Design and Guidance: OOP in Python (Summary)
In this course, you’ve learned when to use classes in your code. This knowledge is just as important as knowing how to write a class with attributes and methods. You’ve even learned about alternatives to inheritance.
SOLID is an acronym for five principles that you should use when thinking about object-oriented code. After this course, you’re well versed in the principles:
- The Single-Responsibility Principle (SRP)
- The Open-Closed Principle (OCP)
- The Liskov Substitution Principle (LSP)
- The Interface Segregation Principle (ISP)
- The Dependency Inversion Principle (DIP)
This course is the third in a three-part series. Part one is an introduction to class syntax, where you learn how to write a class and use its attributes and methods. Part two is about inheritance and class internals.
To learn more about the concepts from this course, check out:
- Python Descriptors: An Introduction
- Build Enumerations of Constants with Python’s
Enum
- Metaclasses in Python
- Callables: Python’s “functions” are sometimes classes
- SOLID on Wikipedia
- The SOLID Principles, Explained with Motivational Posters
Congratulations, you made it to the end of the course! What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment in the discussion section and let us know.
00:00 In the previous lesson, I covered the dependency inversion principle. This last lesson of all three parts summarizes this part of the course and points you at more materials if you want to dig deeper.
00:12 This three-part course started with an introduction to the syntax used for classes in Python and has led all the way to principles governing good object-oriented design. Since Python has a mixed language style, you can choose whether or not to write classes. As you don’t have to artificially wrap all code in a class like some stricter languages, you’re free to let the project guide your decision.
00:35
If you’re finding you have data that naturally groups together and there are operations that interact only with that data, a class might be a good fit. For smaller programs or smaller pieces of data, a dictionary or namedtuple
might be enough.
00:49 A good compromise between the two is a data class, if you prefer. To help guide your object-oriented design, the mnemonic SOLID covers five principles. The single-responsibility principle is it should do only one thing.
01:05 The open-closed principle states you should be able to extend an object without mucking with its internals. The Liskov substitution principle is a harder way of saying duck typing.
01:17 Interface segregation principle states you shouldn’t put stuff in an object that won’t be used. And the dependency inversion principle says interacting objects should use an interface between them. Both part one and part two of this course touched on the descriptor protocol.
01:36
If you wish to dive deeper into descriptors, this article might be your thing. Part two of the course talked about Python’s Enum
. If you’d like to learn more about it, this article can show you some details.
01:49 If you’re not tired of the bad puns yet and you’re willing to dive into the deepest parts of how classes are built and how you can abuse that system, the metaclass course will blow your mind. Python is a crazy, crazy language sometimes.
02:05 In part two of the course, I talked about how many things in the standard library that look like functions actually are callable. If you wish to visit that some more.
02:14 This article from Trey Hunter talks about just that.
02:19 To dig further into SOLID, start with the Wikipedia article. It links to a separate piece for each principle and also links to the original paper. And finally, for little fun, how about the SOLID principles explained with motivational posters?
02:35 I like the one with the duck.
02:39 It’s been quite a three-part journey. I hope you found value here. Thanks for your attention.
mikesult on Oct. 4, 2023
Great three part series, I’m glad you covered the topic of OOP so thoroughly. I loved your warning of potential coding errors with the expression “massive foot gun”. It mades me laugh. Your deadpan humor is greatly appreciated. Thanks for the study of the SOLID principles, with great example code to illustrate the ideas. I appreciate the deep dive into the inner workings of classes and the base object in python.
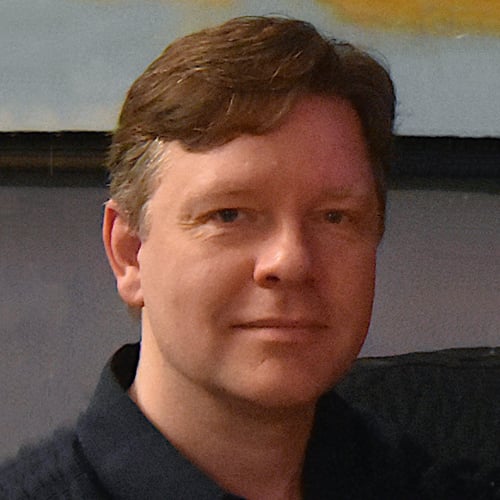
Christopher Trudeau RP Team on Oct. 4, 2023
Hi Mike and Alnah,
Glad you enjoyed it!
Alireza637 on Oct. 10, 2023
Good amount of humor and really good explanations, Thanks Christopher
Become a Member to join the conversation.
alnah on Oct. 2, 2023
Another great course on OOP! Thanks!