Danger Zone: Mixing Mutable and Immutable Data Structures
In this lesson, you’ll see that using a combination of mutable and immutable data structures can still lead to problems. Even if you use an immutable data structure like namedtuple
, you still run the risk of modifying your data set if you store your immutable data structures in mutables ones, like lists.
In the next lesson, you’ll see how to address this problem by replacing the rest of your mutables data structures with immutable ones.
00:00
So, what I’m going to do here is I’m going to say scientists =
and then a list, and some of you might be going, “Well, why is he using a list here?” We can talk about that in a minute.
00:13
Because now, so far, we solved one part of the problem, right? I’ve defined the scientists
list here. You can see here it prints kind of nicely.
00:23
We can improve that a little bit with the pprint
module, so I’m going to
00:30
import the pprint
module. That is like a pretty-printing module, it just gives us better-aligned items here. That’s a little bit easier to read. Okay.
00:43
Now, we’ve got the scientists
list, and while I can’t really go in and do this, what I did earlier—I guess now we’d have to restructure it a little bit because now this isn’t a key, this is actually a field. I can’t do that anymore, but what I can still do is I can do this and delete the first scientist,
01:11 right? And now poor Emmy is all by herself, and that’s a problem because when you look at what I did there, well, I was trying to define this immutable data structure, but what I actually did is I defined these immutable items and then stored them all in a list, which is a mutable data structure in Python.
felixthec on Feb. 6, 2020
As a side note it is also a risk if you have an mutable data structure inside of immutable once.
from pprint import pprint
scientists = tuple([
... {'name': 'Ada Lovelace', 'field': 'math', 'born': 1815, 'nobel': False},
... {'name': 'Emmy Noether', 'field': 'math', 'born': 1882, 'nobel': False},
... {'name': 'Marie Curie', 'field': 'physics', 'born': 1930, 'nobel': True},
... ])
del scientists[0]
Traceback (most recent call last):
File "<input>", line 1, in <module>
TypeError: 'tuple' object doesn't support item deletion
scientists[0]['name'] = 'Ed Lovelace'
pprint(scientists)
({'born': 1815, 'field': 'math', 'name': 'Ed Lovelace', 'nobel': False},
{'born': 1882, 'field': 'math', 'name': 'Emmy Noether', 'nobel': False},
{'born': 1930, 'field': 'physics', 'name': 'Marie Curie', 'nobel': True})
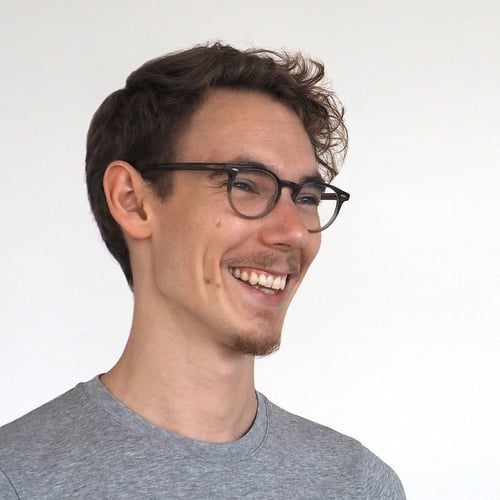
Dan Bader RP Team on Feb. 7, 2020
Good point @felixthec! Unfortunately there’s no immutable dictionary data type in the Python standard library, but something like Pysistence’s PDict
data type would work well here.
Thomas Wilson on March 27, 2020
Can you tell us what the repl is called you’re using in the courses?
jcandali on March 27, 2020
It’s bpython
Become a Member to join the conversation.
Abby Jones on July 24, 2019
If you pass the variables ada and emmy to the new list, you won’t need to use pprint because it prints out nicely.