Think of a variable as a name attached to a particular object. In Python, variables need not be declared or defined in advance, as is the case in many other programming languages. To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign (=
).
Variable Assignment
00:00 Welcome to this first section, where we’ll talk about variable assignments in Python. First of all, I just want to mention that I’m going to be using the IPython shell.
00:09 The reason for that is just that it adds a bit of colors to the prompt and makes it a bit easier to see which types we’re working with, but you don’t need to do this install that I’m going to show you in just a second.
00:19
You can just use your normal Python interpreter and it’s going to work all the same. If you want to install IPython, all you need to do is go to your terminal and type pip3 install ipython
, press Enter, and then wait until it installs.
00:36
I already got it installed. And then instead of typing python
to get into the interpreter, you’re going to type ipython
.
00:46
It gives us the same functionality, only you see there’s some colors involved and it looks a bit nicer. I can do clear
and clear my screen. So it’s going to make it a bit easier for you to follow, but that’s all.
00:58
So, first stop: a standard variable assignment in Python. Unlike other languages, in Python this is very simple. We don’t need to declare a variable, all we need to do is give it a name, put the equal sign (=
) and then the value that we want to assign. That’s it.
01:15
That’s a variable assignment in Python. I just assigned the value 300
to the variable n
. So now I can print(n)
and get the result.
01:26
Or, since I’m in an interpreter session, I can just put in n
and it shows me that the output is going to be 300
. So, that’s the basic, standard variable assignment that you’re going to do many times in Python.
01:38
And it’s nice that you don’t need to declare the variable before. You simply can type it in like this. Now the variable n
is referring to the value 300
.
01:48
What happens if I change it? So, I don’t need to stick with 300
through the lifetime of this variable. I can just change it to something else. I can say “Now this is this going to be 400
.”
02:00
Or, in Python, not even the type is fixed, so I can say n = "hello"
and change it to a string.
02:10
And this is still all working fine. So you see, it feels very fluid, and this is because Python is a dynamically-typed language, so we don’t need to define types and define variables beforehand and then they’re unchangeable for the rest of the program—but it’s fluid, and we can just start off with n
being an integer of the value of 300
and through the lifetime of the program, it can take on a couple of different identities.
02:36
So, apart from the standard variable assignment that we just saw before, n = 300
, we can also use a chained assignment in Python, which makes it quick to assign a couple of variables to the same value.
02:49 And that looks like this.
02:52
I can say n = m = x = y
et cetera, and then give it a value. And now all of those variable names point to 400
, so I can say m
is 400
, x
is 400
, y
is 400
, et cetera. That’s what is called a chained assignment in Python.
03:15 Another way is the multiple assignment statement, or multiple assignments, which works a little bit different and there’s something you need to take care of, but I still want to introduce you to it. If you go ahead here, I can assign two values at the same time in one line.
03:32
So I can say a, b = 300, 400
. The comma (,
) is important, and it’s important that the amount of variables that you’re declaring here on the left side is the same amount of values that you have on the right side.
03:48
I can do this, and now b
points to 400
, a
points to 300
.
03:54 It doesn’t have to be two, there can be more, but just make sure that every time if you use this multiple assignment statement, that the amount of variables you use left is the same as the amount of values on the right. And as a last point in this section, I want to talk a little bit about variable types.
04:14 I already mentioned that variable types don’t have to be fixed in Python. I can start off with
04:21
n
pointing to 300
, which as we know is an integer. Remember, you can always check what the type of a variable is by just saying type()
and passing in the variable in there.
04:33
So it gives me as an output that this is an int
(integer).
04:37
This is just the same as saying “What’s the type()
of 300
or 200
?” directly—it’s an integer—because all that I’m passing in here is a reference to this object. We’ll talk about this more in the next section.
04:52
But now I can easily change the type of this variable, because all I’m doing is pointing it to a different object. So now n
is pointing to a string.
05:01
If I say type(n)
now, it will tell me it’s a str
(string).
05:08 And the reason for this is that variables in Python are simply references to objects. In the next section, we’ll talk much more what’s important about that and how in Python everything is an object.
05:19
And that it for this section! Let’s do a quick recap. Variable assignments in Python are pretty straightforward. We have the standard variable assignment that just goes <variable name> = <your value>
.
05:32 We have another way of doing it that’s called chained assignments, where we can assign a couple of variable names to the same value by just using multiple equal signs.
05:43 Then there’s the multiple assignment statement, which works a little differently, and you have to take care to use commas and the same amount of variable names on the left side as values on the right side.
05:53
It’s going to assign, as expected, n
to 300
, m
to 400
. And then finally, we talked about variable types, that they are fluid in Python and that you can check what the variable type is by using the type()
function.
06:07
And here’s a nice thing to see also, that n
is just a pointer to the 300
integer, because we’re going to get the same result if we say type(n)
or type(300)
.
06:18
They’re both int
(integer) objects. And this is a concept that we’re going to talk about more in the upcoming section when we talk about object references. See you over there.
