Classic Data Structures and Algorithms
Learning Path ⋅ Skills: Python, Data Structures, Stacks, Queues, Linked Lists, Hash Tables, Enums, Search Algorithms
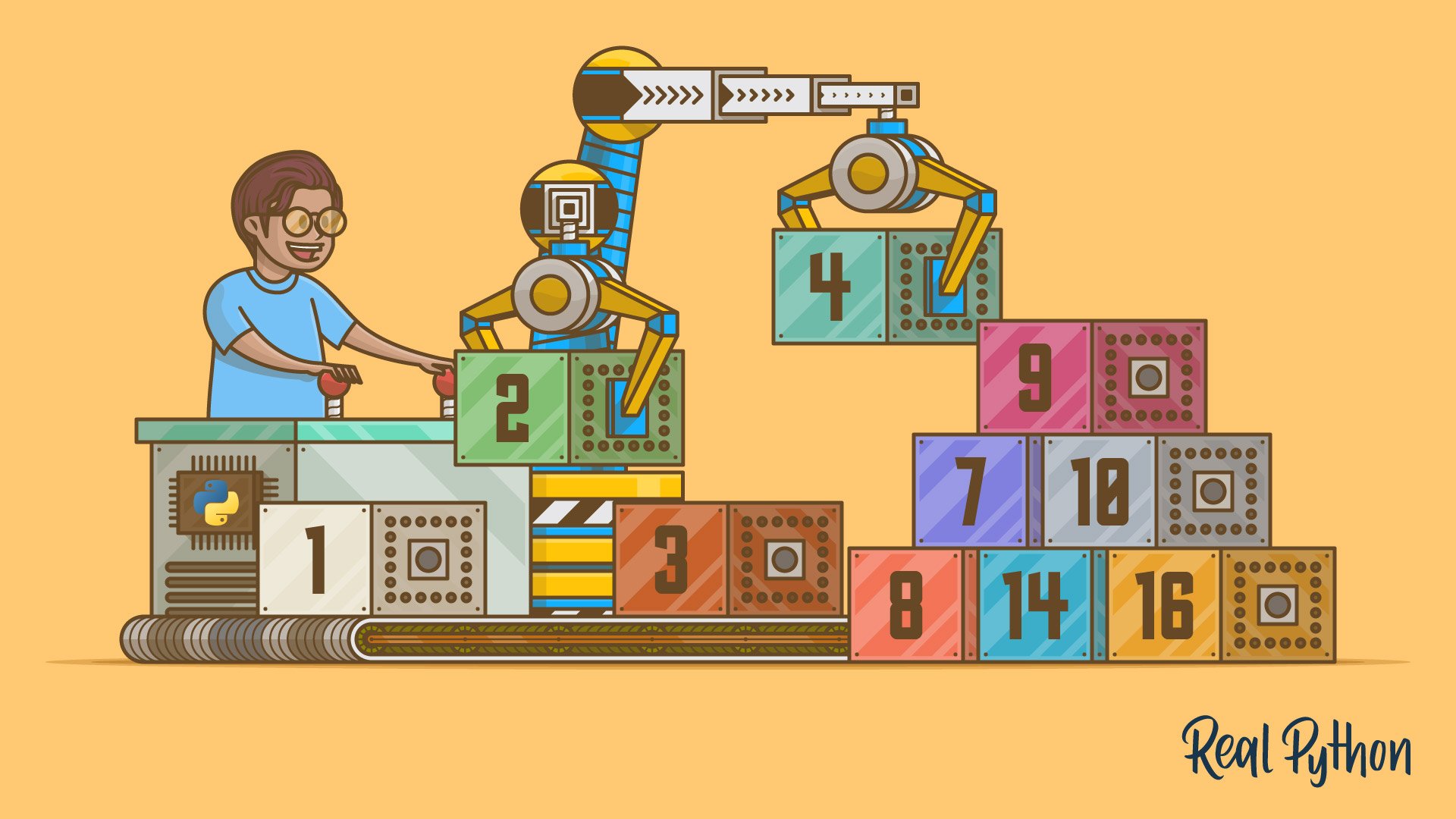
Explore essential data structures and algorithms in Python. Learn stacks, queues, linked lists, hash tables, and sorting techniques. Enhance your coding skills with practical examples and efficient solutions for real-world problems.
Classic Data Structures and Algorithms
Learning Path ⋅ 18 Resources
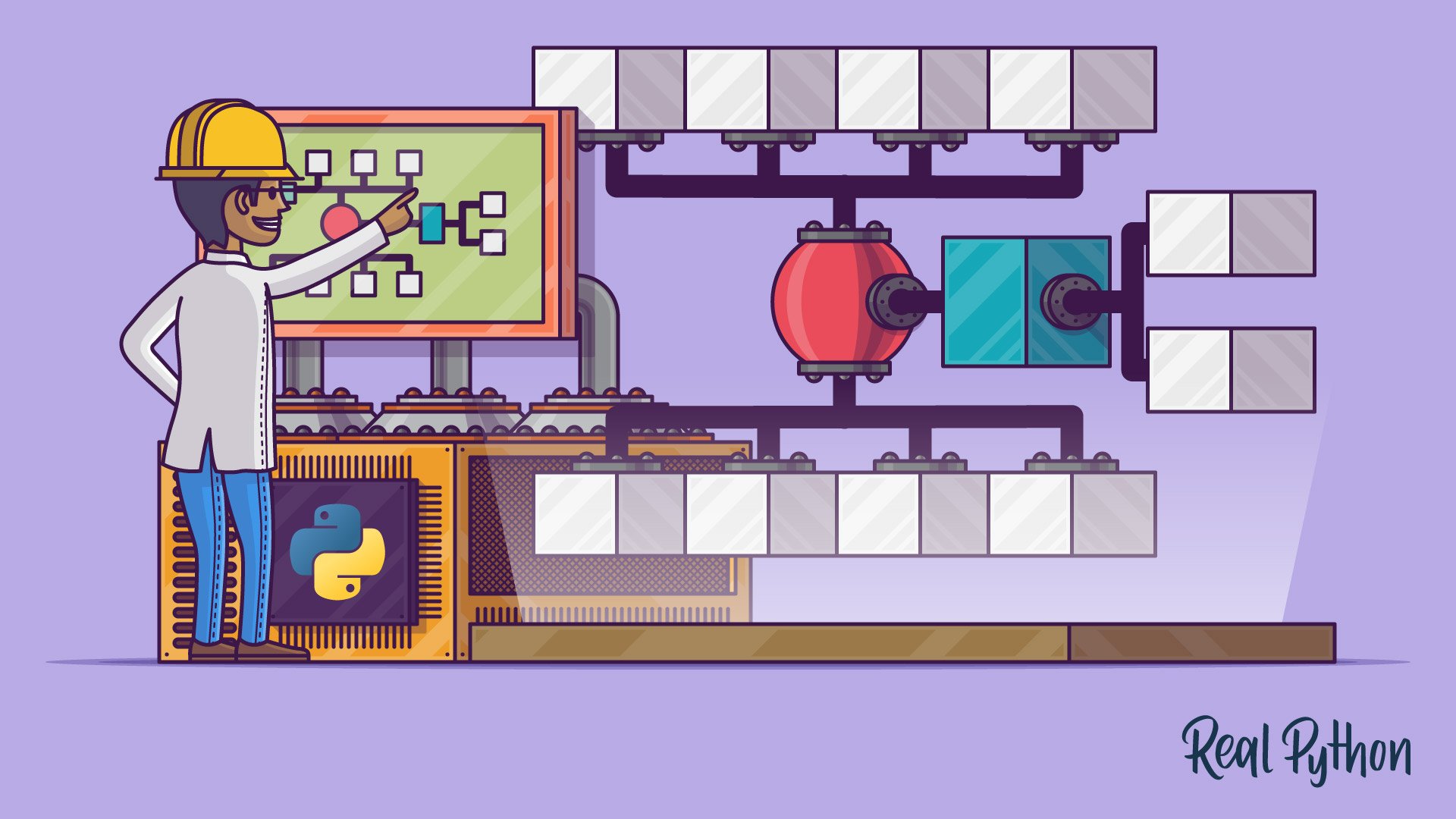
Course
Dictionaries and Arrays: Selecting the Ideal Data Structure
Learn about two of Python's data structures: dictionaries and arrays. You'll look at multiple types and classes for both of these and learn which implementations are best for your specific use cases.
