Checking Whether a File Path Exists
00:00
In this lesson, you will learn how to check whether a file path exists or doesn’t exist. To start this off, it might be unintuitive, but you can create Path
objects whether or not the file path exists.
00:12
It just probably doesn’t make much sense to do so unless you’re planning to create it later on. And you can do this check by using the .exists()
method on a Path
object, which returns True
if the path exists and else False
.
00:26
So let’s look at that in code I will import pathlib
again, and then let’s create a Path
object. I’ll make the home
directory and say pathlib.Path.home()
.
00:43
And now we again have a Path
object that points to /Users/martin
. Now in here, I can create a path that points forward to my desktop. I’ll call this one desk
. home / "Desktop"
.
01:03
I’ll make another one that points to my documents. I call it docs
.
01:12
So if you’ve been paying close attention in the previous videos, you’ve seen that I referenced a file called "hello.txt"
a couple of times. The first time was when we were reading in the content of this file, and that file was located in the documents folder.
01:29
And then later on, I was talking about the desktop and the file hello.txt
there. And now we’ll use this to see which of those two files actually exists. Again, I will need to create the path for both of these.
01:40
So I’ll save it again to a variable, and I’ll say hi_desk
is the path to the desktop combined with then the name of the file.
01:54
And then I’ll make another one that I call hi_docs
. And that’s the documents folder followed again by the name of the file. Okay, so this is just a little bit more training of how do you combine paths and build Path
objects you just learned before, and now you have this path that points to /Users/martin/Desktop.hello.txt
and then the docs path that points to a file called hello.txt
in the documents folder.
02:23
Now which of those two exists? Let’s go and check. I’m gonna say hi_docs.exists()
. That returns True
for me. And if I say hi_desk.exists()
, then I got a False
.
02:38
So this tells me that on this path, /Users/martin/
Documents/hello.txt
, there is actually a file—it’s called hello.txt
—and in the other path that goes across my desktop, there is no file called hello.txt
.
02:54
So I can use this method, .exists()
on any Path
object to figure out whether it exists in your file system or it doesn’t exist.
03:02
Now there’s more that you can do, somewhat related operations. You can figure out whether a path points to a file or to a folder and you can do that by saying hi_
—well, just in this case you use the method that is called .is_file()
or .is_dir()
. Start off by using .is_file()
.
03:21
hi_docs.is_file()
and this returns True
because it is a file. And I can run the same operation on that same path, ask whether it is a directory.
03:36
This returns False
. So let’s check for a directory. Before, I’ve created this desk
, which is the path to my desktop. See if it exists
03:48
Phew, my desktop is still there. That’s good to know. Now is it a directory? desk.is_dir()
and that also returns True
. So these three methods can be useful to figure out does a file or a directory exist, and what is it? Is it a file or a directory?
04:09
So as you’ve seen, you can create Path
objects whether or not the file path exists. You’ve seen that with the hello.txt
file on the desktop that doesn’t actually exist, but we still had a Path
object that points to that location.
04:22
Then if you use the method .exists()
on a Path
object, it returns True
if the path exists and otherwise False
.
04:28
Then you’ve also seen two other methods. .is_file()
returns True
if the path points to a file, otherwise False
. And .is_dir()
basically does the opposite.
04:37
It returns True
if the path points to a directory and otherwise False
. There’s a little gotcha there. Both .is_file()
and .is_dir()
return False
if the path doesn’t exist.
04:47 Let’s check on that real quick before ending this lesson.
04:52
So we know that hi_desk
doesn’t exist,
04:59
so if I ran hi_desk.is_file()
, which you may assume because it’s called hello.txt
, then you still get False
, and that’s simply because the file doesn’t even exist.
05:13
Same with the directory. If I would create a path, let me do that directly without putting another variable here. I’m just going to say a path that goes /Users/
and then to gremlin
.
05:30
That’s a username that I do not have on my computer. I can create a Path
object, but if I
05:38 check whether it is a directory,
05:43
it returns False
, and it is simply because this folder does not exist.
05:55
I’ll leave you with this slide. Those are three useful methods that we can use on Path
objects and to get about an idea of what is there on your file system. Is it a file, is it a folder, is it there at all, basically?
06:09
And in the next lesson, let’s look a little closer at what paths are on a file system and the difference between an absolute and a relative path and what you can do with those using pathlib
.
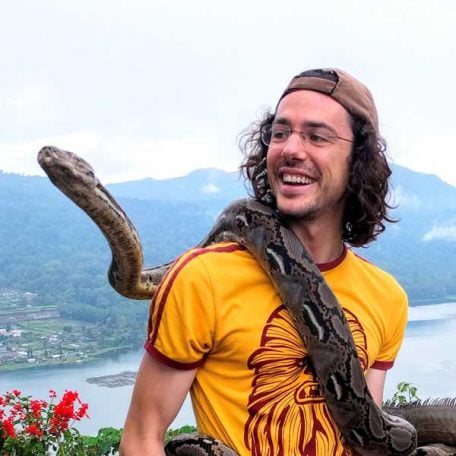
Martin Breuss RP Team on Dec. 6, 2023
Hi @alphafox28js do the two files that you’re checking exist on your computer?
The code that you show doesn’t create a file, it only creates the Path
objects.
If you went to check whether your Desktop or your home folder exist, you should get True
:
>>> import pathlib
>>> home = pathlib.Path.home()
>>> home.exists()
True
>>> desk = home / "Desktop"
>>> desk.exists()
True
If the Path
objects hi_desk
and hi_home
both return False
when you call .exists()
on them, then it means that the files don’t exist in these folders. You can check in your Finder to confirm that they’re not there.
alphafox28js on Dec. 7, 2023
@Martin Breuss, ahhh ok… I think i was under the impression that this would create the actual folder and file by creating the Path Objects… since i have not physically created the folder in file explorer, this would explain why I return false…I think the confusion happened when hi_desk returned the string of the path in the Interactive Window with an output of: WindowsPath(‘C:/Users/ARCHA/Desktop/hello.txt’)
It did not actually create it. It only created the path showing the the object with “”physical”” storage location in the drive.
Thank you sir :)
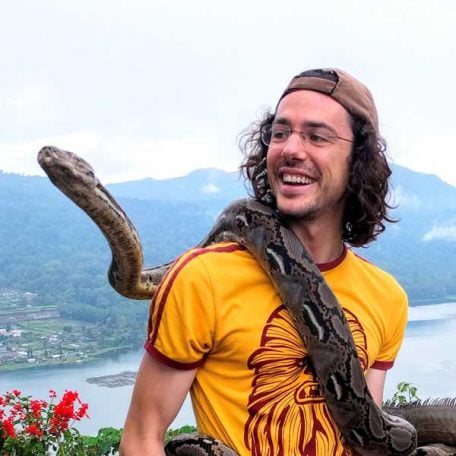
Martin Breuss RP Team on Dec. 7, 2023
Yes, exactly! :) I agree that this can be confusing, happy to help!
Become a Member to join the conversation.
alphafox28js on Dec. 6, 2023
Hi, so for some reason, using the interactive window in VS Code, i am able to return the file paths, but when I go to call the methods, they return false.
Not sure if this is correct…