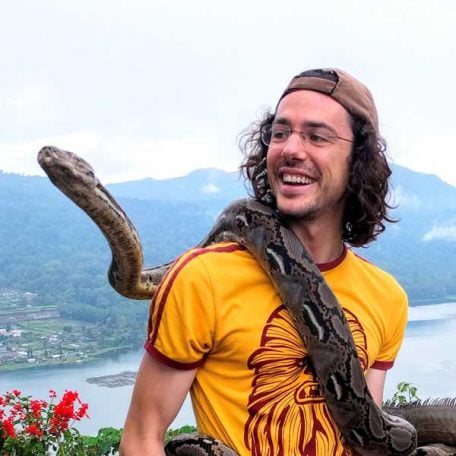
Martin Breuss RP Team on Dec. 10, 2019
You might have to close and re-open your terminal @iamscottdavis
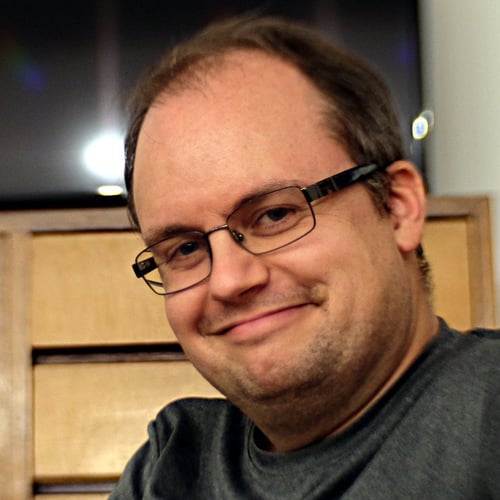
Geir Arne Hjelle RP Team on Dec. 10, 2019
I’ve recently had some weird issues with prompt_toolkit
, one of the dependencies of IPython. Maybe that’s what you’re running into?
I got a cryptic error message like TypeError: __init__() got an unexpected keyword argument 'inputhook'
. If this is your problem as well, the best solution should be to update to IPython >= 7.10 which should have fixed this. Another workaround is to downgrade prompt_toolkit
to version 2.
See some discussion on github.com/ipython/ipython/issues/11975
If you are having other problems, feel free to post your error messages :)
kiran on July 18, 2020
if i declare the variable in any one loop in python.
while True:
a = 2
print(a) #2
now my question is a is local/global variable? in C it is local variable but what about python? in Python even i declare a variable with in the loop it become a global variable?
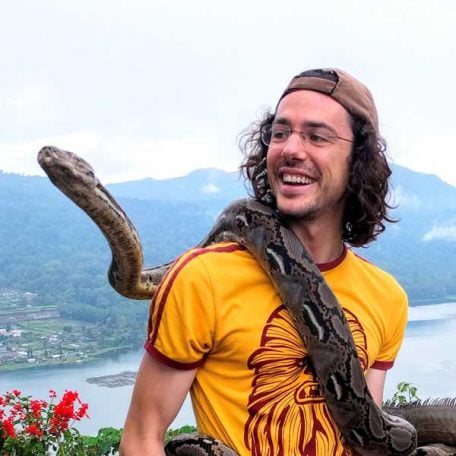
Martin Breuss RP Team on July 18, 2020
In Python, it will keep the last value it got assigned within the loop also outside in the global scope. That is why a
is still accessible and has a value in your example, also outside of the loop’s scope.
DoubleA on Jan. 20, 2021
Hey Martin,
Thanks for pulling this stuff together and explaining it so clearly. I came accross some sort of a variation of the multiple assignment you discussed. Basically, it seems that the number of variable names and variables can be diffrent. What I mean is this:
a, b, *c = 1, True, 0b1010, 'string'
then print(a,b,c)
gives me the following output:
1 True [10, 'string']
Am I right saying that, basically, what happens above is that the variable c
having an asterisk before it will get assigned a list of the two values, incl. the excessive (‘string’) one?
Thanks!
DoubleA on Jan. 20, 2021
Referring back to Kiran’s question:
When I run this code:
a = []
b = [1,2,3,4,5,6]
for elem in b:
a.append(elem*2)
print(globals())
I see that the globals()
function returns, amongst other things, the value of the variables a
,b
and the last value of the iterable elem
. Both variables a
and b
appear to be visible in the global scope as key-value pairs of the following dict:
{'__name__': '__main__', '__doc__': None, '__package__': None, '__loader__': <_frozen_importlib_external.SourceFileLoader object at 0x00000208A1961F70>, '__spec__': None, '__annotations__': {}, '__builtins__': <module 'builtins' (built-in)>, '__file__': 'c:\\doc.py', '__cached__': None, 'b': [2, 4, 6, 8, 10, 12], 'a': [1, 2, 3, 4, 5, 6], 'elem': 6}
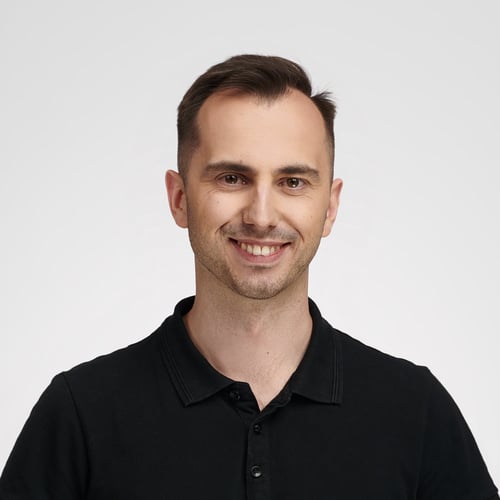
Bartosz Zaczyński RP Team on Jan. 21, 2021
@DoubleA The “starred” expression syntax you were referring to before can be used for extended iterable unpacking.
Become a Member to join the conversation.
iamscottdavis on Dec. 10, 2019
I installed ipython on my chromebook but it won’t run.