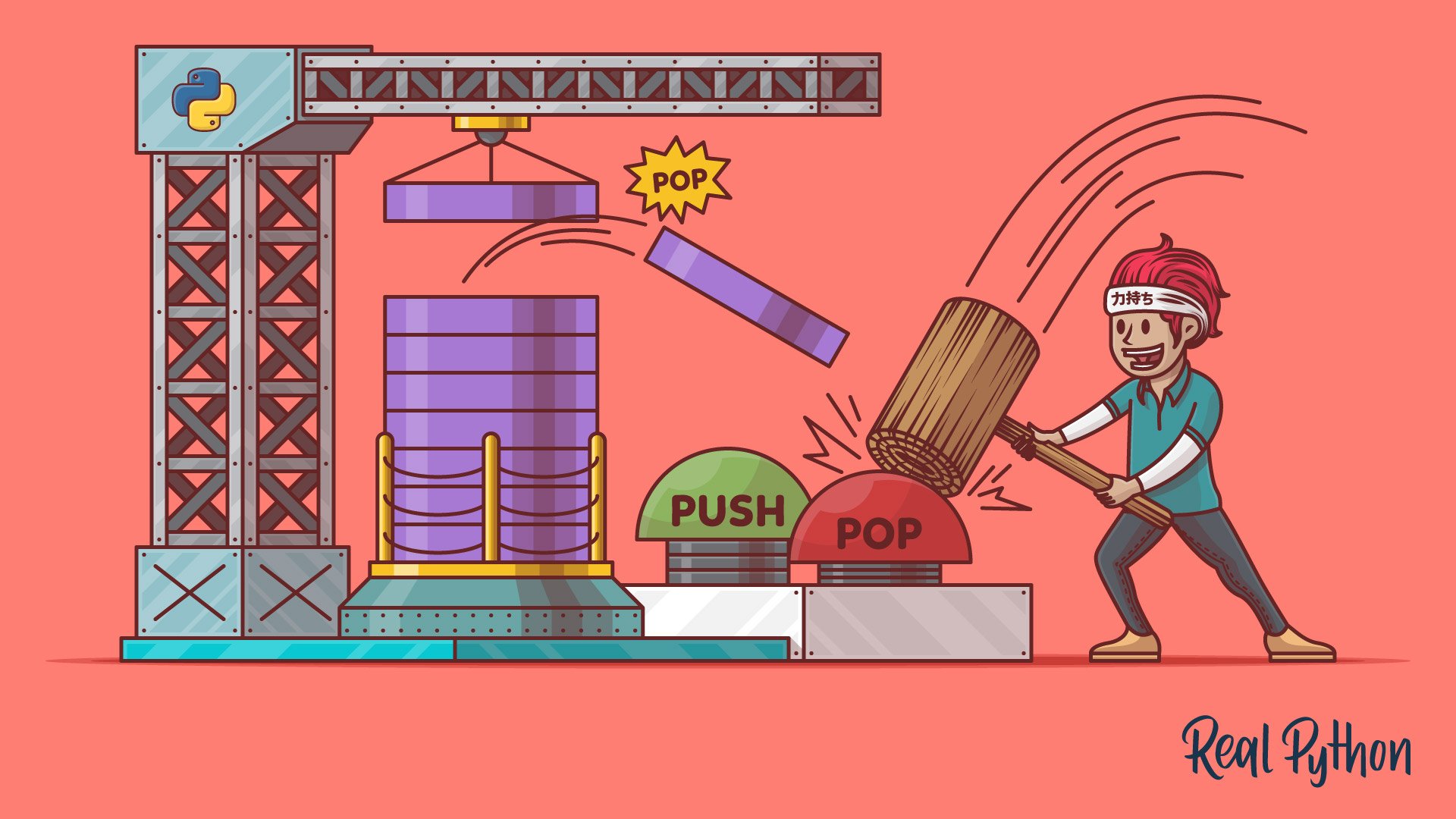
Course
Implementing a Stack in Python
Learn how to implement a Python stack. You'll see how to recognize when a stack is a good choice for data structures, how to decide which implementation is best for a program, and what extra considerations to make about stacks in a threading or multiprocessing environment.
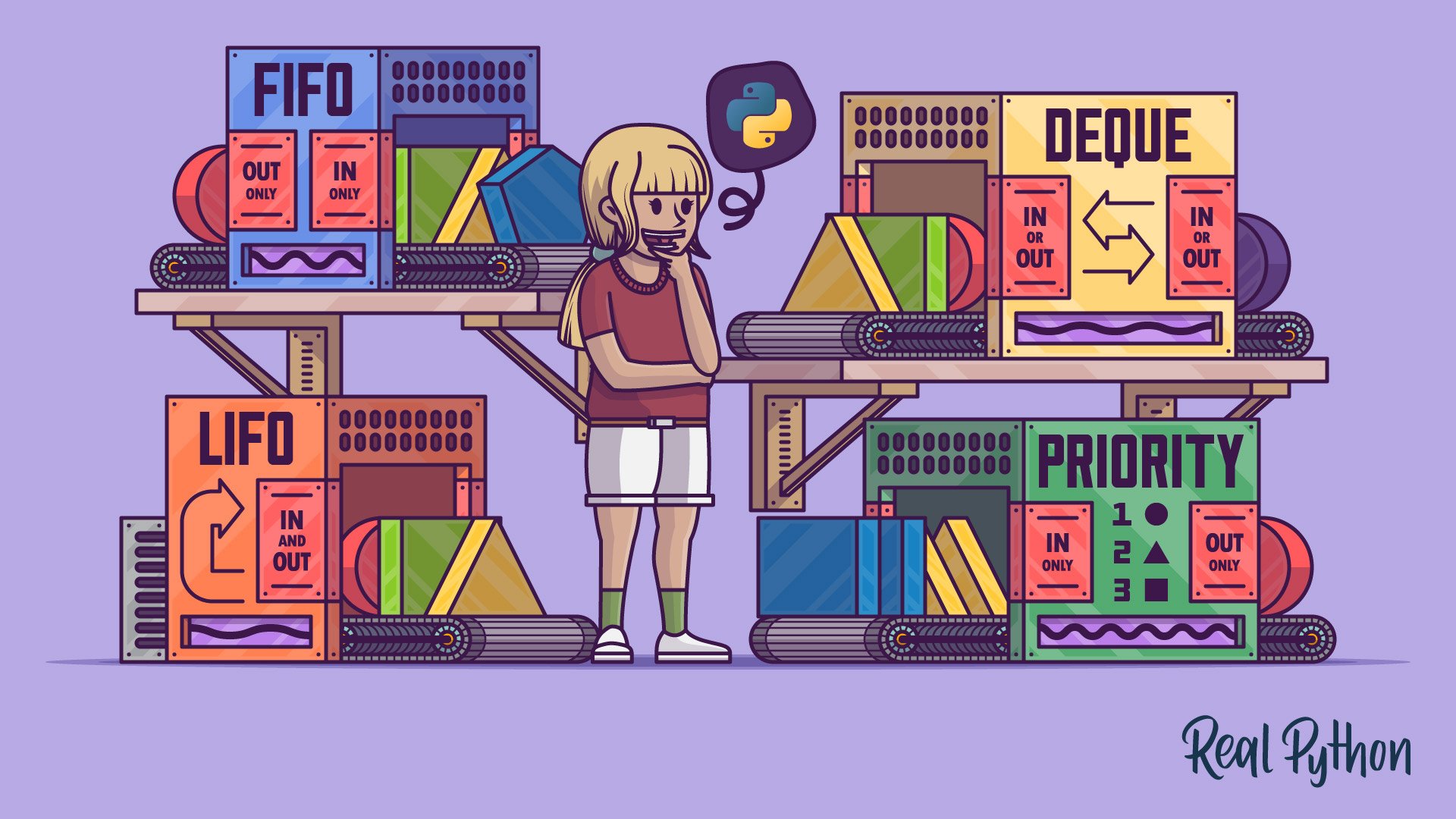
Tutorial
Python Stacks, Queues, and Priority Queues in Practice
In this tutorial, you'll take a deep dive into the theory and practice of queues in programming. Along the way, you'll get to know the different types of queues, implement them, and then learn about the higher-level queues in Python's standard library. Be prepared to do a lot of coding.
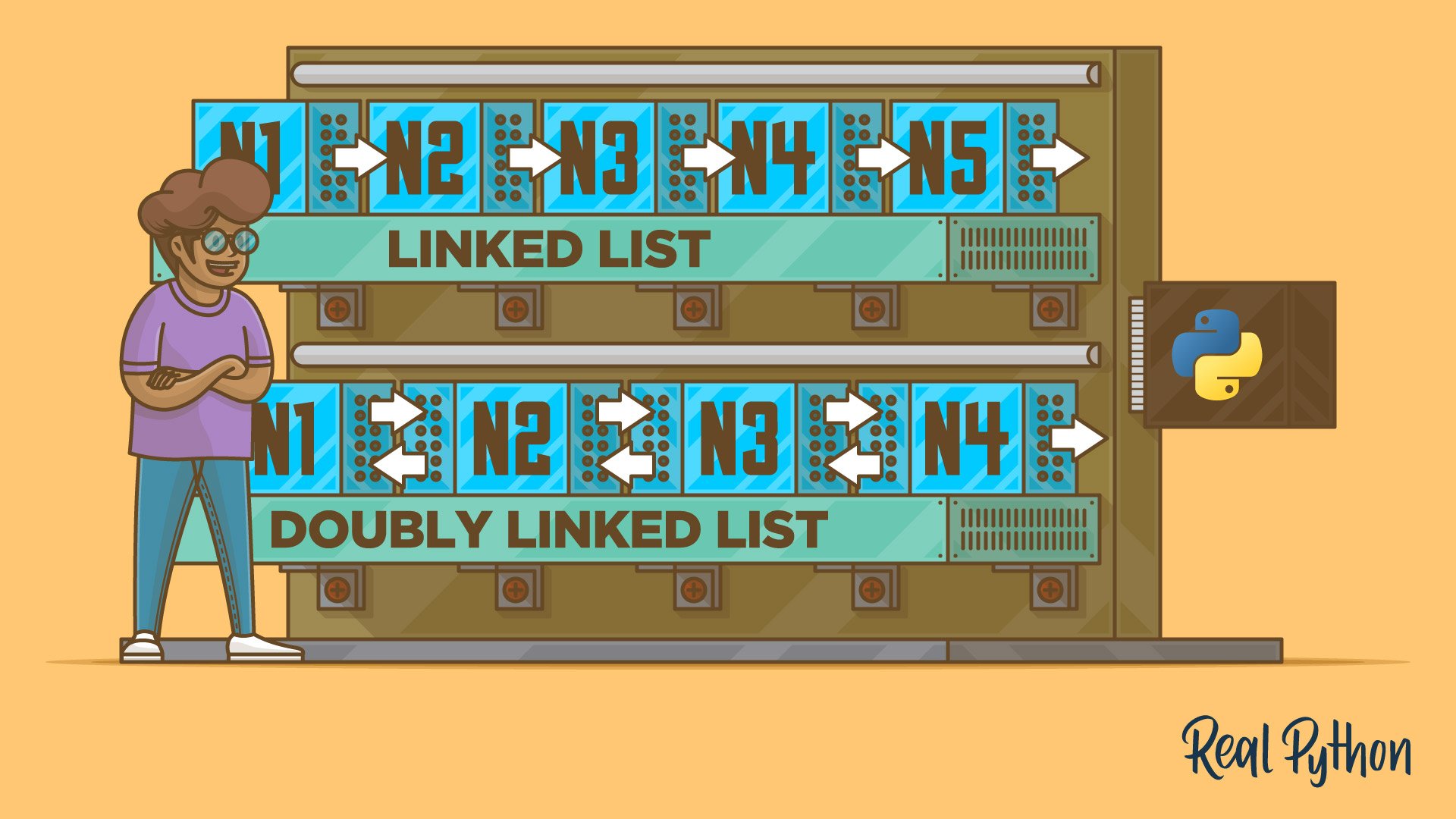
Course
Working With Linked Lists in Python
Learn what linked lists are and when to use them, such as when you want to implement queues, stacks, or graphs. You'll also learn how to use collections.deque to improve the performance of your linked lists and how to implement linked lists in your own projects.
