Work With Lists of Numbers
00:00 In this lesson, you’ll learn some Python tools to work with lists of numbers.
00:06
It’s not uncommon that you’ll have to work with a list of numbers. For example, here we have our numbers
list again. This time it goes all the way up to five, so it contains the integers from one through five.
00:16
And you may want to sum up all of the values that are inside of this list. Now you know that lists are iterable, so you can do this using a for
loop.
00:25
You could define a variable outside of the loop that’s going to hold the total. So I’m going to say total = 0
to initialize the variable, and then start a for
loop where I’m going to say for number in numbers
00:40
and then add them to the total. So I will say total = total + number
.
00:49
And then at the end, I can print out total
and you’ll see that it summed up all of the numbers that are inside of the numbers
list.
00:55 This is a way you can do it, but Python has some tools that make operations like this one faster and easier because you can perform some mathematical operations on collections using built-in functions.
01:08
For example, you have the sum()
function, so that does exactly what you expect. It sums up all the items inside of a collection. So I can say sum()
and pass it numbers
as an argument.
01:22
And now if I press Enter, you can see I get the exact same result, 15
again, because the sum()
function summed up all the integers that are inside of the numbers
list.
01:32 So one plus two plus three plus four plus five, and that comes up to 15.
01:41
There’s also a min()
and the max()
function that do what you’d probably also expect them to do. I think they’re very descriptively named.
01:51
Let’s try them out in IDLE. So if you say min()
and pass it a collection, you’ll get the minimum value in the collection. In the numbers
list, that minimum value is 1
.
02:02
And if you type max()
, open up parentheses and then type in numbers
, close them, press Enter, then you get 5
because that’s the maximum value inside of the numbers
list.
02:13
If we would add something else, let’s say I would say numbers.insert()
and at index two, I’m going to insert 100
,
02:26
and then if I run max()
again on the numbers
list, I’ll get 100
as the output because now 100
is the highest value inside of my numbers
list.
02:37
So sum()
, min()
, and max()
are some useful built-in functions that you can pass a collection to. And then you can perform some common mathematical operations with them.
02:49 And notice that I said collections, so it doesn’t have to be a list. You can do the same thing also with a tuple if you want. You can go ahead and try that out.
02:57 It works exactly the same way as it does with lists.
03:03 In this lesson, you’ve learned how you can work with lists of numbers using some common built-in Python functions. In the next lesson, you’ll look at something quite powerful and interesting called list comprehensions.
03:15 You may have seen those around or heard about them. In the next lesson, we’ll uncover together what list comprehensions are and how we can use them in Python.
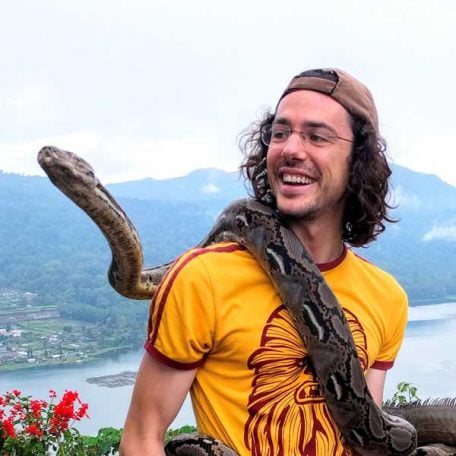
Martin Breuss RP Team on July 5, 2024
@ajackson54 emojis are string characters, so you can enter them like you’d add any other string:
>>> my_mood = "😁"
>>> my_mood
'😁'
You can revisit the course on strings and string methods if you want to learn more.
Become a Member to join the conversation.
ajackson54 on July 4, 2024
How do you enter emojis in your code?