Classes in Python
In this video, you’ll learn what Python classes are and how we use them.
Classes define a type. You’ve probably worked with built-in types like int
and list
.
Once we have our class, we can instantiate it to create a new object from that class. We say the new object has the type of the class it was instantiated from.
We can create multiple objects from the same class, and each object will be unique. They will all have the same type, but they can store different values for their individual properties.
If it helps, think of classes like blueprints that define how an object should be built. Where might we use classes and objects in real software?
00:01 Welcome back to our video series on object-oriented programming in Python. In the last video, we learned what object-oriented programming is and what problem it solves.
00:10
Now, let’s take a look at how we can define our own objects in our Python programs. To create our own objects, we use Python classes. Classes are used to create objects—practically as many unique objects as we want. They also define a type. For example, take a look at this code: my_name = 'Austin'
. Here, we create a new variable called my_name
with a value of 'Austin'
. Behind
00:40
the scenes, this variable is actually referencing an object. The type of the object is str
, short for string, which is a built-in Python data type that you’ve probably used before.
00:53
Python defines a string as a class, and in order to create this object of type str
, we instantiated the str
class. To instantiate a class, or to create an instance
01:05 of a class, just means to create an object from that class. You’ll hear the word instantiation thrown around a lot in programming, so just remember: it means to take a class and create an object from that class.
01:20 To make this a little bit more clear, I’m going to use one of my favorite analogies. Let’s say we are writing a program that creates doors. We’ll start by creating a class for a single door.
01:32
This class, here, is like the blueprint from which we will build all of our Door
objects. The class will specify some properties and behaviors.
01:41
Remember, each of our Door
objects will have these, and they’ll be independent to each Door
object. A door could have properties like height, paint color, and whether or not it’s currently locked.
01:53
It could also have behaviors like open, close, and toggle lock. We’ve defined a Door
class, but we’ve also just defined a new type we can use in our program.
02:04
Let’s create a Door
object with a height of 85 inches, painted red, and we’ll say that when we build it, it’s unlocked. Here, we’ve just instantiated the Door
class.
02:16
Remember, that means that we’ve created an object of type Door
from our Door
class. And we aren’t limited to creating just one.
02:25
Just like how in the real world we can build lots of doors from a single door blueprint, we can instantiate many Door
objects from our single Door
class. Here, I’ve instantiated one more. Notice that each of these Door
objects is unique.
02:40
They both have different heights and colors, and each one can either be locked or unlocked at any time. We can change the paint color of a single Door
and that doesn’t affect the rest.
02:52 These objects are all independent and they don’t rely on the class anymore. The class just tells Python how to create the objects.
03:01 Here’s a question: can you think of any scenarios where classes and objects could be used in real-world programs? In the next video, I’ll give you some examples, and then we’ll learn about how classes are actually built in Python.
Mejato on Feb. 11, 2020
Great OOP intro!
Julie Myers on Aug. 20, 2020
Question
When you create an object, are you creating copies of each method or just pointing back to the methods that exist in the class? If I were to create 5 door objects, that would be 5 copies of each method.
Austin Cepalia RP Team on Aug. 21, 2020
@Julie Myers You are creating copies of each method, but they will all behave the same because they are instantiated from the same class. Class methods are used to modify the individual state (like instance attributes) of each object.
manojkumarsk on Oct. 22, 2020
What is the advantage of using class? What problem is it solving?
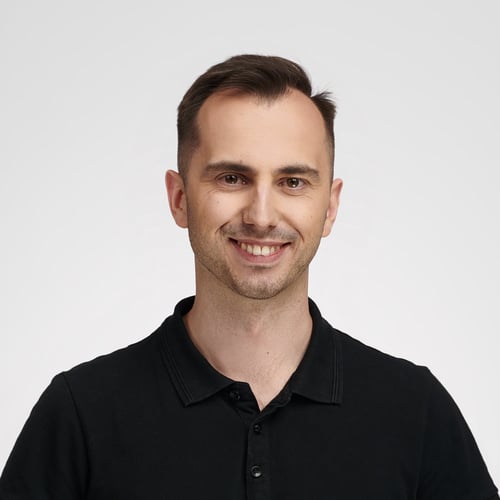
Bartosz Zaczyński RP Team on Oct. 23, 2020
@manojkumarsk That’s a good question! Classes don’t solve any problem that couldn’t be solved without them. They’re only a way to organize your code into a more convenient structure. They let you hide the implementation details and express your code more abstractly, gaining readability, testability, and maintainability. Classes are reusable, which means you don’t need to repeat yourself. Finally, they combine data and behavior, which is arguably more intuitive to think about.
manojkumarsk on Oct. 23, 2020
Thank you . I am a Network Engineer trying to use Python to automate repeated network tasks. I see a lot of Class being used hence asked the question. Thanks for Answering the question.
anaghost on Nov. 2, 2021
It’s all great as always BUT could you please record videos with a darker background similar to the one in these videos realpython.com/lessons/pythonic-loops-overview/ ? We spend a lot of time looking at the screen and a white background is hard for a lot of people I know including myself. That would be much appreciated!
Become a Member to join the conversation.
kwf777 on Nov. 7, 2019
Great course and easy to follow and understand.