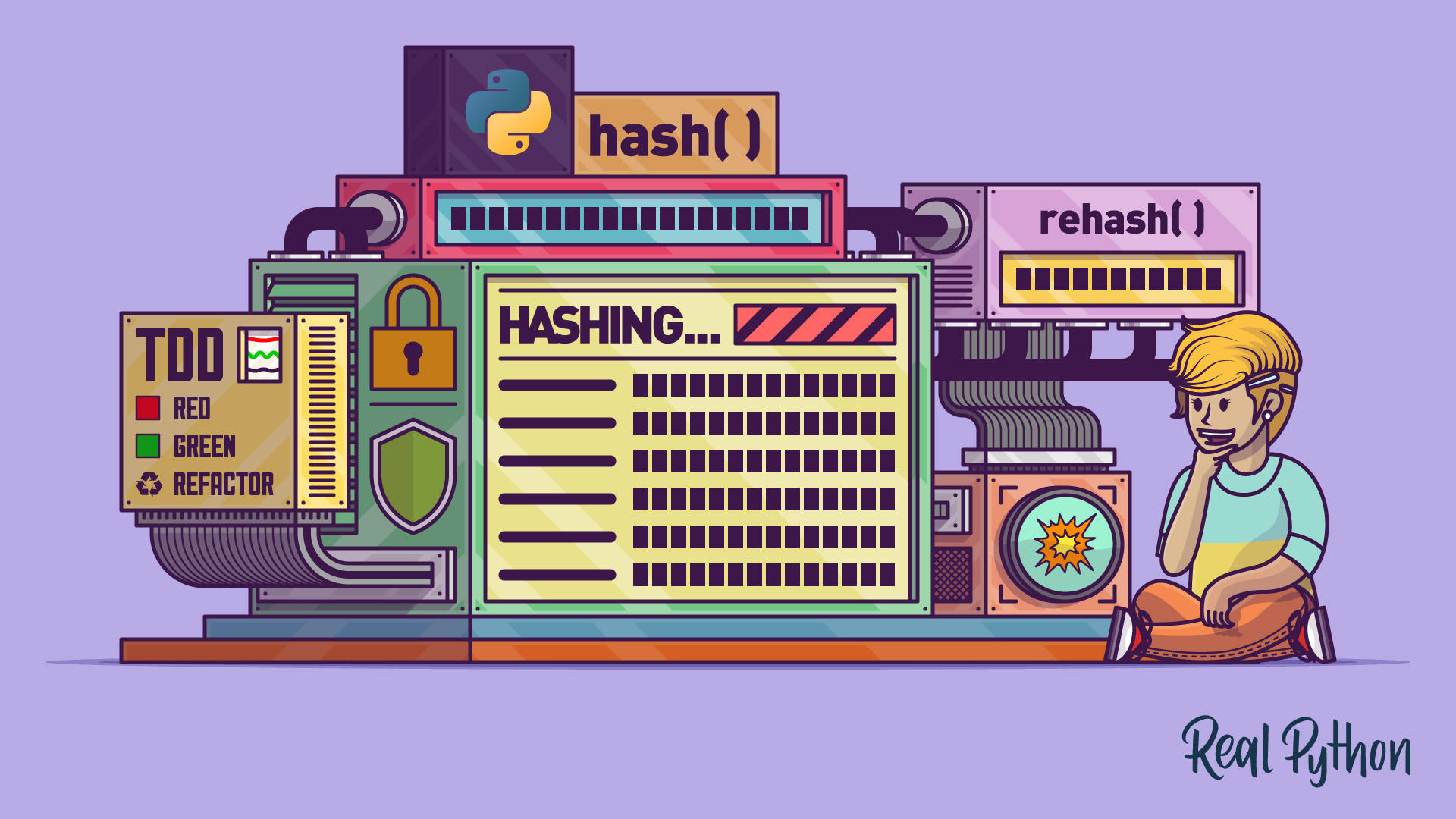
Tutorial
Build a Hash Table in Python With TDD
In this step-by-step tutorial, you'll implement the classic hash table data structure using Python. Along the way, you'll learn how to cope with various challenges such as hash code collisions while practicing test-driven development (TDD).
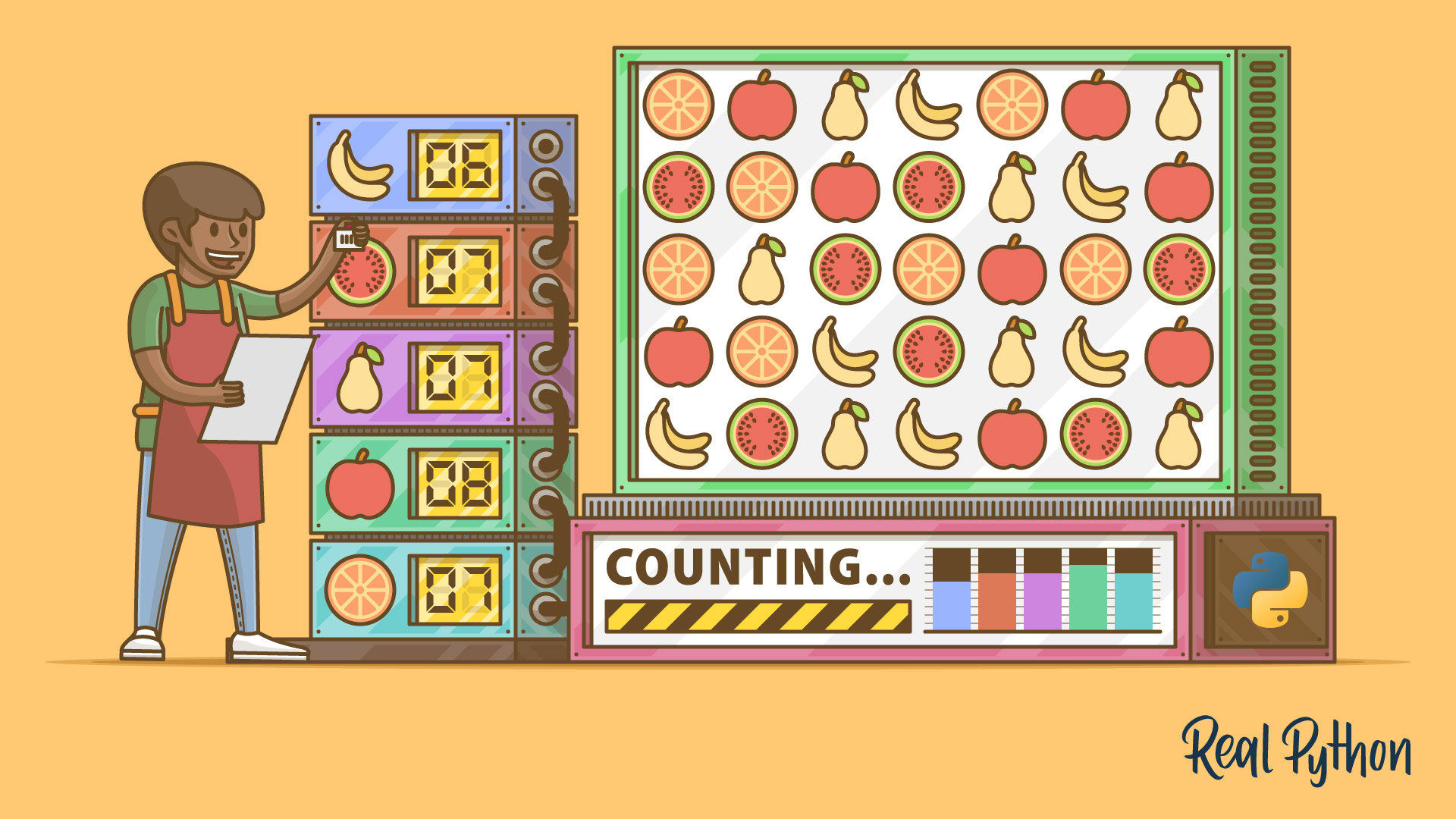
Course
Counting With Python's Counter
Learn how to use Python's Counter to count several repeated objects at once.
