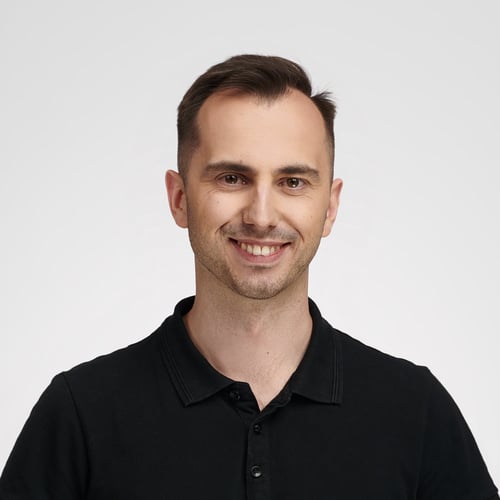
Bartosz Zaczyński RP Team on June 21, 2021
@samhassan1010 Check out the tutorial about map()
, which compares both syntax constructs. In a nutshell, map()
returns a generator that can be evaluated lazily, while a list comprehension returns a list. Other than that, map()
expects a callable as an argument, whereas a list comprehension accepts a Python expression.
Become a Member to join the conversation.
Arif Zuhairi on Sept. 25, 2020
What does it means with it stops when the shortest iterable is exhausted?