Creating Path Objects From Strings
00:00
The main interface for working with file paths in Python is the pathlib
module. It is built in, but you will have to import it in order to work with it.
00:09
You do that by typing import pathlib
. And I will head over to an IDLE session to work alongside the things that I’ll be talking about here. In the IDLE REPL, I’m start off by typing import pathlib
, and it gives me access to the pathlib
module. Now what do you do with the pathlib
module?
00:32
Primarily you’re going to work with Path
objects. Those are objects that represent a file path on your operating system, and you can create them in three different ways.
00:43
You can pass just directly a string in there that represents the file path, you can use Path.home()
or Path.cwd()
, which stands for current working directory, or you can use the /
(forward slash) operator as well as another class method called joinpath()
, which is equivalent to using the /
operator.
01:06 You’ll look at all three of these options in the coming lessons, and let’s start with the one that might look the most straightforward, which is creating it from a string.
01:18
I’m here on a macOS operating system. Let’s say I want to represent a path that looks like that, so it goes /Users/Martin/
, which is my username on this computer, then on to the Desktop/
and finally a file called hello.txt
.
01:37
This is the path I want to represent. Now, the quickest way to create a Path
object for this path is to pass in this value as a string. pathlib.path
… and then I create a string, paste what I wrote up there in the comment, close the parentheses, and press Enter.
01:59
And you can see that this returns a Path
object, just has the value of this specific path. Now you can see that it says PosixPath
here instead of just saying Path
.
02:09
And the reason for that is that I am on a macOS operating system. That is a Unix-based system, like also Linux systems are. This is why it says PosixPath
. If you were on a Windows computer, you’d get something like WindowsPath
prepended to all of the paths that you create on that system. Otherwise, it looks pretty similar. However, if you are on a Windows system, then you might run into a problem.
02:37
Windows paths use \
characters instead of /
characters to represent paths, and the \
character in Python has a special meaning because it starts an escape sequence, which means that if you pass just a plain string with a \
, then Python is going to not interpret the \
character as a \
character but thinks that you want to start an escape sequence.
03:01
This will break your Windows paths if you don’t account for it, and you’ll get syntax errors. There are two ways around it. You can either use a /
instead, which means you can just represent a Windows path with the normal C:
, but and then instead of using a \
like you normally would, you just use a /
instead.
03:24
You can do that and the pathlib
library knows what to do with that, and then correctly creates a Path
object that points to this specific path.
03:33
Another way of doing it is to use raw string, and you can do that in Python by prefixing the string with just the r
character, so you put this r
in front of the string, and then you can use \
characters like you would normally with a Windows path.
03:50 Keep in mind that this is only relevant for Windows because on Unix systems like macOS or Linux, the paths anyways use this forward slash, and that’s not reserved character for Python.
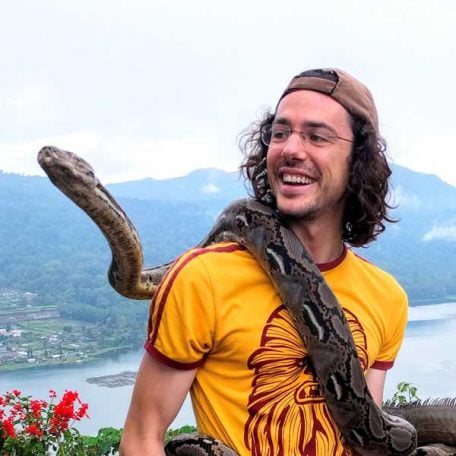
Martin Breuss RP Team on Aug. 31, 2023
@Camille it looks like you accidentally left a whitespace between the r
and the beginning of your path string in the first example. If you remove that space, then your first example works:
>>> chal_path = Path(r"C:\Users\Camille\Documents\pythonWork\python-basics-exercises-master\python-basics-exercises-master\ch12-file-input-and-output")
PosixPath('C:\\Users\\Camille\\Documents\\pythonWork\\python-basics-exercises-master\\python-basics-exercises-master\\ch12-file-input-and-output')
I’m on macOS, so the output is a bit different, but it should work for you as well.
The second example you shared is interesting. Here you escaped the closing double-quote character ("
). \
is an escape character. The r
flag indicates a raw string which means that backslash characters inside the string will just be treated like backslash characters and not escape the following character.
However, if you use a backslash just before the closing quote character, then it gets escaped! That’s why Python throws the SyntaxError
— you never closed the path string.
Hope that helps clear up what happened there! :)
Become a Member to join the conversation.
Camille K on Aug. 31, 2023
r string didnt work for me? what am i doing wrong?
GOT THIS THE FIRST TIME:
GOT THIS THE SECOND TIME when i added another
"\"
:TRIED IT with the forward slashes and it worked fine:
Thank you, Camille