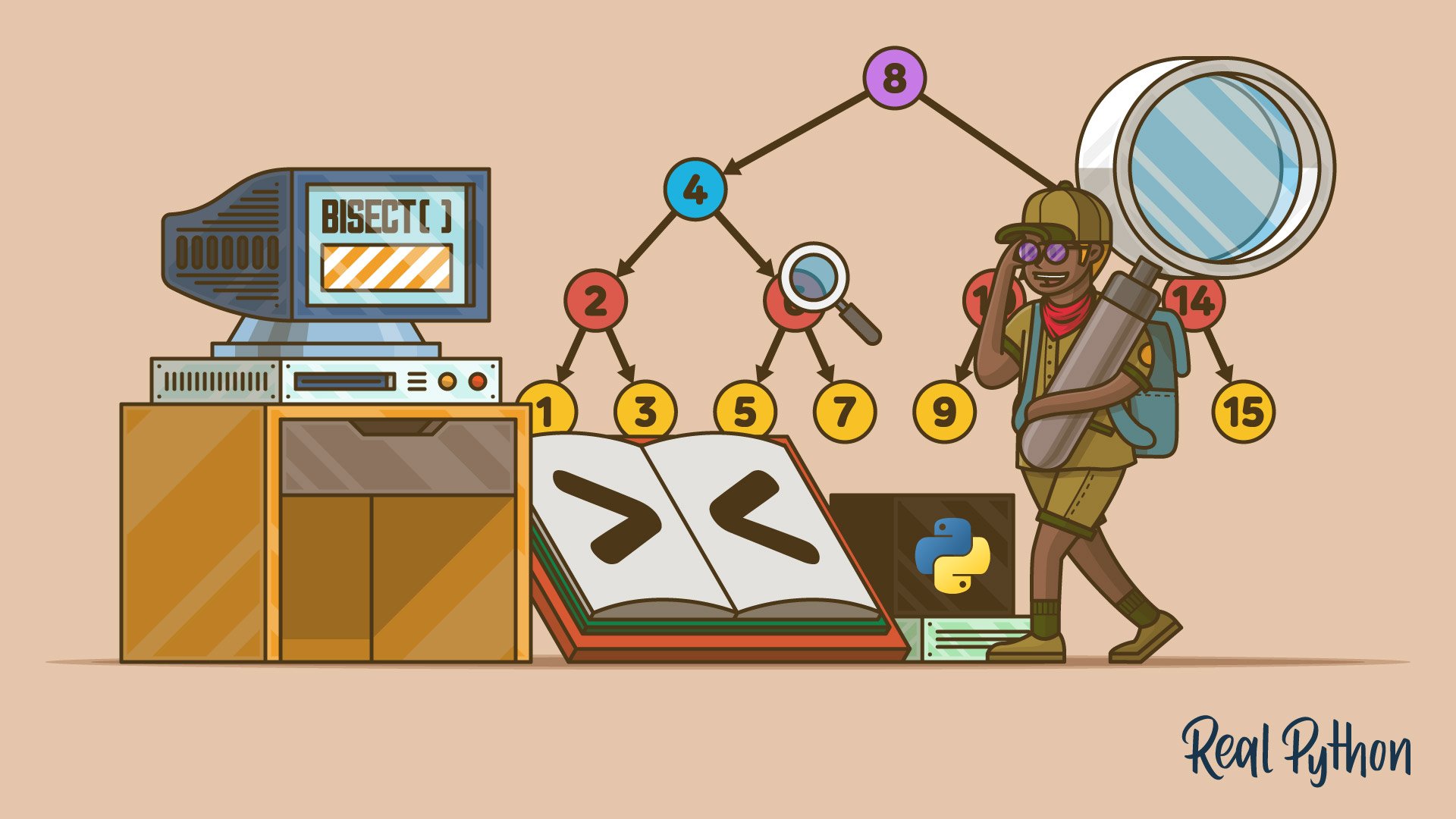
Course
Creating a Binary Search in Python
Binary search is a classic algorithm in computer science. In this step-by-step course, you'll learn how to implement this algorithm in Python. You'll learn how to leverage existing libraries as well as craft your own binary search Python implementation.
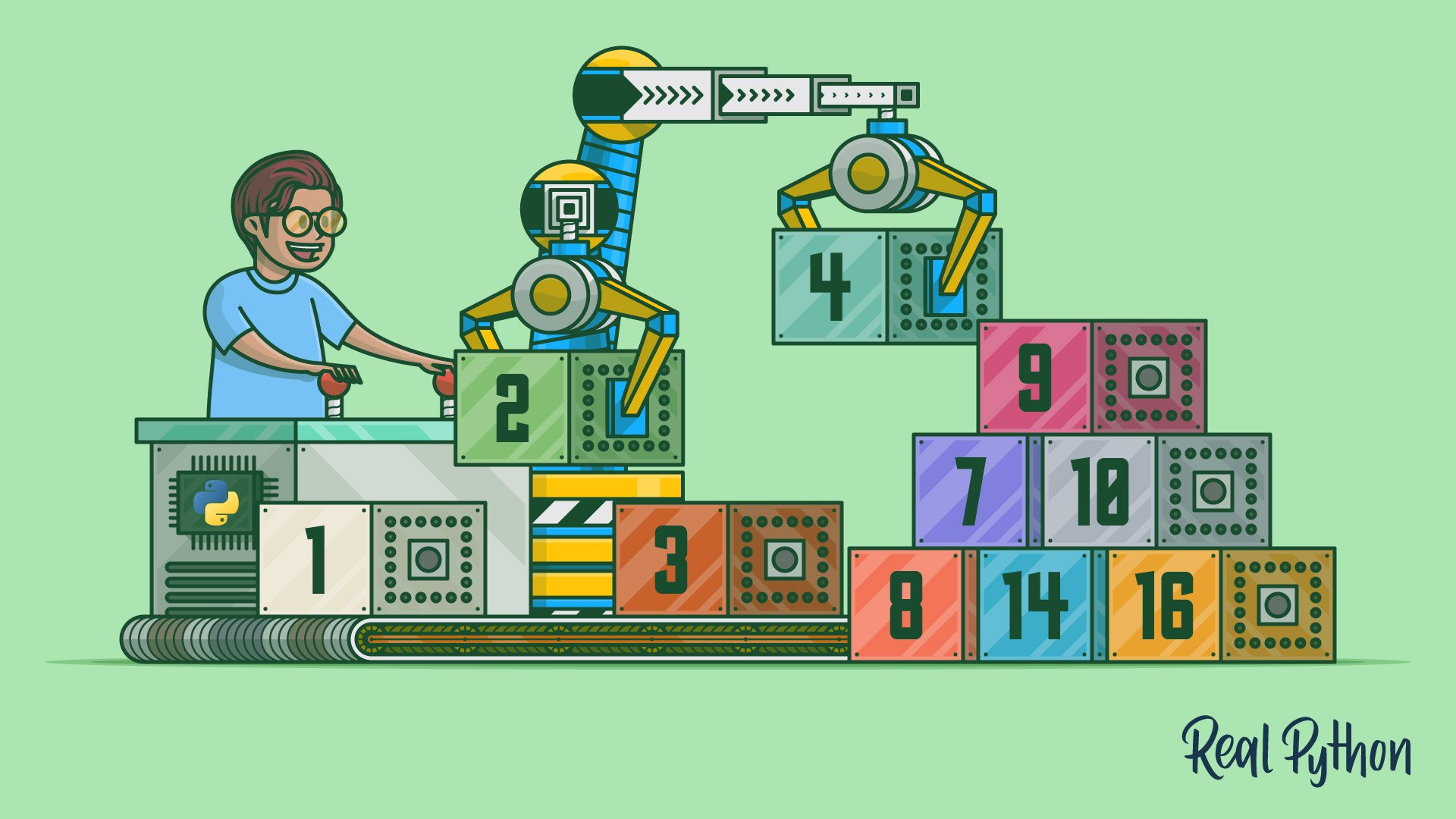
Tutorial
The Python heapq Module: Using Heaps and Priority Queues
In this step-by-step tutorial, you'll explore the heap and priority queue data structures. You'll learn what kinds of problems heaps and priority queues are useful for and how you can use the Python heapq module to solve them.
