Structuring Your Game
00:00 In the previous lesson, I showed you how to make very quick blue speckles in a dazzling display of randomness. In this lesson, I’ll show you how to structure your code files to get ready for larger programs.
00:12
The typical way of running a Python script is to use the python
command and pass it the script file, or if you’re on a Unix-based system, use the hash-bang (#!
) control at the top of the file, which is really just a shortcut for doing the same thing.
00:26 There is another way of doing things, though. You can run a directory instead of running a single file. This can be useful when you want to split your code up into multiple files and you don’t want your user to have to figure out which one is the one to run.
00:40
To use this directory running mechanism, you need a file named __main__.py
, also known as dunder main. The oh-so-clever name for our Asteroids clone is Space Rocks.
00:54
As there’s going to be several files for the code, you can create a directory named space_rocks
and put a __main__.py
file inside.
01:02
There will be other files in there as well, such as game.py
, where a lot of the game logic will live. With this structure in place, calling the Python interpreter with the space_rocks
directory as a parameter will result in your game running.
01:18
Very shortly, I’ll be showing you the first code for Space Rocks. First, though, make sure to create a directory where your files will live. You can do that with the mkdir
command.
01:28
As I mentioned before, the first file you’ll need is __main__.py
. The contents of __main__.py
will be pretty simple. It imports and creates a SpaceRocks
object from the game
file and then runs its .main_loop()
method.
01:42
All of this is done inside of a __name__
check on line 4. If you haven’t seen this before, this if
statement is checking if the script is being run as code, as opposed to being loaded as a module. Inside of a __main__.py
file, this is likely redundant.
01:59 You’ll probably never load this file as a module, but it is best practice to do this and it signals to other developers that this is the program’s entry point.
02:11
The next file you’ll create is game.py
. This is where you’ll keep the main loop of the game and the core logic. The contents here aren’t that different from blue.py
in the previous lesson.
02:22
It’s just organized in a different way. The initializer for the SpaceRocks
object contains the Pygame init()
, set_caption()
, and screen size calls that get you going.
02:35
The surface from the set_mode()
call is being stored for later use inside of the SpaceRocks
object in self.screen
.
02:43
Line 12 defines the core game loop. Inside of here, you’ll find the while
loop. It checks for input, does any game logic, and draws a frame. No different than blue.py
, just reorganized to be a bit more clear.
03:00
In each iteration of the loop, you’re going to check for events that need handling. That’s the ._handle_input()
method. Just like in blue.py
, I’m starting out here just checking for whether or not QUIT
was pushed.
03:14 The second part of the game loop, which is doing the game logic, is defined on line 23. This is just a placeholder for now. There isn’t any logic yet, but this will be where objects can be moved around.
03:26 Expect great spaceship and rock position logic here in the future. Let me just scroll down. The final part of the game loop is the drawing. This first version of Space Rocks does nothing but draw a blue background.
03:40
Not all that exciting, but imagine the frame rate you’re going to get with so little happening in each loop. Let me call python
with the space_rocks
directory, and…
03:54 Isn’t this exciting? Not exactly the easiest game to win, but you’re ready to go! With this, a multi-million dollar budget, and several hundred developers, you could be writing Call of Duty in no time. Okay, enough with the Call of Duty references.
04:08 I promise the next one will be all about Grand Theft Auto.
04:13 Don’t tell Bill Gates I said so, but blue screens are so passé. Next up, I’ll show you how to import graphics. Ultra-violence and drive-by shootings, here you come. Told you there’d be a GTA reference.
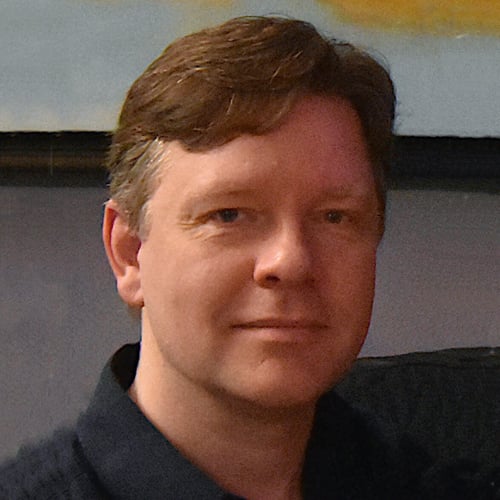
Christopher Trudeau RP Team on Aug. 15, 2023
Hey there,
That error is coming from “bash” which is the shell in your operating system. It is saying it can’t find the Python interpreter.
Have you run Python with other projects before? Used it in an editor like VS Code or PyCharm?
If you’ve got it on your system, then for some reason that bash session can’t see it, which likely means there is something wrong with your path.
If this is the first time you’re using Python (or first time outside of an editor), then you may need to install it. These courses/articles may be helpful for you:
realpython.com/installing-python/
realpython.com/courses/installing-python-windows-macos-linux/
Hope that helps. …ct
Become a Member to join the conversation.
De87627k6 G7r41 on Aug. 14, 2023
I set up the code in this video correctly, but when I type in “python space_rocks/ in the terminal (-bash: python: command not found) pops up. I’m not entirely sure what is going wrong. The main.py and game.py folders are in space_rocks.