When to Use List Comprehensions
This lesson wraps up the course on list lomprehensions with a word of warning. Comprehensions are a powerful feature of Python but they can lead to unreadable code when overused or when poorly formatted.
You learned when not to use list comprehensions and also what strategies you can apply to help you decide when to use them.
Resources
Congratulations, you made it to the end of the course! What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment in the discussion section and let us know.
00:00 All right. So, the other thing I wanted to talk about is that you want to be careful with how often and under what circumstances you use these list comprehensions.
00:11
So, one downside of list comprehensions is that, well, they are more terse than for
loops. They can feel—or they can get a little bit overwhelming if someone is not familiar with them.
00:20 So with this feature, I think it makes sense to balance it with, for example, who you’re working with. If you want to introduce Python to a new team, maybe blasting them with a bunch of list comprehensions isn’t the greatest way to do that.
00:34
Because a for
loop is probably going to seem more familiar to them than hitting them with a list comprehension right away. So depending on the developer audience you’re working with, these list comprehensions might be a good feature to use, or they might not be.
00:47 This is something to keep in mind. The other thing is that these list comprehensions can also be nested. You can have list comprehensions inside list comprehensions.
00:56 And this is something that I would encourage to stay away from that. It’s much better to turn that into a loop or actually even move part of it into a separate function call, because it’s going to be a lot easier to read and a lot easier to maintain.
01:10 So, you can write some pretty gnarly list comprehensions, and usually—you know, it’s tempting, I know it’s tempting—but usually it’s not a great idea to do that, because it gets super confusing really quickly and it gets extremely hard to maintain.
01:24 So it makes sense to really spend some time to think about it, whether it’s better to use a list comprehension in a specific use case or not. So, that was a word of warning here at the end, but I think it’s definitely something you want to keep in mind.
01:37 Like, all code is communication and you always want to make sure you’re communicating your intent clearly, and that you’re not writing code just for the sake of making it extremely terse or concise or overly concise, right? Because—I know it’s fun, I’ve done it in the past and I’ve done it to other people—and really, it just kind of sucks, so I would recommend that you don’t do that. I mean, definitely use list comprehensions—they are awesome.
02:01
I think for something like that, in my mind, that’s much cleaner than using the equivalent for
loop, but be careful with deep nesting and some of the crazier list comprehensions you might want to write.
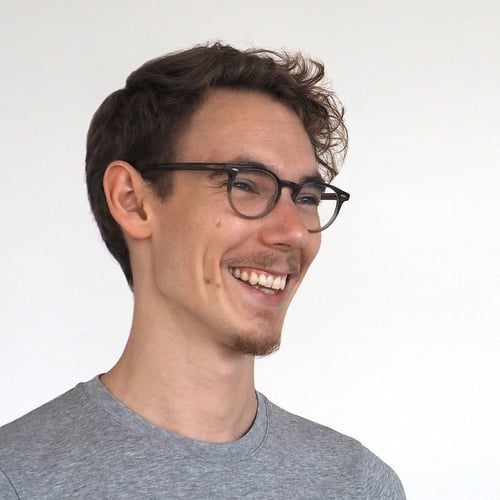
Dan Bader RP Team on March 13, 2019
Thanks Eriberto, that’s great to hear! We’ve got some more list comprehension tips in our upcoming Python Interview Tips article.
The Python Tricks book also has a chapter on them if you’re looking to dig deeper :)
govindarajuswaroop on Aug. 9, 2019
HI,
How is list comprehension different from map() function? They seem to do the same functionality.
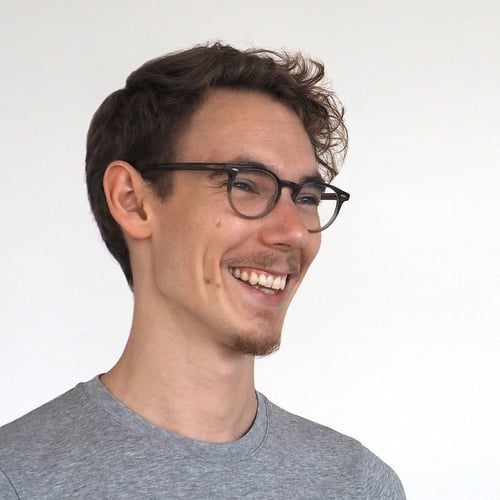
Dan Bader RP Team on Aug. 9, 2019
List Comprehension vs
map()
in Python: How is a list comprehension different from themap()
function? They seem to do the same functionality.
