Officially, variable names in Python can be any length and can consist of uppercase and lowercase letters (A-Z
, a-z
), digits (0-9
), and the underscore character (_
). An additional restriction is that, although a variable name can contain digits, the first character of a variable name can’t be a digit.
Naming Conventions
00:00 Now that you know what variables are and that they’re pointers to objects, in this section, you’re going to take a look at the different variable names that you can use.
00:08 What are the conventions? And we’re also going to take a quick look at PEP 8. We are at section 4 of our course, talking about variable names. And as I mentioned, we’re going to first look at the naming conventions and then peek into PEP 8.
00:22 I’m going to talk about what that is and why you don’t have to be worried about it in just a second after this first section. Let’s get started. So, variable naming conventions.
00:33 You can name things in many ways, okay? And Python is aware of that. But there are still some suggestions on what you should do in order to keep it as structured and as compatible with other code as possible.
00:47
One thing that’s not really a restriction is that a variable in Python can be of any length. So we looked before at a very short one, n = 300
.
00:57
Our variable name here is n
. It’s very short, it’s just one letter. And that’s perfectly fine. At the same time, it could also be this_is_a_really_really_really_long_name
equals, well, let’s call it 400
. So this is fine, right?
01:18 It’s going to be pretty annoying if you have to type this out every time that you want to print it, so make sure that you use tab completion as I did just now.
01:26 But it’s no problem for Python. It’s still the same thing as just assigning it a single letter. The length doesn’t matter.
01:35 Next thing, Python variables can be uppercase and lowercase characters or a mix of those too. There is a caveat to this. There’s certain restrictions on when you’re meant to use what.
01:47 Like, they have a certain meaning, but it’s not going to be an error if you use an uppercase or a lowercase variable. For example, we already did lowercase up here. This is all lowercase as well.
02:00
Now I can do an uppercase one. I can say CONSTANT = 42
.
02:13 And with autocompletion, you see Python is not complaining using this as a normal variable name. The convention with uppercase is that it should refer to constants, so things that don’t really change. But that’s just as a side note.
02:29
So, we’re using here lowercase, we’re using uppercase. I could do a mix, I could say MIXmeNOT
, ha, and that’s fine for Python. It’s not complaining.
02:44
Ah, so, if can type it at all, ha. Okay, there you go. But you want to avoid that. The general way that a variable should look like in Python is using underscores (_
) and lowercase characters for most of the names that you’re going to be using. Let’s take a look.
03:03 Here’s an example already. It’s not a great variable name because it’s not very descriptive at all for what the value it is pointing to. But in terms of the formatting, I’m using lowercase and underscores. This is what you want to do.
03:17 Avoid using stuff like that, it just makes it more difficult to read. While it’s not going to produce an error for you—it’s still works—Python is discouraging you from using something like that.
03:27
So a good variable name would be, for example, num_videos = 10
. So that’s good, it’s readable. Let’s clear that up.
03:41 This is a good variable name. It’s easily readable, it’s clear what it’s about, it’s descriptive of the value that it points to. Let’s say at the beginning, when you’re starting your programming journey with Python at the beginning, in 80% of the cases you’re going to be fine if you default to a variable naming scheme like that. You use lowercase letters, you use underscores to separate different words, and you try to be descriptive.
04:08 So, this also shows us underscores are fine in a variable name. What else? We can use digits in our variable names, but not at the beginning. So, while this works…
04:24
Not a problem. It’s all fine. I cannot say… For example, this is going to be an error. You see? We’re getting a SyntaxError
, which just tells us in Python that our variable name cannot start with a number.
04:41 Numbers are fine. They can be part of a variable name, they can be at the end or somewhere in the middle.
04:55 Not a problem. But not at the beginning.
05:02 And finally, something that’s new with Python 3, you can use Unicode characters for your variable names as well. So what we can do now, finally, is we can assign variable names like this.
05:14
I can say voilà
! Let’s write it correctly, like this.