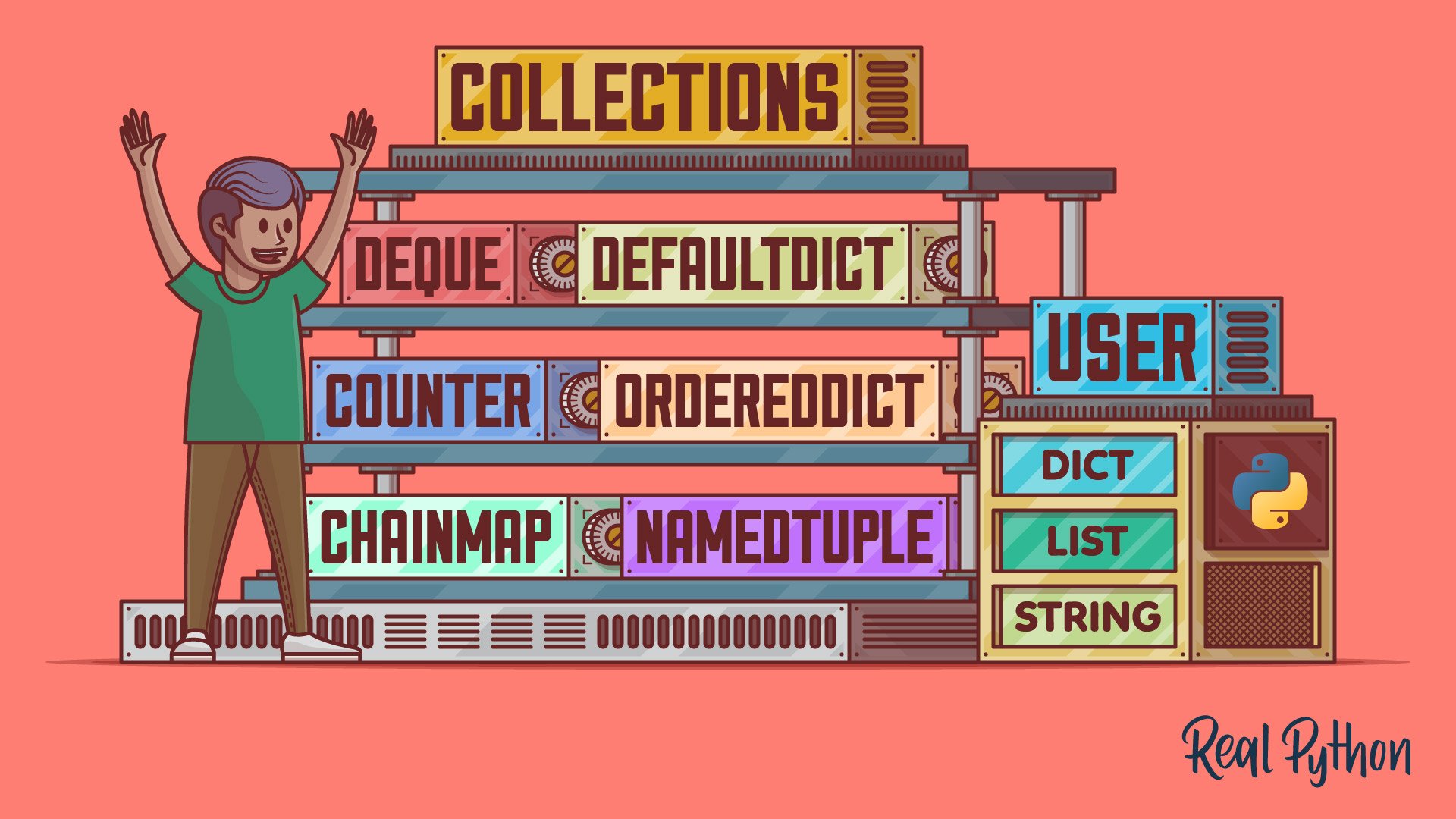
Tutorial
Python's collections: A Buffet of Specialized Data Types
Learn all about the series of specialized container data types in the collections module from the Python standard library.
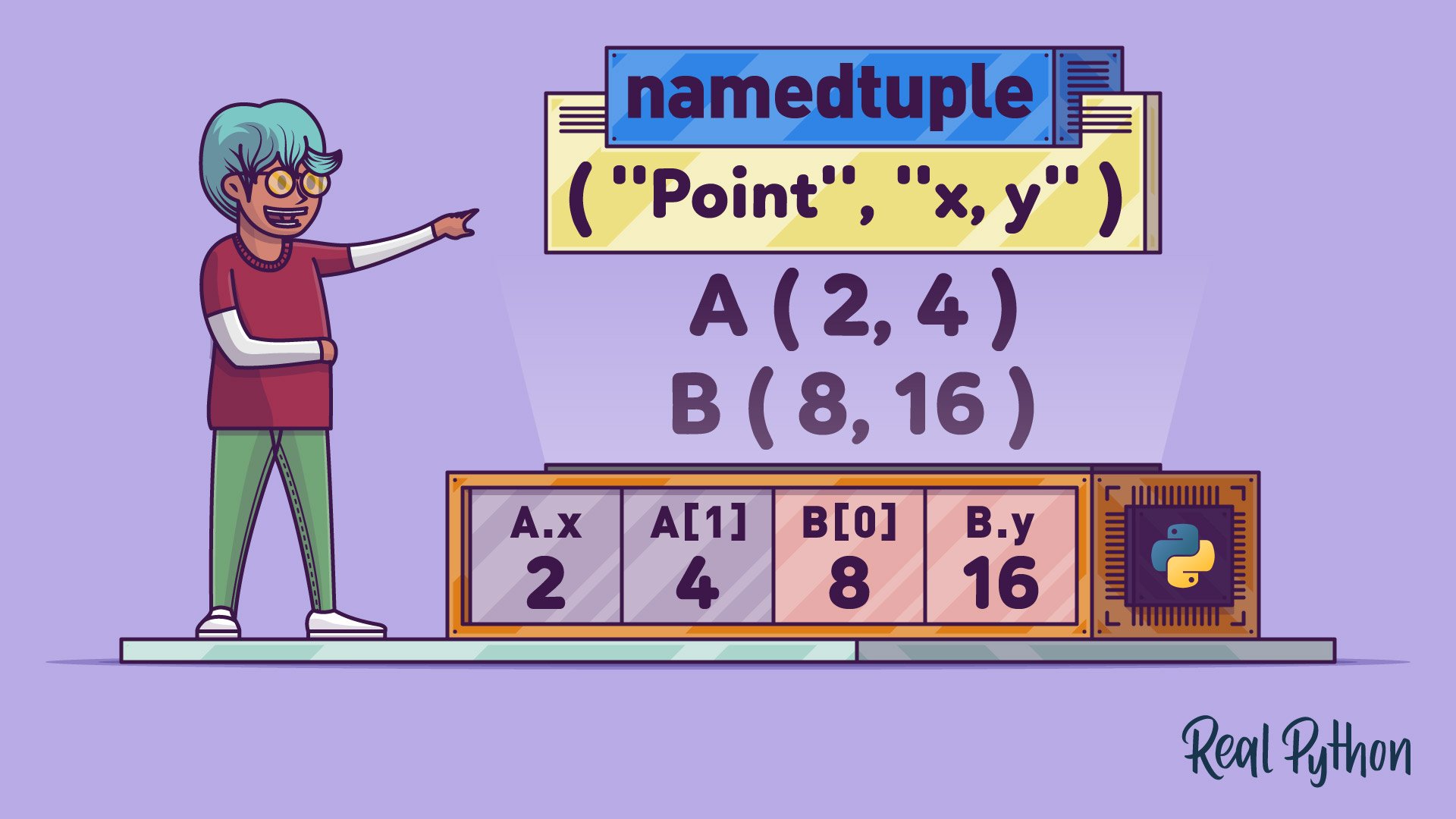
Course
Writing Clean, Pythonic Code With namedtuple
Learn what Python's namedtuple is and how to use it in your code. You'll also learn about the main differences between named tuples and other data structures, such as dictionaries, data classes, and typed named tuples.
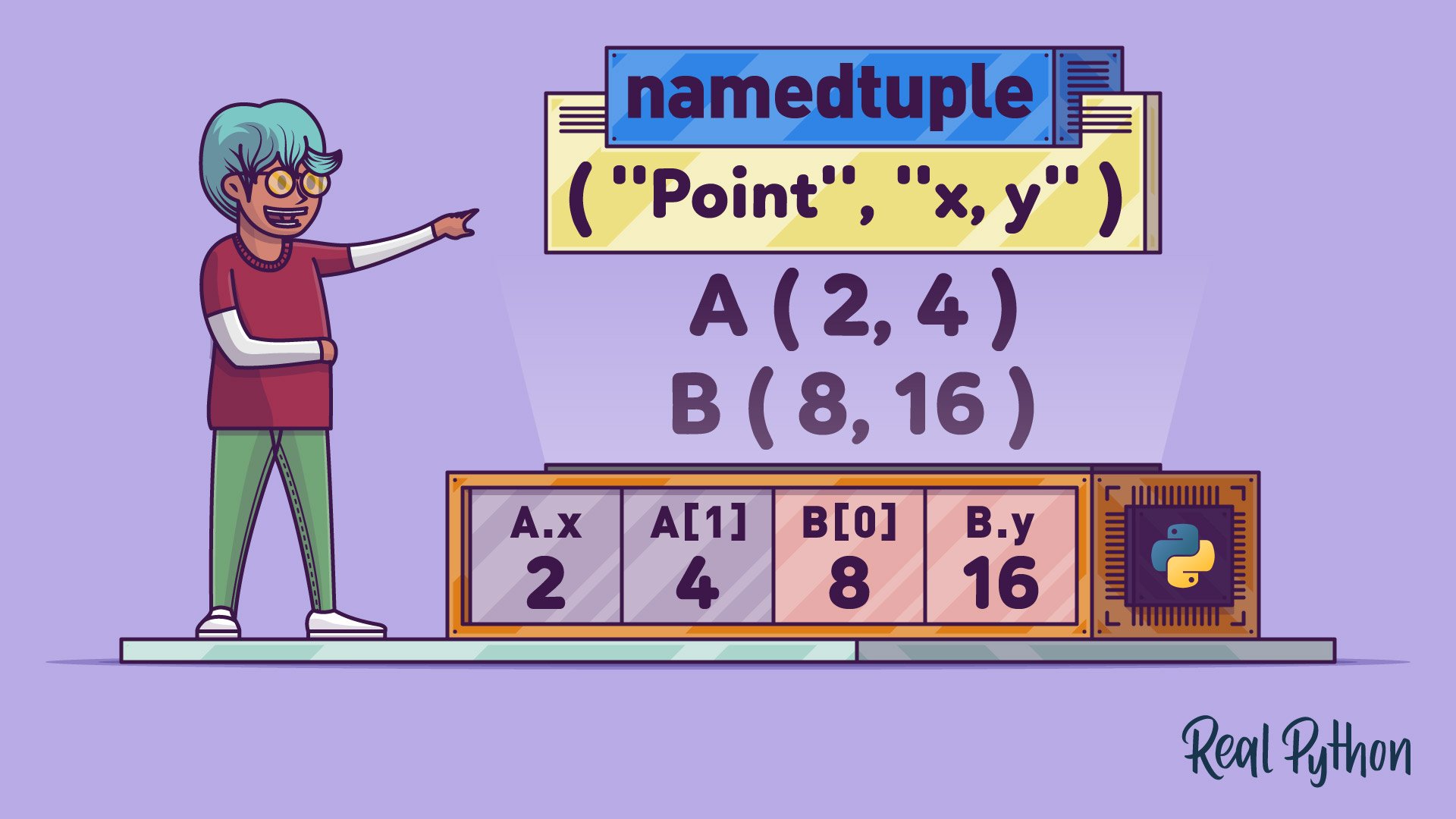
Interactive Quiz
Write Pythonic and Clean Code With namedtuple
