Building Your First Dash Application
00:00 Building your first Dash application. For development purposes, it’s useful to think of the process of building a Dash application in two steps. Firstly, define the looks of your application using the app’s layout.
00:15
Secondly, use callbacks to determine which parts of your app are interactive and what they react to. Create an empty file named app.py
in the root directory of your project, and then open it up in the editor of your choice. Here, you can see the first few lines of app.py
being entered.
00:41
In the first section of the code, you import required libraries and modules: dash
, dcc
, which is part of dash
, html
, which is also part of dash
, and pandas
, which is imported with the traditional alias of pd
.
00:57
Each of these provides a building block for your application. dash
is used to initialize your application. dcc
allows you to create interactive components, such as graphs, dropdowns, and date ranges. html
lets you access HTML tags. And pandas
helps you read and organize the data.
01:22 In the next section of the code, you read the data and pre-process it for use in the dashboard. You filtered some of the data because the current version of your dashboard isn’t interactive, and the plotted values wouldn’t make sense otherwise.
01:49
In the last line seen onscreen, you create an instance of the Dash
class. If you’ve used Flask before, then initializing a Dash
class may look familiar.
01:59
In Flask, you usually initialize a WSGI application using Flask(__name__)
. Similarly, for a Dash app, you used Dash(__name__)
, as seen onscreen.
02:11 Next, you’ll define the layout property of your application. This property dictates the look of your app. In this case, you’ll use a heading with a description below it and two graphs. Onscreen, you can see the code that defines it.
03:38
This code defines the .layout
property of the app
object. This property determines the looks of your application using a tree structure made of Dash components.
03:49 You’ll see two sets of components in almost every app. Dash HTML Components provides you with Python wrappers for HTML elements. For example, you could use this library to create elements such as paragraphs, headings, or lists. Dash Core Components provides you with Python abstractions for creating interactive user interfaces.
04:10 You can use it to create interactive elements, such as graphs, sliders, or dropdowns. On lines 15 to 22, you can see the Dash HTML Components in practice.
04:22
You start by defining the parent component, an html.Div
. Then you add two more elements: a heading, html.H1
, and a paragraph, html.P
, as its children.
04:34
These components are equivalent to the <div>
, <h1>
, and <p>
HTML tags. You can use the component’s arguments to modify attributes or the content of the tags.
04:46
For example, to specify what goes inside the <div>
tag, you use the children
argument in html.Div()
. There are also other arguments in the components such as style
, className
, or id
that refer to attributes of the HTML tags.
05:01 You’ll see how to use some of these properties to style your dashboard in the next section. The part of the layout shown on lines 15 to 22 is transformed into the HTML code that you see onscreen. This HTML code is rendered when you open your application in the browser.
05:18
It follows the same structure as your Python code, with a <div>
tag containing an <h1>
and a <p>
element.
05:26
On lines 23 to 26 in the layout code snippet, you can see the Graph
component from Dash Core Components in practice. There are two dcc.Graph
components in the app layout.
05:39 The first one plots the average prices of avocados during the period of study, and the second plots the number of avocados sold in the United States during this same period.
05:50
Under the hood, Dash uses Plotly.js to generate graphs. The dcc.Graph
components expect a figure object or a Python dictionary containing the plot’s data and layout. In this case, you’re providing the latter. Finally, these last two lines of code run the application.
06:19
These lines make it possible to run the Dash application locally using Flask’s built-in server. The debug=True
parameter from app.run_server()
enables the hot-reloading option in your application.
06:32 This means that when you make a change to your app, it reloads automatically without you needing to restart the server. The full code for the application is included in the course files, but it’s always a good idea to enter the code yourself.
06:47 It will help with understanding what’s going on inside the code, and also remembering the syntax. It will also help when you come to create your own project from scratch, which, after all, is the main idea of this course.
07:00
Now it’s time to run your application. Open a terminal inside your project’s root directory and in the project’s virtual environment. Run Python app.py
, and then go to localhost:8050
, using your preferred browser, as seen onscreen.
07:18 Your dashboard should look like this.
07:24 The good news is you’ve created a working version of the dashboard. The not-so-good news is there’s still some work to do before you can show this to others.
07:33 The dashboard is far from visually pleasing, and you still need to add some interactivity to it. If you want to stop the app running, press Control + C in the terminal window, and it should shut down.
07:46 In the next section of the course, you’ll see how to style your Dash application, starting with applying a custom style.
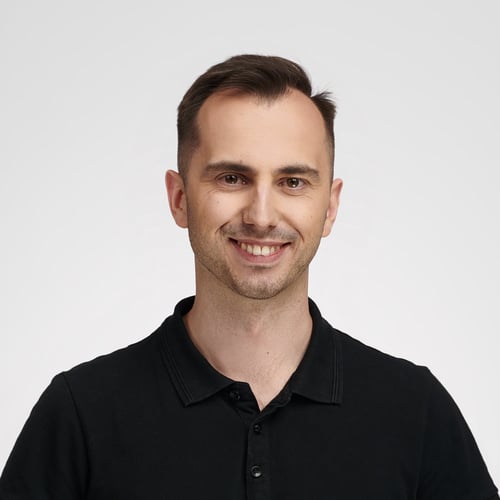
Bartosz Zaczyński RP Team on Dec. 15, 2021
@erico It sounds like you might have installed Dash to a virtual environment while VS Code is using the system-wide Python interpreter or vice versa. You can check which Python the editor is using in the bottom left corner of the window.
Become a Member to join the conversation.
erico on Dec. 15, 2021
Hi. Would someone be able to point me in the right direction to look for help? I am debugging in VS Code, but it says that the dash module is not found, even though I did pip install it.
If I run the script with just the attempted imports of dash in VS Code, it errors with the above. If I run it from the cli in the venv, it executes fine
Many Thanx!