Understanding the Constructor and Attributes
00:00
In this lesson, you’re going to cover the constructor and attributes. That means you’re going to be covering the .__init__()
constructor. That’s quite a mouthful, so in Python, the __
is often shortened to dunder—d for double, under for underscore, d under, dunder.
00:24
So you can say the dunder init constructor. The .__init__()
method is also sometimes referred to as just the constructor method or simply the constructor. Now, you may have noticed I called it the method.
00:39 You’ll note in a second that the constructor looks a lot like a function, and in many ways, it is a lot like a function. But in Python, functions that are part of classes are called methods, making this not the constructor function, but the constructor method. You’ll be getting into more on methods later in the course. You’ll be covering attributes, which is basically where the information lives in classes.
01:07
And you’ll also be covering the self
parameter, which is basically how the code within a class can refer to the instance of the class.
01:18
So instead of having a class that doesn’t have any functionality or information in it, it’s just a container of sorts, you can add an .__init__()
constructor.
01:29
Now that’s defined as you would a normal function, with the def
keyword, a space, and then you have __init__
, and then you open the brackets to accept the parameters.
01:41
And you’ll note here that the first parameter always has to be self
. Doesn’t have to be called self
, but it almost always is. This is another one of those conventions. Just call it self
.
01:54
Basically the first argument that’s always going to be passed to the .__init__()
function will be a pointer to the actual instance of the class.
02:02
After the self
, you can think of it as a normal function that accepts parameters. In this case, since you’re defining a point, you want the x
and y
coordinates, and so those are the parameters for your function. As usual, you have your colon, and you start with the indented block to define the function body.
02:22
Then, to assign the x
and y
value to the instance of the class, you use the self
keyword. self.x = x
.
02:35
So now the instance will have the .x
attribute assigned the value that you passed in at construction.
02:45
Here’s another example. In this case of Doggo
, you have the self
keyword as before, but in this case, instead of passing in the x
and y
, you’re passing in a name and an age, because those are the only bits of information that you’re interested in right now. And it follows much the same pattern. You take self
, which represents the instance.
03:04
You use dot notation (.
) to reference the attribute .name
, and then you assign it to the value that was passed into the constructor.
03:17
So what do constructors look like in practice? Say you’re taking the Point
example. Now the class Point
is defined … you can instantiate …
03:31
but you’ll get an error if you have parameters to your constructor. Using the brackets after the Point
will automatically call the constructor, so you can think of this as a call to this function.
03:48 This function has three parameters. The first one is populated automatically, but the second two you have to provide.
04:00
As you can see, you’re getting some suggestions here, so let’s say 10
and 10
, and now that will instantiate properly. You’re going to be covering instantiation with attributes in a later lesson, but that’s basically what it looks like in practice.
04:18
So you’ll have seen the self
keyword. Now what is that exactly? Let’s explain that. self
refers to the instance of the class.
04:28
So in the constructor, when you try and instantiate a class, self
refers to the instance. So if I, say, assign p
to one of these,
04:43
and then you see p.x
now has access to that because it’s been assigned to the instance with the self
keyword in the constructor.
04:57
You can think of it as on “its self.” So self
is the name commonly given to the first argument passed to the constructor and other methods that you’ll come to soon.
05:07
It’s a convention to call it self
. It’s just the first thing that’s passed to the constructor methods. You can call it me
or I
or anything else you like, but don’t call it something else because everyone in the Python world calls it self
, and you’ll see that in examples everywhere. So just don’t think about it.
05:30
So when you instantiate a class, what happens is that you’re calling the constructor if there is one. You define the self
parameter of the function, and then you can assign the attribute values to the self
object.
05:42
So you can calculate stuff. You can just make a straight assignment to say self.greeting = "Hello, World!"
, and you don’t need any arguments for that.
05:51
But usually what you want to do is instantiate the object, pass in a bunch of values, and you want those values to end up on the instance of the class, and the way you assign them is with the self
keyword.
06:03
You do self.
whatever the attribute is you want and you assign it the value. Any instance setup that you want to do, any building of lists or dictionaries or more attributes or calculated values, you can stick all of that in the constructor.
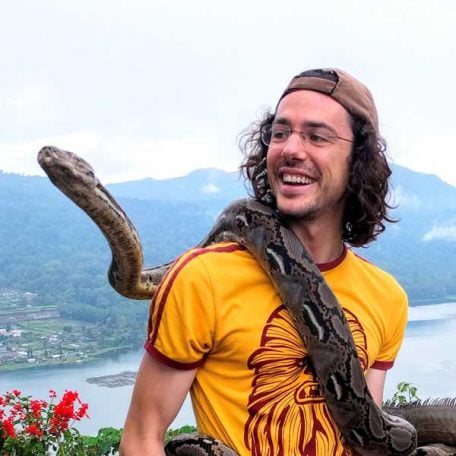
Martin Breuss RP Team on Dec. 7, 2023
Hi @Pedro Gomes yes, that’s right :) However, both .__new__()
and .__init__()
usually run when you’re instantiating an object in Python, so these terms aren’t used super strictly.
We have a super in-depth article on Python Class Constructors for more detail, though!
Pedro Gomes on Dec. 7, 2023
Thanks Martin, I will check the article carefully. :)
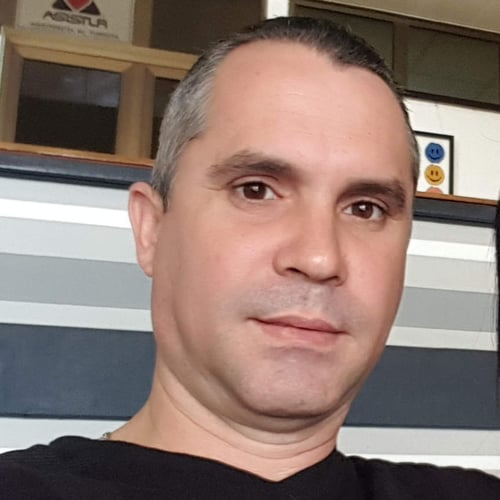
Leodanis Pozo Ramos RP Team on Dec. 8, 2023
The .__init__()
method is the initializer, while the .__new__()
method is the instance creator. Both methods are implicicly called when we call the class constructor Point(10, 10)
.
Become a Member to join the conversation.
Pedro Gomes on Dec. 7, 2023
Hello, wouldn’t the
__init__
method be the class initializer? The constructor at the time would be__new__
.