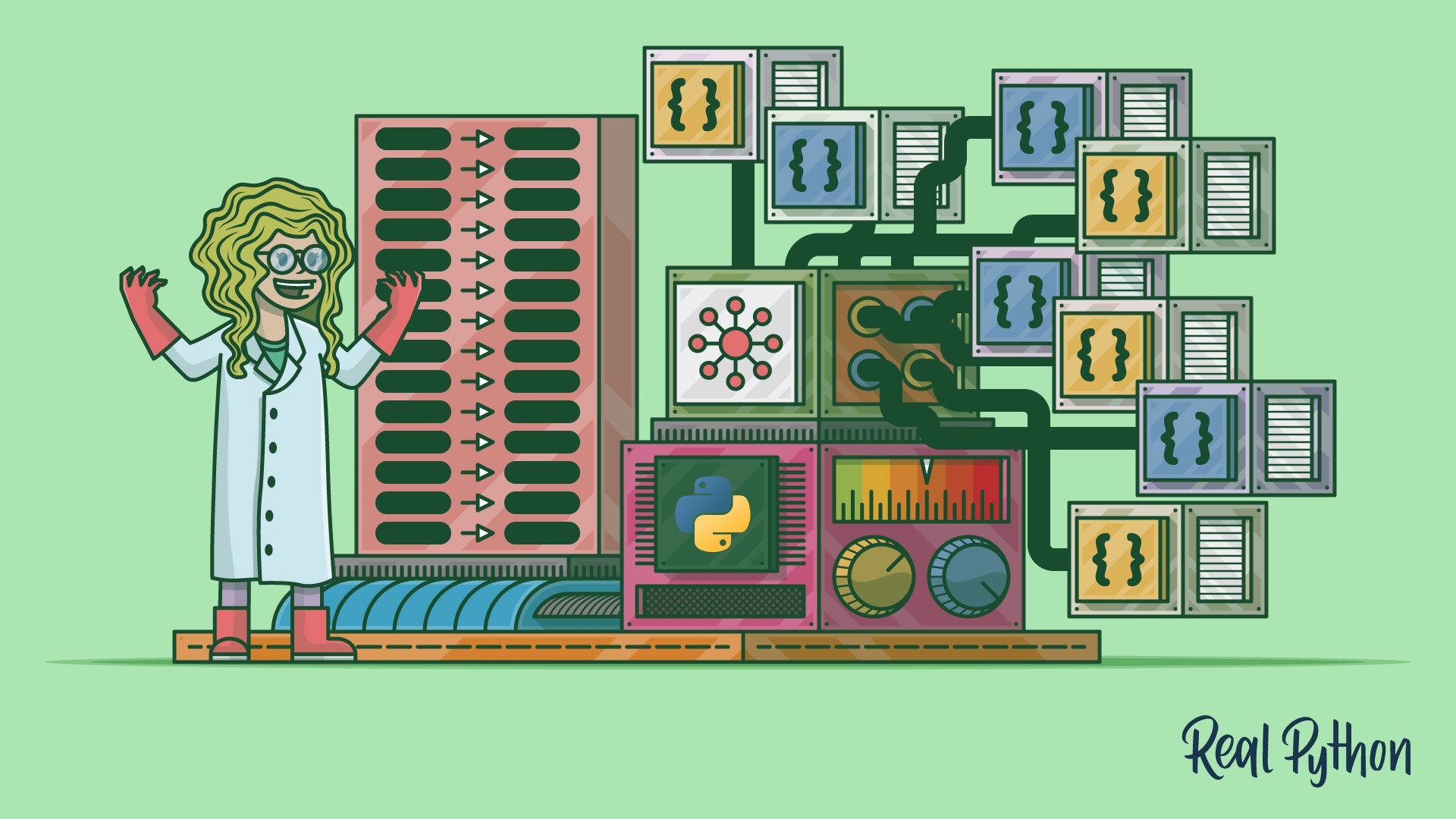
Tutorial
Python's ChainMap: Manage Multiple Contexts Effectively
Learn about Python's ChainMap and how to use it to group multiple dictionaries together and manage them as a single one. ChainMap is handy when you need to manage multiple scopes and contexts and define access priorities.
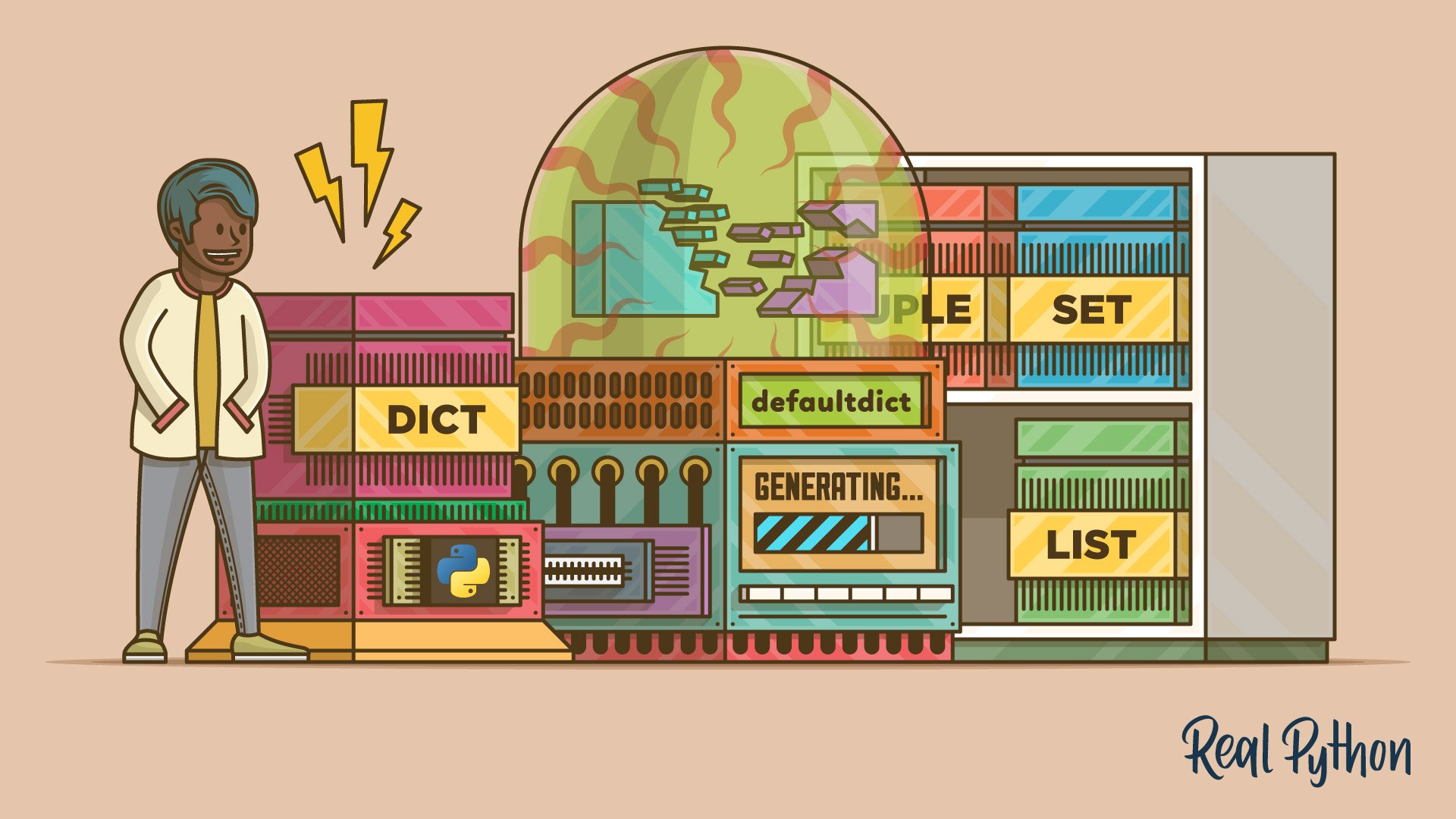
Course
Handling Missing Keys With the Python defaultdict Type
Learn how the Python defaultdict type works and how to use it for handling missing keys when you're working with dictionaries. You'll also learn how to use a defaultdict to solve problems like grouping or counting the items in a sequence or collection.
