Python vs JavaScript for Python Developers (Summary)
This course was an introduction to JavaScript for Python programmers and covered a broad swath of JavaScript, especially concentrating on areas that would be unexpected for programmers coming from another language.
In this course, you learned:
- Where JavaScript comes from and where it is used
- How JavaScript’s type system is different from Python’s
- How to write functions in JavaScript
- The two ways of creating objects in JavaScript
- General language syntax in JavaScript
- Surprises and behaviors in JavaScript that Python programmers wouldn’t expect
Here are some resources for more information about JavaScript package managers:
- Bower: A package manager for the web
- npm: The Node package manager
- Yarn: A package manager that doubles down as project manager
Here are some resources for more information about JavaScript test tools:
- Jasmine: A behavior-driven development framework for testing JavaScript code
- Jest: A JavaScript testing framework with a focus on simplicity
- Mocha: A JavaScript testing framework running on Node.js and in the browser
Here are some resources for more information about JavaScript libraries and frameworks:
- jQuery: A fast, small, and feature-rich JavaScript library
- Angular: A JavaScript framework for web, mobile web, native mobile, and native desktop
- React: A JavaScript library for building user interfaces
- Vue.js: An adoptable ecosystem that scales between a JavaScript library and a full-featured framework
Congratulations, you made it to the end of the course! What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment in the discussion section and let us know.
00:00
In the previous lesson, I finished the section on quirks by covering the this
keyword and how it is set based upon the calling context of a function. In this lesson, I’ll wrap everything up by summarizing what you’ve covered and briefly talk about where to go next.
00:15 I started out giving a brief history of how JavaScript came about and the places where it can be run. I talked about types in JavaScript and how, unlike Python, there are two kinds: primitive and reference.
00:28 I also spoke about how JavaScript supports autoboxing, changing primitive types into their corresponding reference objects on the fly as needed. After that, there was a lesson on functions in JavaScript, followed by one which delved into objects.
00:43 Both the old way of using function-based constructors and the new way of using classes was discussed.
00:51
There were two lessons on the details of the syntax of JavaScript. These covered code blocks, ternary operators, statements and semicolons, identifiers, comments, strings, variable scoping, the switch
statement, freezing objects and using them like enumerations, the arrow function shortcut, using destructuring assignments, the with
statement, generators, and asynchronous functions using the async
and await
keywords. With the basic syntax down, I spent several lessons on the places where JavaScript’s behavior may not seem intuitive for those coming from other languages.
01:32
A lot of these quirks are due to the fact that JavaScript has far surpassed its original purpose. Plenty of things have been added to the language to make it easier to use and to make your code cleaner. Due to supporting backward compatibility, though, the original odd things are still in the language. Over the course of several lessons, I covered how arrays aren’t like arrays that you’re used to, how sorting an array is string-based by default, how semicolons being optional can bite you, the different kinds of looping structures, the lack of errors when calling a function-based constructor without the new
keyword, how var
works with the global scope, within function scope, and with hoisting, how function signatures are merely a suggestion, how JavaScript’s automatic typecasting can cause unexpected results when comparing different types, how numbers are all floats except for the new BigInt
, the fact that there are two kinds of empty, and finally, a long detailed lesson on how the this
keyword works.
02:36 Learning the syntax of a language is only the beginning. Modern languages have a daunting learning curve due to all the libraries and tools out there. JavaScript is no different.
02:46 There are two contexts for JavaScript usage: server-side using Node or within the browser. Although everything that I’ve covered works in the browser, it is only the tip of the iceberg. Within the browser, you’re going to want to make changes to the HTML in order to interact with the user.
03:02 This was the original purpose of JavaScript, and still its most common usage. Within a browser, there are a series of built-in functions for managing what the user sees.
03:11 The HTML is mapped to the DOM, or Document Object Model—a model of what is being presented. You use the built-in functions to manipulate the DOM, thus interacting with the user. JavaScript on its own is quirk-errific, but JavaScript plus HTML plus CSS, both of which are quirky in their own right, and you’re entering the world of quirk-errendous.
03:34 Whether that word I just made up is based on “tremendous” or “horrendous” is something you’ll have to discover for yourself.
03:41 If you’re using JavaScript for a bit of form validation, you can get away with just adding some files to your web server and serving them up. For large projects, you’re going to want to think about a tool chain for helping you manage your code. JavaScript has tools for package management like Bower, npm, and Yarn. There are testing frameworks such as Jasmine, Jest, and Mocha, and scaffolding tools such as cookiecutter and Yeoman.
