Sorting Numbers With sorted()
00:00 A great way to get into sorting with Python is to sort a list of numbers. Lists are an ordered data structure in Python, so we should be able to see the differences pretty clearly after sorting.
00:11
I’m going to go ahead and open up my interpreter and then make a new list called numbers
. And this will just be [6, 9, 3, 1]
. Once you have your list, you can go ahead and just call sorted()
on that. All right.
00:30
You can see here that this returned the list that we had before, and now it is in order. There’s a couple of things that you should note here. First, we didn’t have to define or import sorted()
.
00:42
The sorted()
function is one of Python’s builtins, so it will always be available in a regular installation of Python. Second, because you just passed in numbers
, the list, sorted()
worked in its default mode and sorted these in ascending order, or smallest to largest.
00:59
Another thing to note is if you take a look at numbers
again, you can see that it’s still the original, which means that sorted()
did not change what numbers
equaled.
01:09
What it did do was return a list, which you can see here. That means that you can assign this list to a new variable. Let’s see how that works. Go ahead and make a new list, and call this something like numbers_sorted
.
01:24
You can set this equal to sorted(numbers)
.
01:29 Now if you want to take a look at that,
01:32
you can see that you saved the ordered list. Now that you can use sorted()
on lists, let’s try some different data types in Python. Go ahead and make a tuple, which will be inside parentheses. And you can make this the same way, so it’ll be (6, 9, 3, 1)
.
01:51
Now, note that tuples maintain their order but are immutable, which just means you can’t change them after you define them. While you’re here, go ahead and make a set called numbers_set
,
02:04
and set this equal to something like {5, 5, 10, 1, 0}
. Keep in mind that sets do not save the order, and also can’t have duplicate values. So if you try to take a look at that, even though this is what you passed in, it’s now {0, 1, 10, 5}
.
02:22
So, let’s see how sorted()
works on this. So, numbers_tuple_sorted
, just set that equal to sorted()
and pass in your numbers_tuple
.
02:34 And go ahead and do that with the set as well.
02:41
All right! Let’s take a look at what we have. So, numbers_tuple_sorted
—you can see here that you’ve got a list, which is [1, 3, 6, 9]
. And if you take a look at the set,
02:57 you’ll see that you have a list here, which is what was left of the set and in order. Now, if you need that data in its original data types, you can always cast that back.
03:07
So if you just call tuple()
and then pass in numbers_tuple_sorted
, you can now return your tuple, which has its values in order.
03:17
But be careful when you try to do this with a set, because if you pass in the numbers_set_sorted
, you’ll get your set back but because sets are not ordered, you lose the order that you had before. All right!
03:31
That’s all there is to using sorted()
with numbers. The big takeaways from here is that sorted()
will not affect the original value that you pass into it and will only return the sorted version of that value.
03:43
The other thing to note is that no matter what you pass into sorted()
, assuming sorted()
can sort it, you’ll get a list as the return value. In the next video, you’ll see how to use sorted()
with strings.
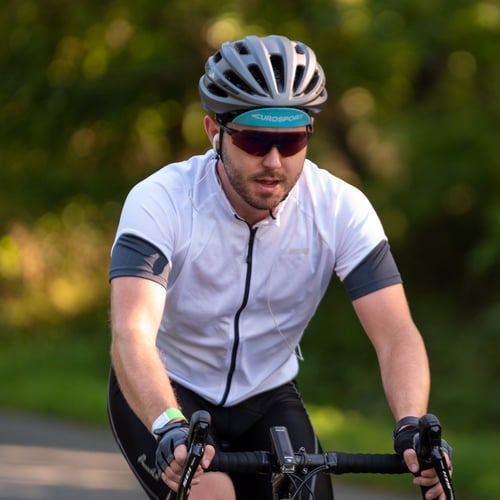
Joe Tatusko RP Team on Feb. 28, 2020
Hi Karthik! In these videos I’m using Atom with Material themes.
B S K Karthik on Feb. 29, 2020
Thank you Joe
Become a Member to join the conversation.
B S K Karthik on Feb. 28, 2020
Hi Joe, which editor have you used for the demos.please let me know.