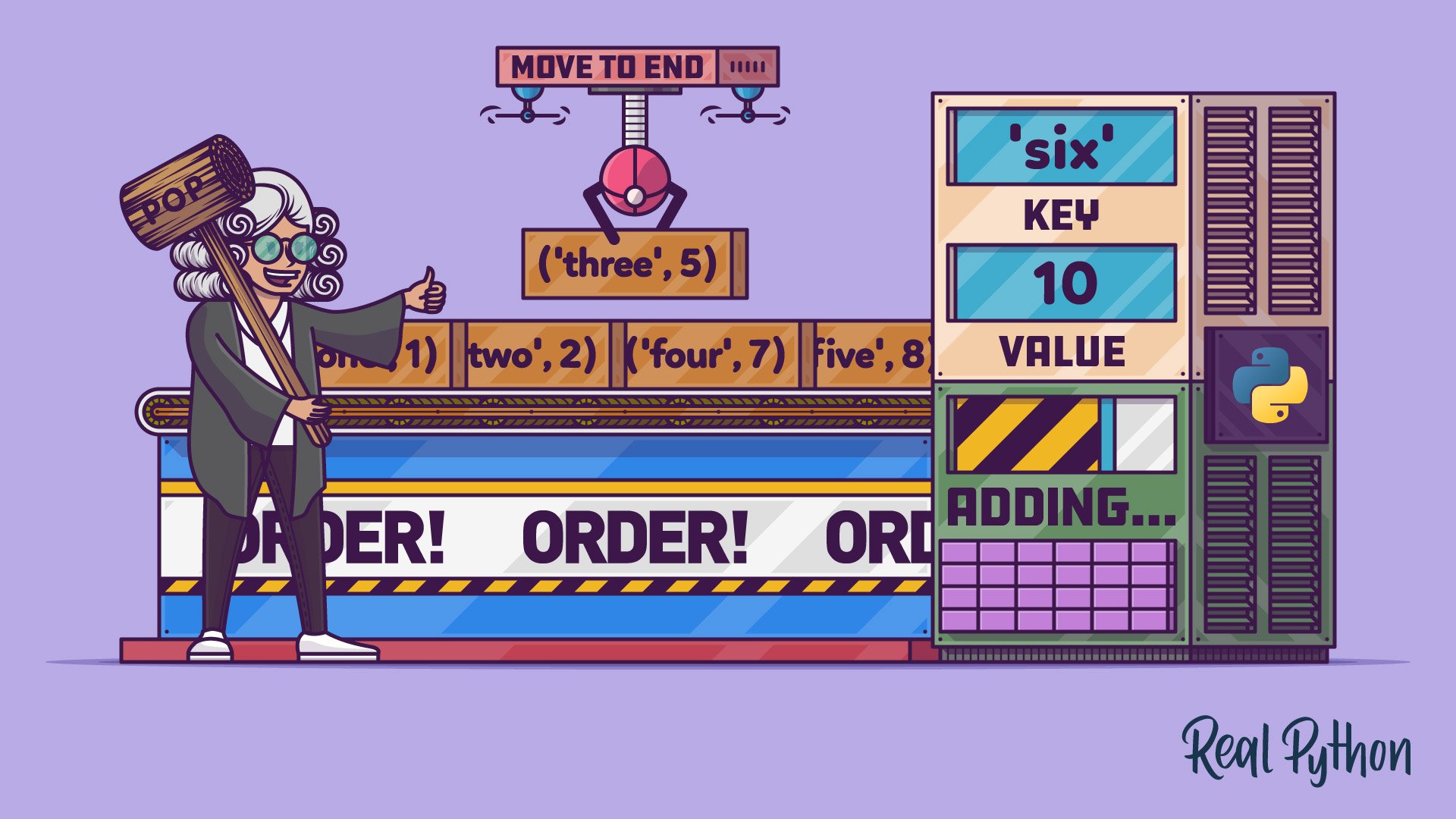
Course
Using OrderedDict in Python
Learn what Python's OrderedDict is and how to use it in your code. You'll also learn about the main differences between regular dictionaries and ordered dictionaries.
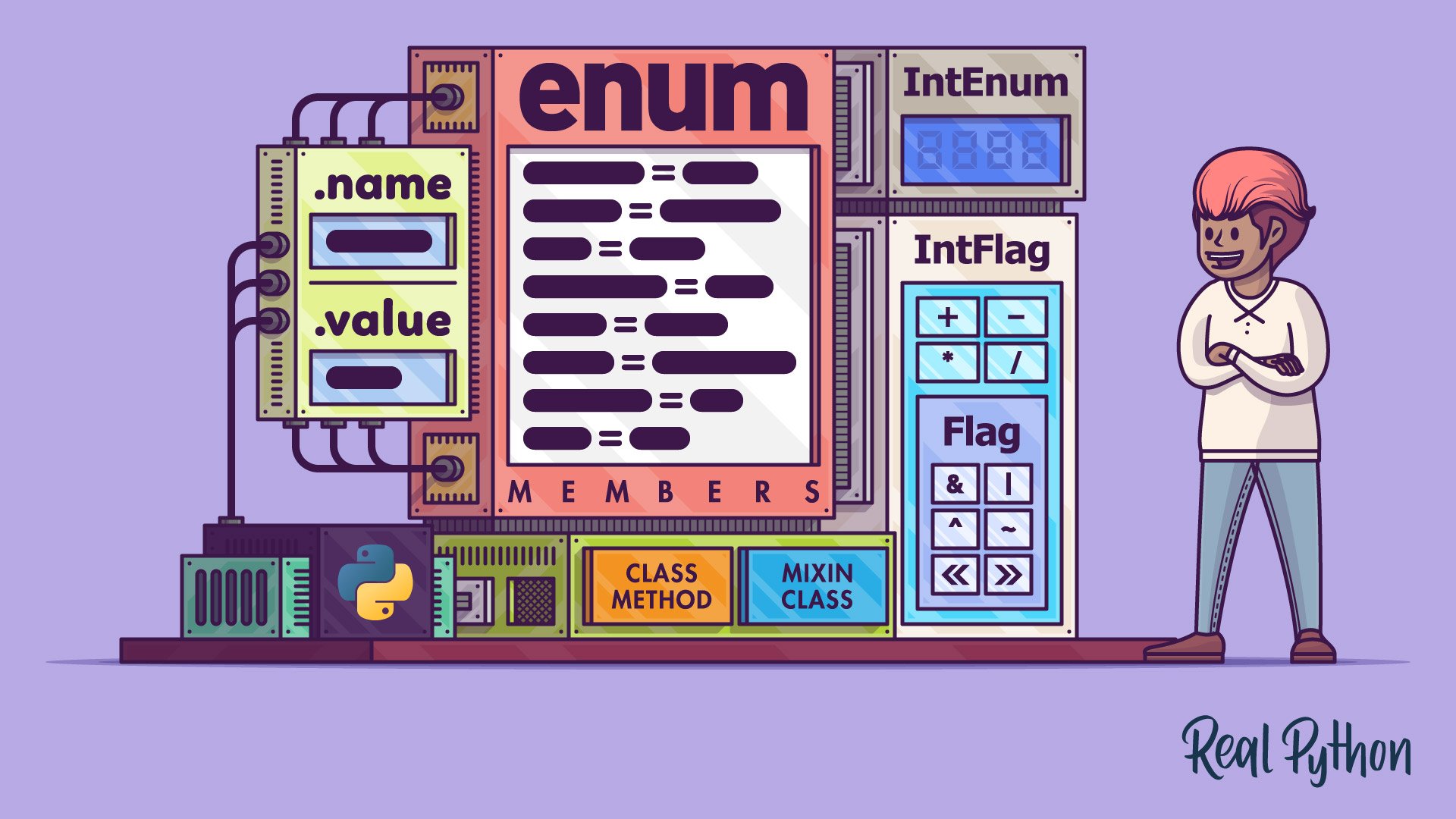
Course
Building Enumerations With Python's enum
In this video course, you'll discover the art of creating and using enumerations of logically connected constants in Python. To accomplish this, you'll explore the Enum class and other associated tools and types from the enum module from the Python standard library.
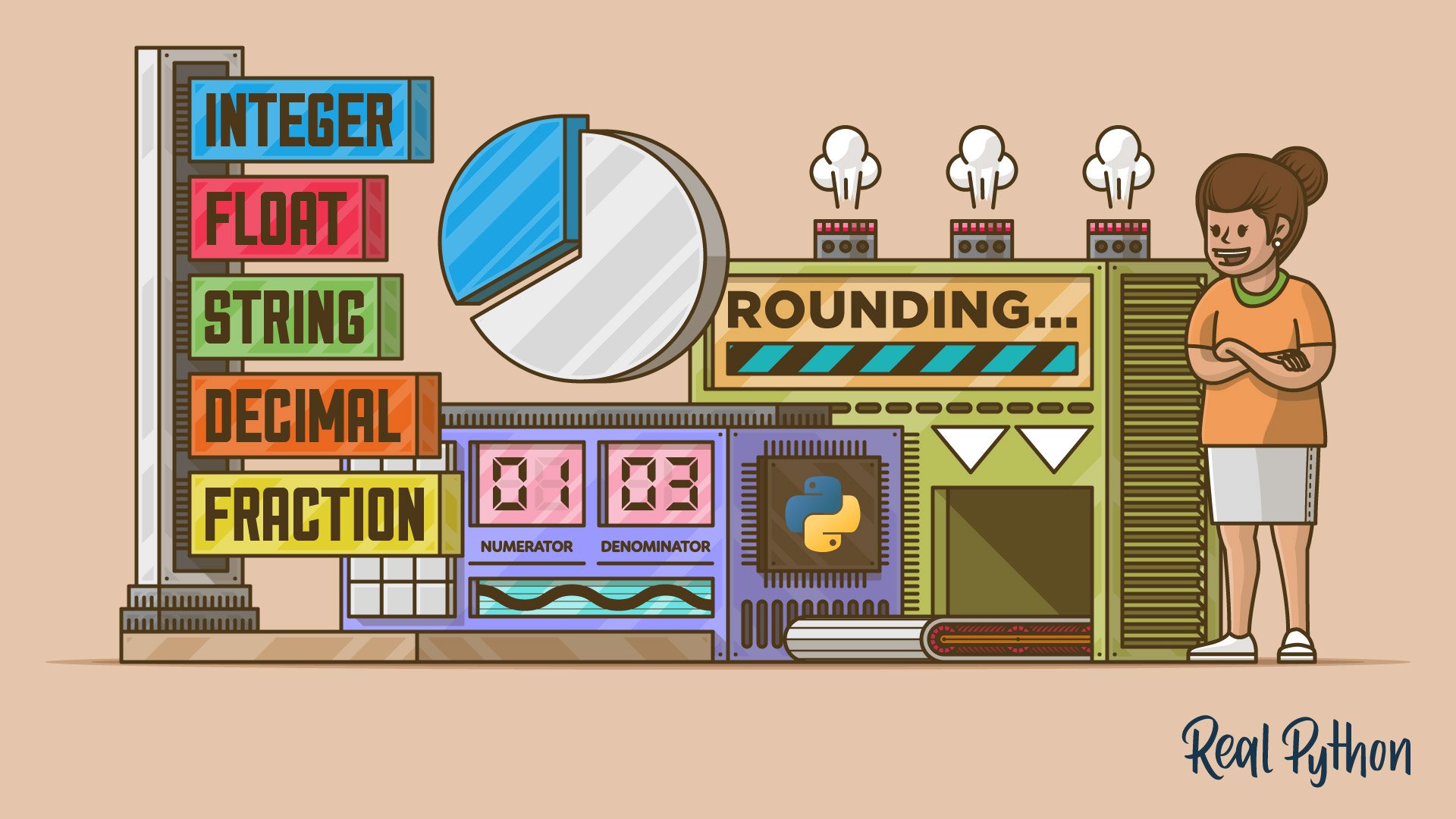
Tutorial
Representing Rational Numbers With Python Fractions
Learn about the Fraction data type in Python, which can represent rational numbers precisely without the rounding errors in binary arithmetic. You'll find that this is especially important in financial and other high-precision applications.