05:23
"FRANCE"
! And Python’s fine with that. Using a Unicode character like this à
with the accent on it would not have worked in Python 2.
05:34 That’s a new thing that works with Python 3, but now you can use it as parts of variable names and that’s perfectly fine. It opens it up to a couple more languages that might not have been able to be programmed in using the characters that they use normally in their language.
05:48 So, that’s a great improvement in Python 3, and just another reason for using Python 3.
05:56 All right, so these are the variable name conventions. We just went over them quickly now. And we’re going to do a recap in just a second. But first, in the next video, I want to show you PEP 8, which is a document where all of these conventions are written down and where you can always go back to check on them. See you there in the next video.
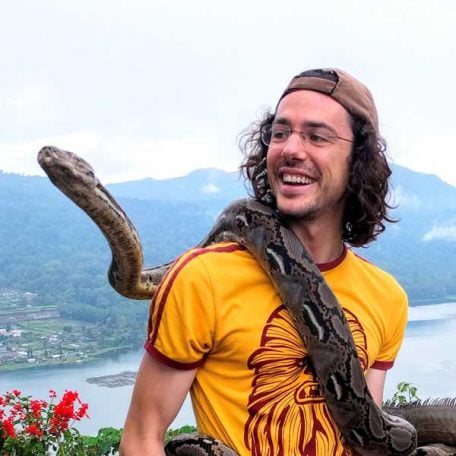
Martin Breuss RP Team on April 7, 2020
Hello @jasonwilliams78, I am not quite understanding what you’re asking. I think you are referring to the previous video about the Python Pub Quiz?
a, b = 250, 250
for _ in range(250, 260):
if a is not b:
break
a += 1
b += 1
print(a)
Both a
and b
are being incremented in this for
loop, as long as they have the same id()
. As soon as they refer to two different objects, the if
statement catches and the loop break
s, which means they don’t get incremented anymore.
However, a
should not be 250
at the output. Maybe you have a bug somewhere in your code?
jasonwilliams78 on April 8, 2020
thanks Martin!, I understand now. But yes I have 250 for the output. Using Jupyter notebook. Will check over it again
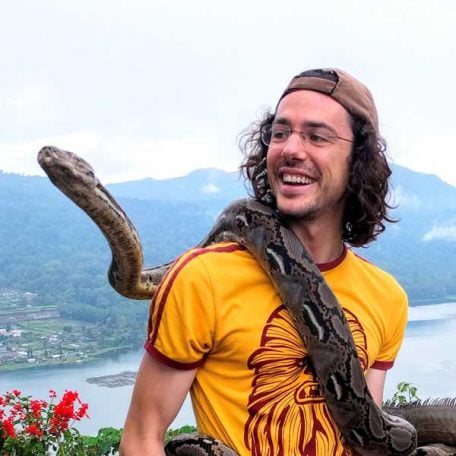
Martin Breuss RP Team on April 9, 2020
I tested the code in a Jupyter notebook, since who knows, maybe notebooks handle caching differently, but my output is as expected.
Take a look at your indentation and make sure that it matches the example above.
If it still shows 250
as output, maybe you can publish your notebook on GitHub so I can give your code a tryout.
jasonwilliams78 on April 10, 2020
I figured out why the output wasn’t accurate. I had the a,b incrementors set inside the local variable namespace. Once I moved them up one level to the for loop scope I they were both able to increment correctly.
a, b = 250, 250
for i in range(250,260):
if a != b:
break
a += 1
b += 1
print(a,b)
260 260
jasonwilliams78 on April 10, 2020
Good evening, Martin. Can you tell me if I have a valid solution to this. My code is below the instructions.
