Combine filter() and reduce()
00:00
In this lesson, you’ll learn what the reduce()
function is and how to use it. Also, you’ll learn how you can combine it with filter()
.
00:09
reduce()
is a function that combines elements of an iterable, using a specified function to produce a single result. reduce()
is a part of the functools
module.
00:21
This means that in order to use it, you’ll have to import it from the functools
module. The functools
module in Python is a built-in module that provides higher-order functions and utilities to enhance the functionality of functions and callable objects.
00:39
reduce()
is useful when you need to apply a function to an iterable and reduce it to a single cumulative value.
00:50
reduce()
takes in three arguments: function
, iterable
, and initial
. By the way, the reason that reduce()
is considered as a higher-order function is that it takes in another function as one of its arguments, just like filter()
and map()
.
01:07
The function argument represents the operation or calculation that will be applied to the elements of the iterable. The iterable
argument is the collection of elements that will be iterated over and reduced to a single value.
01:23
It can be a list, tuple, or any iterable object. The initial
value argument provides an optional starting value for the reduction. If provided, the function is first applied to this initial value and the first element of the sequence. If you leave it empty, the first element of the sequence is used as the initial value.
01:47
Let’s put reduce()
into action.
01:50
Compute the sum of even numbers. Your goal is to extract the even numbers of a list using filter()
and then adding them all together using reduce()
.
02:03
In this example, you’ll filter the odd numbers of a list using filter()
, and then you’ll use reduce()
to add all the even numbers and return a single value as the result. You’ll need to import reduce()
first: from functools import reduce
.
02:21
Let’s re-create the same list of numbers from compute the square of even numbers example: numbers =
[1, 3, 10, 45, 6, 50]
.
02:33
Now it’s time to filter odd numbers from this list. You’ve already done this multiple times. You’ll need a predicate function, is_even
, that checks the remainder of a number when divided by 2
. def is_even()
, number
as an input, return number % 2 == 0
.
02:54
So if the number is divided by 2
, and the remainder is 0
, is_even()
will return True
.
03:02
Now you can use the filter()
function with is_even
as its predicate function and numbers
as its iterable and store the results in even_numbers
.
03:11
even numbers = list(filter())
, is_even
as a predicate function and numbers
as an iterable.
03:22
Let’s check the even numbers. You got 10
, 6
, and 50
, so you have successfully filtered odd numbers. Perfect. Now that you have your even numbers, it’s time to use reduce()
to add them all together.
03:37
reduce()
. As reduce’s function argument, let’s create a lambda function that takes two numbers, a
and b
and returns a + b
. even_numbers
as reduce’s iterable argument.
03:50
Before you see the results, let’s think about what you expect to see here. You’re adding all the even numbers together, and reduce()
is meant to give you a single value representing their sum.
04:03
10
plus 6
plus 50
is equal to 66
, so you expect to see 66
here. Let’s see if it worked. And it did.
04:13
You got 66
. When you called reduce()
, it started from the first two values of even_numbers
, so 10
and 6
.
04:21
Then it applied the lambda function that you created on them. 10
plus 6
is equal to 16
, so the first cumulative result, which is called an accumulator, is 16
. Now 16
is going to be added to the next element, which is 50
, so 16
plus 50
equals 66
, and since there are no more elements, reduce()
returns a single value of 66
, which is the sum up all the numbers in even_numbers
list.
04:53
You can turn this into a one-liner. Instead of creating a new list, like even_numbers
, you can directly use filter()
as reduce’s iterable argument because, as you know, filter()
returns an iterator.
05:07
Let’s keep everything the same, but instead of using even_numbers
, let’s use filter()
directly reduce()
, lambda
keyword, a
and b
as input numbers, returning a + b
, and filter()
as reduce’s function argument.
05:26
Let’s put in is_even
and numbers
as the iterable. Let’s see if you get 66
again. And you do. To recap, filter()
extracted the even numbers of the numbers
list and returned an iterator. You’re using that iterator directly as reduce’s iterable argument.
05:47
Then reduce()
started from the first two elements of the iterable, so 10
and 6
, and applied the lambda function that you created and added them together. Now you have an accumulator of 16
.
06:03
Next, reduce()
added the third element of the iterable, which is 50
, to the accumulator, so 16
plus 50
equals 66
.
06:12
That was all of the values in the iterator that filter()
produced, so reduce()
returns 66
, which is the sum of all the elements of its iterator.
06:24
By the way, you may have noticed unlike filter()
and map()
, where you needed to call the list()
function on the result, you don’t need to do that here since what you get is a single value, not an iterator.
06:37
Good job! You just used reduce()
and filter()
to compute the sum of even numbers of a list.
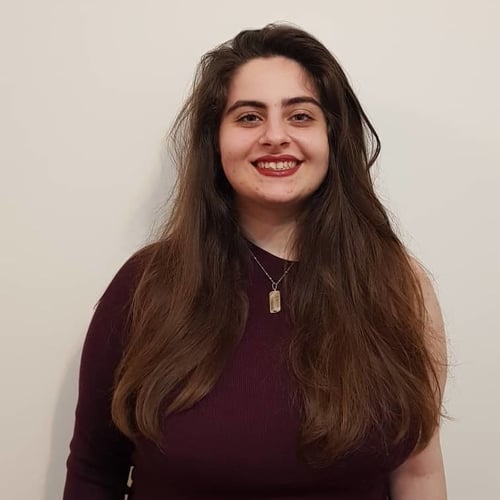
Negar Vahid RP Team on July 11, 2023
Hey there @Karel Dongen That’s a great question. This solution definitely is Pythonic and showcases the power of lambda functions and filter even further. However, it makes the code a little less readable. Hats off to you for coming up with this pro solution!
Become a Member to join the conversation.
Karel Dongen on July 10, 2023
Any reason its not reduced to:
As that gives te same outcome.