Logging In and Out
00:00
Logging In and Out. As you saw in the last section, Django will try to use a template called registration/login.html
, which currently doesn’t exist, leading to the error that you saw.
00:15
Go ahead and create it using the code seen on-screen to display a Login
heading, followed by login form.
00:39
The {% csrf_token %}
line inserts a cross-site request forgery (CSRF) token, which is required by every Django form to help ensure security.
00:51
Django uses a dictionary, also known as a context, to pass data to a template while rendering it. In this case, a variable called form
will already be included in the context.
01:02
All you need to do is display it. Using {{ form.as_p }}
will render the form as a series of HTML paragraphs. This makes it look a bit nicer than just using {{ form }}
.
01:18 Finally, there’s a button for submitting the form and, at the end of the template, a link that will take your users back to the dashboard.
01:30 Head back to your browser and refresh the login page. It should now appear in a basic form with the Username and Password fields present, along with the Login button.
01:42 You can further improve the looks of the form by adding a small CSS script to the base template, as seen on-screen.
02:01 By adding the code you’ve just seen to the base template, you’ll improve the looks of all of your forms, not just the one in the dashboard. Refreshing the page once more, you should see a login page with improved appearance.
02:18
Use the credentials of your admin user and press Login, but don’t be alarmed if you see an error, as you’ll see on-screen. According to the error message, Django can’t find a path for accounts/profile/
, which is the default destination for your users after a successful login.
02:37 Instead of creating a new view, it makes much more sense to reuse the dashboard view here. Fortunately, Django makes it easy to change the default redirection.
02:48 All you need to do is add one line at the end of the settings file.
02:59 Try to log in again, and this time, you should be redirected to the dashboard without any errors. Now your users can log in, but it’s probably a good idea to let them log out as well.
03:12
This process is more straightforward because there’s no form. They just need to click a link. After that, Django will redirect your users to accounts/logout/
and will try to use a template called registration/logged_out.html
.
03:29 However, just like before, you can change that redirection. For example, it would make sense to redirect your users back to the dashboard. As you may already have guessed, all you need to do is add one line at the end of the settings file.
03:50 Now that both login and logout are working, it’s a good idea to add proper links to your dashboard.
04:06
If a user is logged in, then user.is_authenticated
will return True
, and the dashboard will show the Logout link. If a user is not logged in, then the user variable will be set to AnonymousUser
, and user.is_authenticated
will return False
.
04:24 In that case, the Login link will be displayed. The updated dashboard should look as seen on-screen for non-authenticated users. And when you’re logged in, you should see something similar to what’s being seen on-screen now.
04:42 Congratulations, you just completed the most important part of the user management system: logging users in and out of the application. But there are a couple more steps ahead of you. So in the next section, you’ll take a look at what’s needed to allow users to manage their own passwords.
Varun Kapoor on Sept. 23, 2022
Sorry its ok my bad, I had two django projects and it was using the settings file of the project I was not adding this redirect url to. I deleted the project I did not need and then correctly pointed to the correct settings.py file in the manage.py file.
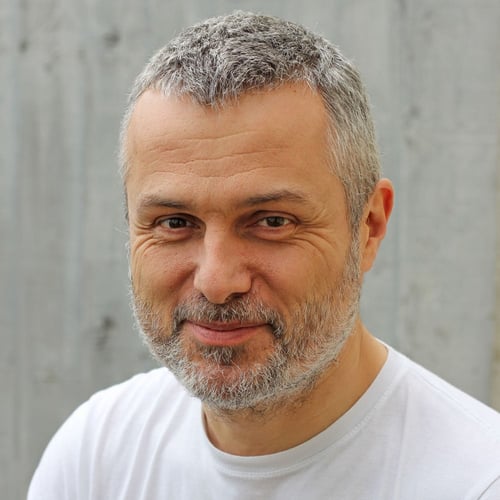
Darren Jones RP Team on Sept. 27, 2022
No problem, glad you’ve got it sorted. Everyone has done things like this!
Ann Gepulle on Oct. 12, 2023
Hi Darren, thank you for this tutorial. I am learning Django as I build a mini social app. Now I am trying to add the user management by following your tutorial. I must say that it is very clear but I keep on bumping to some challenges. For example, I am having this error:
Reverse for ‘logout’ not found. ‘logout’ is not a valid view function or pattern name.
This is after I added the
<div>
{% if user.is_authenticated %}
<a href="{% url 'logout' %}"><Logout/a>
{% else %}
<a href="{% url 'login' %}">Login</a>
{% endif %}
</div>
Login worked as I have added the below in urls.py:
path("accounts/", include("django.contrib.auth.urls")),
Please bear with me as I am learning Django. Thanks in advance.