Great question! I’m going to quote a comment thread from python-guide.org where this was discussed:
PEP 202 says:
List comprehensions provide a more concise way to create lists in situations where map() and filter() and/or nested loops would currently be used.
Interestingly Guido van Rossum (the creator of Python) says using map with lambda as one of his “regrets” and to use a list comprehension instead. Then in the very next section he says a for
loop is more clear than reduce().
- map(), filter()
- using a Python function here is slow
list comprehensions do the same thing better
reduce()
- nobody uses it, few understand it
- a for loop is clearer & (usually) faster
Source: www.python.org/doc/essays/ppt/regrets/PythonRegrets.pdf
Also Guido said:
“It has been argued that the real problem here is that Python’s lambda notation is too verbose, and that a more concise notation for anonymous functions would make map() more attractive. Personally, I disagree—I find the list comprehension notation much easier to read than the functional notation, especially as the complexity of the expression to be mapped increases. In addition, the list comprehension executes much faster than the solution using map and lambda. This is because calling a lambda function creates a new stack frame while the expression in the list comprehension is evaluated without creating a new stack frame.”
Source: python-history.blogspot.com/2010/06/from-list-comprehensions-to-generator.html
There’s also a performance difference, please see stackoverflow.com/questions/1247486/python-list-comprehension-vs-map
lordchuffnel on Nov. 12, 2019
Could you please link to or make a video on n-dimensional lists, and list comprehension with lambda.
good video, thank you
Pygator on Dec. 22, 2019
The formula for the general list comprehension with condition and expression is a helpful abstraction.
Ghani on Oct. 22, 2020
Very good presentation; thanks!
skrabbit9000 on July 6, 2021
IMHO, if your list comprehension has to be broken up into multiple lines due to its length, then I don’t see the advantage of using it over a for loop anymore.
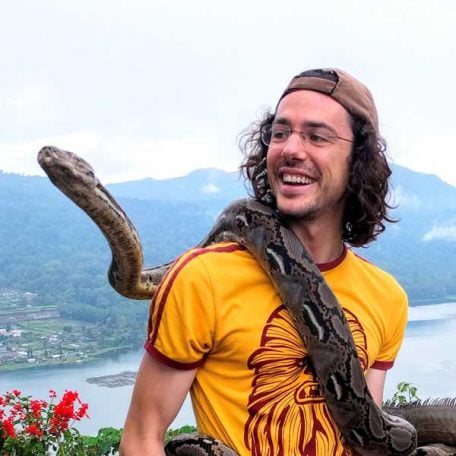
Martin Breuss RP Team on July 6, 2021
@skrabbit9000 I agree with that. For long list comprehensions it’s usually better to stick with for
loops instead.
Glenn Lehman on Oct. 11, 2021
Thank you for putting this together. It helped me to better understand what goes into the decision of when to use a list comprehension.
MAStough on Jan. 15, 2022
Thanks for the great, quick overview of list comprehensions.
ranjeet on March 9, 2022
Nice presentation, also fully agreed with, avoid to use nested list comprehension into your code. Better to go for loops.. Thanks Dan for nicely compiled the course.
amribrahim31 on July 5, 2022
Great break down with the generalized templates there, its a solid way to imprint the syntax in the brain. I have used list comprehensions a lot in projects and found that proper naming (especially the variable for each item) really helped with those lengthy and hard to read comprehensions. An example from the one used on the course can be seen below:
def is_even(x: int) -> bool:
return x % 2 == 0
def get_squared_value(x: int) -> int:
return x**2
even_squares = [get_squared_value(number)
for number in range(10)
if is_even(number)
]
Oskar on Sept. 21, 2023
Thanks for explaining this concept.
It’s fun how after just seeing a few examples and understanding how a list comprehension is “set up”, you suddenly can read more code.
I was following a different lesson, about api integrations here and was having trouble understanding this syntax:
max(country["id"] for country in countries) + 1
But now I understand… I think.
Although, the example above is iterating through an object and not a list. But guess the principle is the same.
I would’ve loved to see something like this being handled in this lesson.
Sneha Nath on Feb. 8, 2024
Very comprehensive lesson on list comprehensions. Thank you, Dan!
Become a Member to join the conversation.
Eriberto on March 12, 2019
Good presentation of list comprehension, helped me a lot understand the concept and the execution sequence.