For more information on concepts covered in this course, please check out:
Getting to Know Pygame
00:00 In the previous lesson, I gave an overview of the course. In this lesson, I’m going to show you how to get started with Pygame, drawing simple shapes to the screen and animating them.
00:11 Pygame is a third-party library and as such, needs to be installed. It is recommended that whenever you install libraries, you use a virtual environment.
00:19
There are links in the notes below that can show you how to do that if you haven’t done it before. With your virtual environment activated, run the pip install
command to download and install Pygame.
00:34 Video games are structured around a central game loop. Inside of each iteration of the game loop, you handle any input from the user or outside events such as the network, determine where your moveable pieces are in the game, and draw all the things visible to the user onto the screen, rendering a single frame of the game.
00:55 Like movies, games are made up of a series of frames shown to the screen in rapid succession. To get the appearance of fluid motion, you want at least 25 frames per second, also known as FPS.
01:10 Modern gaming hardware often goes way past this minimum, with high fidelity games routinely using 60 frames per second.
01:18 In order to achieve the goal of 25 FPS, you’ll first have to draw a frame. A frame is just an image shown to the screen, albeit for a very short time. Graphic systems use a Cartesian coordinate system to describe where things are in the frame.
01:34
This means the frame is described with an x- and y-axis, with each pixel being described by an (x, y)
tuple called a point.
01:45
Different graphics libraries treat the coordinate system differently. Instead of using an origin in the center of the screen like your algebra class, Pygame uses the top left-hand corner as (0, 0)
.
01:56
In order to draw this circle halfway across and a quarter of the way down, you describe it as 200
on the x-axis and 75
on the y-axis. The r
parameter to the circle()
function specifies the radius.
02:12
An r
of 15
will give you a circle with a diameter of 30 pixels. For this sample screen size of 400 wide and 300 tall, (200, 75)
gives you the circle you see here.
02:26 Not all graphics systems use the top-left corner as the origin. This means when loading images in, you may have to translate coordinate systems. Another peculiar side effect of this origin is that moving up on the screen is a negative direction. You’ll see more on this later.
02:45 Now that you’re oriented and you’ve seen a circle, let’s go write some code.
02:51
Here’s the code for your first “game.” Did you hear the air quotes around that word? To get started, you’ll need to import the pygame
library and initialize it.
03:00
The init()
call on line 5 gets Pygame set up. On line 6, I’m setting the screen’s title, and on line 7, I tell Pygame how big the screen will be. In this case, I’ve chosen 800 pixels wide and 600 pixels tall. Note that the set_mode()
method takes a tuple specifying the dimensions, rather than two parameters.
03:25
The set_mode()
method returns a surface. Surfaces are where you can draw things. In this case, the surface is the main screen of the program.
03:34
I’m storing this away in a variable called screen
so that I can draw to this surface later.
03:41
I mentioned that games have a game loop, and here is yours. This while
loop loops forever, or until someone trips the quit condition. Inside this loop, you normally handle any user interaction, the calculation of the positions of objects, and the rendering of a frame.
03:59 Once all that is done, you start the next loop.
04:03
In this first program, the only user interaction is handling the QUIT
event. This inner loop on line 10 goes through all of the events that have happened since the last iteration of the game loop. For this simple program, the only event you are interested in is if the close button on the app has been pressed.
04:23
Line 14 fills in the background of the screen by calling the .fill()
method on the screen
surface, .fill()
takes an RGB tuple that specifies the color to fill with.
04:34
In this case, it is a shade of blue. Were you expecting something different in a program named blue
?
04:42
Lines 15 through 17 are about positioning the objects to be drawn. In this case, the only thing being positioned is a single circle. I’m randomly choosing an x
, y
, and radius value for the circle. Note the choice of the range of values for x
and y
.
04:59
The radius of the circle is always between 2 and 10, so the ranges of x
and y
are chosen so that the circle always appears on the screen. With appropriate random values selected, line 18 draws the actual circle.
05:15
The pygame.draw
module contains many functions for drawing lines and shapes. The circle()
function takes a few parameters. The first is a surface to draw on. This is the background screen.
05:28
The second, a color to draw with, once again an RGB value for a different shade of—well, what else?—blue. The third is an (x, y)
tuple representing the point where to draw the circle.
05:40 And finally, the radius of the circle that needs to be drawn.
05:45
The last line here calls display.flip()
. The drawing that has been happening so far hasn’t actually been drawn to the screen, but to a buffer.
05:54 You want to do things this way so that everything is shown on the screen at the same time. If you’re drawing lots of objects, you don’t want them to blink in and out as each is created.
06:04
This flip()
method takes everything in the surface buffer and updates the screen with a copy of the buffer all at once. Let’s see all of this in action.
06:20 This seizure-inducing display is your first Pygame “game.” A nice, pretty shade of sky blue is filled into the screen, than a navy blue circle is drawn randomly.
06:32 The game loop continues, doing this over and over. The quick speckling you see here gives you an idea of how many frames your program is spitting out per second.
06:42 Later on, I’ll show you how to control that frame rate, but for now, you can play around. Change the circles, dig into Pygame docs, and get fancy. See if you can add a rectangle. Call of Duty it is not, but everyone has to start somewhere. Next up, you’ll write Call of Duty. No, wait. No, you won’t.
07:03 You’ll see how to get ready for larger programs and how to organize your code.