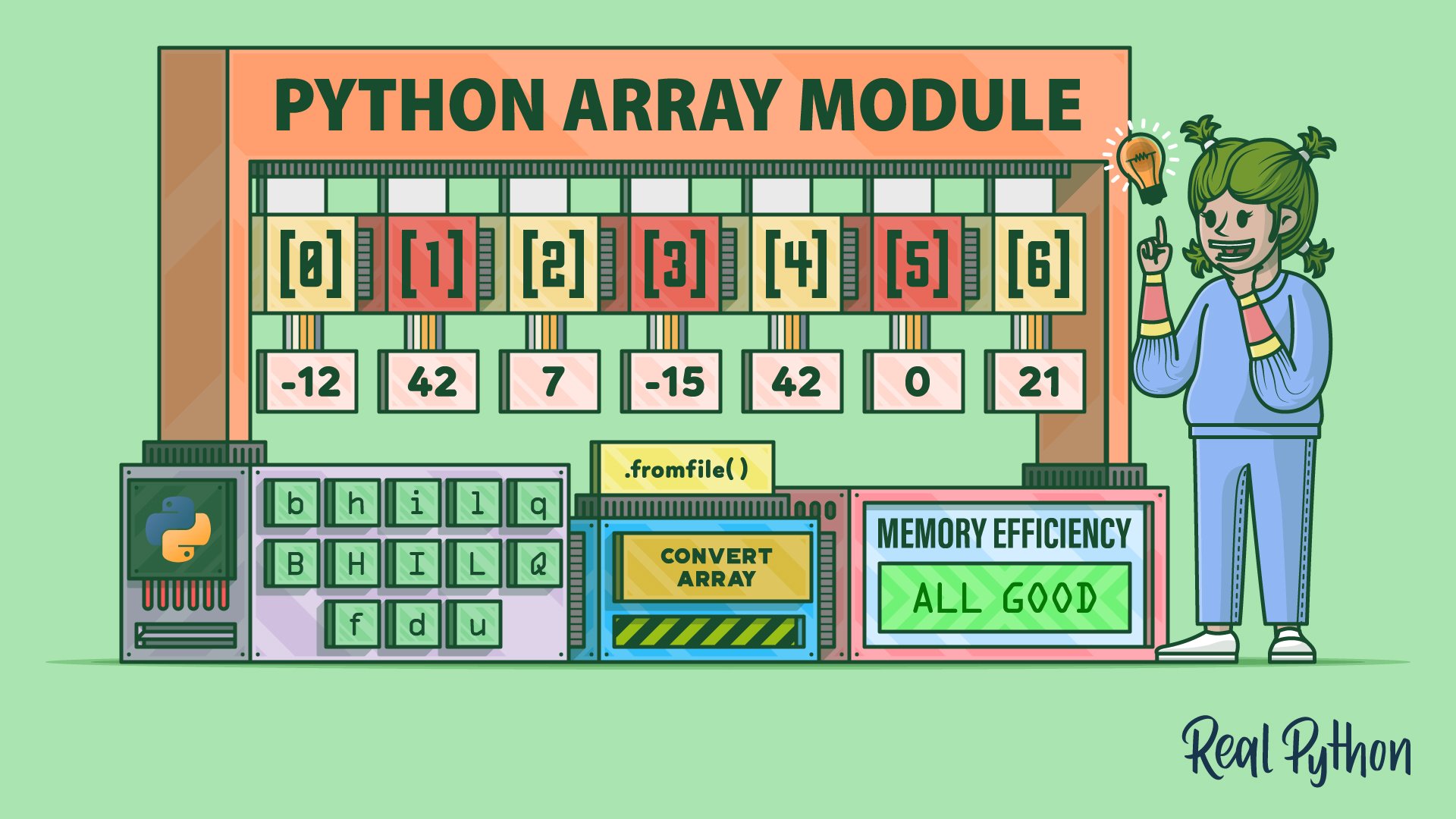
Tutorial
Python's Array: Working With Numeric Data Efficiently
In this tutorial, you'll dive deep into working with numeric arrays in Python, an efficient tool for handling binary data. Along the way, you'll explore low-level data types exposed by the array module, emulate custom types, and even pass a Python array to C for high-performance processing.
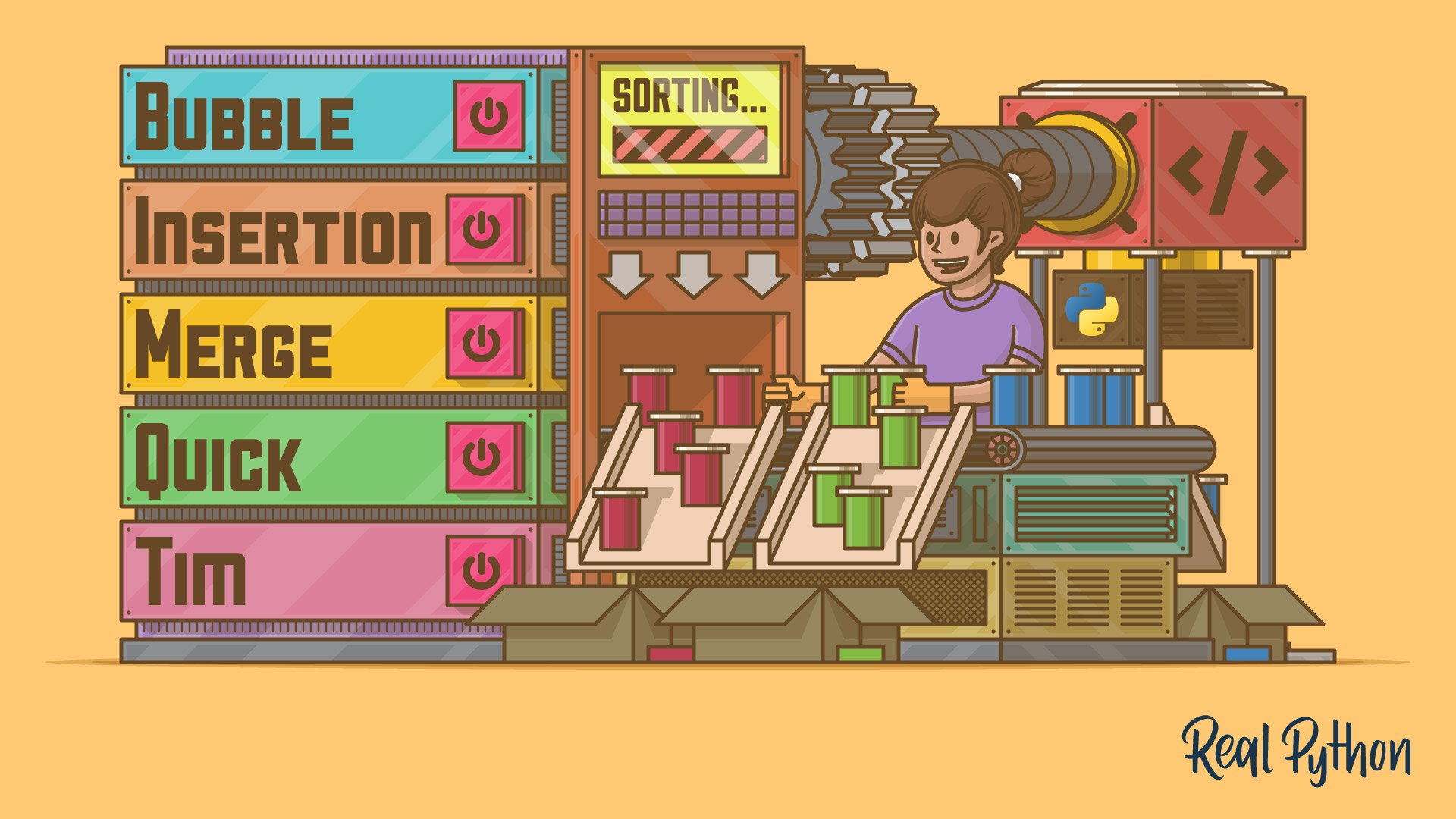
Course
Introduction to Sorting Algorithms in Python
Learn all about five different sorting algorithms in Python from both a theoretical and a practical standpoint. You'll also learn several related and important concepts, including Big O notation and recursion.
Got feedback on this learning path?
Looking for real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session. Happy Pythoning!