The .sort() Method
00:00
So far, you’ve used the Python built-in function sorted()
to organize your iterables, like lists and strings. Now you’re going to learn how to use the .sort()
method, which is pretty similar and yet completely different. .sort()
is a method of the list
class, which means it can only be used on lists.
00:17 This also means that you call it off of a list instead of calling it as a function and passing in a list. Let’s go to the interpreter and see how that works.
00:27
So, you’re just going to make a quick list here, values_to_sort
, and set this equal to [5, 2, 6, 1]
. If you were to take a look at that, you’ve got your list. To use the .sort()
method, you’ll just take your values_to_sort
, and then you’ll call .sort()
off of that. Note here that the interpreter didn’t return anything. Instead, .sort()
is modifying the list in place.
00:53
So if we take another look at that, you’ll see that the list is now sorted. There’s no way to get back to the original order of this list, so keep that in mind. Now, because .sort()
is a list
method, it won’t work on other things like strings.
01:06
So if you just said some_string
and set this equal to 'abc'
—maybe some just random letters in there. If you had some_string
and you tried to call .sort()
off of that, you’ll get an error. And that’s because the str
(string) class does not have .sort()
.
01:22
You could still go ahead and say sorted()
and pass in that string,
01:28
and that would return the sorted string. Finally, if we make another values_to_sort
—and let’s just set this equal to a couple of numbers here—
01:41
if you wanted to make a new variable called sorted_values
and you were to set this equal to values_to_sort
and then call .sort()
from that, now if you take a look at sorted_values
, you’ll see that nothing is returned.
01:55 To be a little clearer, let’s actually just print that out.
02:02
And you can see that sorted_values
is None
. That’s because .sort()
doesn’t return a value the way that sorted()
does.
02:09
So the big differences to keep in mind when using .sort()
instead of sorted()
is that .sort()
does not return any ordered output.
02:17
So if you try to assign the output to a new variable, it’ll just be None
. And then the other big thing is that the sorting takes place on the list in place, which means that the original data is changed and there’s no way to go back. Fortunately, when it comes to reverse
and key
, .sort()
behaves the same way.
02:36
So if you’re to make a list called phrases
and you just type in some sentences like 'when in rome'
, 'what goes around comes around'
,
02:47
and 'all is fair in love and war'
….
02:52
Let’s use .sort()
on phrases
02:56
and pass in a lambda
function for the key
, which will be x
and then you’re going to take this, split it,
03:07
grab the third word, and the second letter of each word. Finally, set reverse
equal to True
. So remembering the lambda
functions, each of these strings here will be passed in. They’ll be split, so they’ll be turned into lists of words. You’ll grab the second indexed word—so 0
, 1
, 2
. And then from there, you’ll grab the second letter. And then phrases
will be sorted based on that.
03:35
So if you take a look at phrases
, you can go and take a look at the third word and the second letter—so 'r'
, 'o'
, and 'a'
.
03:47
And that makes sense because reverse
was set equal to True
. And that’s all there is to .sort()
! You can see that it’s pretty similar to sorted()
but it has some major differences to how it treats your data and what it outputs. Generally, you’ll want to use one or the other, and we’ll cover that in a later video. For now, we’re going to shift our focus into talking about some common issues that can come up when you’re sorting with Python.
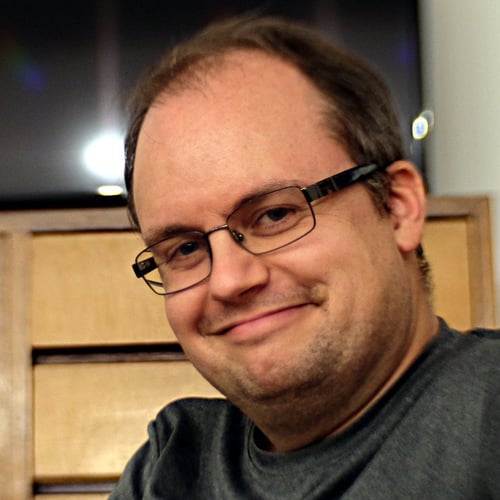
Geir Arne Hjelle RP Team on March 3, 2023
Hi Irene, you can define your custom sort order by using the key
argument. This is covered in an earlier video in this course. You can also read about it at realpython.com/python-sort/#sorted-with-a-key-argument
Additionally, the following tutorial focuses on sorting dictionaries but does provide several examples of using key
that’ll work on any iterable: realpython.com/sort-python-dictionary/#using-the-key-parameter-and-lambda-functions
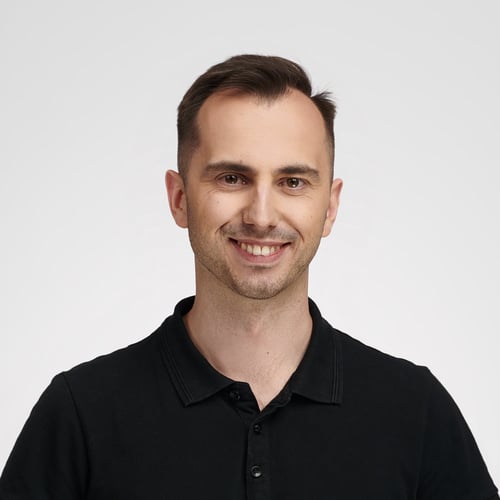
Bartosz Zaczyński RP Team on March 3, 2023
@Irene Galstian Just like you, I’ve been bitten by the fact that Python’s built-in sorted()
function doesn’t support Unicode characters well. However, you can achieve the expected result in a few ways.
