FastAPI PUT and DELETE Requests
00:00 In this lesson, you’ll learn how to correct the number of sides in the dodecagon shape using your RestAPI, and you’ll also use the API to delete documents too. According to the REST standard, updates should be done using requests with the PUT HTTP method.
00:17
Just like with GET and POST, there’s a decorator for that. Since you want to update a specific document, the endpoint includes a shape_id
variable that identifies the document requiring the update. In addition, you’ll need to supply some data in the @put
body telling Mongita how to update the document. Therefore, the handler function for the PUT request will accept a Shape
model class.
00:42
The function signature looks like this. Inside of the update_shape
body, you should first check to see if a document with the ID exists. The count_documents()
method of the collection will return the number of documents meeting the criteria of a filter document. The filter document to find a document with a specific ID looks like this.
01:06
Instead of updating specific fields, for brevity you’ll replace the entire document with a new one. The result will be the same. The .replace_one()
method of the collection accepts the filter document, which again will look for documents with a specific ID and select the first.
01:23
The second value is the replacement document, which is the new shape. Remember to call the .dict()
method on the shape object. And finally return that shape.
01:36
If there is no document with the shape_id
, raise an HTTPException
with the status code 404
for “not found.”
01:52 There is another option for the update. Mongita supports the idea of an upsert. In other words, if an update is attempted for a document that does not exist, the replacement document will be created automatically.
02:04
Thus, upsert is a portmanteau of “update” and “insert.” To perform the upsert, add the upsert
keyword argument to the .replace_one()
method with a value of true
.
02:20 You can now remove the code to check if the document exists.
02:38
Head back to the browser and refresh the documentation. Look for the PUT method to update the shape. Click the Try it out button and fill out the JSON, this time with the number of sides being 12
.
02:59 Click the Execute button and see the returned shape with the correct number of sides this time. Go to the GET method for all shapes, execute it, and see that the change has been committed and the database reflects the dodecagon with twelve sides.
03:22
Just one more method left to finish the API: the DELETE method. Again, there is a @delete
decorator that takes a path.
03:39
Use the .delete_one
method of the shapes
collection to attempt to delete the shape. This method just accepts a filter document.
03:48
The .delete_one()
method returns a delete result with a deleted_count
property. The deleted_count
is the number of documents removed, if any. Thus, if no document with the shape_id
exists, the deleted_count
is 0
and no error is raised, so you could stop here and return a value indicating that there was no error.
04:07
Or you could check the deleted_count
and raise 404
if no shapes were found.
04:19
Refresh the docs in the browser. Look for the DELETE method, click the Try it out button, and enter a shape_id
of 2
for the dodecagon.
04:30
Click the Execute button and see the status of the request is 200
, and the returned JSON indicates the delete was completed. Go back to the GET method to list all shapes. Click the Try it out button. Click the Execute button.
04:51 And the dodecagon has been removed. That’s it. Your API is complete. In the last lesson of the course, you’ll review what you have learned.
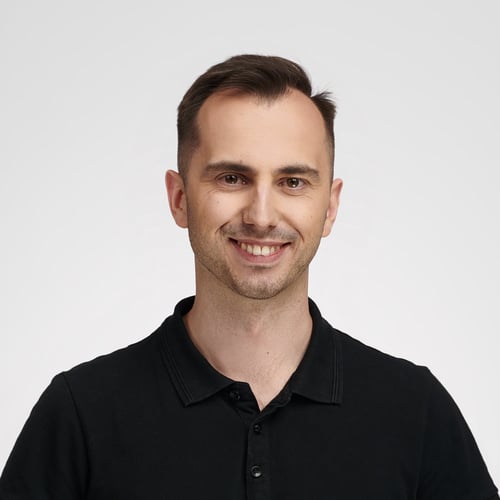
Bartosz Zaczyński RP Team on July 25, 2022
@oholl It’s an object-oriented representation of the HTTP request’s payload or message body, which FastAPI deserializes from JSON and injects into the function. If you display the auto-generated documentation at 127.0.0.1:8000/docs, and scroll down to that endpoint, then you’ll see an example payload.
You can send such a PUT request using cURL in your commandline, for example:
$ curl -X PUT http://127.0.0.1:8000/shapes/42 -H 'Content-Type: application/json' --data '{"name": "square", "no_of_sides": 4, "id": 42}'
chelseasablich on Aug. 29, 2022
Hello all,
Nice tutorial - thanks. Wondering if there are any FastAPI tools to help stop duplication - currently I could create many shapes with an id of 1. A check could be implemented in the API to see if any other shape object already have an id 1 but is there any feature in the Pydantic model, or similar, which could be implemented to help this?
Thanks
danilolimadutra on March 17, 2024
@chelseasablich
As far I could understand, Mongita is a lightweight, serverless, file-based, single-user Python database library that implements a subset of the MongoDB/PyMongo interface. However, it does not support all MongoDB features. Mongita does not support the unique argument in the create_index method, which is used to enforce uniqueness of a field in MongoDB.
If you need to enforce uniqueness of the id field in your Shape model, you’ll need to handle it manually in your application code. For example, before inserting a new shape, you could check if a shape with the same id already exists:
@app.post("/shapes")
async def create_shape(shape: Shape):
"""Create a new shape."""
if shapes.count_documents({"id": shape.id}) > 0:
raise HTTPException(status_code=400, detail=f"Shape with id {shape.id} already exists")
shapes.insert_one(shape.model_dump())
return shape
Become a Member to join the conversation.
oholl on July 21, 2022
Hi, where does the second argument
shape
in update_shape come from?