Working With my_enum()
00:00
Now that you’ve built your own my_enum()
function, it’s time to put it in use.
00:07 So just a few words about the setup right here. I’m still on VS Code, but in this lesson, you will work in the terminal. You can use any terminal you want. I’m using VS Code, and this has the advantage I can show you the file you just created in the last lesson above for reference. So one thing you have to make sure before you start, and that’s that you’re in the same folder that you created the files from the lessons before.
00:38
So to quickly show you what I mean by this, you have to make sure that the my_enum.py
file is in the same folder as you are working right now, because you want to import the my_enum
function from the my_enum.py
file in the Python interpreter. And that’s what we’re doing now.
01:04
So python3 -m bpython
, that’s the Python interpreter I’m using to test my syntax highlighting, but you can use the usual Python interpreter depending on your taste. So, as I just said, from my_enum
—that’s the file—import my_enum
.
01:26 This wouldn’t work if we would be in some different folder. So that’s why you have to make sure you start the interactive Python interpreter from the same folder.
01:38
So now that you’re inside of the interpreter and you imported my_enum
, it’s time to paste the seasons
variable again. That’s the list of Spring
, Summer
, Fall
, and Winter
.
01:51
And now let’s give the my_enum
function a first try. So, for val in my_enum
. There you see Python already suggests what you can use: the sequence, which is the seasons
variable, and start
.
02:13
You want to start with 1
.
02:17
print(val)
. So, val
in this case is the tuple count
and item
, or more specifically the seasons
value at this point in the for
loop.
02:31
So we run it. You see it prints the tuple, and the first part is the count, and the second part is the value of seasons
that is returned by my_enum()
at this point. Well, actually I should be a little bit more specific here.
02:51
I said it returns this item. Well, actually it yields this item. So what does this yielding mean? You can think about yielding as handing something out. So to show you what I mean by this, let’s repeat the for
loop again: for val in my_enum(seasons, start=1):
03:23
So every line is a handout from the for
loop. So as you can see, you can repeat this for
loop over and over again, and it will produce your expected output.
03:35
This works because you’re creating a generator object every time you run the loop. However, when you assign my_enum
to a variable, the behavior is a bit different because the yield
statement will literally hand out the tuples of your generator object until it’s empty, and there is nothing to hand out anymore.
03:55
When you then want to look over it again, it will be empty, and there is nothing to hand out. So let me show you. If you create a new variable called counted seasons
04:10
that equals my_enum()
, you pass in seasons
, let’s say the start
value is 1
, you can, instead of accessing the my_enum()
function here, you can access the variable, and there it might get even more clear what this yielding/handout means.
04:33
So for val in counted_seasons:
04:44
So looks the same. If you’re doing it again, though—for val in counted_seasons:
, and you’re printing val
,
04:57
nothing is returned. That’s because, basically, your my_enum()
function handed out everything that there was. So now counted_seasons
is empty, and there is nothing to return anymore.
05:10
So this is exactly the same that the enumerate()
function does. So let’s see if this is actually the case. So instead of my_enum()
function, you use the enumerate()
function, pass in
05:27
(seasons, start=1):
print(val)
—so exactly the same output as before. And if you’re creating the counted_seasons
variable, this time with the enumerate()
function,
05:56 again, you have the same output. So you loop through it again.
06:03
You see that it’s empty. So what you just created behaves exactly the same, like the enumerate()
function. Let’s explore this a little bit more.
06:14
So maybe you have heard about the next()
function. So the next()
function takes an iterator and the optional default
value.
06:25
This optional default
value, we’ll come to it in a second. But this iterator will be the counted_seasons
variable. However, currently our counted_season
’s variable is empty because all the tuples that were in there were yielded out, so you have to fill it up again.
06:43
So basically you can say counted_seasons = my_enum()
, pass in seasons
, pass in start
. Now we filled it up, and if you’re calling the next function now
07:01
with counted_seasons
, you always just get the next item back. That’s what your my_enum()
function yields at this point. So once this tuple is yielded, this my_enum()
generator object basically saves the state where it’s in right now and stops, until you touch it again.
07:26
That’s what you did in the for
loop. So basically you looped through it, as you touched the item every time it was yielded, and you do the same with next()
.
07:36
So the next()
function gets the next tuple yielded back—2 Summer
—and you can go through it until the end of the list. However, once there is nothing to yield back anymore, you will encounter StopIteration
error. That’s expected, and that’s why this next()
function contains this optional default
value. So if you don’t want to get back an error, you can say, well, if there is nothing in it anymore, then just return None
.
08:08
So now it returns None
. I have no idea why there was this little visual glitch here, but here you see there is no error anymore. So to recap, the my_enum()
function that you created and just imported here behaves exactly the same like the enumerate()
function that is built in in Python.
08:30
The count
variable counts up, and with each step you’ll yield back the tuple count
and item
, which is in this case, the seasons
string that you saw here.
08:44 So that’s all there is for now. In the next lesson, you will get a final summary of the course.
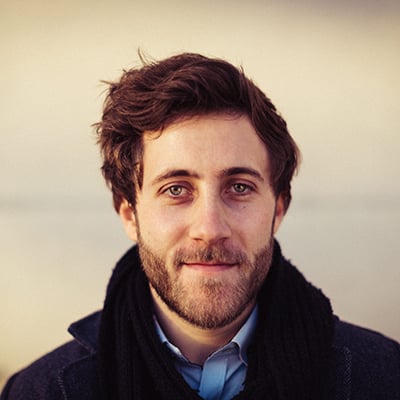
Philipp Acsany RP Team on Jan. 28, 2022
Hi Patrick, I know that feeling! 😅
Can you share your code with me so I can investigate?
Patrick on Feb. 3, 2022
# seek.py
def my_enum(sequence, start=0):
count = start
for item in sequence:
yield count, item
count += 1
-----
# main.py
seasons = ["Sad", "upset", "unhappy", "grey day today"]
for val in my_enum(seasons, start=1):
print(val)
circuit_board = ["Main Motherboard", "2 PCIe x1 Slots", "PCIe x16 for video", "CPU fan",
"3 standard PCI Slots", "Chipsets", "4 or more DIMM slots"]
for circuit in my_enum(circuit_board, start=1):
print(circuit)
break_it_down = ['Power supply', 'DVD drive', 'Power Cords', 'CPU',
'Floppy drive old school', 'Hard Drive', 'Motherboard']
for down in my_enum(break_it_down, start=1):
print(down)
Patrick on Feb. 3, 2022
I guess i was’n using the interpreter
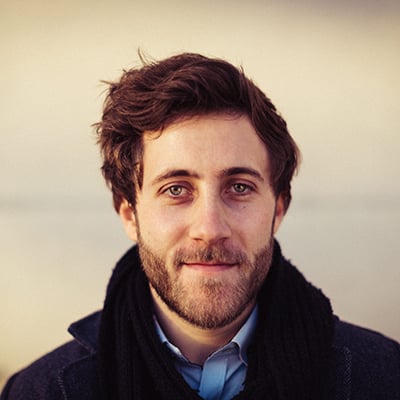
Philipp Acsany RP Team on Feb. 6, 2022
Ah, gotcha! So it works for you now, Patrick?
Become a Member to join the conversation.
Patrick on Jan. 28, 2022
03:35 This works because you’re creating a generator object every time you run the loop. However, when you assign my_enum to a variable, the behavior is a bit different because the yield statement will literally hand out the tuples of your generator object until it’s empty, and there is nothing to hand out anymore.
Yes but this did not work in pycharm this keeps handing out the same generated function even when i use a new variable. And i just hope you understand what i wrote cause i don’t know if i do lol