First, you can set the locale to the desired language and use the following transformation function as the sorting key:
>>> import locale
>>> names = [
... "Łukasz",
... "Józef",
... "Małgorzata",
... "Paweł",
... "Radosław",
... "Mirosław",
... "Stanisław",
... "Żaneta",
... "Świętosław",
... ]
>>> locale.setlocale(locale.LC_ALL, "pl_PL.UTF-8")
'pl_PL.UTF-8'
>>> for name in sorted(names, key=locale.strxfrm):
... print(name)
...
Józef
Łukasz
Małgorzata
Mirosław
Paweł
Radosław
Stanisław
Świętosław
Żaneta
Alternatively, you can use a third-party library like PyICU, which is a Python binding to IBM’s ICU library, or a pure-Python implementation of the Unicode Collation Algorithm called PyUCA:
>>> import pyuca as pyuca
>>> names = [
... "Łukasz",
... "Józef",
... "Małgorzata",
... "Paweł",
... "Radosław",
... "Mirosław",
... "Stanisław",
... "Żaneta",
... "Świętosław",
... ]
>>> collator = pyuca.Collator()
>>> for name in sorted(names, key=collator.sort_key):
... print(name)
...
Józef
Łukasz
Małgorzata
Mirosław
Paweł
Radosław
Stanisław
Świętosław
Żaneta
If you’d like to sort more complex objects, for example, people by their first name and last name, then you can make a compound key by wrapping the collator instances in a tuple:
>>> from typing import NamedTuple
>>> import pyuca as pyuca
>>> class Person(NamedTuple):
... first_name: str
... last_name: str
...
... def __str__(self):
... return f"{self.first_name} {self.last_name}"
...
>>> collator = pyuca.Collator()
>>> def compound_key(person):
... return (
... collator.sort_key(person.first_name),
... collator.sort_key(person.last_name),
... )
>>> people = [
... Person("Łukasz", "Nowak"),
... Person("Józef", "Kowalski"),
... Person("Małgorzata", "Wiśniewska"),
... Person("Paweł", "Wójcik"),
... Person("Radosław", "Kowalczyk"),
... Person("Mirosław", "Krawczyk"),
... Person("Stanisław", "Mazur"),
... Person("Żaneta", "Jabłońska"),
... Person("Świętosław", "Łuczak"),
... ]
>>> for person in sorted(people, key=compound_key):
... print(person)
...
Józef Kowalski
Łukasz Nowak
Małgorzata Wiśniewska
Mirosław Krawczyk
Paweł Wójcik
Radosław Kowalczyk
Stanisław Mazur
Świętosław Łuczak
Żaneta Jabłońska
img on March 3, 2023
Bartosz, I can’t thank you enough! My task is a concordance of Vedic Sanskrit words, which should follow the order of Sanskrit alphabet: aāiīuū etc. Your example gives me hope.
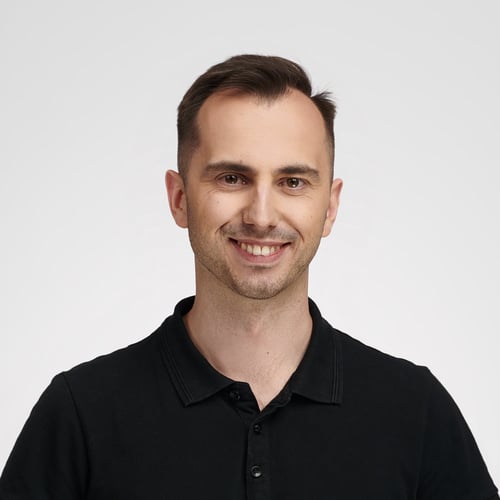
Bartosz Zaczyński RP Team on March 3, 2023
@img Glad you found it helpful! Let us know if you encounter any other challenges.
Become a Member to join the conversation.
img on March 3, 2023
Does anybody know how to define a custom way of sorting, rather than the default English alphabet order? If there is a course/video on here about this, please pount me to it, as no joy so far with searching.