04:05 There’s also a whole world of frameworks in this space. They range from things like jQuery, which helps you manipulate the DOM and was originally built so you didn’t have to remember differences in the various browser implementations, to full single-page application frameworks such as Angular, React, and Vue. Angular is TypeScript-only, but as that is a superset of JavaScript, you can transition fairly easily. React is both JavaScript and TypeScript.
04:34 Both Angular and React are fairly opinionated about how to build and manage your code. That can make for a steep learning curve but it also means you’re more likely to be able to reuse other people’s pluggable components. Vue is a lighter-weight framework. I’ve been using this one a lot as of late.
04:50 A lot of the web development I do is in Django and I don’t usually write single-page applications. From page to page in my software, I need a varying degree of interactivity.
05:00 As Vue is a bit less opinionated than Angular or React, I find it easier to drop in on those pages where I need more power, while sticking to straight Django templating where I can.
05:12 You’ve covered a lot and there’s still loads more out there. Thanks for sticking with me through it all. Whether you find yourself writing JavaScript or Python, happy coding!
dudutt on July 12, 2021
Nice course! I like this kind of introduction with opinions and tips about what is preferred to use and what is more likely to go wrong. I’m learning Python and Javascript for a project, so I’ve felt like this course was made for me.
Marko on Dec. 19, 2021
Your courses are highly informative and they are a great resource for students such as myself. If you find the time, please make a Python vs C# course!
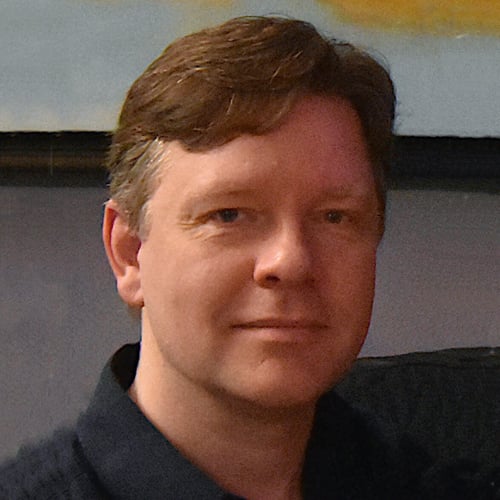
Christopher Trudeau RP Team on Dec. 20, 2021
Hi Marko,
Glad you found it useful. I’ve never done any C#, so somebody else at RP would have to take that on. I did see a Java article get posted recently:
James Walters on April 6, 2022
Thanks Chris! Your dated references weren’t lost on me.
As someone who last attempted to pick up JavaScript sometime in the late 00’s and rage-quit after encountering the footgun of floating point arithmetic (I built a calculator: 3 + 3
was 6
, + 3
was 9
, + 3
was 11.98
), this at least cast these various traps and pitfalls in terms I can understand, having learned Python in the last few months. This is the resource I’ll refer people to as JS newcomers coming from Python!
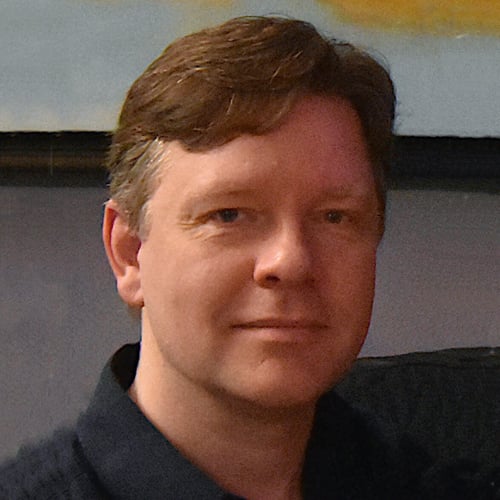
Christopher Trudeau RP Team on April 7, 2022
Glad you enjoyed it. Floating point is messy everywhere, JS just makes it worse because strings can get involved.
Python REPL:
>>> 0.1 + 0.2
0.30000000000000004
What Python does do well is it has a module for fractions and decimals that can give you the precision you probably want.
See:
realpython.com/python-numbers/
and
Become a Member to join the conversation.
Alain Rouleau on June 27, 2021
Great introduction to JavaScript as it compares to Python. Lots of information packed into a short period of time, that’s for sure. But it really does give you a sense of how everything works. Thanks a lot and keep up the good work!