Preparing Your Package for Publication on PyPI
After implementing your packages functionalities, you’ll now add additional files, which are needed in order to publish your package to PyPI.
The setup.py
file should be placed in the top folder of your package. A fairly minimal setup.py
for reader looks like this:
import pathlib
from setuptools import setup
# The directory containing this file
HERE = pathlib.Path(__file__).parent
# The text of the README file
README = (HERE / "README.md").read_text()
# This call to setup() does all the work
setup(
name="realpython-reader",
version="1.0.0",
description="Read the latest Real Python tutorials",
long_description=README,
long_description_content_type="text/markdown",
url="https://github.com/realpython/reader",
author="Real Python",
author_email="YOUR_EMAIL_ADDRESS_HERE",
license="MIT",
classifiers=[
"License :: OSI Approved :: MIT License",
"Programming Language :: Python :: 3",
"Programming Language :: Python :: 3.7",
],
packages=["reader"],
include_package_data=True,
install_requires=["feedparser", "html2text"],
entry_points={
"console_scripts": [
"realpython=reader.__main__:main",
]
},
)
00:00 Now the code part of the package is ready to upload, but there are a couple more steps before you can share your project with the world. First is coming up with a name. Great names are short and memorable, but there’s a chance that they’re already taken.
00:13
Most of the time, coming up with a name is harder than writing the actual code. For this example I’m going to use a format that is <username>-reader
, so in my case it would be joetats-reader
.
00:25
This ensures that I won’t have any name collision when I’m uploading. In addition, you can have a different name on PyPI as what will actually be used to import the package. For the realpython-reader
, you pip install realpython-reader
, but you import reader
by itself.
00:41
Try to keep these names the same or very similar so that it’s easier for your users to follow along. To clean things up, go ahead and make a new folder in your top directory and call it reader
.
00:55 Now move all the files you made in the last video into there.
01:02
Your top directory should now just contain this reader/
folder. Go ahead, and in that top directory create a new file and call that setup.py
.
01:13 Copy and paste the code from below, and you should end up with something like this. Let’s take a look at what’s going on here.
01:21
The most important lines in this file are the name
, the version
, and the packages
. At a bare minimum, you need all three of these to send your project to PyPI.
01:32
The name
is how the project will show up on the website. So in my case, I’m going to replace realpython
with joetats
. For version
, PyPI will only allow one upload per version number, but we’ll talk about more of that later. In this case, just leave it at "1.0.0"
.
01:51
If you scroll down to packages
, you’ll see that only "reader"
is here, and this refers to the folder that you just made. While the rest of this isn’t necessary, it does help out a lot when describing your project.
02:04
These different fields will be used on PyPI to help categorize your project and make it easier to search for it. You can see that there’s a short description, there’s a long description—which refers to this README
, which is a file we haven’t added yet—and the description’s content type, which you’ll see is Markdown.
02:22
There’s some contact information about where the project’s located, how to get in contact with the author, license, and classifiers. So if you go over to PyPI, you can do a search for the actual project—so realpython-reader
.
02:42
So if you see on this page, a lot of those fields that you fill out end up in here, and actually everything on here is built out of these setup.py
and any README
file that you include.
02:54
So if you see, most of this README
down here is from the README
file that you haven’t added yet.
03:01
But if you look over on the left side, you can start to see you’ve got your licensing, your author, maintainers, and any classifiers that are added. Let’s go back to the setup.py
and take a look at two more lines that are on here. There’s an install_requires
, and this includes the dependencies.
03:23
So if you look inside feed.py
, you can see that you need to import feedparser
and html2text
. So, any third-party packages or libraries that are needed would go in here. And then entry_points
will create a script to run whenever you execute your package.
03:41
So in this case, it’s going to make something that will execute main()
from that __main__.py
file inside reader/
. Awesome! So now your project’s configured to be able to be built, but it’s time to add a README
file so that you can get that full PyPI project page. Thanks for watching.
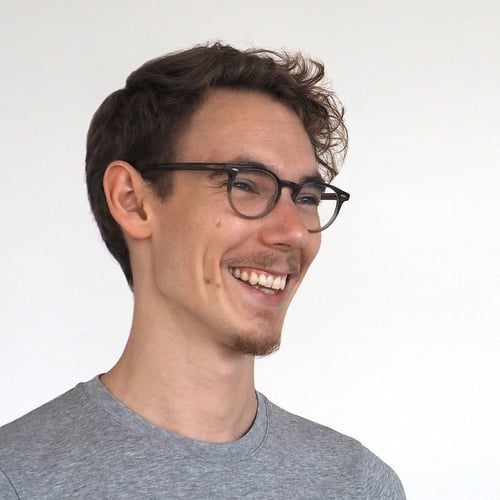
Dan Bader RP Team on May 31, 2020
Thanks, I’ve moved the setup.py
example to the video description to make it easier to find (previously it was only linked under “Supporting Materials”). Best, Dan
Become a Member to join the conversation.
rorydaulton on May 31, 2020
At time stamp 1:13 the video for this lesson “Preparing Your Package for Publication on PyPI” says, “Copy and paste the code from below”. The code was for
setup.py
. Could you add that code, along with any others, into the text below the video? (Similar code may need to be placed into other lessons in this series.)