1. Create a tuple data with two values. The first value should be the tuple (1, 2) and the second value should be the tuple (3, 4). 2. Write a for loop that loops over data and prints the sum of each nested tuple. The output should look like this: Row 1 sum: 3 Row 2 sum: 7data = ((1,2),(3,4)) for i in data: i = sum(data[0]) i = sum(data[1]) print(f”row 1 sum: {row1}”) print(f”row 2 sum: {row2}”)
jasonwilliams78 on April 10, 2020
data = ((1,2),(3,4))
for i in data:
i = sum(data[0])
i = sum(data[1])
print(f"row 1 sum: {row1}")
print(f"row 2 sum: {row2}")
Ganesh Kadam on May 1, 2020
a , b = 250, 250
for i in range(250,260): if a is not b: break a += 1 b += 1
print(a)
My output is : 257
Could someone please help here? Am I missing something?
Ganesh Kadam on May 1, 2020
a , b = 250, 250 print(id(a)) print(id(b))
print(a is b)
for i in range(250,260): if a is not b: break a += 1 b += 1
print(a)
Output:./test.py 139706676028128 139706676028128 True 257
Am I doing this right?
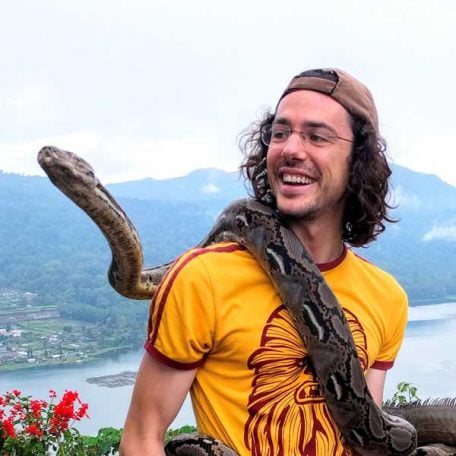
Martin Breuss RP Team on May 2, 2020
You’re doing it right @Ganesh. :)
kamjag on May 17, 2020
Hello @Martin Breuss I am really new in Python, When I run the below steps : a, b = 250, 250 print(id(a)) print(id(b)) for _ in range(250,260): if a is not b: break a += 1 b += 1 print(a)
OUTPUT is : 251
BUT when I run below steps: a , b = 250, 250 print(id(a)) print(id(b))
for _ in range(250,260): if a is not b: break a += 1 b += 1
print(a)
OUTPUT is : 257
Why am I getting diffrent results when my both steps are almost same except indentation in second steps in a += 1 b += 1
Kindly guide. Thanks in advance.
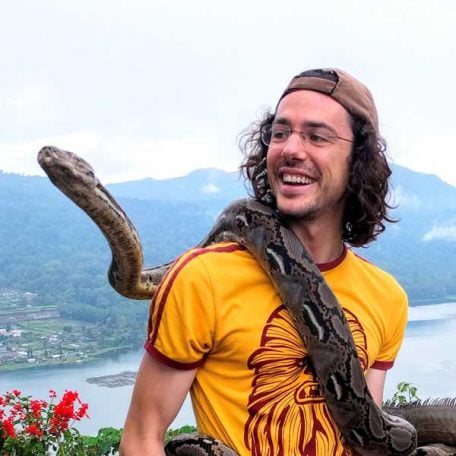
Martin Breuss RP Team on May 17, 2020
Hello @kamjag. It’s difficult to tell since your code formatting isn’t clear. You can use triple-backticks for proper code formatting, like so:
def hello():
print("hello")
If you post it again with proper formatting, I can take a closer look. But one thing to note is that indentation has meaning in Python. So whether a line of code is indented or not will make a difference in your code. Hope that helps!
