Learn about Python naming conventions. In this lesson, you’ll see how to choose sensible names for Python objects such as variables, functions, modules and so on. You’ll also see what naming styles are compliant with PEP 8 and which one’s aren’t.
Python Naming Conventions
00:04
As you write Python, you need to name quite a few things like variables, functions, and the scripts themselves. It’s important to have a good system to naming so that you can refer to what you want without searching through code all the time. In this video, you’ll learn naming styles that are PEP 8 compliant, as well as some tips on how to choose useful names for things. Right off the bat, you should know to never use an l
or a capital O
or a capital I
as a single-letter name for anything. Here it almost looks like I’m trying to reassign the value of 0
to the value of 2
.
00:37 Even though the syntax highlighting in my editor makes this clear that that’s not the case, you can’t assume that somebody else will have the same syntax highlighting or even any syntax highlighting at all. Okay.
00:48
Let’s get to some naming styles. For functions, you want to use a lowercase word or words. So something like this is okay. And if you need to use multiple words, go ahead and separate them with an underscore (_
), like so. For variables, follow the same rules, except now you can use single letters if you choose.
01:08 If you’re going to use multiple words, you should still separate them out with underscores.
01:15 Constants are a little bit different, and in this case, you’re going to use all capital letters and separate them out with underscores, like before.
01:28 Using these all capital letters implies that this constant is going to remain constant and not change through the script. With classes, things get a little bit different.
01:40 Now you’re going to start each word with a capital letter,
01:45 and if you need to use multiple words, you’ll just put them right next to each other and separate them out by having a capital letter in the next word. There’s no underscores here.
01:55 If you add methods to your class, you’re going to treat them just like your functions and use your lowercase words that are separated out by underscores as needed.
02:05 When it comes time to save everything in a module, you’ll go ahead and use your lowercase words separated by an underscore. Make sure you’re using short words to keep things clean in the directory.
02:20 If you bundle everything up into a package, you’ll use short lowercase words, and if you need to use multiple words, you will not separate them with an underscore. So, there you go!
02:30 Those are the naming styles per PEP 8.
02:35 Now, these are great, but they still don’t help you choose the names for your variables and functions, so let’s take a look at that. I’m just going to delete all of this.
02:45
Single letter variable names are fine if you’re using them for mathematical functions, because that makes sense. What doesn’t make sense is using them for something like a name, because if I have something like x = 'John Smith'
03:02
and then I want to have a y
and a z
, which are equal to x
that’s split at the space, if I go to look at this, it might not make any sense.
03:15
Instead, let’s put some care into the name of this. And because this is a name, call it name
.
03:22
Now, you can call .split()
on name
and you probably have a pretty good idea that this is going to be the first_name
, and this’ll be the last_name
.
03:34 Now if you have to refer to a first name and a last name later on in the script, you’ve got a pretty good idea of where they are. Additionally, you may want to shorten the names of your functions so that you don’t have to type them out all the time. Let’s do something like this.
03:51
And all this function is going to do is double. I know that db()
means double, but maybe somebody else doesn’t. So instead of using db()
, maybe you should make this function something like multiply_by_two()
, because that’s what it’s doing. Now if you see later on in the script something that’s like multiply_by_two()
, you’ve got a pretty good idea of what that function is doing.
04:19 The keys when naming things in Python are to follow a style so that you stay organized, and to use meaningful names. Don’t be afraid to type a little more because it may save you quite a few headaches later on. That’s it for now! In the next video, you’ll learn how to lay out your code effectively. Thanks for watching.
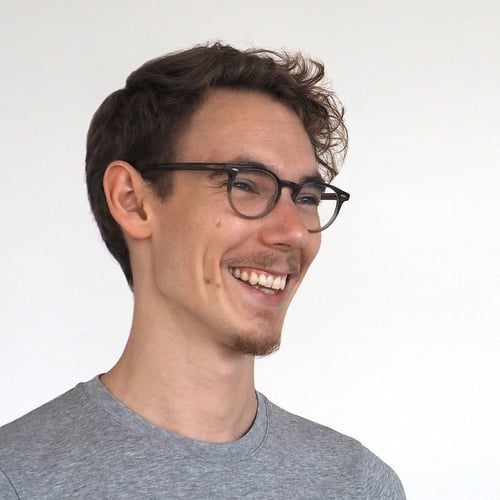
Dan Bader RP Team on March 21, 2020
For function and method arguments PEP 8 recommends using lowercase words connected by single underscores. For example:
class MyClass:
pass
def something(my_class):
# my_class is an instance of MyClass
pass
Become a Member to join the conversation.
David Y D on March 20, 2020
This was very clear, thank you! I have 1 question. You said classes should be named
FirstLetterOfEachWordCapital
, but what do you name a class being passed into a function (def somthing(MyClass):
ordef somthing(my_class):
)?