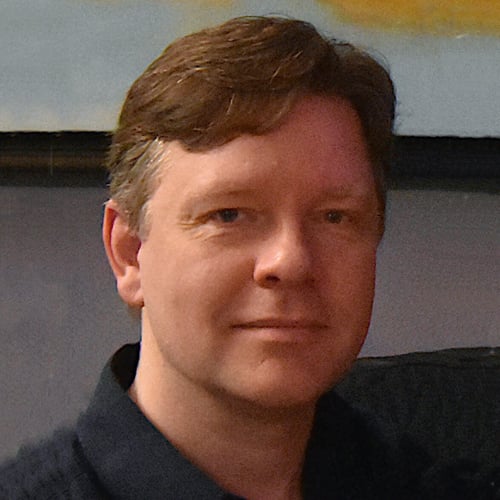
Christopher Trudeau RP Team on Nov. 17, 2022
Hi @info97,
No, checking the events isn’t mandatory. For example, I tested this with the event part of “blue.py” commented out:
# blue.py
import pygame
import random
pygame.init()
pygame.display.set_caption("Kinda Blue")
screen = pygame.display.set_mode((800, 600))
while True:
# for event in pygame.event.get():
# if event.type == pygame.QUIT:
# quit()
screen.fill((135, 206, 235))
x = random.randint(10, 790)
y = random.randint(10, 590)
r = random.randint(2, 10)
pygame.draw.circle(screen, (0, 0, 150), (x, y), r)
pygame.display.flip()
And except for the fact that you can’t close the window using the close icon in the top left, it works. You need to CTRL-C to get out.
I’m on an older Mac using Python 3.9 and Pygame 2.0.1. I had some trouble getting things installed when I attempted a new version, but didn’t troubleshoot it, I simply went with what I used when I wrote the course. I mention this as you may be having complications due to versions.
Try the code above and see if it works. If so, compare it against what you wrote. If not, reply back and we’ll do our best to help you work through it.
info97 on Nov. 18, 2022
Hi Christopher,
thanks for your reply. I copied the code exactly as you posted it here and the problem remains. I just get the app icon in the tray. When right clicking on it and choosing “show all windows” there is actually none. Perhaps it is version related. I am now on Python 3.11 and had to install pygame with a –pre option. (pip install pygame –pre)
Fortunately checking the events brings the window on and I can continue learning. Thanks a lot!
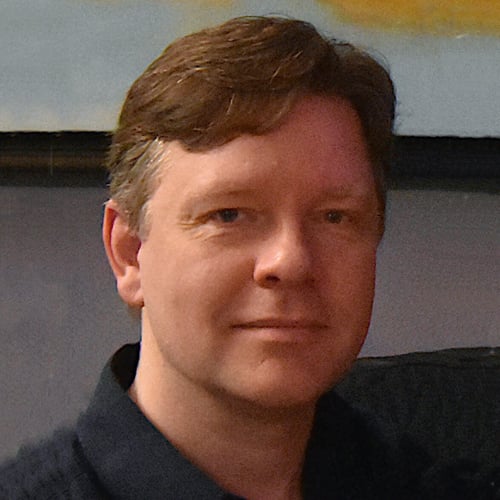
Christopher Trudeau RP Team on Nov. 18, 2022
Strange. Not sure why that’s happening. I’d be curious how much you can pare it down. If you just do a single pygame.event.get()
and do no processing on it will it work?
info97 on Nov. 21, 2022
Hi Christopher,
sorry, was away for a bit.
Yes, just the line pygame.event.get()
does it, the window appears. Commenting it out brings the strange behaviour back again…
info97 on Nov. 22, 2022
Looks like it is more an os - thing. The code you gave me runs without any problem on windows 11 with pygame 2.1.3.dev8 (SDL 2.0.22, Python 3.11.0).
Just to let you know
vulpes31415 on Jan. 7, 2023
@4:18 “if the close button on the app has been pressed”
For blue.py and space_rocks I get stuck in an infinite loop. Typing all iterations of quit do not exit the program. Is there a button or something I am missing?
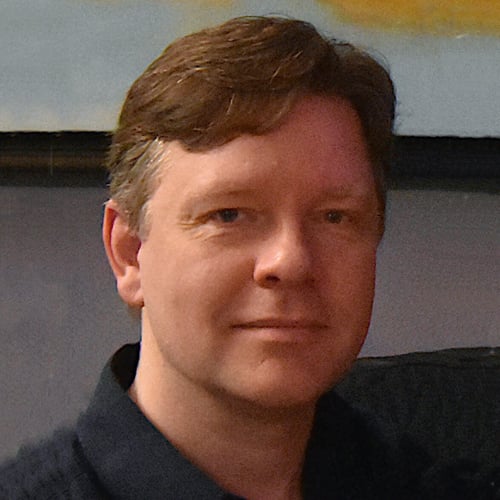
Christopher Trudeau RP Team on Jan. 8, 2023
Hi @vulpes31415,
The event loop that checks for pygame.event.QUIT
is what responds to the close button. Even without that, CTRL-C in the terminal should close your program.
As you might see from comments above, there appear some OS/version specific times where that event loop is problematic. Depending on your situation you may need to stick with CTRL-C.
Become a Member to join the conversation.
info97 on Nov. 16, 2022
Hi there,
is checking the events mandatory? Whenever I delete this piece of code I get no error, but no screen is shown, just the icon in the tray. -> Mac M1 OS Monterey, Python 3.6.9, Pygame 2.1.2