Ajay on May 25, 2020
Got answer as 257 and know the exact reason behind this. Thanks martin for wonderful explaination.
a, b = 250, 250
for _ in range(250, 258):
print (f"Value of a {a}")
print (f"ID of a {id(a)}")
print (f"Value of a {b}")
print (f"ID of a {id(b)}")
if a is not b:
print (f"Value of a {a}")
print (f"Value of a {b}")
break
a += 1
b += 1
print (a)
**# Output
Value of a 250 ID of a 1385560464 Value of a 250 ID of a 1385560464 Value of a 251 ID of a 1385560480 Value of a 251 ID of a 1385560480 Value of a 252 ID of a 1385560496 Value of a 252 ID of a 1385560496 Value of a 253 ID of a 1385560512 Value of a 253 ID of a 1385560512 Value of a 254 ID of a 1385560528 Value of a 254 ID of a 1385560528 Value of a 255 ID of a 1385560544 Value of a 255 ID of a 1385560544 Value of a 256 ID of a 1385560560 Value of a 256 ID of a 1385560560 Value of a 257 ID of a 56217840 Value of a 257 ID of a 64785344 Value of a 257 Value of a 257 257**abp on April 23, 2021
a = 300
b = a
print(id(a), id(b))
Only if I use different values for both the variables, I get different memory addresses for them. What is the issue here?
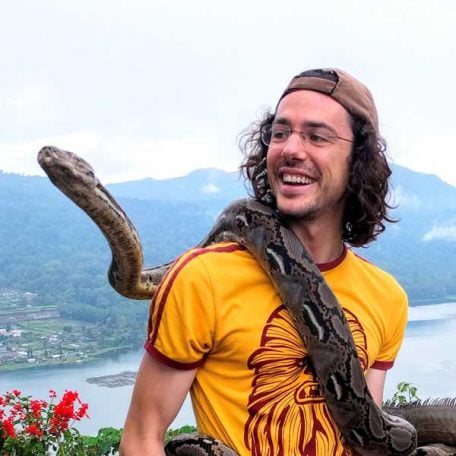
Martin Breuss RP Team on April 24, 2021
Hi @abp! In this case you’re literally making another reference to the same object. You’re saying something like:
a
points to the integer object with the value300
.b
points toa
, which points to the integer object with the value300
.
Or, for a kinda textual-visual representation:
b
==>a
==>300
tl;dr: You’re only creating one object, and pointing two variables to it.
Uncle Den on May 26, 2025
I am not sure if something changed as I am running python 3.13 but the output of this is not as expected per this course
a = 25
b = 25
c = 4556
d = 4556
print(a == b)
print(id(a) == id(b))
print(id(c))
print(id(d))
print(c == d)
print(id(c) == id(d))
output
True
True
2354073722160
2354073722160
True
True
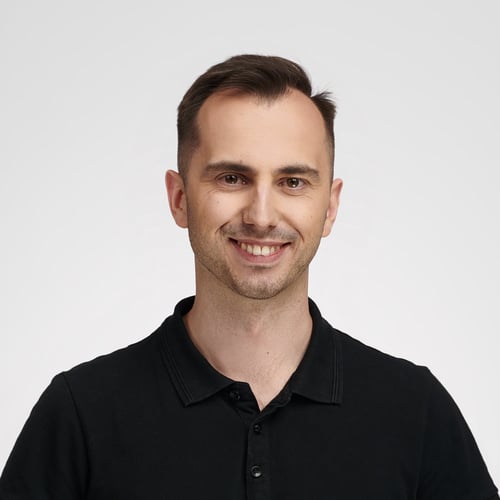
Bartosz Zaczyński RP Team on May 27, 2025
@Uncle Den This will depend on whether you’re running this code in the Python REPL or as a standalone Python script.
If you run this code from a Python script rather than an interactive REPL session, then you may observe a different behavior. That’s because Python cuts corners by caching repeated values when it finds them in your source file. On the other hand, the interpreter can’t make any assumptions upfront when you’re typing code interactively in the REPL.
(There are a few more subtle details that happen behind the scenes that I omitted for brevity.)
Uncle Den on May 27, 2025
Thanks, That make sense from what I have found in practice. Keep up the good work at Real Python.
Become a Member to join the conversation.
jasonwilliams78 on April 7, 2020
Hello Martin, can you please explain why we have incrementors for a and b in the for loop that they are not being incremented why ‘a’ remains at 250 for the output ?