Printing to File Streams
print()
normally prints to the <stdout>
file stream. You can change this behavior using the file
parameter. You can access the <stdout>
stream directly from the sys
library:
>>> import sys
>>> result = sys.stdout.write('hello\n')
hello
>>> result
6
You can also change where print()
prints to:
with open('file.txt', mode='w') as file_object:
print('hello world', file=file_object)
00:00
In the previous lesson, I covered sep
, end
, and flush
and how you use those arguments to change the output of print()
. In this lesson, I’ll be talking about how to print to things besides the screen. print()
doesn’t actually implement writing characters to the screen.
00:16 It’s a wrapper to code that does this work. Operating systems have three standard streams. You can think of a stream as a way for characters to go in and out of the system.
00:28
The system streams are stdin
(standard in) for reading input, stdout
(standard out) for displaying output, and stderr
(standard error) for displaying errors. In most applications,
00:39
stdout
and stderr
both go to the console screen. Inside of Python’s sys
library, you can see the streams themselves. If you’re familiar with file objects, you’ll notice the mode='r'
there is similar to when you use open()
with an 'r'
to say you want to read from a file. That’s because all of the streams are file-like objects. stdout
has mode='w'
because it’s output, and stderr
—also mode='w'
for output.
01:11
Let’s look at that inside of the REPL. Import sys
and call in the stdout
stream the .write()
method. This works just like print()
.
01:23
In fact, this is what print()
is doing. Notice that one of the differences here, though, is print()
automatically appends a '\n'
—.write()
does not, so you have to put it in there explicitly. The .write()
method returns the number of bytes that it spit out to the stream. In this case, the result is 6
. I know 'hello'
only has five characters, but that '\n'
actually counts. Five plus the one character for the '\n'
, gives us the 6
.
01:53
In addition to the other arguments, print()
also supports an argument called file
. file
takes a file-like object and wherever it points is where the resulting output goes.
02:03
It defaults to the stdout
stream. Instead of writing to a stream, you can also write to a file. Open the file in write mode, you get your file object, pass that file object in as the file
argument, and print()
is now printing to that file instead of to the screen. One word of warning though: print()
and stdout
are both meant to handle string data.
02:27 If you’re working with binary data, things go wrong here. There are other ways of writing binary content to files.
02:35
Next up, I’ll show you how to add methods to your classes to control what gets displayed when they get sent to print()
.
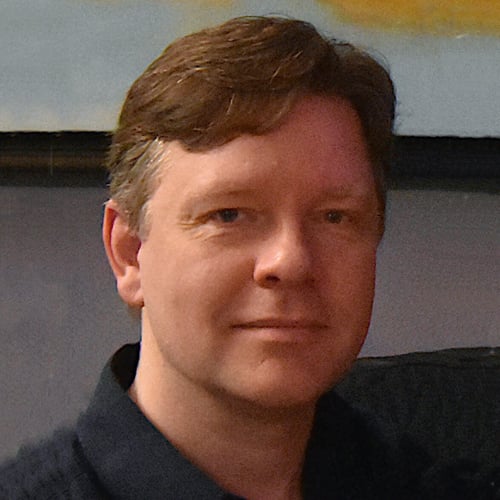
Christopher Trudeau RP Team on May 19, 2020
Not commonly. There are a couple bizarre corner cases (later in the course I mock out print and then need to print something to screen), but these are rare and far between.
Grouchy Old Fart on Sept. 29, 2023
Great course, all the way through. One minor bug that will probably not stop the world from spinning. However I am trying to figure out if I missed something or did something wrong.
When I did the:
import sys
sys.stdin
The IDLE environment returned:
<idlelib.run.StdInputFile object at 0x0000023BAE4F1A20>
Rather than the <_io.textIOWrapper...
message shown in the video.
This is on a Windows 10 machine running Python 3.11.4. Details below:
Python 3.11.4 (tags/v3.11.4:d2340ef, Jun 7 2023, 05:45:37) [MSC v.1934 64 bit (AMD64)] on win32
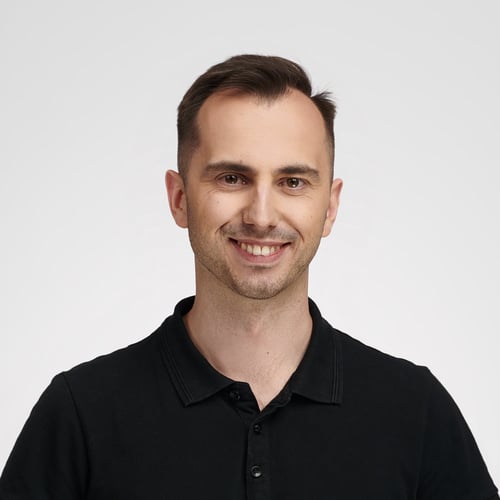
Bartosz Zaczyński RP Team on Sept. 29, 2023
@Grouchy Old Fart It’s a feature, not a bug. The sys.stdin
object represents the standard input stream. Because IDLE is a graphical user interface wrapper over the standard Python REPL, it doesn’t run in a terminal environment. Instead, IDLE wraps the standard input stream in a file-like object to make it compatible with the event loop of the graphical user interface.
Become a Member to join the conversation.
eshivaprasad on May 17, 2020
Are there situations in which sys.stdout.write() is preferable than print()?