Ann Gepulle on Oct. 12, 2023
Hello again, Darren. Got it! I found a syntax error on my dashboard.html
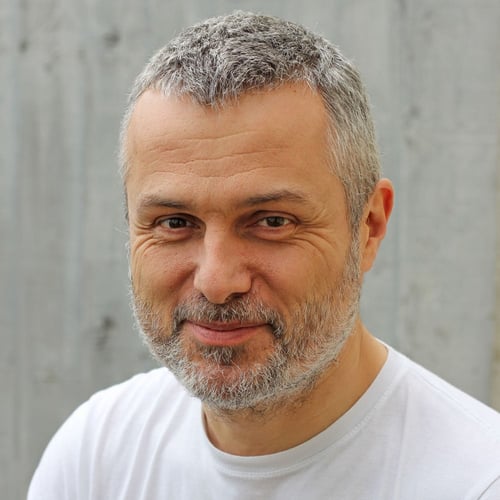
Darren Jones RP Team on Oct. 17, 2023
Hi Ann! Well done for sticking at it and finding the error. It’s a hugely important (and often overlooked!) skill to find issues like this. I’ve spent literally hours finding similar problems and often it’s a case of “Oh, it was just that that did it!!!”
khushboo gupta on Feb. 2, 2024
hi * i wanna ask you that in vedio you just put accounts/login but not render in views.py how the site know that template exists?
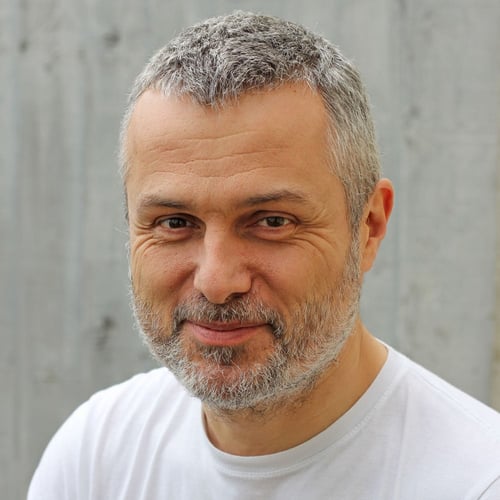
Darren Jones RP Team on Feb. 7, 2024
@khushboo gupta - This is making use of Django’s built-in authentication - django.contrib.auth.urls - as seen in the previous chapter at around 0:45. You’ll see a list of URLs that this provides and you just need to add the templates at the locations that auth.urls is expecting, and you’re good to go.
nk095291 on Feb. 19, 2024
I’m getting following error when trying to get log out.
Method Not Allowed (GET): /accounts/logout/
Method Not Allowed: /accounts/logout/
[19/Feb/2024 23:56:05] "GET /accounts/logout/ HTTP/1.1" 405
I changed the code to this and it worked,
{% if user.is_authenticated %}
<form action="{% url 'logout' %}" method="post">
{% csrf_token %}
<button type="submit">Logout</button>
</form>
{% else %}
<a href="{% url 'login' %}">Login</a>
{% endif %}
just want to know why I’m the only one having this issue, is it because of different version of Django or Python?
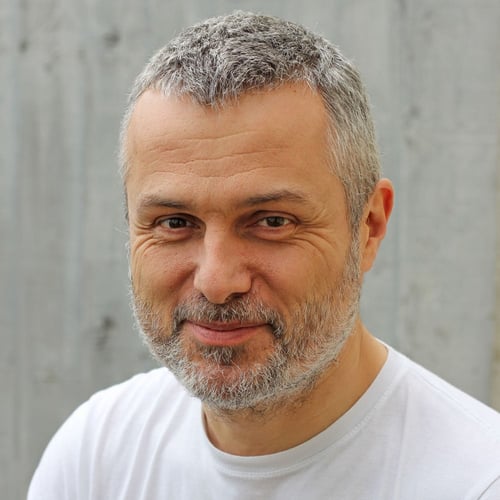
Darren Jones RP Team on Feb. 20, 2024
@nk095291 - This is due to changes made in Django 5.0 (which I would guess you are running) - [1]. Logging out on Django >= 5.0 requires a POST, and the code you have put here is what’s now required - a POST with a CSRF token.
I shall make a note of this and look to update the course in the future to reflect this change. Thanks for taking the time to post (no pun intended!)
[1] - docs.djangoproject.com/en/dev/releases/5.0/#deprecated-features-5-0
Become a Member to join the conversation.
Varun Kapoor on Sept. 23, 2022
This did not work for me: LOGIN_REDIRECT_URL = “dashboard” do I have to also update the project urls file for this to work? Sorry I must have missed that part.